A sentinel value in C++ is a special value that signifies the end of a data set, often used in loops to control their termination.
Here's a simple example of using a sentinel value in C++:
#include <iostream>
int main() {
int number;
std::cout << "Enter numbers (enter -1 to stop): \n";
while (true) {
std::cin >> number;
if (number == -1) break; // Sentinel value
std::cout << "You entered: " << number << std::endl;
}
return 0;
}
Understanding Sentinel Values
What is a Sentinel Value?
A sentinel value is a special value used in programming to signify the end of a sequence of inputs or to indicate that a specific condition has been met. Commonly known examples of sentinel values include `-1` for terminating temperature readings or `NULL` for pointers. Their primary function in algorithms is to control the flow of loops or act as markers in data structures, allowing the programmer to write cleaner and more efficient code.
Why Use Sentinel Values?
Using sentinel values in your C++ programs can provide significant benefits:
- Efficiency: Sentinel values can streamline processes by removing the necessity for additional variables that specify how many times a loop should execute or when to stop processing data.
- Simplicity: They simplify control flow by consolidating various conditions into a single terminative point, thus enhancing code readability and maintainability.
- Common Patterns: You'll frequently find sentinel values employed in scenarios such as reading input until an end condition is met, traversing linked lists, and terminating recursive functions.
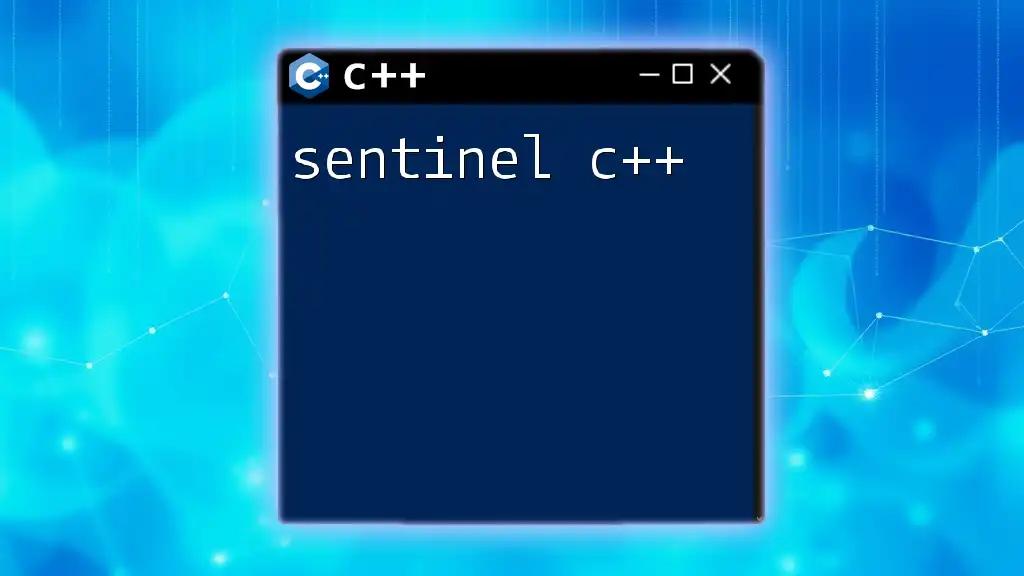
Implementing Sentinel Values in C++
Basic Example with Sentinel Values
One of the simplest applications of a sentinel value is counting user inputs. Below is how you can implement this concept in C++.
#include <iostream>
using namespace std;
int main() {
int number;
int count = 0;
cout << "Enter numbers (enter -1 to stop): " << endl;
while (true) {
cin >> number;
if (number == -1) break; // Sentinel value
count++;
}
cout << "Total numbers entered: " << count << endl;
return 0;
}
In this code, the sentinel value `-1` is used to determine when to stop accepting input. The loop continues to run until the user input is `-1`, effectively counting how many valid numbers have been entered. This eliminates the need for separate termination conditions, simplifying the code.
Advanced Example: Sentinel Values in Arrays
Sentinel values can also manage data collection in arrays effectively. Here’s an example that expands on collecting inputs until a specific condition is met.
#include <iostream>
using namespace std;
int main() {
int arr[100];
int i = 0;
cout << "Enter positive numbers (enter 0 to stop): " << endl;
while (true) {
int num;
cin >> num;
if (num == 0) break; // Sentinel value
arr[i++] = num;
}
// Output the numbers entered
cout << "You entered: ";
for (int j = 0; j < i; j++) {
cout << arr[j] << " ";
}
cout << endl;
return 0;
}
In this example, the sentinel value `0` indicates when the input should cease, allowing us to gather positive numbers into an array without prior knowledge of its size. After the user has finished entering numbers, we can print them all out, demonstrating how effectively the sentinel value simplifies data collection.

Best Practices for Using Sentinel Values
Choosing Proper Sentinel Values
When utilizing sentinel values, it's crucial to select appropriate ones that do not occur in typical data sets. Characteristics of a good sentinel value include being a unique indicator that stands out from valid data. For example, using `-1` for a series of positive temperatures is acceptable since `-1` would naturally not be a temperature in many real-world applications.
Handling Edge Cases
It's essential to anticipate potential issues that might arise from sentinel values. Special cases occur frequently, especially when the data can include negative or zero values, as illustrated in the examples above. Always consider edge cases where the sentinel might inadvertently be accepted as valid input. Testing and clear definition of what constitutes a valid input can mitigate these risks.
Avoiding Ambiguities with Sentinel Values
To prevent confusion around the intent of sentinel values, thoroughly document their use in the code. Comments can clarify the function of the sentinel, guiding future developers or even yourself when revisiting the code. Effective documentation can reduce misinterpretations and keep your codebase easier to manage.

Common Mistakes with Sentinel Values
Overcomplicating Logic with Sentinel Values
One common mistake is introducing unnecessary complexity by complicating the design with multiple sentinel values or convoluted conditions. This can lead to confusion and bugs, as the underlying logic becomes more challenging to follow. Always seek to keep your implementation straightforward, ideally using a single well-defined sentinel value.
Not Handling Sentinel Values Properly
Improper handling of sentinel values, such as failing to check for their presence before processing data, can lead to program crashes or incorrect behavior. Consider this example:
for (int j = 0; j <= i; j++) { // Incorrect: This should be j < i
cout << arr[j] << " ";
}
In the example above, if `i` represents the number of inputs, attempting to print beyond the bounds of the array can result in undefined behavior. Be diligent about your loop conditions, ensuring they respect the boundaries set by your sentinel values.

Conclusion
In summary, \sentinel value in C++ programming is a potent feature that enhances control over loops and data input. By selecting appropriate sentinel values, handling edge cases, and maintaining simplicity, you can write clearer and more efficient programs. Engage with these concepts in your coding practice and explore various scenarios to solidify your understanding. Through experience, you'll not only find the proper use of sentinel values but also discover new ways to optimize your code.

Additional Resources
To continue expanding your knowledge on using sentinel values and related topics, consider exploring online courses, programming books, and tutorials that delve deeper into C++ programming techniques. There are numerous resources available for you to enhance your understanding and skills.
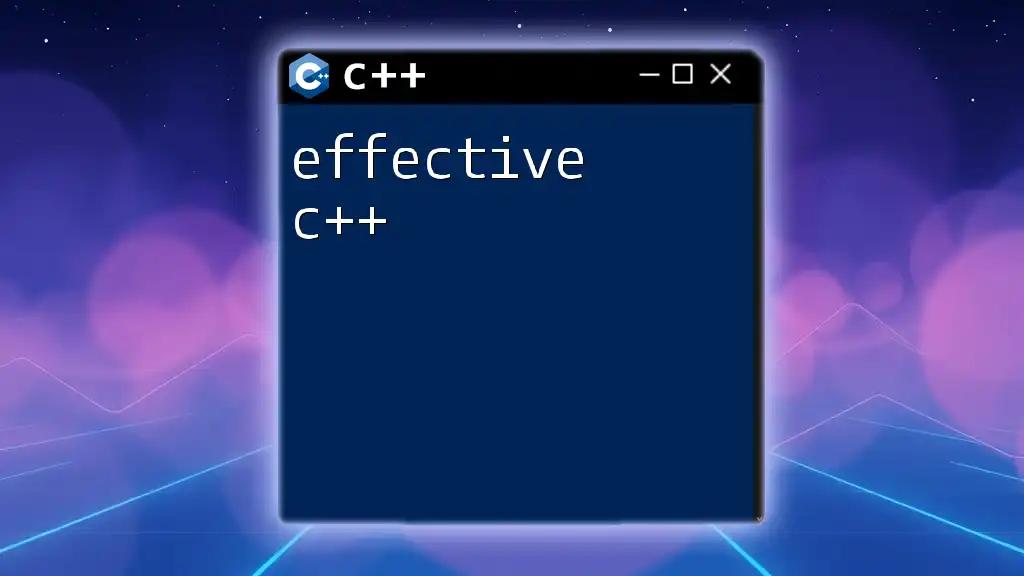
FAQs About Sentinel Values
-
Are there situations where sentinel values should not be used? Yes, in contexts where input can naturally include the sentinel value (e.g., `0` could be valid in some calculations), it is better to consider alternatives like maintaining a separate variable for count.
-
Can multiple sentinel values be used? While it is possible to use multiple sentinel values, it typically complicates the logic. Aim for clear, predictable structures that minimize confusion.