The `erase` function in C++ is used to remove elements from a list (or other standard container) by specifying either an iterator pointing to the element to erase or a range of elements.
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
myList.erase(myList.begin()); // Erases the first element
// To erase a range: myList.erase(myList.begin(), myList.end()); // Erases all elements
for (int n : myList) {
std::cout << n << ' '; // Output: 2 3 4 5
}
return 0;
}
Understanding C++ Lists
What is a List in C++?
In C++, a list is a part of the Standard Template Library (STL) and is represented by the `std::list` class. It is a doubly linked list that allows for efficient insertion and deletion of elements from any position. Unlike `std::vector`, which must allocate contiguous memory, `std::list` can allocate memory independently for each element, making it suitable for scenarios that require frequent insertions and deletions.
Key Differences:
- Memory Allocation: `std::vector` uses contiguous memory, while `std::list` does not.
- Access Time: Accessing elements by index in `std::vector` is O(1), while in `std::list`, it is O(n) due to the linked structure.
Use Cases: Use `std::list` when:
- Frequent insertions and deletions are required.
- You do not need to access elements by their indices often.
Importance of Erasing Elements
Erasing elements from a list is crucial for:
- Managing memory effectively by removing unused or unneeded elements.
- Maintaining the integrity of data. For instance, removing duplicates or outdated entries.
Before removing an element, consider the performance impacts. Erasing elements in a list is generally O(n) as it may require traversing the list to locate the element.
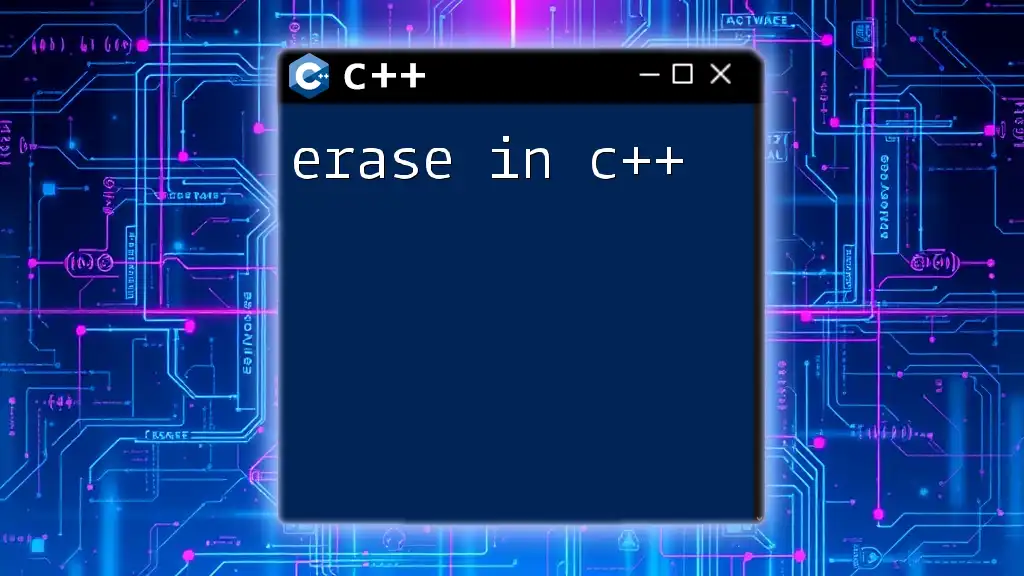
The Basics of Erasing Elements in C++ Lists
Syntax and Methods
The primary method for removing elements from a `std::list` is `erase()`, which modifies the list in place. The basic syntax is:
list.erase(iterator position);
Example:
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
auto it = myList.begin();
myList.erase(it); // Erases the first element
// Output the list
for (int n : myList) { std::cout << n << " "; } // Output: 2 3 4 5
}
Erasing Elements by Value vs. Iterator
You can erase elements either by iterator or by value. When erasing by value, you typically use the `remove()` method followed by `erase()` to ensure the element is removed completely from the list.
Example:
myList.remove(3); // Removes the value '3'
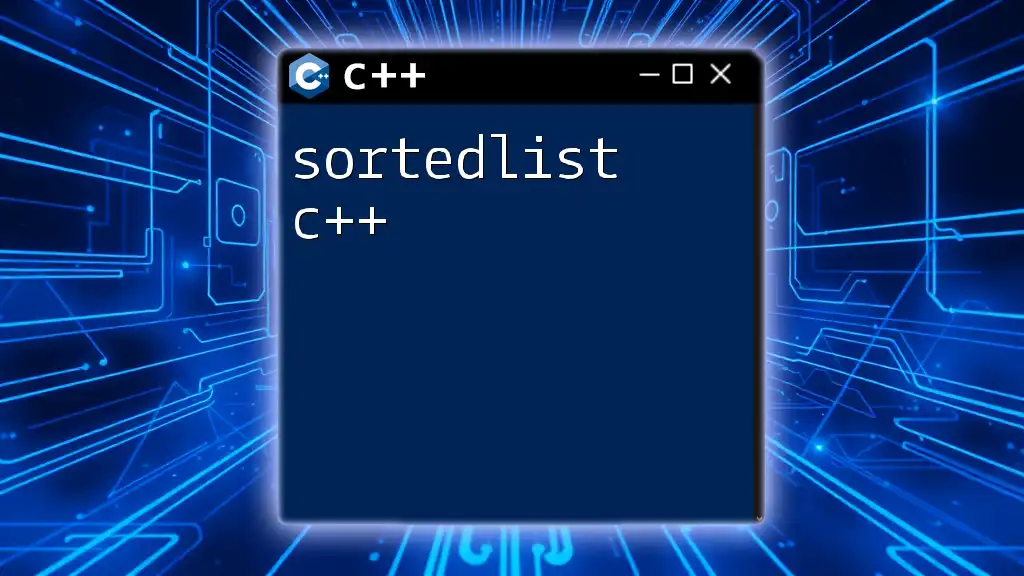
Detailed Usage of `erase()`
Erasing a Single Element
To erase a single element using an iterator, first, get the iterator to the desired element, then call `erase()`.
Example:
auto it = myList.begin();
std::advance(it, 2); // Move iterator to the third element
myList.erase(it); // Erase the element at the third position
Erasing a Range of Elements
You can also erase a range of elements using iterators. This is particularly useful when you want to remove multiple contiguous elements in one operation.
Using `erase()` with Two Iterators
The syntax for removing a range is:
list.erase(iterator first, iterator last);
Example:
myList.erase(myList.begin(), myList.end()); // Erases all elements
Conditional Erasure: Using `remove_if()`
C++ provides a powerful algorithm, `remove_if()`, which can be used in combination with `erase()` to conditionally remove elements.
The syntax generally looks like this:
list.remove_if(condition);
Example:
myList.remove_if([](int n) { return n % 2 == 0; }); // Erases all even numbers
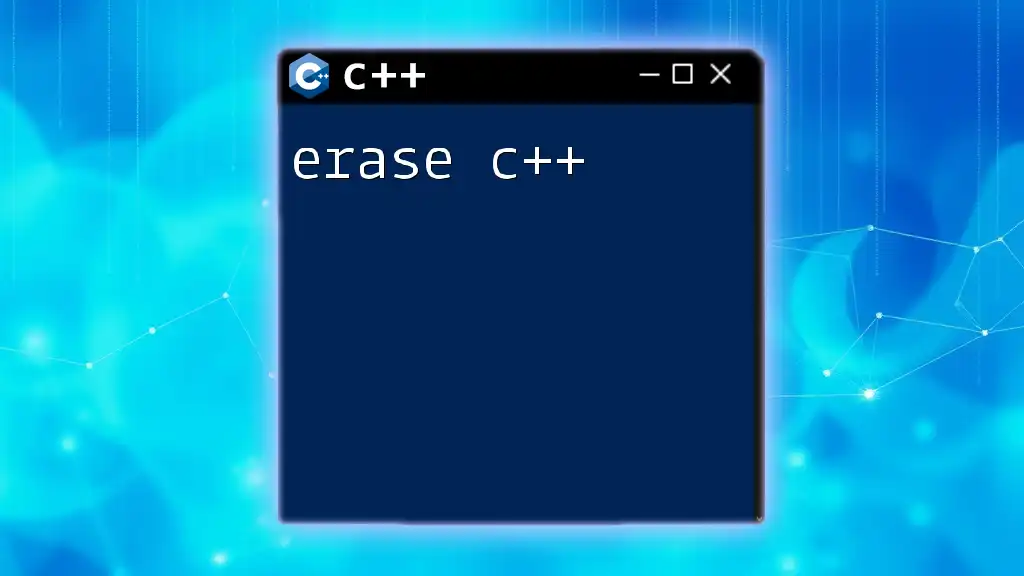
Performance Considerations
Time Complexity of Erasing Elements
Understanding the time complexity associated with `erase()` operations is essential for writing efficient code. Generally speaking:
- Erasing a single element: O(1) if you have the iterator, O(n) if you need to search for the element.
- Erasing a range of elements: O(n) for locating the elements plus O(m) for the erase operation, where m is the number of elements being erased.
Best Practices for Erasing Elements
To ensure optimal performance when erasing elements, consider the following recommendations:
- Prefer using iterators for erase operations when possible, especially in loops.
- Use `remove_if()` when applicable to simplify the removal of elements based on conditions, thus reducing the need for multiple scans of the list.
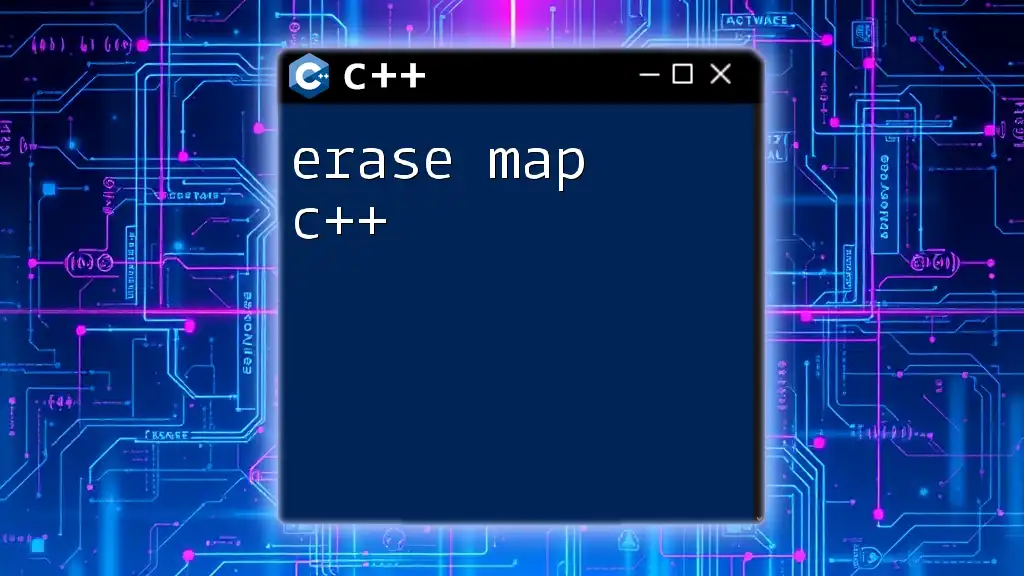
Common Errors and Troubleshooting
Avoiding Common Mistakes
One common mistake when working with `erase()` is dereferencing invalidated iterators. After an `erase()` call, any iterators pointing to the erased element or elements after it become invalid.
Tip: Always update your iterator after an `erase()` operation. It’s a good practice to move it to the next valid element using the return value of `erase()`. For instance:
it = myList.erase(it); // Erase and update the iterator
Debugging Erase Operations
If elements are not being removed as expected:
- Check if you are using the correct iterator.
- Ensure that you correctly handle the range or condition specified for removal.
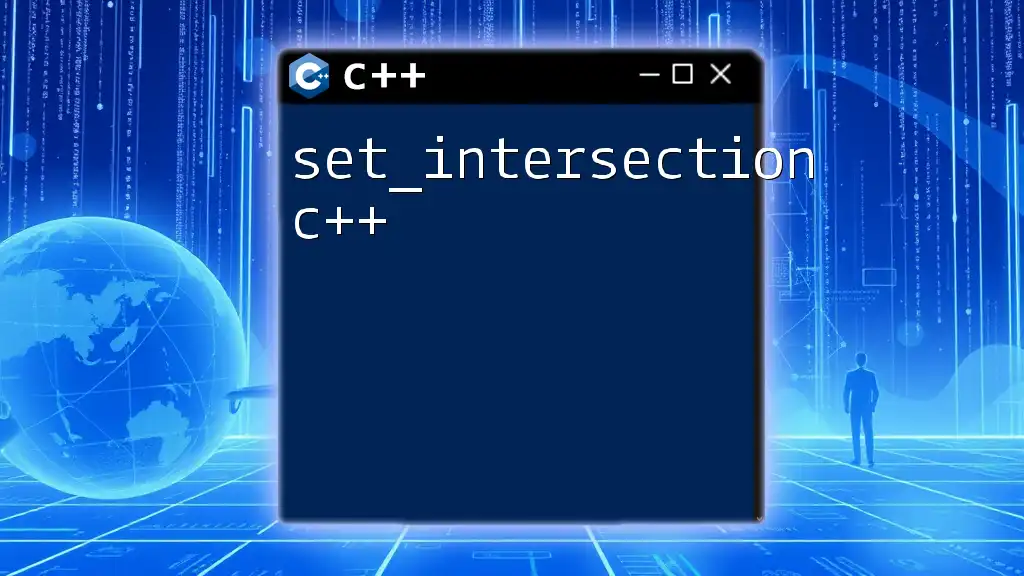
Conclusion
In summary, mastering the usage of the `erase()` method is vital when working with lists in C++. Understanding various methods of erasing elements—be it single elements, ranges, or conditional removals—will enhance your efficiency in managing lists. Always keep performance considerations in mind to ensure that your applications remain fast and responsive.
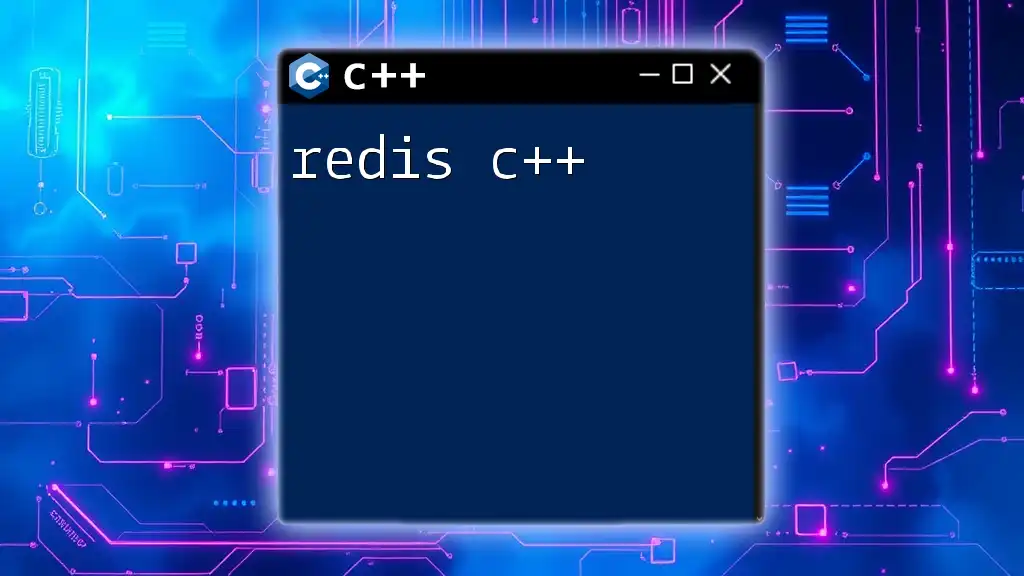
Further Resources
For more information and guidance on `std::list` and other STL containers, refer to the following:
- Official C++ documentation on [std::list](https://en.cppreference.com/w/cpp/container/list).
- Online tutorials and communities focused on C++ programming and the STL.