Reversing a linked list in C++ involves altering the pointers of the nodes so that they point to the previous node instead of the next one, resulting in the list's order being flipped.
struct Node {
int data;
Node* next;
};
Node* reverseList(Node* head) {
Node* prev = nullptr;
Node* current = head;
Node* next = nullptr;
while (current != nullptr) {
next = current->next; // Store next node
current->next = prev; // Reverse current node's pointer
prev = current; // Move pointers one position forward
current = next;
}
return prev; // New head of the reversed list
}
What is a Linked List?
A linked list is a linear data structure that consists of a sequence of nodes, where each node contains data and a pointer (or reference) to the next node in the sequence. Linked lists are dynamic in nature, meaning they can grow and shrink in size as needed, making them flexible compared to arrays.
Importance of Reversing a Linked List
Reversing a linked list is a common operation used in various algorithms and data manipulation tasks. Some real-world applications include:
- Undo functionality in applications, where previous states are held in a linked list-like structure.
- Data structures like stacks, which inherently require reversal of items.
- Algorithms that depend on accessing elements in reverse order for efficiency.
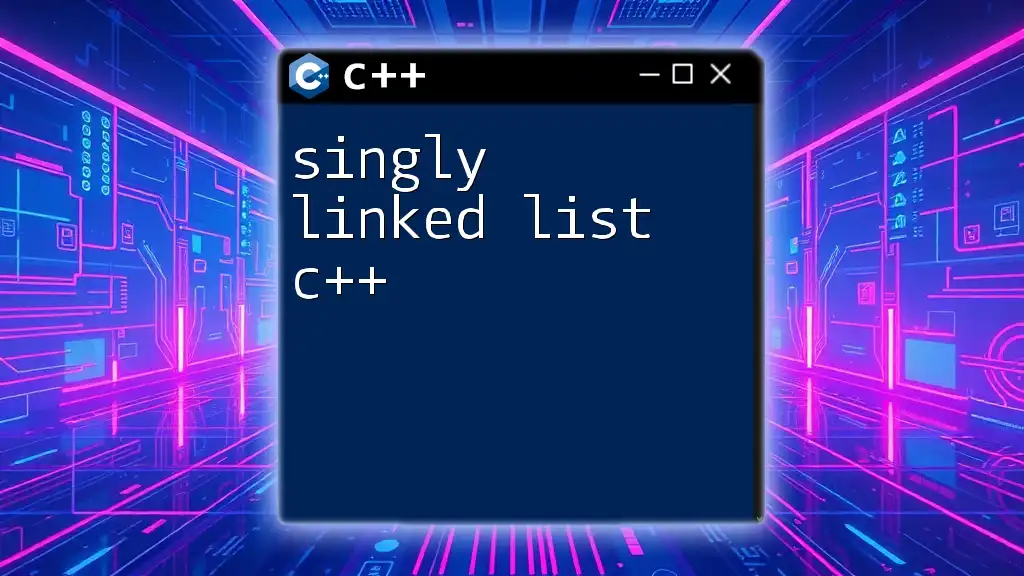
Understanding the Structure
Node Structure in C++
Before diving into the reversal process, it's crucial to understand the fundamental building block of a linked list: the node. A node typically contains:
- A data field to hold a value.
- A pointer (or reference) to the next node.
Here’s a simple definition of a node in C++:
struct Node {
int data;
Node* next;
};
Creating a Singly Linked List
To manipulate a linked list, we often need to create and manipulate a list structure. Below is a simple function to initialize a linked list by inserting new nodes at the front:
void insert(Node*& head, int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = head;
head = newNode;
}
This approach keeps the most recently added node at the head, making it easy to access.
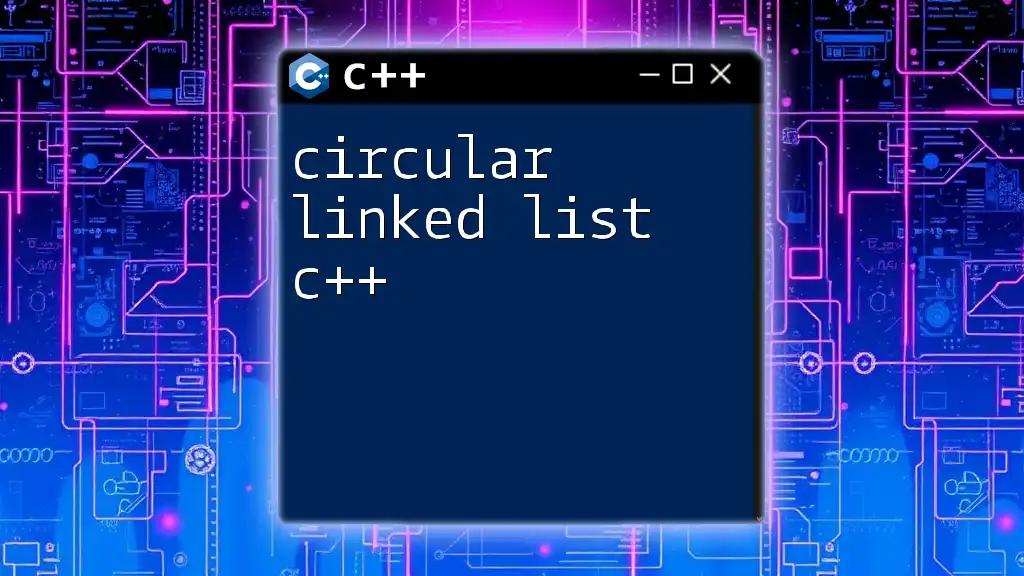
How to Reverse a Linked List in C++
Overview of the Reversal Process
The fundamental concept behind reversing a linked list is to reorient the pointers so that each node points to its previous node rather than its next one. This requires careful manipulation of pointers to maintain the integrity of the list.
Iterative Approach to Reverse a Linked List
The iterative approach for reversing a linked list is straightforward and efficient. Here’s how it works:
- Initialize three pointers: `prev`, `current`, and `next`.
- Traverse through the list, and for each node:
- Temporarily store the next node.
- Reverse the current node's pointer to point to the previous node.
- Move the `prev` and `current` pointers one step forward.
Here’s the code implementing this approach:
Node* reverseIterative(Node* head) {
Node* prev = nullptr;
Node* current = head;
Node* next = nullptr;
while (current != nullptr) {
next = current->next; // Store next node
current->next = prev; // Reverse the current node's pointer
prev = current; // Move prev and current one step forward
current = next;
}
return prev; // New head of the reversed list
}
Detailed Explanation of the Iterative Method
In this method, the `current` pointer traverses the list, while the `prev` pointer gradually builds the reversed linked list. Once `current` becomes `nullptr`, `prev` points to the new head of the reversed list.
- Time Complexity: O(n) - where n is the number of nodes in the list, as we make a single pass through it.
- Space Complexity: O(1) - since only a constant amount of extra space is used for the pointers.
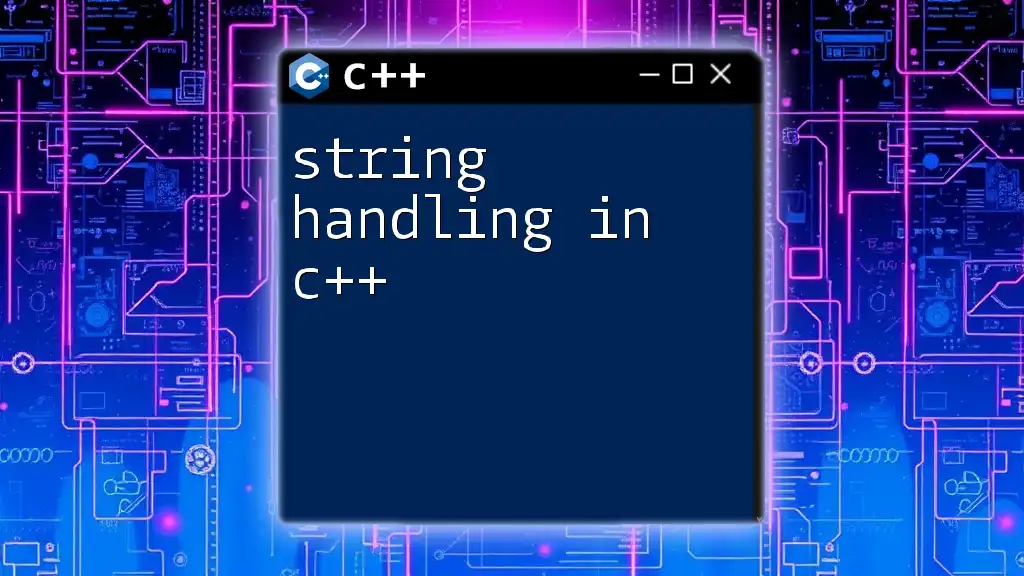
Alternative Method: Recursive Approach
Understanding the Recursive Approach
The recursive method for reversing a linked list leverages the call stack to handle pointer manipulation. Each recursive call processes one node until it reaches the end of the list, returning the new head of the reversed list. This elegant solution, however, incurs additional memory overhead due to the call stack.
Code Example for Recursive Reversal
Here's how to implement the recursive approach:
Node* reverseRecursive(Node* head) {
if (head == nullptr || head->next == nullptr) {
return head; // Base case: empty or single node
}
Node* newHead = reverseRecursive(head->next);
head->next->next = head; // Reverse the current node's pointer
head->next = nullptr; // Set the current node's next to null
return newHead; // Return new head of reversed list
}
Explanation of the Recursive Method
- The base case checks if the list is empty or contains only one node, in which case it simply returns the head.
- The recursive call processes the rest of the list while the current node's pointer is then redirected to point to itself, effectively reversing the direction.
- Finally, the method returns the new head of the list.
- Time Complexity: O(n) - due to traversing each node once.
- Space Complexity: O(n) - due to the call stack.
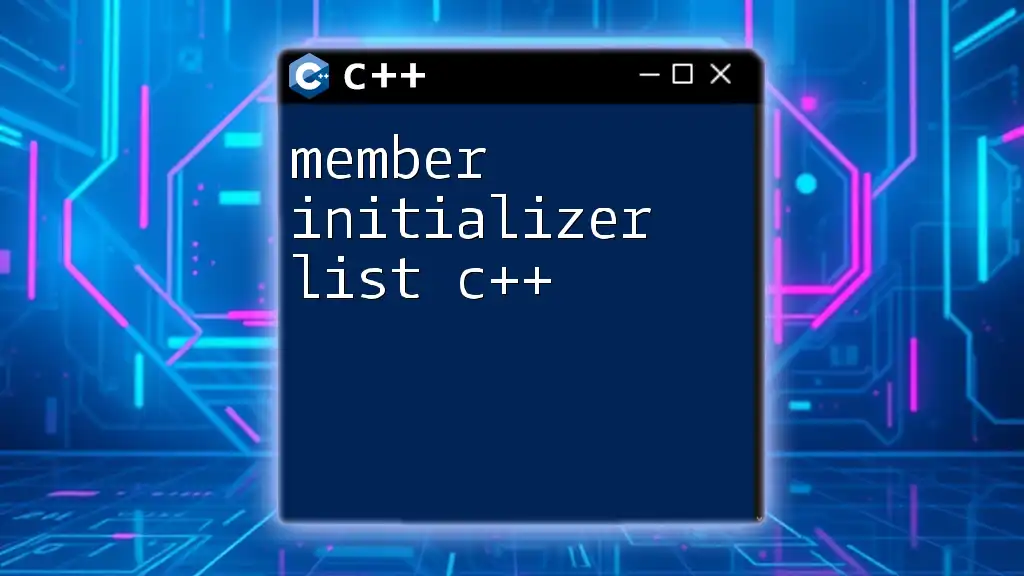
Practical Examples
Example: Reversing and Displaying a Linked List
Combining the insertion, reversal, and display functionalities into one program is an effective way to visualize the process. Below is a utility function to display the linked list:
void displayList(Node* head) {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
Full Program Example
Here is a complete program demonstrating the insertion, reversal, and display functionality:
int main() {
Node* head = nullptr;
insert(head, 1);
insert(head, 2);
insert(head, 3);
std::cout << "Original List: ";
displayList(head);
head = reverseIterative(head);
std::cout << "Reversed List (Iterative): ";
displayList(head);
head = reverseRecursive(head);
std::cout << "Reversed Back (Recursive): ";
displayList(head);
return 0;
}
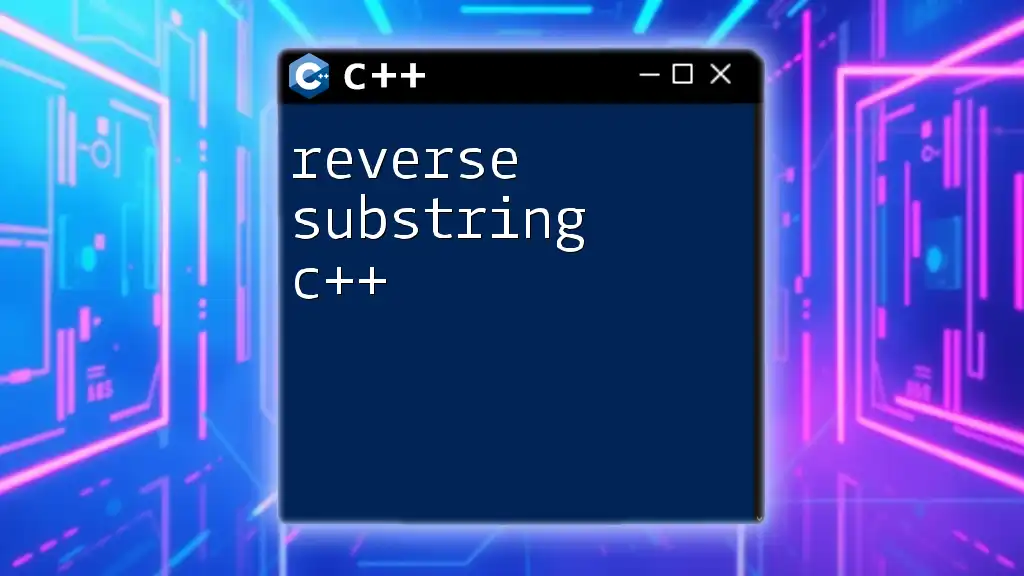
Conclusion
Reversing a linked list in C++ is a crucial skill for anyone looking to understand data structures better. Understanding both iterative and recursive methods provides a robust toolbox for handling linked lists in various programming scenarios.
By mastering these techniques, you can use them in more complex algorithms and applications, enhancing your software development skills. Keep practicing with these examples and consider exploring further reading on data structures and algorithms!