In C++, you can initialize an object using its constructor, which sets the values of its member variables at the time of creation.
class MyClass {
public:
int x;
MyClass(int val) : x(val) {} // Constructor for initialization
};
MyClass obj(10); // Initializes obj with x set to 10
What is Object Initialization in C++?
Object initialization refers to the process of assigning initial values to an object's attributes at the time of its creation. This is a crucial aspect of object-oriented programming, as it ensures that every instance of a class starts its life in a valid state. It is vital to note that there is a difference between object initialization and simple declaration; while declaration creates the object in memory, initialization gives it meaningful values and settings.
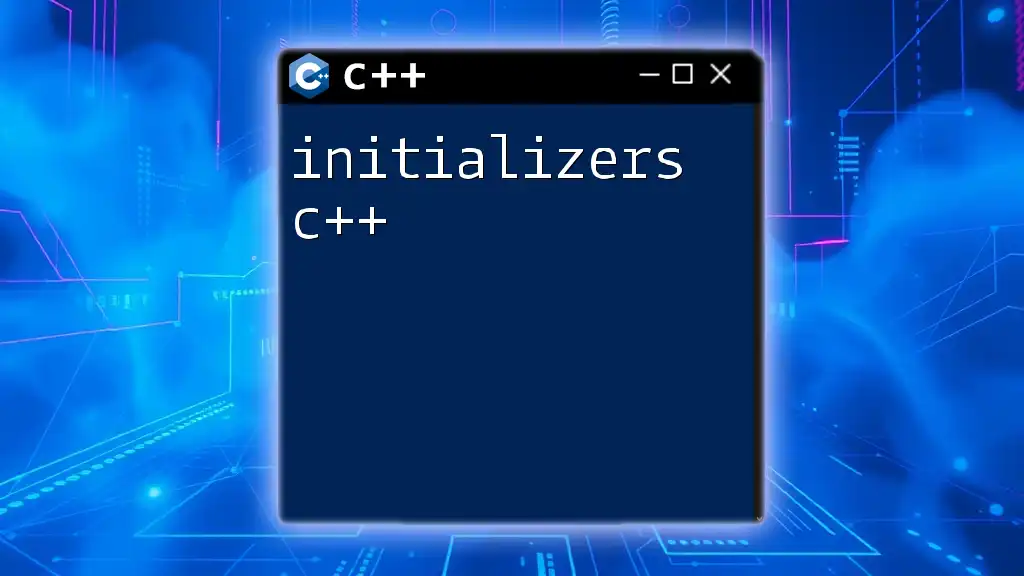
Different Methods to Initialize Objects in C++
C++ provides several ways to initialize objects, each with its own syntax and use cases. Let's explore some of the most common methods available.
Default Initialization
In default initialization, an object is created without any initialization parameters. This method is often employed when a class has a default constructor.
For example:
class MyClass {
public:
int x;
MyClass() {} // default constructor
};
MyClass obj; // Default initialization
In this example, the member variable `x` remains uninitialized until explicitly set later.
Value Initialization
Value initialization occurs when an object is created and initialized using the constructor, which can set member variables to a default value automatically. This is primarily used in contexts where you want to ensure no garbage values remain.
Example:
MyClass obj = MyClass(); // value initialization
Here, an instance of `MyClass` is created, and the default constructor is invoked, initializing `x` to zero if defined.
Copy Initialization
Copy initialization makes use of an existing object to initialize a new object of the same class type. This typically requires the class to define a copy constructor.
Example:
MyClass obj1;
MyClass obj2 = obj1; // Copy initialization
In this example, `obj2` becomes a copy of `obj1`, allowing for values and the state to be replicated.
Direct Initialization
Using direct initialization allows you to invoke a constructor directly at the time of object declaration. This method provides clear expressions of intent when you wish to initialize with specific parameters.
Example:
MyClass obj(/* constructor parameters */); // Direct initialization
In this case, you can pass parameters to the constructor directly, allowing for immediate setup of instance attributes.
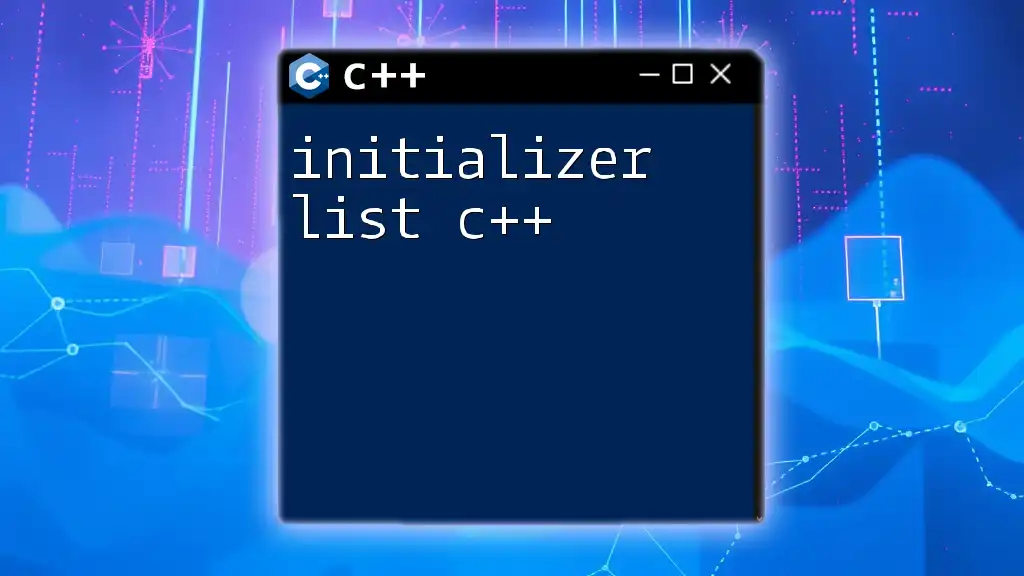
C++ Object Initialization with Constructors
Understanding Constructors
A constructor is a special member function that is automatically called when an object of a class is created. Constructors ensure that an object is set up correctly prior to its use.
There are various types of constructors, including:
- Default constructors: Take no parameters.
- Parameterized constructors: Accept parameters to customize the object's initialization.
- Copy constructors: Initialize a new object as a copy of an existing object.
Using Constructors for Object Initialization
Here's how to implement constructors for effective object initialization:
Default Constructor Example:
class MyClass {
public:
int x;
MyClass() : x(0) {} // initializes x to 0
};
MyClass obj; // uses default constructor
In the code above, invoking `MyClass()` sets `x` to `0` upon creation.
Parameterized Constructor Example:
class MyClass {
public:
int x;
MyClass(int val) : x(val) {} // parameterized constructor
};
MyClass obj(10); // initializes x to 10
This constructor initializes `x` with a user-defined value, allowing for dynamic setups of attributes.
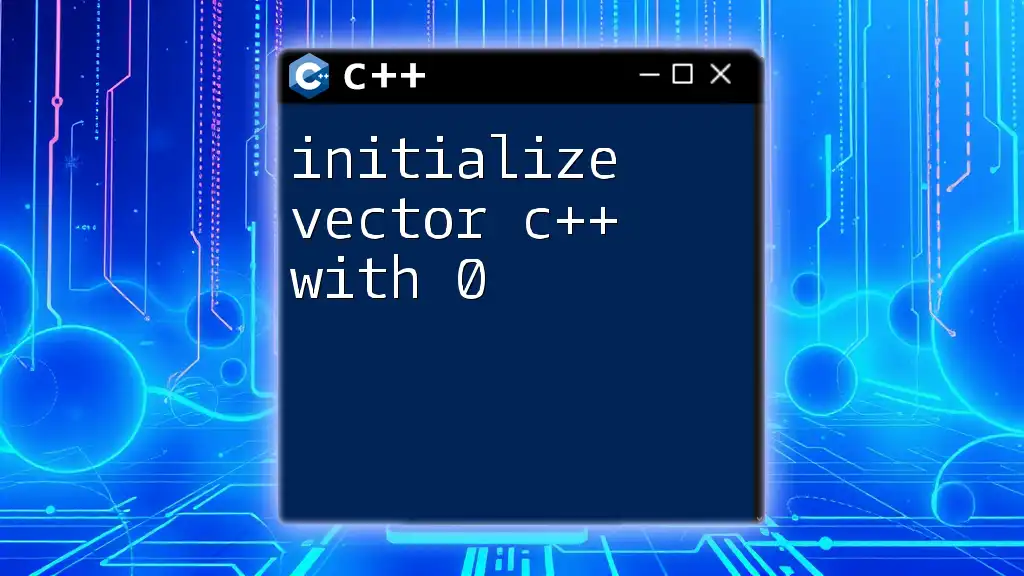
C++ Object Initialization with Member Initializer Lists
What are Member Initializer Lists?
Member initializer lists are techniques used in constructors to initialize data members directly. They are often preferred for efficiency, as they can eliminate the need for a default constructor followed by assignment.
Using Member Initializer Lists
Consider the following code example:
class MyClass {
public:
int x;
MyClass(int val) : x(val) {} // using member initializer list
};
MyClass obj(5); // x is initialized to 5
Here, the member variable `x` is initialized directly through the initializer list with the provided value, making the operation potentially more performance-friendly and cleaner.
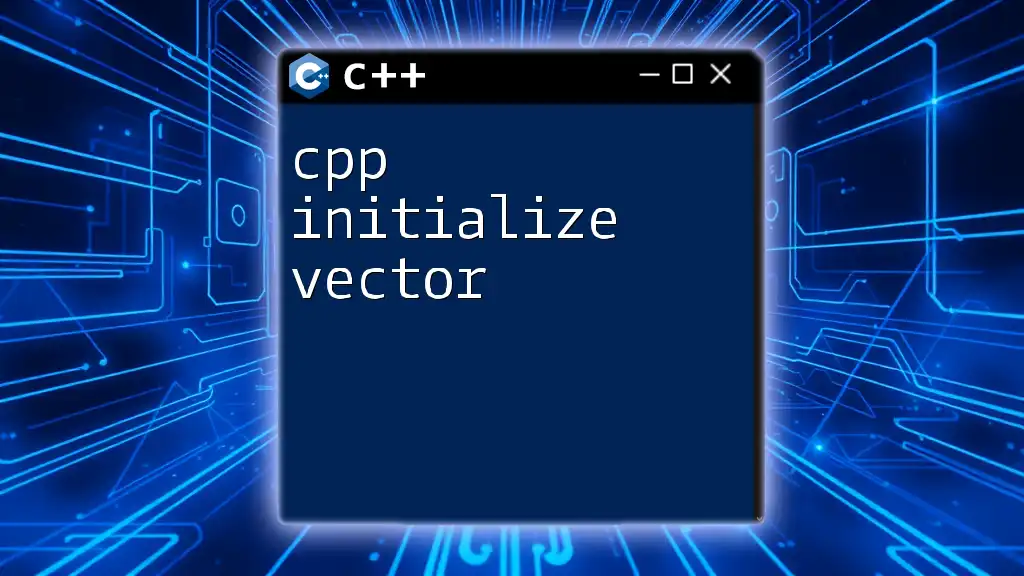
Special Cases in Object Initialization
Dynamic Object Initialization
When you need to create objects at runtime and want to manage the lifetime dynamically, you can use dynamic initialization through pointers. This allows you to allocate memory for objects as needed.
Example:
MyClass* obj = new MyClass(); // dynamic initialization
After creating an object in this manner, remember to `delete` it to prevent memory leaks.
Initializing Arrays of Objects
You can also create arrays of objects. Each object in the array can be initialized using a constructor or default values.
Example:
MyClass arr[3]; // array of MyClass objects
In this case, each member of the array `arr` will use the default constructor, leading to each `x` being uninitialized unless specified otherwise.
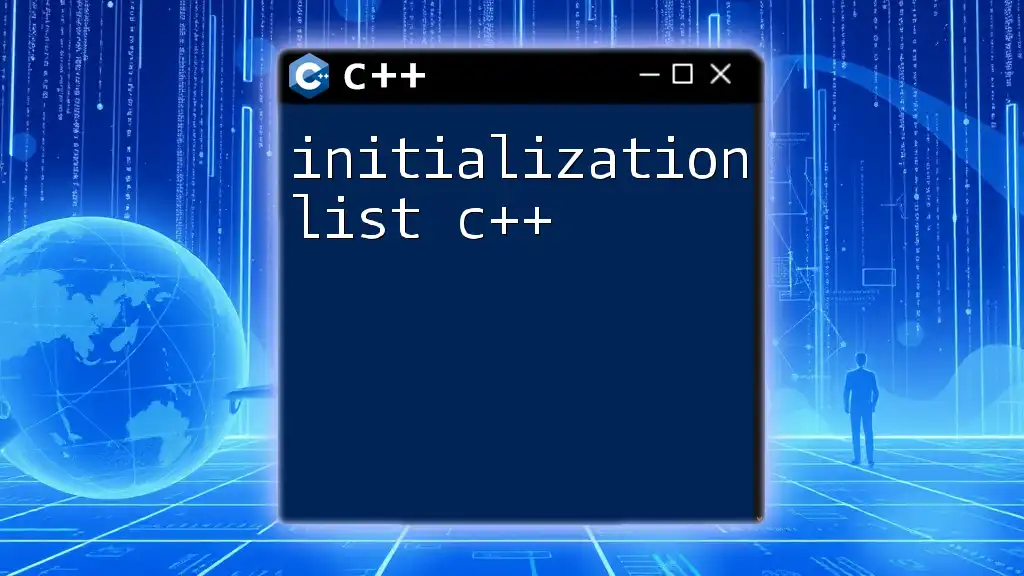
Common Mistakes to Avoid in Object Initialization
While working with object initialization, several common pitfalls can lead to unexpected results or bugs:
- Not using constructors to initialize objects properly, which can lead to uninitialized members.
- Forgetting to `delete` dynamically allocated objects, resulting in memory leaks.
- Misinitializing arrays of objects by assuming they are automatically initialized to zero.
Debugging tips involve carefully reviewing constructors and ensuring initialization procedures are appropriately followed.
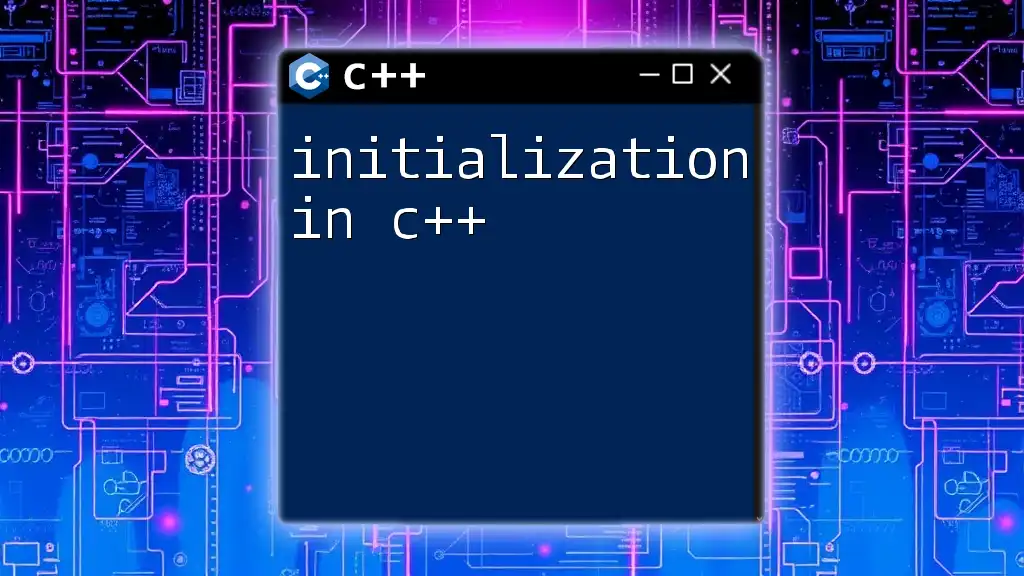
Conclusion
In summary, understanding how to initialize an object in C++ is crucial for ensuring program stability and correctness. By leveraging various initialization methods such as default, value, copy, and direct initialization—as well as utilizing constructors and initializer lists—you can effectively manage the setup of your objects. Consider your specific requirements and the clarity of your code when choosing an initialization method.
By grasping these principles, you'll improve your object-oriented programming skills and overall proficiency in C++. For more in-depth exploration of these topics, consider exploring additional resources or participating in courses that delve deeper into C++.