To initialize a 2D vector in C++, you can use nested vectors to define the number of rows and columns, as shown in the following code snippet:
#include <vector>
int main() {
std::vector<std::vector<int>> vec(3, std::vector<int>(4));
return 0;
}
Understanding 2D Vectors
What is a 2D Vector?
A 2D vector in C++ is essentially a vector of vectors, allowing you to create a dynamic array that can grow as needed. This structure is particularly useful when representing data in a grid-like format, such as matrices or game boards.
Unlike a 1D vector which holds a single list of elements, a 2D vector organizes data in rows and columns, providing a more intuitive way to manage two-dimensional data structures.
Why Use 2D Vectors?
Utilizing 2D vectors has several advantages:
- Dynamic Sizing: Unlike static arrays, 2D vectors can adjust their size to fit the needs of your application.
- Flexibility: You can easily add or remove rows and columns, providing much more control over your data structure.
- Easy Access: Accessing elements is straightforward and intuitive, which simplifies both reading and writing code.
Practical applications of 2D vectors include:
- Representing game maps or grids in game development.
- Storing mathematical matrices for computations or transformations.
- Managing multi-dimensional datasets in scientific computing.
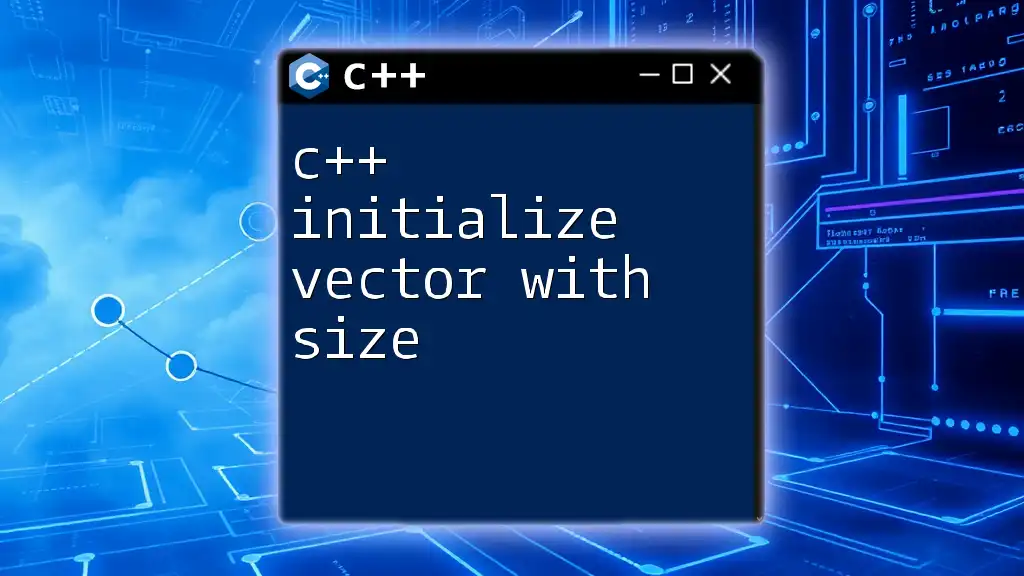
C++ 2D Vector Initialization Techniques
Using Nested Vectors
Syntax and Structure
One of the most common ways to initialize a 2D vector in C++ is by using nested vectors. The outer vector represents rows, while each inner vector represents columns. The syntax for initializing a 2D vector is as follows:
std::vector<std::vector<int>> vec(3, std::vector<int>(4, 0));
In this example:
- The outer vector `vec` contains 3 elements.
- Each inner vector contains 4 elements initialized to 0.
Dynamic Initialization
You can also initialize a 2D vector dynamically based on user input. For instance:
int rows, cols;
std::cin >> rows >> cols;
std::vector<std::vector<int>> vec(rows, std::vector<int>(cols));
In this case, the number of rows and columns is determined at runtime, making your program adaptable to varying inputs.
Using `std::array`
Overview of `std::array`
`std::array` is a fixed-size container, which makes it a good choice when you know the sizes ahead of time. The primary difference between `std::array` and `std::vector` is that the size of `std::array` cannot be changed after initialization.
When to Choose `std::array`
`std::array` is often preferred for performance-critical applications where the array size is known at compile time. Here’s how you can initialize a 2D array using `std::array`:
std::array<std::array<int, 4>, 3> arr = {{{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}}};
This creates a 3x4 array, explicitly initializing each element. Remember, unlike with vectors, the dimensions must be constant and defined at compile time.
Initializing with Fill Values
Using the Fill Constructor
Another straightforward method for initializing 2D vectors is using the fill constructor, which allows you to initialize all elements to a specific value. For example:
std::vector<std::vector<int>> vec(3, std::vector<int>(4, 1));
In this example, every element of the vector is set to 1. This is useful when a default initialization value is necessary.
Initialize 2D Vector with Custom Objects
Defining a Custom Class
When working with more complex data structures, you might want to use objects in your vectors. Here’s how you can create a custom class and initialize a 2D vector with its objects:
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
std::vector<std::vector<Point>> points(2, std::vector<Point>(2, Point(0, 0)));
In this example, we define a Point class with two integer attributes (`x` and `y`). We then create a 2D vector that contains 2 rows and 2 columns of Point objects, each initialized with 0.
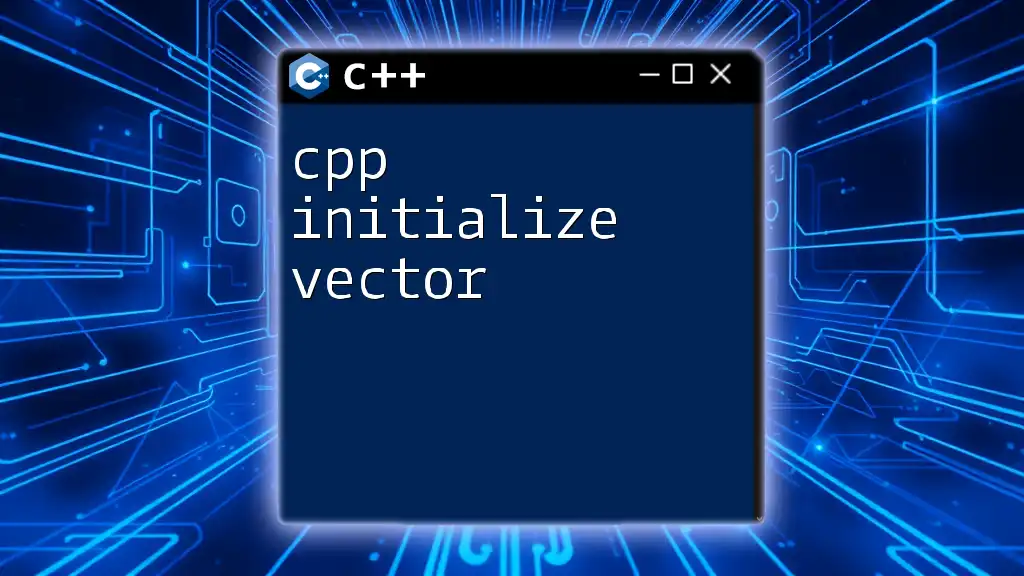
Accessing and Modifying Elements in a 2D Vector
Accessing Elements
Accessing elements in a 2D vector can be achieved using either the `[]` operator or the `at()` method. For example:
int value = vec[1][2]; // Accessing value at row 1, column 2
This retrieves the element located at row 1 and column 2. The `at()` method provides bounds checking, throwing an exception if the indices are out of range:
int value = vec.at(1).at(2); // Safe access with bounds checking
Modifying Elements
Changing the elements within a 2D vector is equally straightforward. You can modify an existing value by simply assigning a new value as follows:
vec[0][0] = 10; // Modifying value at row 0, column 0
This updates the value located at the specified row and column with 10.
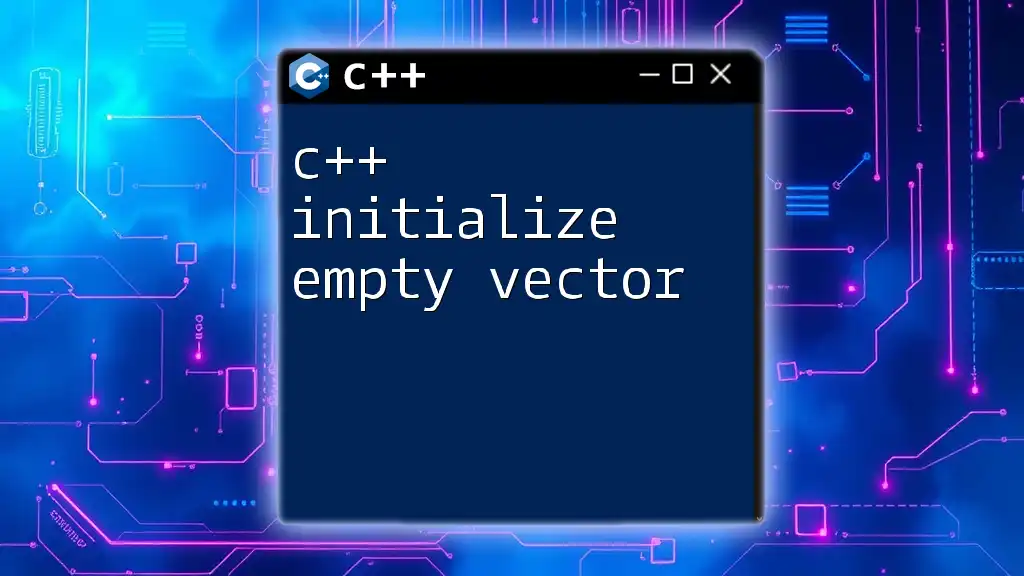
Potential Errors and Debugging
Common Errors in 2D Vector Initialization
Some common pitfalls when initializing or accessing elements of a 2D vector include:
- Out of Bounds: Attempting to access elements outside of the initialized rows or columns.
- Uninitialized Vectors: Not initializing the inner vectors can lead to accessing invalid memory locations.
Debugging Techniques
To effectively debug issues:
- Utilize debugging tools like gdb to step through your code.
- Implement logging or `cout` statements to track the flow of your program and the values of variables at key points.
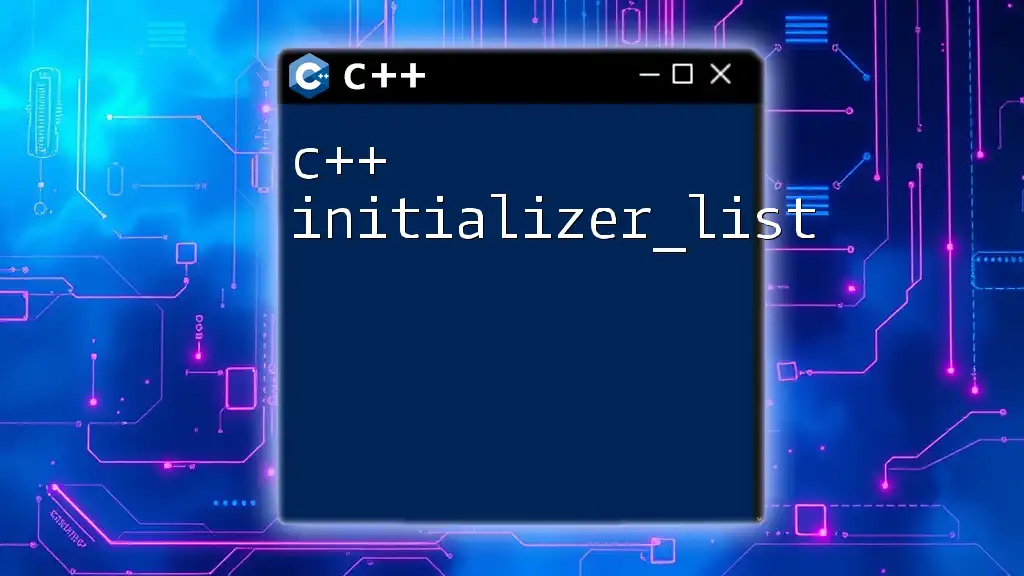
Conclusion
Understanding how to initialize 2D vectors in C++ is crucial for efficient data management in numerous applications, from simple computations to complex game programming. Mastering these techniques will significantly enhance your C++ capabilities, facilitating the creation of robust and adaptable programs.
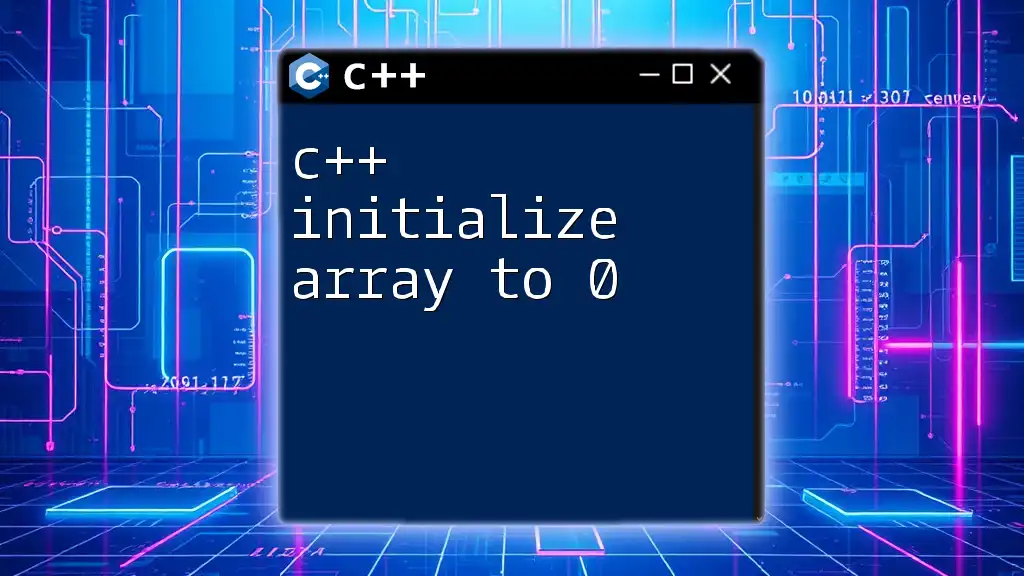
Additional Resources
For those looking to further explore C++, various resources are available, including comprehensive books, online courses, and community forums. Exploring frameworks and libraries designed for C++ can also expand your understanding and practical skills.
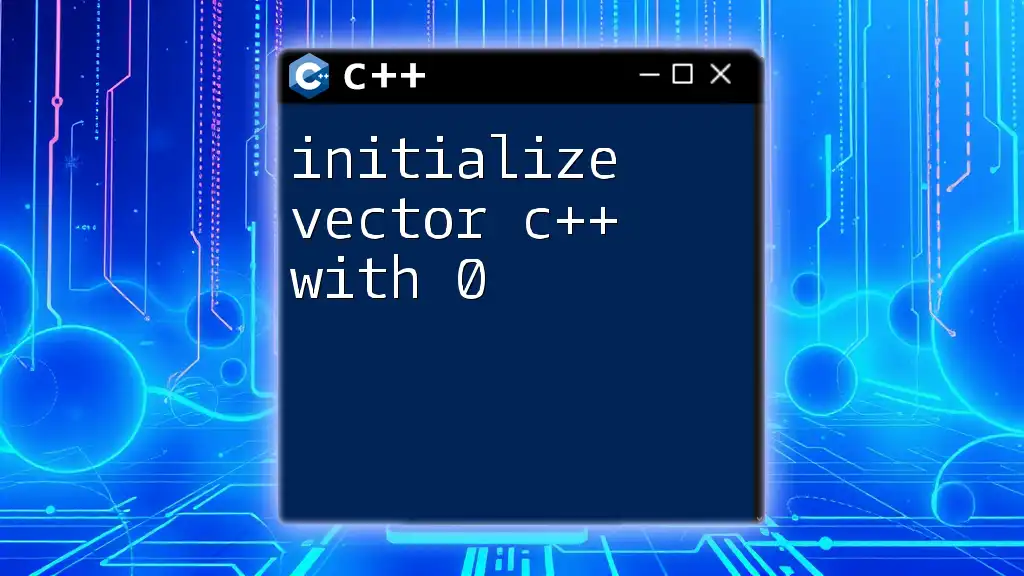
FAQs
What is the maximum size of a 2D vector in C++?
The maximum size of a 2D vector is limited by the available system memory. C++ does not impose a specific size limit, but practical limitations exist based on the implementation and environment.
Can you initialize a 2D vector without specifying dimensions?
Yes, you can initialize a 2D vector without specific dimensions using a simpler syntax, allowing it to dynamically grow. However, it's generally beneficial to set initial dimensions for a clearer data structure.
How do you delete a 2D vector in C++?
To delete a 2D vector, you can simply rely on the automatic memory management of C++. When a vector goes out of scope, or you explicitly use `vec.clear()`, its elements are automatically deallocated.