A C++ visualizer is a tool that allows users to visually represent and analyze the execution of C++ code, helping to enhance understanding of how code flows and operates.
Here’s a simple code snippet that demonstrates the use of a C++ visualizer to showcase a basic sorting algorithm:
#include <iostream>
#include <vector>
void bubbleSort(std::vector<int>& arr) {
int n = arr.size();
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
std::swap(arr[j], arr[j + 1]);
}
}
}
}
int main() {
std::vector<int> data = {64, 34, 25, 12, 22, 11, 90};
bubbleSort(data);
std::cout << "Sorted array: ";
for (int num : data) {
std::cout << num << " ";
}
return 0;
}
Overview of C++ Visualizers
What is a C++ Visualizer?
A C++ visualizer is a tool designed to help developers understand, analyze, and debug their C++ code through graphical representations. It provides visual feedback on code structure, execution flow, and data interactions, which can be significantly more impactful than reading lines of code. The primary purpose of a C++ visualizer is to simplify the understanding of complex coding patterns and relationships within the code.
Importance
Using a C++ visualizer can greatly enhance the coding experience by allowing programmers to visualize what the code is doing in real-time. This is especially important when dealing with complex algorithms, data structures, or when debugging intricate issues. Visualizers can lead to faster and more efficient coding, as they often help developers catch errors early and understand code behavior better.
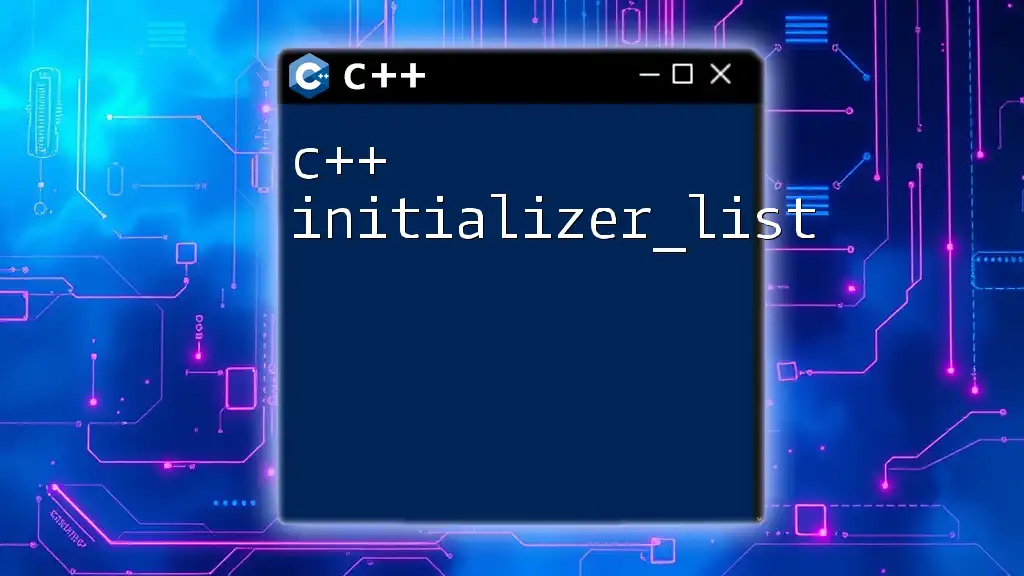
Benefits of Using a C++ Visualizer
Enhanced Understanding of Code
When programmers are faced with complex coding logic, a C++ visualizer can provide clarity. By visualizing the relationships between different components, such as classes, functions, and data structures, developers can better grasp how everything fits together. For instance, when analyzing a recursive function, seeing the recursive calls represented visually can help developers instantly recognize issues with base cases or termination conditions.
Moreover, visualizers greatly assist in the debugging process. They allow developers to see the state of variables at different points in execution. For example, when inspecting the following code snippet:
int main() {
int x = 10;
int y = 20;
int sum = x + y;
return 0;
}
A visualizer can show the values of `x`, `y`, and `sum` in real-time, making it much easier to track down logical errors or unexpected behavior.
Learning and Teaching Tool
For beginners, C++ visualizers serve as an excellent educational resource. They facilitate a hands-on learning experience where learners can see the immediate impacts of their code changes. This interactivity fosters better retention and understanding of programming concepts.
In educational settings, instructors can leverage visualizers as teaching aids, helping students visualize flow, syntax, and data structures. This approach can make learning much more engaging and memorable.
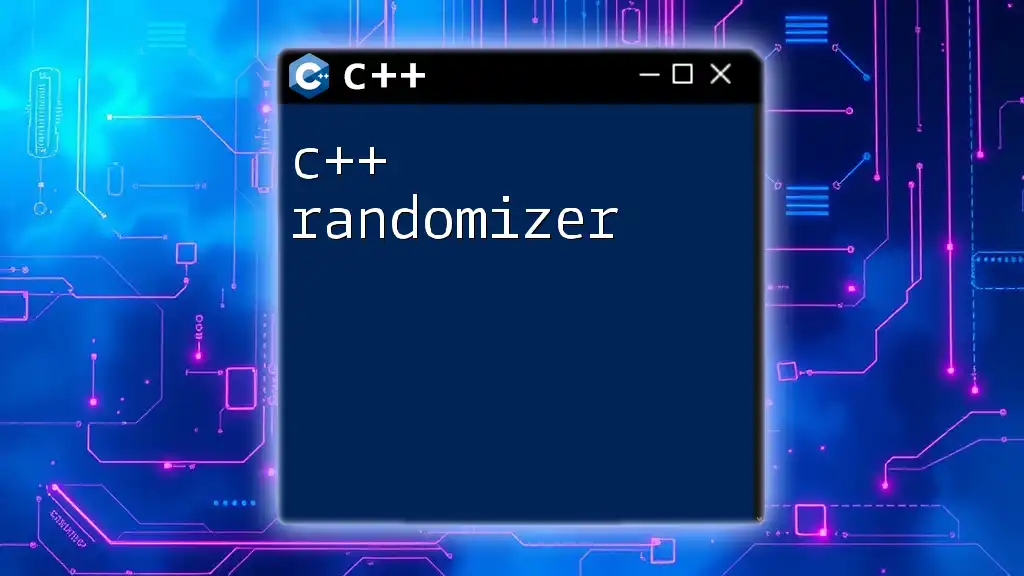
Popular C++ Visualizers
Visual Studio Debugger
The Visual Studio Debugger is one of the most robust tools available for visualizing C++ code. It offers a multitude of features like step-through debugging, real-time variable inspection, and call stacks visualization, making it a powerful ally for developers.
For example, when using the Visual Studio debugger, you can set breakpoints in your code. This allows you to pause execution at key points and inspect variable values dynamically. Suppose we use the following code:
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(5, 6);
return 0;
}
With a breakpoint set at the `return a + b;` line, you can inspect the values of `a` and `b` as the execution halts, providing an invaluable opportunity to ensure correctness.
gdb and gdbgui
gdb (GNU Debugger) paired with gdbgui provides a cross-platform solution for C++ debugging. This combination allows developers to visualize the execution flow without the complexities of a command-line interface.
Using gdb, you can initiate debugging of your program with a simple command:
gdb my_program
Within gdb, commands like `run`, `break`, and `print` allow you to control execution and view state changes. With gdbgui, you can visualize this information in an intuitive interface, displaying stack traces and variable states graphically.
Code::Blocks
Code::Blocks is another powerful IDE that integrates debugging and visualization features for C++. It is user-friendly and provides a simple interface for visualizing projects and code structures.
To visualize your project in Code::Blocks, you can utilize its built-in debugger. It offers a graphical representation of your project's files and structures, minimizing the complexity that comes with navigating large codebases. This coherence allows developers to easily analyze and understand their code organization.
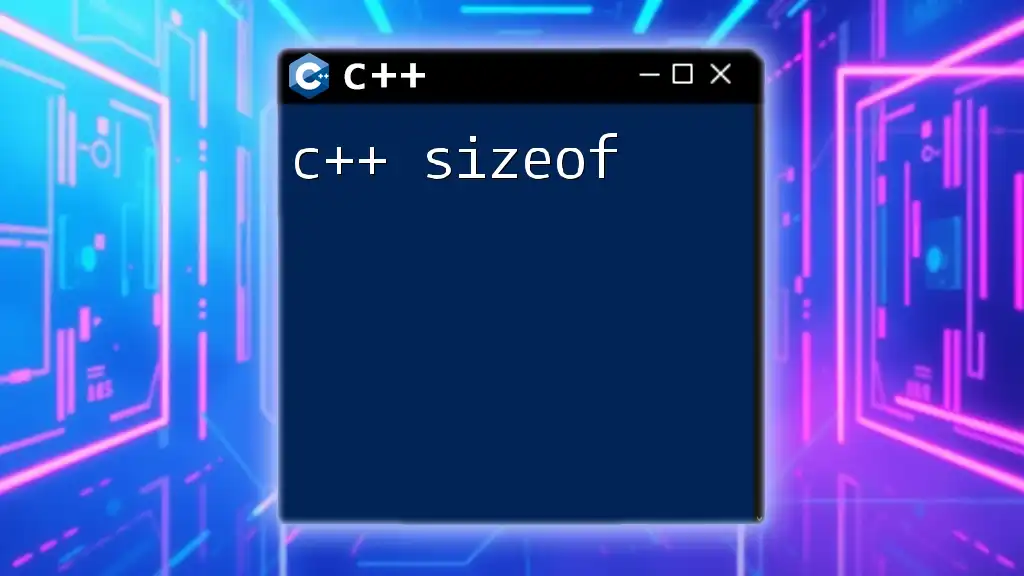
How to Set Up a C++ Visualizer
Requirements
To get started with a C++ visualizer, you will typically need specific software tools. Common tools include IDEs like Visual Studio, Code::Blocks, or command-line utilities like gdb. Ensure you have proper installations because these will serve as the foundation for your visual debugging experience.
Installation Guide
For tools such as Visual Studio, simply download the installer from the official website, follow the installation prompts, and select the C++ development tools during the setup. For gdb, use your system’s package manager to install it, or download it directly.
Configuration
Once installed, it’s essential to configure your visualizer environment appropriately. In Visual Studio, you can adjust settings for breakpoints, variable watches, and the layout of the debugging interface. Additionally, in gdbgui, configurations can be made for color coding and interface preferences. This customization will enhance your visual debugging experience and make it more efficient.
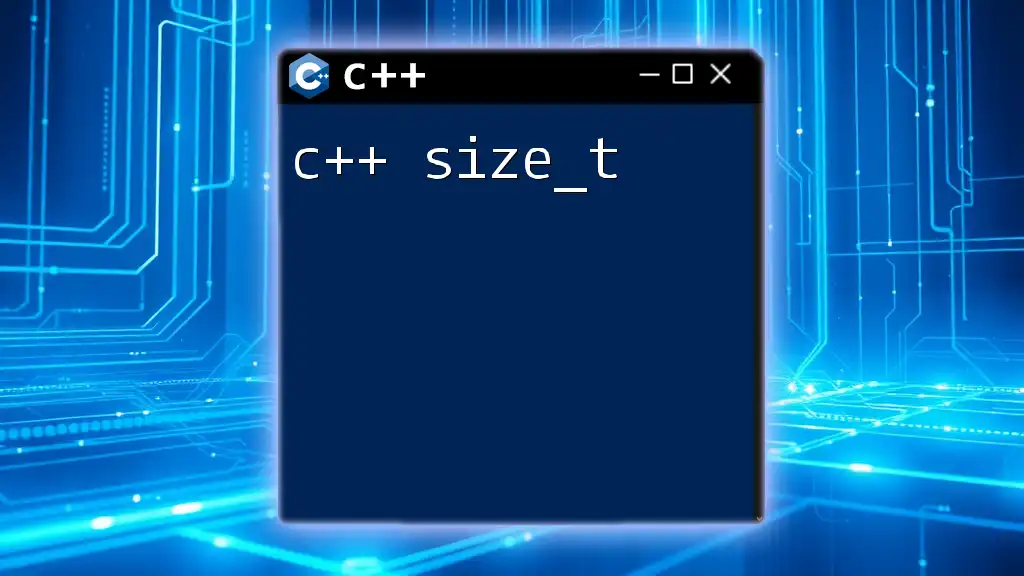
How to Use a C++ Visualizer Effectively
Inspecting Variables
A significant part of using a C++ visualizer effectively is managing breakpoints efficiently. Breakpoints allow you to halt your program execution at critical points. Setting breakpoints can be done by clicking beside the line numbers in most IDEs.
Utilizing features like "Step Into" and "Step Over" enhances your ability to analyze the program flow, enabling you to see how control moves from one part of your code to another. Inspecting variables during these pauses helps in elucidating the program state and data flow.
Viewing Data Structures
When working with complex data structures, a C++ visualizer can assist you in understanding how these structures work. For example, consider the following array example:
int arr[] = {10, 20, 30, 40};
A visualizer can depict a memory layout of this array, allowing you to see how each element is stored sequentially in memory. Furthermore, using pointers alongside visualizers can help clarify address-based data manipulation.
Memory Management Visualization
Visualizing memory management allows developers to differentiate between static and dynamic memory allocations easily. Tools can help illustrate the transitions of memory allocation and deallocation. For instance, consider this code snippet:
int* ptr = new int(5);
Here, a visualizer can show the allocation of memory for `ptr` on the heap, allowing effective tracking of memory usage, which is crucial in preventing memory leaks.
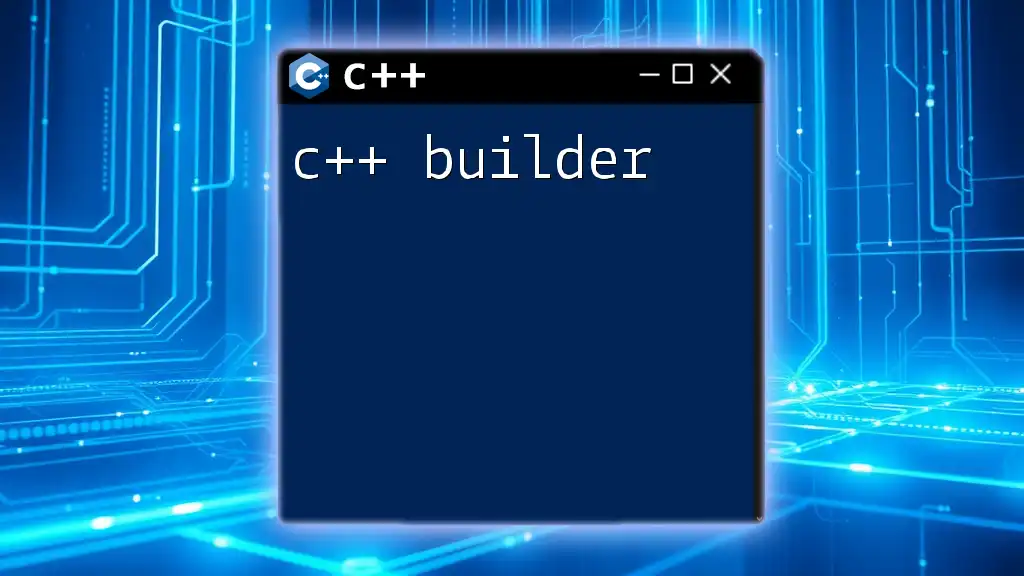
Advanced Features of C++ Visualizers
Real-time Execution Monitoring
Advanced C++ visualizers come with profiling tools that allow developers to monitor performance and identify bottlenecks in real-time. This capability is invaluable for optimizing code, especially in performance-critical applications.
Multi-threading Visualization
As applications become more complex with the use of multi-threading, visualizing thread states can make a significant difference in debugging. By using tools that represent thread interactions and state transitions graphically, developers can identify deadlocks and data races more effectively. Consider the following multi-threaded code snippet:
std::thread t1([] { /* code */ });
A visualizer can help illustrate the starting and stopping states of `t1`, enhancing your ability to manage and debug multi-threaded applications.
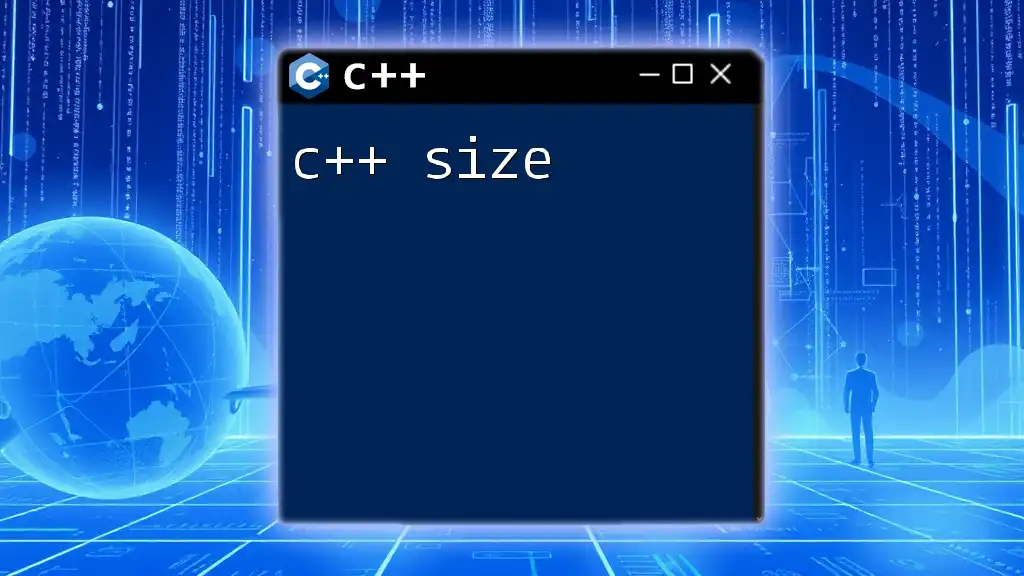
Best Practices for Using C++ Visualizers
Tips for Effective Debugging
Organizing your breakpoints and visualizations can drastically change your debugging efficiency. Effectively manage breakpoints by naming them and grouping them logically. This way, you can quickly enable or disable them based on the debugging context.
Combining Visualizers with Other Tools
A savvy approach involves integrating visualizers with other development tools. When paired with version control systems like Git and code linters, C++ visualizers can enhance code quality and ensure consistency across development environments.
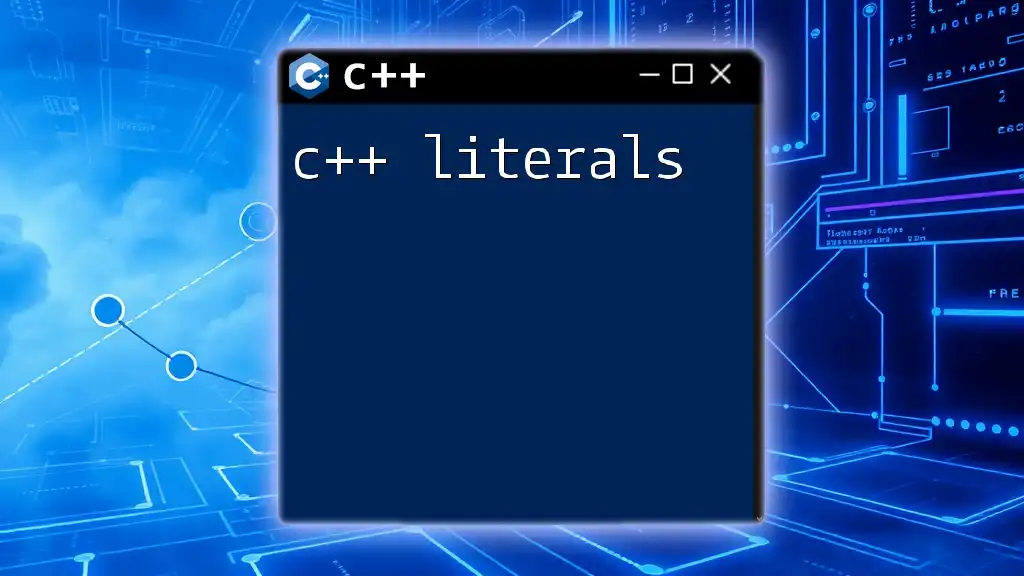
Conclusion
Summary of C++ Visualizers
In summary, a C++ visualizer is a crucial tool for improving code understanding and debugging efficiency. By leveraging the various features offered by modern visualizers, developers can visualize their code, inspect states, and optimize performance more effectively.
Future of C++ Visualizers
As programming evolves, so too will the capabilities of C++ visualizers. Emerging trends might include more sophisticated automated debugging and visualization techniques, making it even easier for developers to produce high-quality, efficient code.
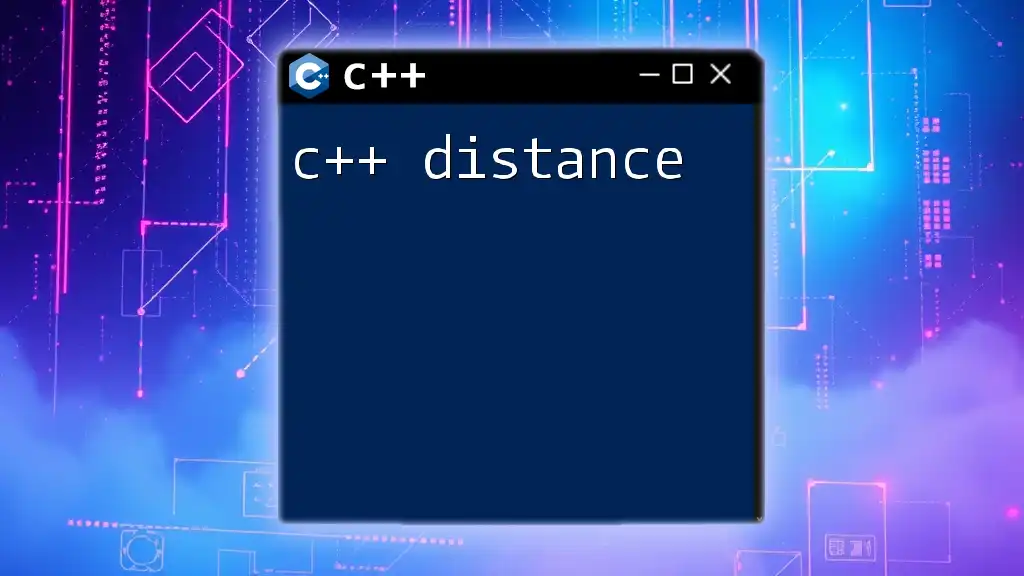
Additional Resources
Recommended Tools and Libraries
To further explore C++ visualizers, consider checking out tools like Clion, Visual Studio Code with extensions, or specialized debugging tools like Valgrind for memory profiling.
Online Courses and Tutorials
For those looking to deepen their understanding, various online platforms offer dedicated courses on C++ programming and debugging, which can provide complementary knowledge to using visualizers effectively.