In C++, the `sizeof` operator is used to determine the size, in bytes, of a data type or object.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
int a;
std::cout << "Size of int: " << sizeof(a) << " bytes" << std::endl;
return 0;
}
What is .size() in C++?
The .size() function in C++ is a member function that returns the number of elements in a container, such as a vector, list, or string. Understanding the c++ size function is essential for manipulating data structures effectively. It helps you determine how many elements are currently stored in the container, enabling better memory management and control in your programs.
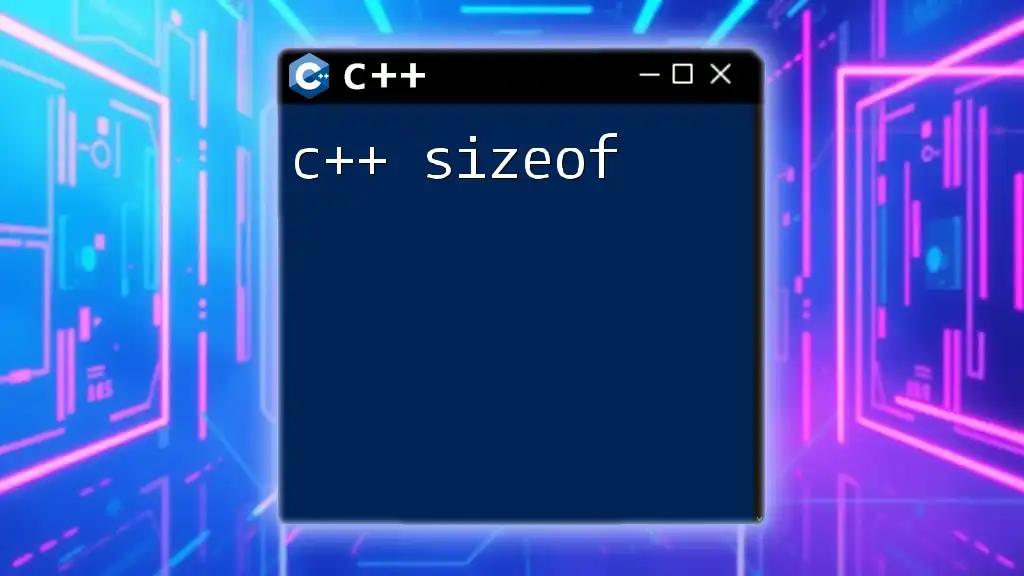
The .size() Function in Standard Containers
Working with Vectors
Vectors are dynamic arrays that are part of the C++ Standard Template Library (STL). They offer flexibility in terms of size and are very commonly used due to their performance and ease of use.
Using the .size() method with vectors is straightforward:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::cout << "Size of the vector: " << numbers.size() << std::endl; // Output: 5
return 0;
}
In this example, the vector `numbers` has five elements. The .size() function returns `5`, indicating the total number of elements contained within the vector.
Working with Strings
In C++, strings are treated as objects of the `std::string` class and provide a range of functionalities, including the ability to check their length using the .size() method.
Here’s how we can retrieve the size of a string:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::cout << "Size of the string: " << text.size() << std::endl; // Output: 13
return 0;
}
In this example, the `text` string has a size of `13`, which includes all characters, including spaces and punctuation.
Working with Other STL Containers
C++ provides several other containers in its Standard Template Library. Each of these containers also implements the .size() function, allowing you to easily determine their size.
Using .size() with Lists
Lists in C++ are doubly linked lists. They are not as memory efficient as vectors, but they provide advantages in insertion and deletion of elements.
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3};
std::cout << "Size of the list: " << myList.size() << std::endl; // Output: 3
return 0;
}
In this case, the list `myList` has three elements, and calling .size() returns `3`.
Using .size() with Deques
Deques are double-ended queues that allow fast insertion and deletion from both ends.
#include <iostream>
#include <deque>
int main() {
std::deque<int> myDeque = {1, 2, 3, 4};
std::cout << "Size of the deque: " << myDeque.size() << std::endl; // Output: 4
return 0;
}
For the deque `myDeque`, the size is `4`.
Using .size() with Arrays
Native arrays in C++ do not have a .size() method, making it a common pitfall for programmers unfamiliar with C++. To get the size of a native array, you must use the `sizeof` operator.
#include <iostream>
int main() {
int myArray[] = {1, 2, 3, 4, 5};
// To get the size, we need to use sizeof
std::cout << "Size of the array: " << sizeof(myArray) / sizeof(myArray[0]) << std::endl; // Output: 5
return 0;
}
Here, the size of the array is calculated by dividing the total size in bytes by the size of one element, resulting in `5`.
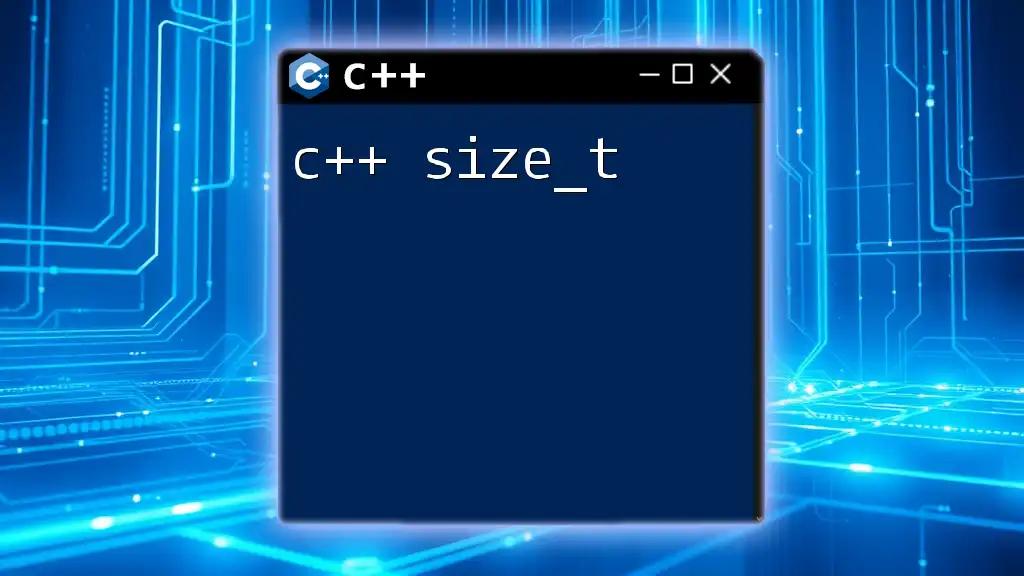
Different Requirements for Size Calculation
In some cases, the .size() function might not be suitable for measuring the size of a structure. For example, in custom data structures, one must implement a similar method to track the number of elements properly. This underscores the importance of understanding the data structure being used and the overhead that comes with the implementation of the .size() function.

The .size() Function vs. Other Size Measurement Methods
It's crucial to differentiate the .size() function from the `sizeof` operator. While .size() returns the number of elements in a container, `sizeof` returns the total size of a data structure in bytes, which can offer valuable insights for managing memory.
Understanding Size in the Context of Pointers
When dealing with pointers, the size of the pointer does not correlate to the size of the data it points to. If you use .size() on a pointer, it will not yield meaningful information about the data being pointed to. Always ensure you are working with proper data types when measuring sizes.

Performance Considerations
Using the .size() function has minimal overhead, especially for STL containers designed to return sizes quickly. However, if your program calls .size() within loops excessively or in performance-critical sections of the code, it could lead to unwanted performance hits. Understanding when and where to check size can improve the overall efficiency of your program.

Common Pitfalls When Using .size()
There are several traps developers can fall into when using the .size() function:
- Using .size() on native arrays: As mentioned earlier, it won't work as expected and can lead to misunderstandings regarding the number of elements in an array.
- Assuming size remains constant post modifications: The size of a container changes dynamically with additions and deletions. Always check the size after changes.
- Misunderstanding empty containers: Calling .size() on an empty container will return `0`. Be cautious not to use this to indicate whether a container is initialized or if it has data.
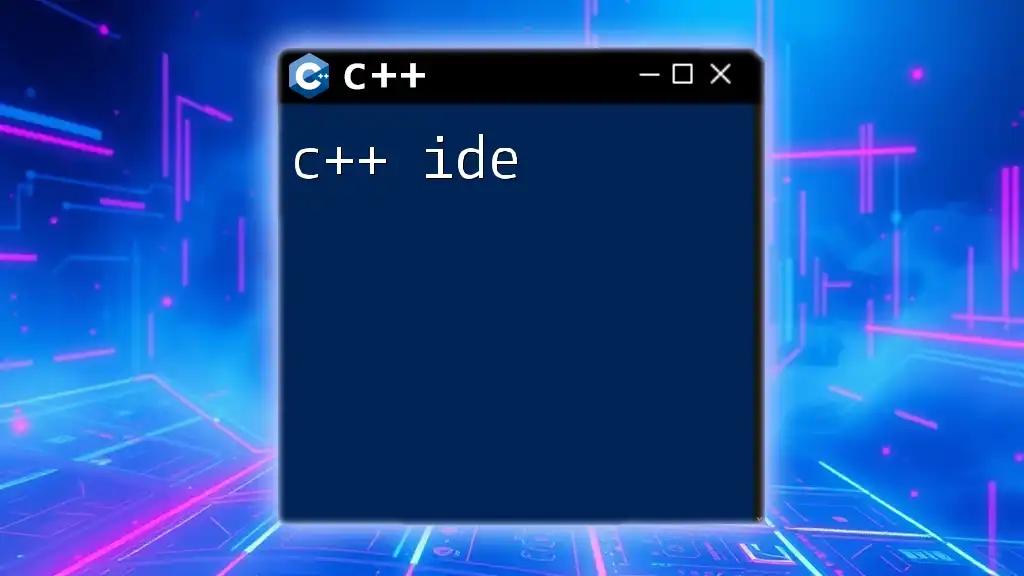
Conclusion
Understanding the c++ size concept through the .size() function is critical in effective C++ programming. It allows developers to query container sizes and fosters better memory management. With the knowledge gained in this article, you are better equipped to utilize the .size() method and improve your programming practices by accurately tracking data structure sizes.