The `seekg` function in C++ is used to set the position of the get pointer in a file stream, allowing you to read data from a specific location within the file.
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
file.seekg(10); // Move the get pointer to the 10th byte
char ch;
file.get(ch); // Read a character from the current position
std::cout << ch << std::endl; // Output the character
file.close();
return 0;
}
Understanding File Streams in C++
What are File Streams?
File streams are essential for handling input and output operations in C++. They allow developers to interact with files seamlessly. Using streams, you can read data from files and write data to them.
In C++, we primarily work with two types of file streams:
- Input File Streams (`ifstream`): Used for reading data from files.
- Output File Streams (`ofstream`): Used for writing data to files.
In some cases, you might use `fstream`, which combines both input and output functionalities.
Creating File Streams
To work with file streams in C++, you first need to include the `<fstream>` header. Here’s a basic code snippet to open a file for reading:
#include <fstream>
std::ifstream inputFile("data.txt");
if (!inputFile) {
std::cerr << "Error opening file." << std::endl;
}
In this example, we create an input file stream and check if the file opened successfully. Proper error handling is crucial in file operations.
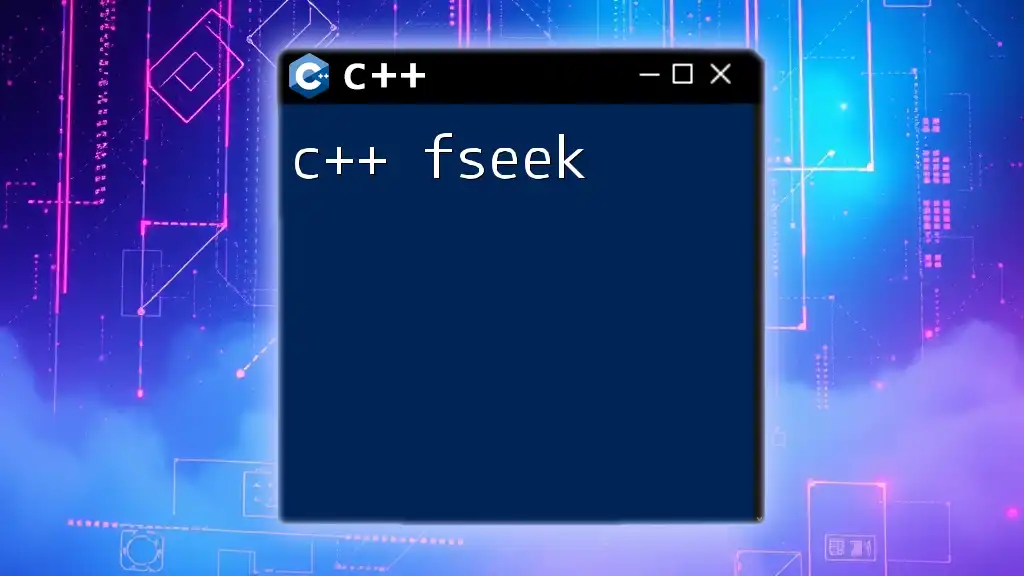
The Role of `seekg`
What Does `seekg` Stand For?
The `seekg` function is part of the file stream library that manipulates the position of the current character in input file streams. It stands for "seek get", indicating its purpose to change the position from where the next character will be read.
Function Signature of `seekg`
The function signature can be summarized as follows:
std::ifstream& seekg(std::streampos pos);
std::ifstream& seekg(std::streamoff off, std::ios_base::seekdir way);
- `seekg(pos)`: Moves the pointer to the specified position directly.
- `seekg(off, way)`: Moves the pointer relative to a specific position (beginning, current, or end).
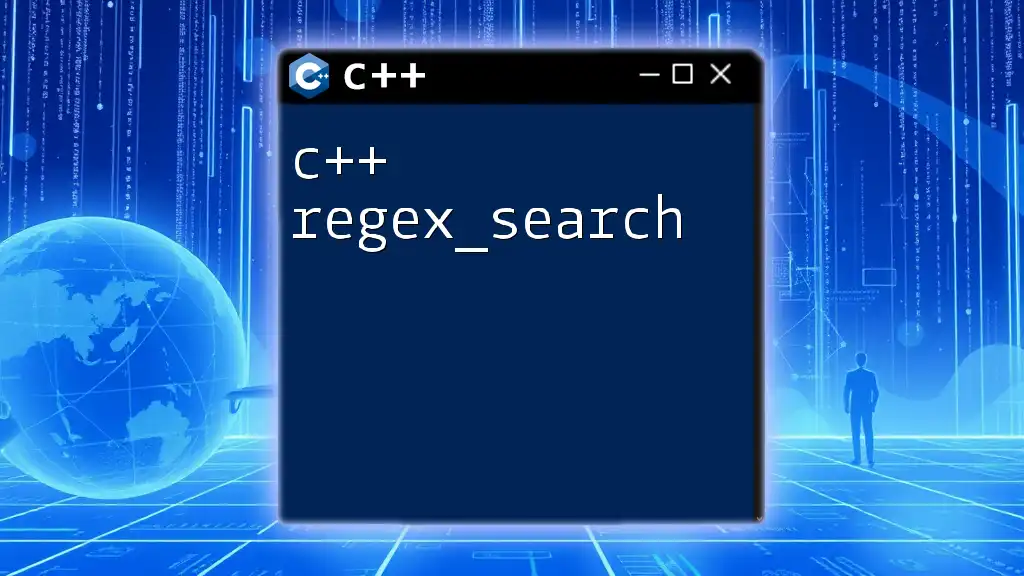
How to Use `seekg`
Basic Usage of `seekg`
Using `seekg` is quite straightforward. Here’s a simple example demonstrating how to seek to a specific position:
#include <fstream>
#include <iostream>
int main() {
std::ifstream inputFile("data.txt");
inputFile.seekg(10); // Move to the 10th byte
char ch;
inputFile.get(ch); // Read the character at the 10th position
std::cout << "Character at position 10: " << ch << std::endl;
return 0;
}
This code snippet opens a file and moves the pointer to the 10th byte. It then reads and outputs the character found at that position.
Using `seekg` with Different File Positions
Using Absolute Positions
When you want to jump to an exact byte position, you can directly use absolute positioning as follows:
inputFile.seekg(20); // Move to the 20th byte
This moves the pointer to the 20th byte from the beginning of the file. This is particularly useful when you know the exact byte offset you want to read from.
Using Relative Positions
You can also move the pointer based on its current position or the end of the file. This is accomplished by using the following constants:
- `std::ios::beg`: Beginning of the file.
- `std::ios::cur`: Current position.
- `std::ios::end`: End of the file.
Here’s how to use it:
inputFile.seekg(0, std::ios::end); // Move to the end of the file
std::streampos fileSize = inputFile.tellg(); // Get the file size
inputFile.seekg(-10, std::ios::cur); // Move back 10 bytes from the current position
In this example, you can observe how `seekg` allows dynamic file positioning based on where you currently are within the file.
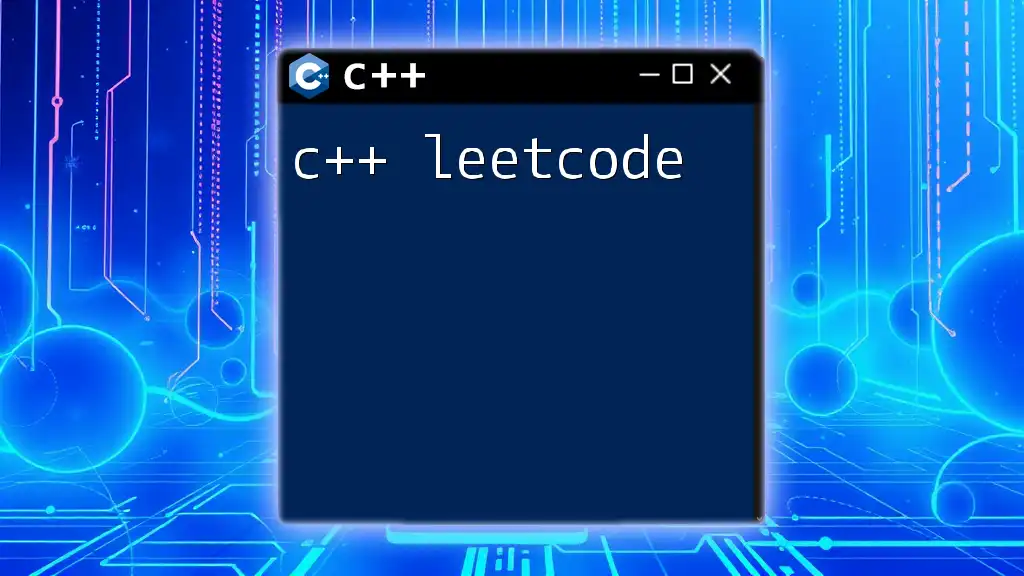
Practical Applications of `seekg`
Reading Specific Parts of a File
One practical use of `seekg` is reading specific sections of larger files. Suppose you are analyzing a data file with records and you need to access a specific record directly without reading through the entire file.
// Example: Reading a specific record
inputFile.seekg(50); // Jump to the desired record offset
std::string record;
std::getline(inputFile, record); // Read the line
std::cout << "Record at offset 50: " << record << std::endl;
This example demonstrates how you can efficiently navigate to a record without unnecessary reads.
Reverting the File Pointer
If you need to go back to a previous position, `seekg` can be incredibly useful. For instance:
inputFile.seekg(0); // Go back to the start of the file
This allows you to re-read data or process the file multiple times as needed.
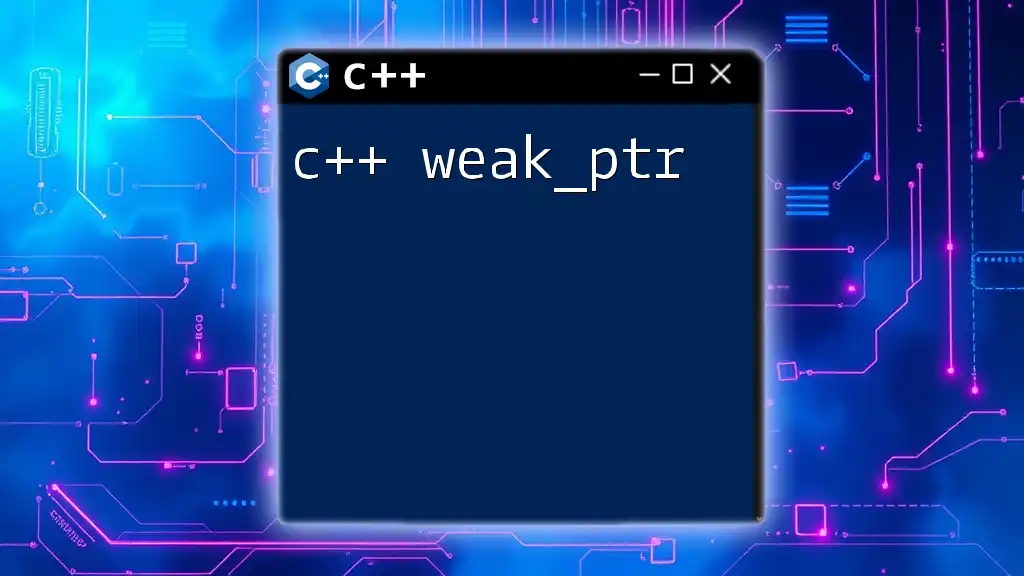
Error Handling with `seekg`
Common Errors and Solutions
When working with file I/O, various issues may occur, such as:
- Invalid positions: Seeking beyond the file's length can cause errors.
- File not open: Forgetting to check if the file opened correctly.
Use proper error checking to handle these situations gracefully:
if (inputFile.bad()) {
std::cerr << "Error occurred while seeking." << std::endl;
}
Best Practices
To avoid common pitfalls with `seekg`, adhere to these best practices:
- Always check if the file stream is in a good state before seeking.
- Use `tellg()` to confirm your current position before moving the pointer back or forward.
- Be cautious with mixed input/output streams to avoid confusion.
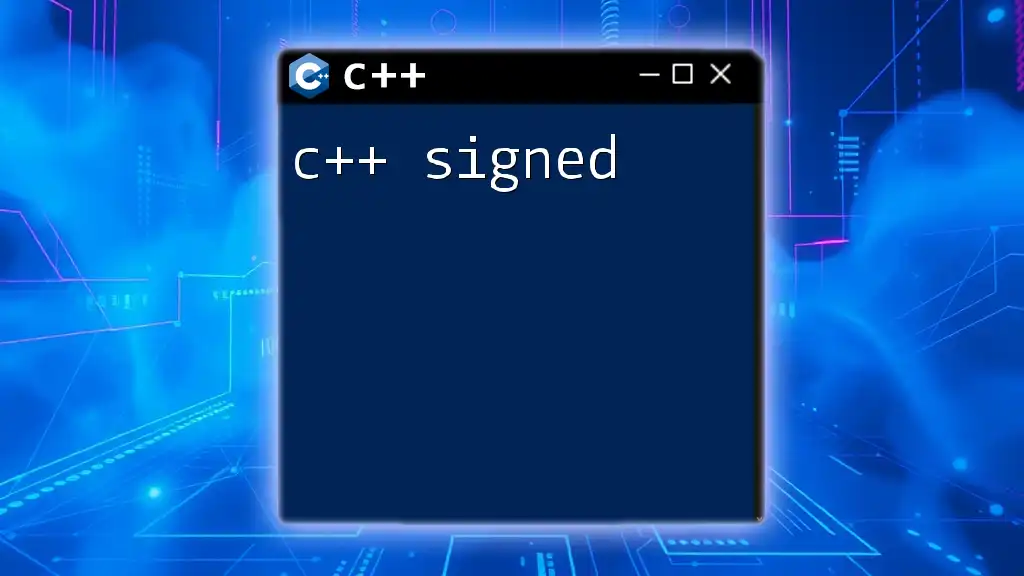
Advanced Usage
Combining `seekg` with Other File Operations
Using `seekg` in combination with other file operations can give you greater control over file processing. For example, you can read and modify data dynamically:
inputFile.seekg(20);
std::string data;
std::getline(inputFile, data);
// Modify data here
// Write it back with an ofstream if necessary!
This flexibility can lead to more efficient file handling strategies.
Using `seekg` in Binary Files
When working with binary files, `seekg` becomes particularly significant. Consider this code snippet where we navigate through a binary file:
#include <fstream>
std::ifstream binaryFile("data.bin", std::ios::binary);
binaryFile.seekg(0, std::ios::end);
std::streampos size = binaryFile.tellg();
binaryFile.seekg(0, std::ios::beg); // Reset to start
// Read a specific number of bytes.
char* buffer = new char[size];
binaryFile.read(buffer, size);
In this example, you see how `seekg` allows for precise control over binary data, facilitating efficient data processing.
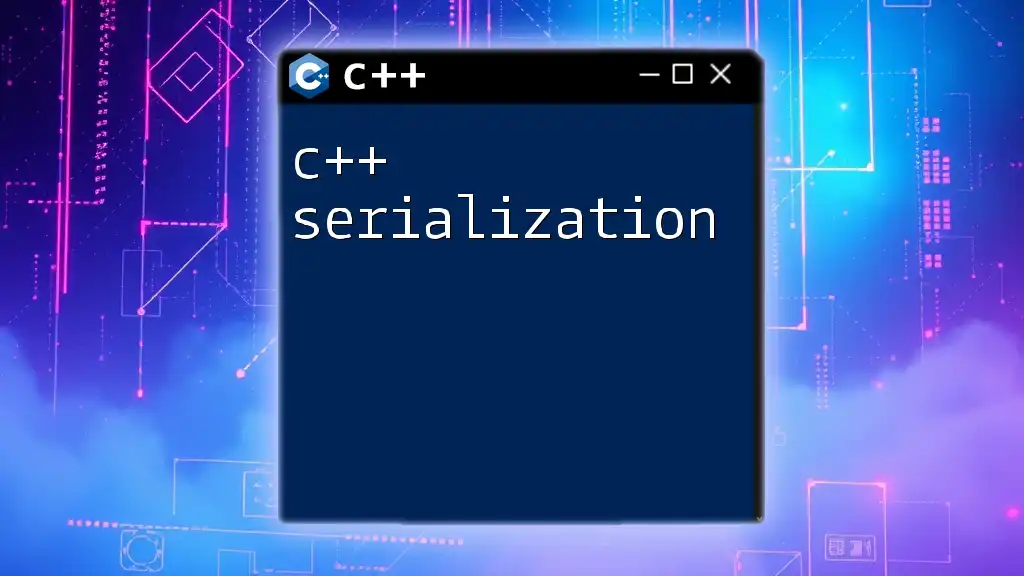
Conclusion
Recap of Key Points
Understanding `seekg` is essential for effective file handling in C++. It provides a powerful means to navigate within files, allowing you to read or write data at your discretion. Remember to always manage your file pointers carefully to avoid common errors and enhance your file processing capabilities.
Encouragement to Practice
To master `seekg`, integrate it into your coding projects. Experiment with various file formats, sizes, and read/write operations. The more you practice, the more adept you will become at file manipulation in C++.
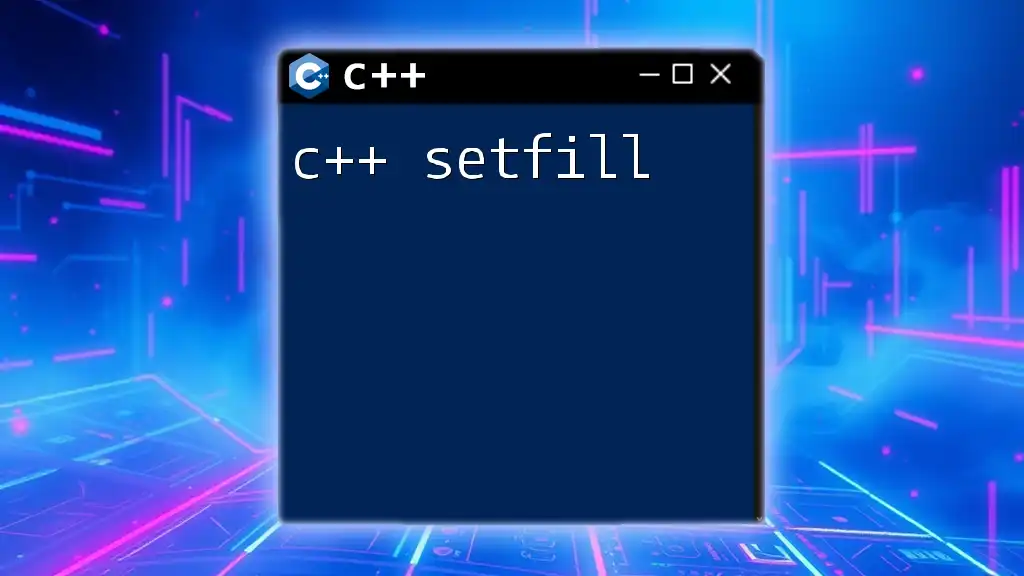
Additional Resources
Links to Documentation
To deepen your understanding of file handling in C++, consider checking out the official C++ documentation and reputable programming websites that offer guides on file streams.
Community Forums and Tutorials
Participate in online communities or forums like Stack Overflow to ask questions, share your experiences, and learn from others who also work with C++. Engaging with fellow programmers can enhance your understanding and provide support as you continue your journey with C++.