In C++, the set union operation combines two or more sets, ensuring that all elements from the sets are included without duplicates.
Here's a code snippet demonstrating how to perform a set union using the Standard Template Library (STL):
#include <iostream>
#include <set>
#include <algorithm>
int main() {
std::set<int> set1 = {1, 2, 3};
std::set<int> set2 = {3, 4, 5};
std::set<int> result;
std::set_union(set1.begin(), set1.end(), set2.begin(), set2.end(), std::inserter(result, result.begin()));
for (int num : result) {
std::cout << num << " ";
}
return 0;
}
Understanding the Set Union in C++
What is a Set Union?
A set union is a fundamental operation in set theory, combining two or more sets to produce a new set that contains all unique elements from the participating sets. The resulting set will include every element found in either of the original sets without duplicates.
For instance, if you have two sets:
- Set A: {1, 2, 3}
- Set B: {3, 4, 5}
The set union of A and B would yield:
- Union: {1, 2, 3, 4, 5}
Understanding set unions is crucial in programming, particularly when dealing with collections of data. It allows developers to easily merge information, making it a powerful tool for data manipulation and analysis.
The `set_union` Function in C++
The C++ Standard Library provides a powerful function called `set_union` that facilitates this operation. This function is found in the `<algorithm>` header and works specifically with sorted ranges.
Syntax:
set_union(first1, last1, first2, last2, result);
Parameters:
- `first1`, `last1`: Iterators defining the range of the first input set.
- `first2`, `last2`: Iterators defining the range of the second input set.
- `result`: An output iterator where the union of both input ranges will be stored.
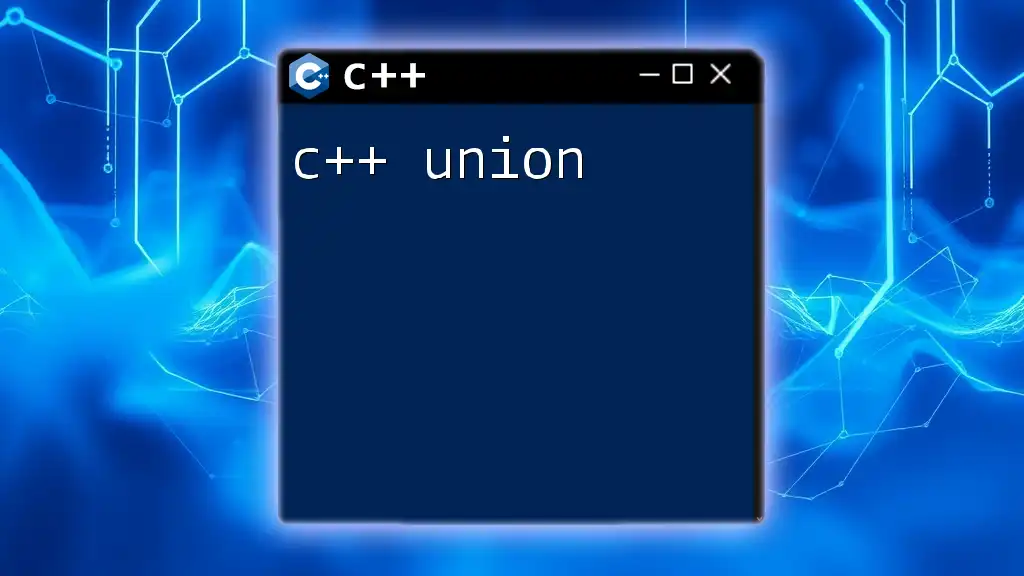
How to Use `set_union` in Your C++ Code
Basic Example of Set Union
To illustrate how the C++ set union works, consider the following example where we combine two sets of integers:
#include <iostream>
#include <set>
#include <algorithm> // For set_union
int main() {
std::set<int> setA = {1, 2, 3, 4};
std::set<int> setB = {3, 4, 5, 6};
std::set<int> resultSet;
std::set_union(setA.begin(), setA.end(), setB.begin(), setB.end(),
std::inserter(resultSet, resultSet.begin()));
std::cout << "Union Set: ";
for (const auto& elem : resultSet) {
std::cout << elem << " ";
}
return 0;
}
Explanation: In this code, we have two sets, `setA` and `setB`, which both contain integers. The `set_union` function takes the elements of both sets, combines them, and stores the result in `resultSet`. The use of `std::inserter` ensures that the elements are inserted correctly into `resultSet` while preserving their uniqueness.
Output: The output of the above program would be:
Union Set: 1 2 3 4 5 6
Advanced Usage of `set_union`
Combining multiple sets can be performed through repeated usage of the `set_union` function. This approach allows you to create a union set from several input sets.
Handling Duplicates: When performing a set union, it's essential to remember that duplicates are automatically handled since sets in C++ only allow unique elements. Thus, if any elements are repeated across the sets being combined, they will appear only once in the resultant set.
Consider merging three sets:
std::set<int> setC = {7, 8};
std::set<int> combinedSet;
std::set_union(setA.begin(), setA.end(), setB.begin(), setB.end(),
std::inserter(combinedSet, combinedSet.begin()));
std::set_union(combinedSet.begin(), combinedSet.end(), setC.begin(), setC.end(),
std::inserter(resultSet, resultSet.begin()));
for (const auto& elem : resultSet) {
std::cout << elem << " ";
}
In this snippet, we merge three sets, with each merging operation ensuring duplicates are not present in the final output.
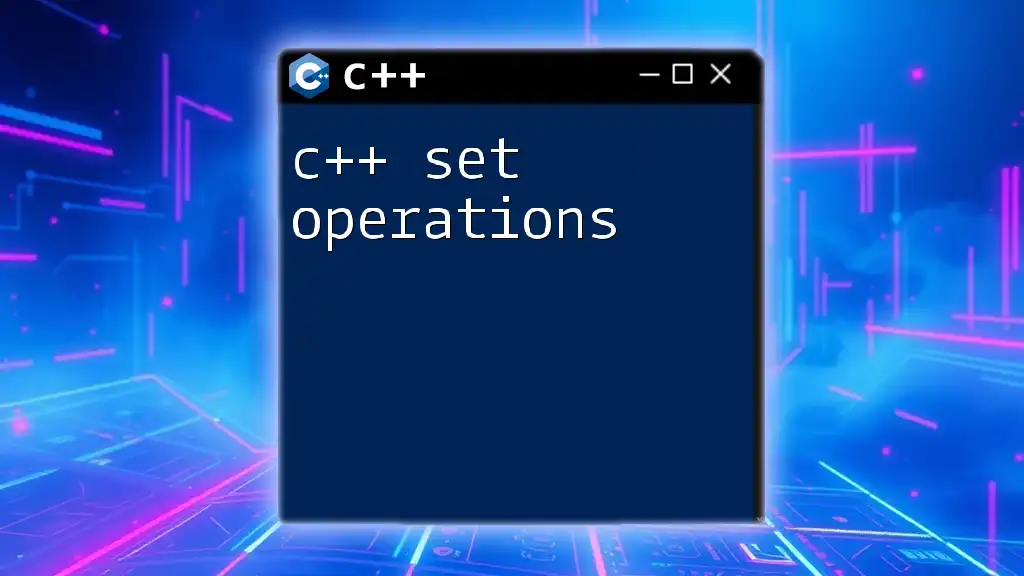
Handling Different Data Types in Set Union
Set Union with Different Data Types
The `set_union` function is versatile and works with various data types, such as `std::string`. Here’s an example demonstrating how to use `set_union` with strings:
#include <string>
#include <set>
#include <algorithm>
int main() {
std::set<std::string> setA = {"apple", "banana", "cherry"};
std::set<std::string> setB = {"banana", "dragonfruit", "elderberry"};
std::set<std::string> resultSet;
std::set_union(setA.begin(), setA.end(), setB.begin(), setB.end(),
std::inserter(resultSet, resultSet.begin()));
// Output the result
for (const auto& elem : resultSet) {
std::cout << elem << " ";
}
return 0;
}
Explanation: This function proceeds in the same way as the integer example, collecting unique fruits from both sets. This highlights the utility of `set_union` for combining strings just as effectively.
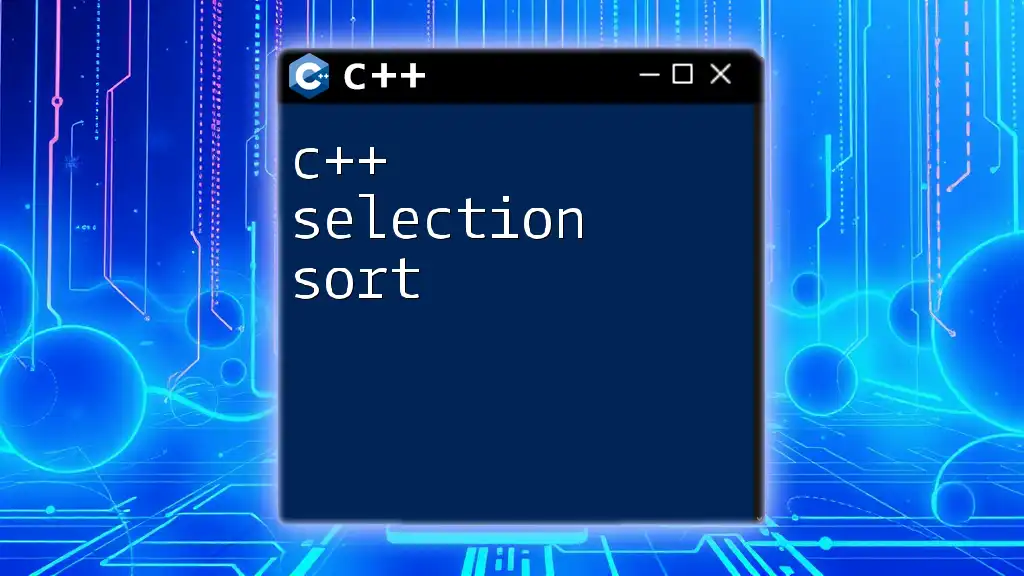
Performance Considerations When Using Set Union
Time Complexity of Set Union
The time complexity of the `set_union` function is O(n + m), where n and m are the sizes of the two input sets. This efficiency stems from the fact that both sets must be sorted beforehand. If you encounter large datasets, the performance benefit of sets becomes evident.
Best Practices for Efficient Set Union Operations
- Optimal Input Sizes: Consider limiting the size of the input sets. Working with smaller sets can significantly improve performance and efficiency.
- Utilize Sorted Sets: Ensure that the sets are sorted before applying `set_union`, as it operates under the assumption that input ranges are in ascending order.
- Choose Appropriate Data Structures: In cases where the order of elements is unimportant, alternatives like `unordered_set` may offer performance advantages.
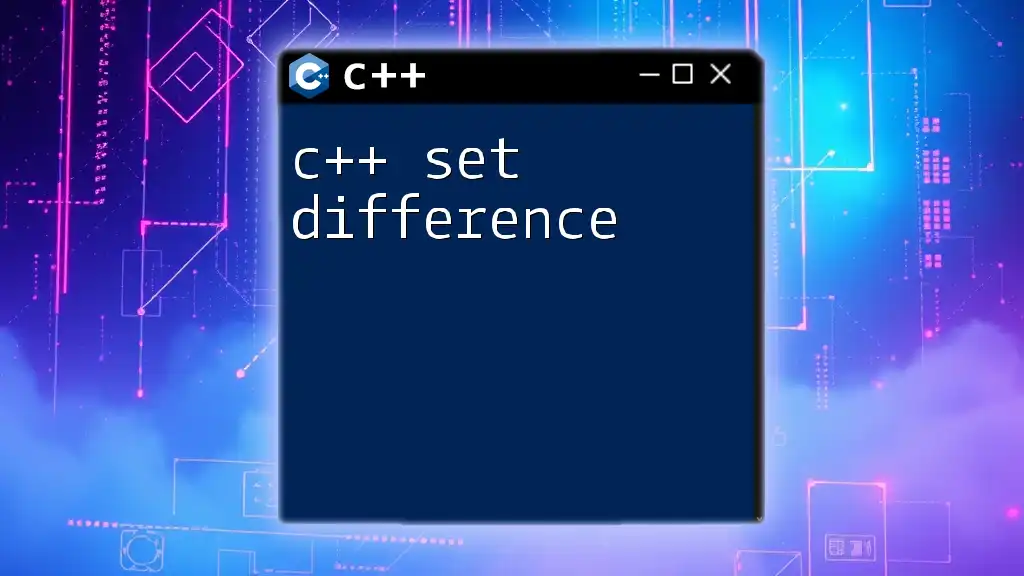
Common Mistakes to Avoid with `set_union`
- Omitting Required Headers: Always ensure that the `<set>` and `<algorithm>` headers are included before using sets and any algorithm functions.
- Incorrect Initialization of Sets: Avoid pitfalls by ensuring sets are initialized properly to prevent unexpected behavior during operations.
- Not Using the Insert Iterator: When storing results, forgetfulness about the `std::inserter` could lead to runtime errors or performance issues.
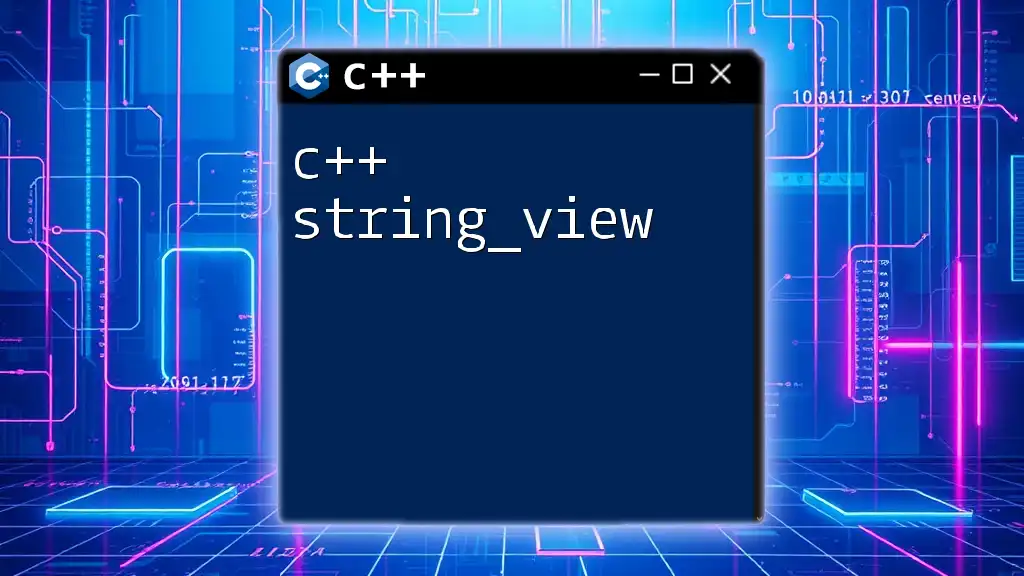
Conclusion
In conclusion, mastering C++ set union is integral to effective data management in programming. This operation allows developers to effectively combine data sources and manage unique values, making it a key concept in the toolkit of C++ programmers. Practicing with various examples and understanding the theoretical underpinnings will equip you with the knowledge necessary to use `set_union` effectively in your projects.
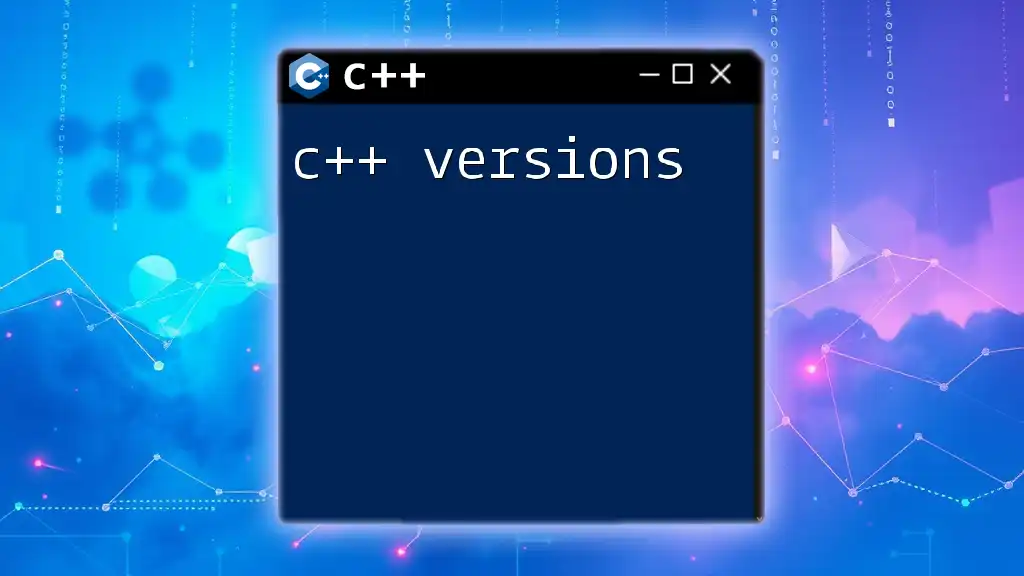
Additional Resources
For those eager to delve deeper into C++ sets and related algorithms, numerous resources are available. Consider exploring online forums, community discussions, comprehensive books, and tutorials to further enhance your understanding and skills in C++.