Selection sort is a simple sorting algorithm that repeatedly selects the minimum element from the unsorted portion of the array and moves it to the beginning.
Here’s a code snippet demonstrating selection sort in C++:
#include <iostream>
using namespace std;
void selectionSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
int minIdx = i;
for (int j = i + 1; j < n; j++)
if (arr[j] < arr[minIdx])
minIdx = j;
swap(arr[minIdx], arr[i]);
}
}
int main() {
int arr[] = {64, 25, 12, 22, 11};
int n = sizeof(arr) / sizeof(arr[0]);
selectionSort(arr, n);
cout << "Sorted array: ";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
The Selection Sort Algorithm in C++
How the Selection Sort Algorithm Works
The C++ selection sort algorithm operates on the principle of repeatedly finding the minimum element from an unsorted portion of the array and placing it at the beginning. This process is repeated until the entire array is sorted. The algorithm can be broken down into a few simple steps:
- Initialization: Start with the first element of the array and assume it is the minimum.
- Finding the Minimum: Iterate through the remaining elements to find the actual minimum value in the unsorted part of the array.
- Swapping: If a new minimum is found, swap it with the first element of the unsorted portion.
- Progression: Move the boundary between the sorted and unsorted portions one position to the right (i.e., the first element in the unsorted section now becomes part of the sorted section).
- Repeat: Continue the process until the entire array is sorted.
This algorithm is particularly intuitive due to its straightforward nature. However, it does not take advantage of more complex strategies that could speed up the sort, making it less efficient for larger datasets.
Pseudocode for Selection Sort
Here’s a simple pseudocode representation to provide an abstract understanding:
function selectionSort(array):
for i from 0 to length(array) - 1:
minIndex = i
for j from i + 1 to length(array):
if array[j] < array[minIndex]:
minIndex = j
swap array[i] with array[minIndex]
This pseudocode captures the essence of the selection sort algorithm, highlighting the looping structure and the fundamental operations performed.
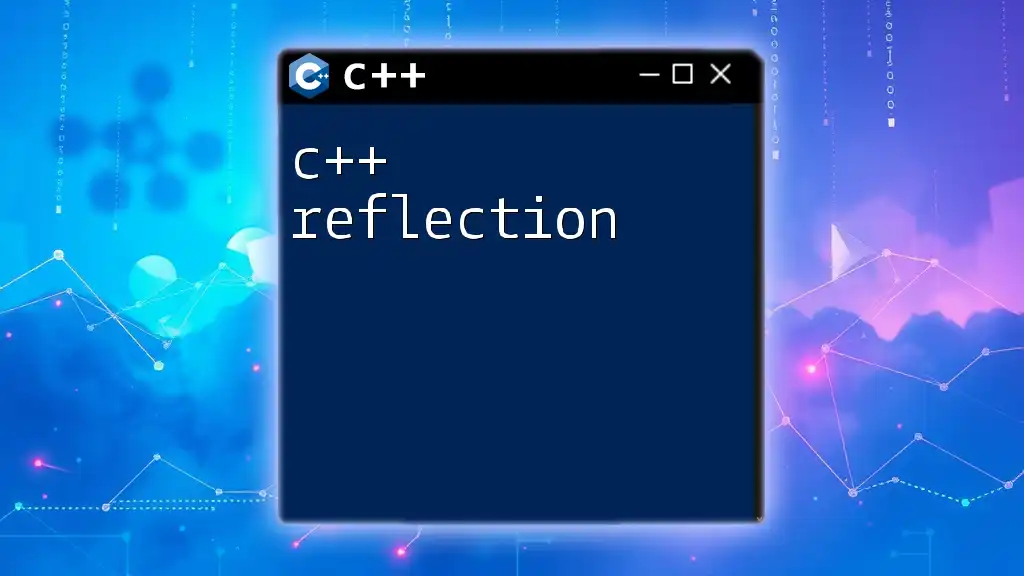
Implementing Selection Sort in C++
Setting Up Your C++ Environment
Before diving into the coding, ensure your C++ environment is set up. This typically involves:
- An IDE or text editor (like Visual Studio, Code::Blocks, or even a simple text editor)
- A C++ compiler (GCC, Clang, or Visual C++)
- Basic knowledge of compiling and running C++ programs
C++ Code Example for Selection Sort
Let’s look at a C++ selection sort implementation:
#include <iostream>
using namespace std;
void selectionSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
swap(arr[i], arr[minIndex]);
}
}
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
cout << endl;
}
int main() {
int arr[] = {64, 25, 12, 22, 11};
int n = sizeof(arr) / sizeof(arr[0]);
selectionSort(arr, n);
printArray(arr, n);
return 0;
}
Explanation of the Code Snippet
- Function Definition: The `selectionSort()` function takes an integer array and its size as inputs.
- Loop Structures:
- The outer loop runs from the first element to the second-last, signifying the range of the sorted section.
- The inner loop iterates through the unsorted part and finds the index of the minimum element.
- Swapping: The `swap()` function is called to place the found minimum at the start of the unsorted section.
- Printing the Array: The `printArray()` function simply outputs the sorted array.
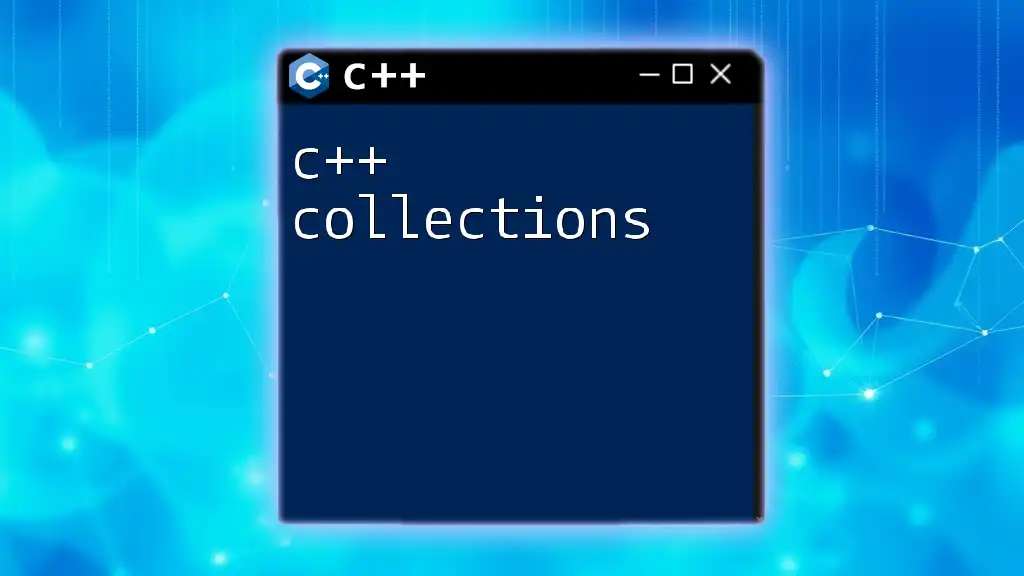
Analyzing the Selection Sort Algorithm
Time Complexity of Selection Sort
The time complexity of the selection sort algorithm is as follows:
- Best Case: O(n²)
- Average Case: O(n²)
- Worst Case: O(n²)
The algorithm performs quadratic time complexity because for every element, it examines each of the remaining elements to find the minimum.
Space Complexity of Selection Sort
The space complexity is O(1) since this algorithm sorts the array in place, requiring a constant amount of additional space regardless of the input size. This characteristic makes it memory efficient compared to other sorting algorithms that may require additional arrays or structures.
Advantages and Disadvantages of Selection Sort
- Advantages:
- The selection sort algorithm is simple to implement and understand.
- It performs well for small datasets:
- Stability: While the traditional implementation is not stable (it may change the order of equal elements), a slightly modified version can be made stable.
- Disadvantages:
- It is generally inefficient for larger datasets compared to faster algorithms such as quicksort, mergesort, or heapsort.
- The number of comparisons remains quadratic, which can lead to notable performance degradation with larger arrays.
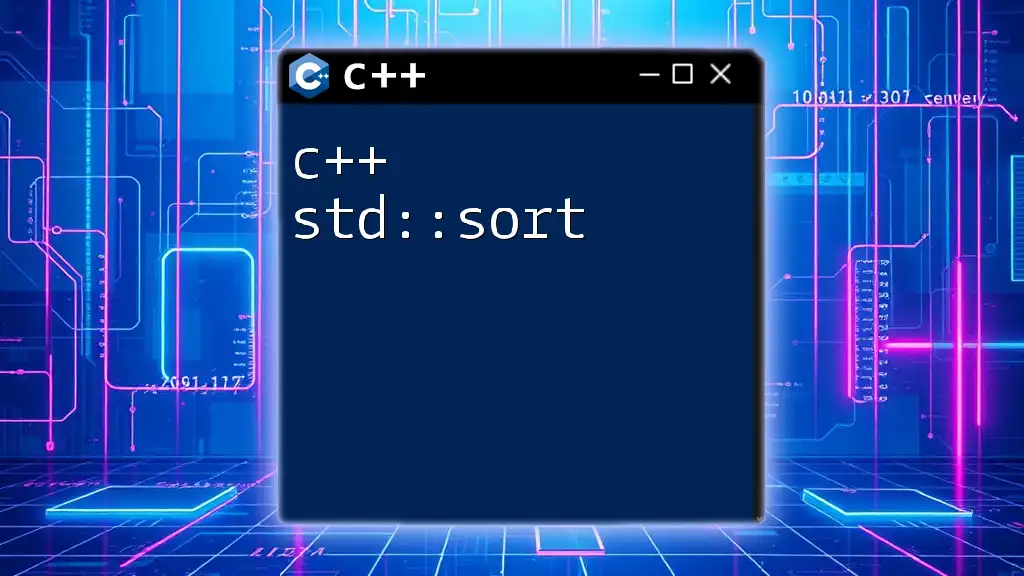
Practical Use Cases for Selection Sort in C++
When to Use Selection Sort
While the C++ selection sort might not be the first choice for large datasets, it can still be beneficial in certain scenarios, such as:
- Educational Purposes: It's an excellent introductory algorithm for teaching sorting concepts in computer science courses.
- Small Datasets: Its simplicity makes it ideal for applications where the dataset is small and the overhead of more efficient algorithms isn't justified.
- Memory-Constrained Environments: Since it requires minimal additional space, it can be favorable when memory usage is critical.
Alternatives to Selection Sort
If you find the selection sort algorithm isn’t suitable for your needs, consider utilizing other sorting algorithms:
- Bubble Sort: A simple algorithm that works similarly to selection sort but with different iterating techniques.
- Insertion Sort: Efficient for small data sets and can be adapted to work as a hybrid with more complex algorithms.
- Quicksort and Mergesort: Highly efficient for large datasets but come with increased complexity and require additional memory.
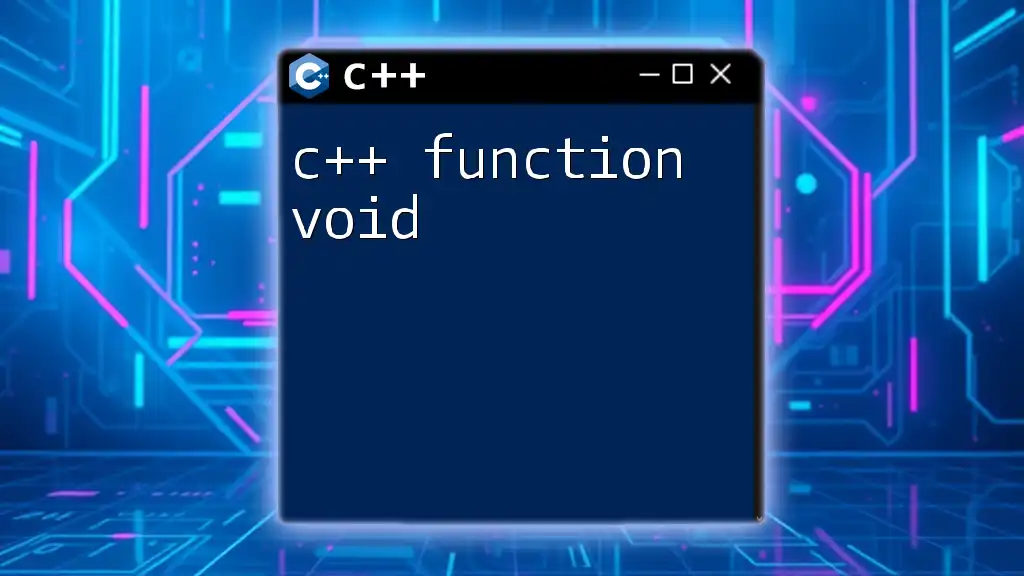
Conclusion
In summary, the C++ selection sort is a foundational algorithm for sorting, characterized by its clarity and straightforward methodology. Despite its inefficiencies with larger datasets, it remains a valuable tool for education and certain practical applications. By practicing the implementation and modification of the selection sort, learners can develop a better grasp of algorithmic thinking and problem-solving in C++.
Encouragement for Practice
To solidify your understanding, consider implementing selection sort with various datasets and experimenting with modifications. Explore different data structures (such as linked lists) and analyze how selection sort performs with them.
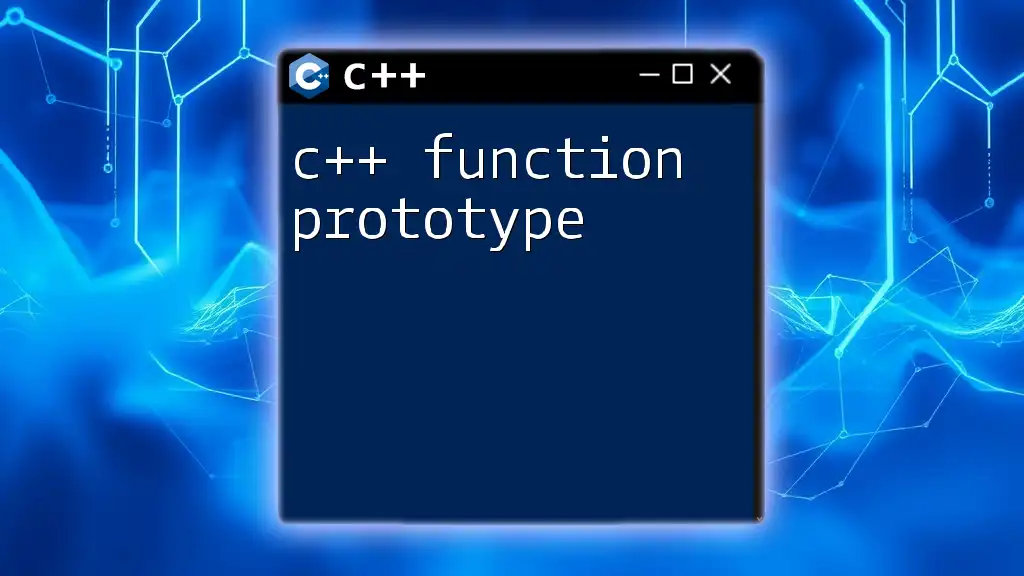
Additional Resources
Books and Online Courses
Explore various educational materials and platforms that cover sorting algorithms in detail, as well as C++ fundamentals. Resources may include textbooks on algorithms or online courses available on platforms like Coursera or Udacity.
Online Coding Platforms
Leverage coding challenge platforms such as LeetCode or HackerRank to practice sorting algorithms and test your C++ selection sort implementation in diverse scenarios.
Community and Forums
Don't hesitate to engage with communities on platforms like Stack Overflow or Reddit to ask questions, share insights, and learn from other programmers’ experiences related to selection sort and sorting algorithms in general.
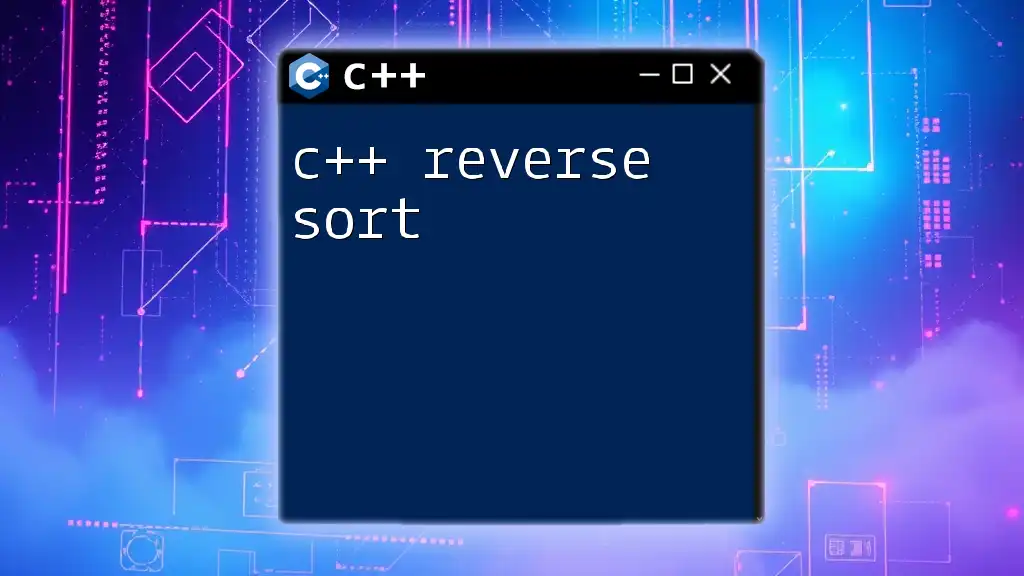
FAQs
What is the average-case time complexity of selection sort?
The average-case time complexity of selection sort is O(n²), as it performs the same number of comparisons in average scenarios as it does in worst-case scenarios.
Is selection sort stable?
The traditional version of selection sort is not stable, meaning that equal elements can be reordered. However, with some modifications, it can be made stable.
How does selection sort compare to insertion sort?
Selection sort typically performs worse than insertion sort, especially on partially sorted data, since insertion sort has a best-case time complexity of O(n) in sorted scenarios. Selection sort always operates in O(n²) time.