In C++, you can retrieve environment variables using the `getenv` function from the `<cstdlib>` header, which returns the value of the specified environment variable as a string pointer. Here's a code snippet demonstrating this:
#include <iostream>
#include <cstdlib>
int main() {
const char* path = getenv("PATH");
if (path) {
std::cout << "PATH: " << path << std::endl;
} else {
std::cout << "Environment variable PATH not found." << std::endl;
}
return 0;
}
Understanding Environment Variables
What are Environment Variables?
Environment variables are dynamic values that can affect the way running processes on a computer behave. They can hold configuration settings, paths to important directories, and other data that software can access while executing. Unix-like and Windows operating systems commonly make use of these variables.
In C++, environment variables are often used to retrieve information about the operating environment, making them crucial for tasks like configuring application settings, determining resource locations, or handling execution parameters dynamically.
Why Access Environment Variables in C++?
Accessing environment variables in C++ can be particularly essential for many reasons:
- Dynamic Configuration: Enables applications to change their behaviors based on the environment they are running in.
- Flexibility: Allows developers to avoid hardcoding sensitive data, paths, or configuration parameters, thus enhancing portability.
- Interoperability: Makes it easier to access information set by the operating system or by other applications, fostering smoother interactions across different systems.
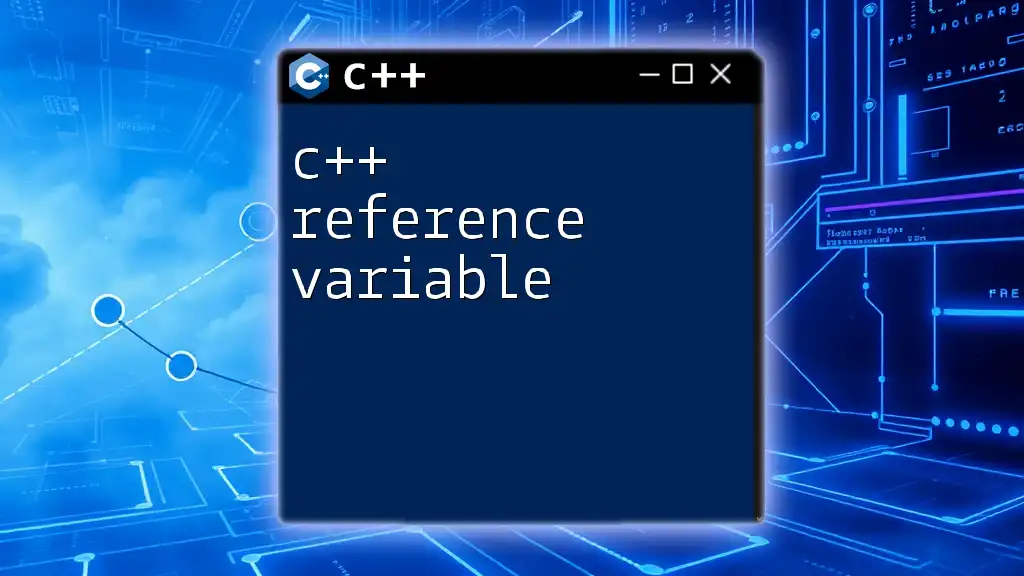
Getting Started with C++
Setting Up Your C++ Development Environment
Before accessing environment variables using C++, it's crucial to have a functioning development environment. Some recommended Integrated Development Environments (IDEs) include:
- Visual Studio: An excellent choice for Windows users.
- Code::Blocks: A cross-platform IDE that supports multiple compilers.
- Eclipse IDE for C/C++: Suitable for those who prefer a robust and flexible development environment.
Once your IDE is set up, ensure you can compile and run C++ programs successfully.
Basic Syntax for Accessing Environment Variables
C++ provides a standard library function, `getenv()`, to access environment variables. This function is essential for retrieving the value of a variable using the following syntax:
char* getenv(const char* name);
- name: This is the name of the environment variable you want to retrieve.
- Return Value: The function returns a pointer to the value of the environment variable if it exists. If the variable does not exist, it returns `nullptr`.
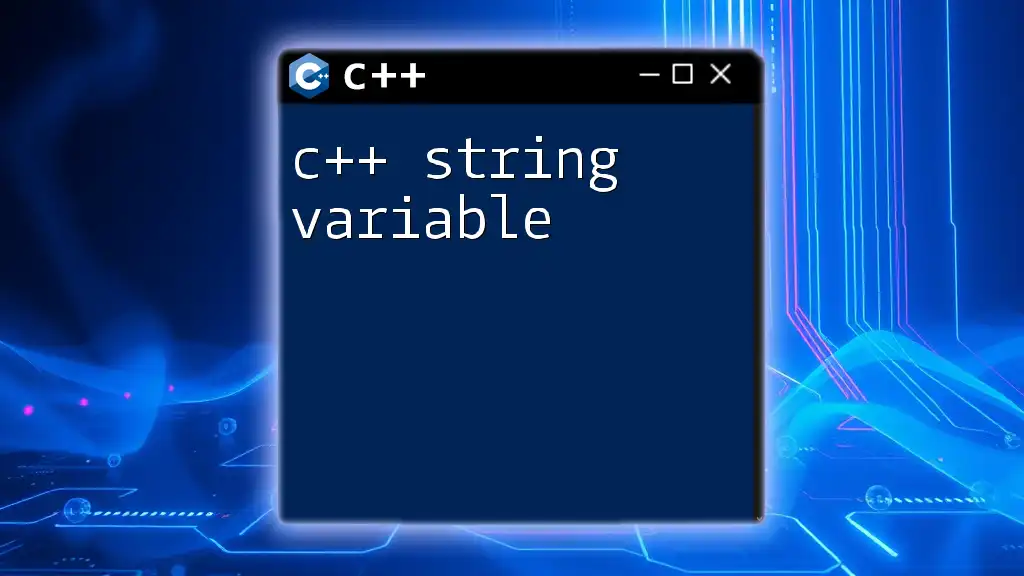
Using `getenv` to Retrieve Environment Variables
How to Use `getenv()`
The essence of using `getenv()` lies in being mindful of the pointer that it returns. A null pointer indicates that the specified environment variable wasn’t found, which is an important aspect to handle gracefully in a program.
Example: Getting a Single Environment Variable
Let’s see how to retrieve a specific environment variable, such as `PATH`, which commonly holds a list of directories where executable files are located.
Here’s a simple code example:
#include <iostream>
#include <cstdlib>
int main() {
const char* path = getenv("PATH");
if (path != nullptr) {
std::cout << "PATH: " << path << std::endl;
} else {
std::cout << "PATH variable not found." << std::endl;
}
return 0;
}
Explanation of the code:
- We include the necessary headers: `<iostream>` for input and output operations, and `<cstdlib>` to use `getenv()`.
- The `getenv()` function is called to retrieve the `PATH` environment variable.
- We check if the returned pointer is `nullptr`. If it is not, we print the variable’s value. If it is `nullptr`, we print a message indicating that the variable was not found.
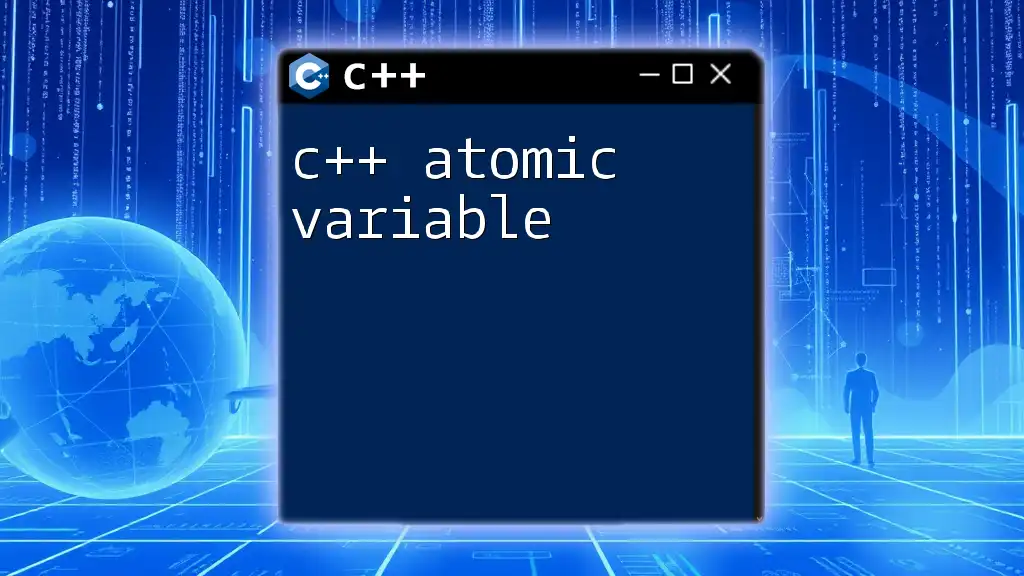
Working with Multiple Environment Variables
Looping Through Environment Variables
There might be a situation where you need to explore all environment variables available to your application. This can be achieved using the `environ` variable, which is an array of strings representing the environment.
Here’s an example of how to loop through each environment variable:
#include <iostream>
#include <cstdlib>
extern char **environ;
int main() {
for (char **env = environ; *env != 0; env++) {
char *thisEnv = *env;
std::cout << thisEnv << std::endl;
}
return 0;
}
Explanation of the code:
- The `extern char **environ;` declaration points to the array of environment variables.
- We iterate through this array, printing each environment variable until we reach the end, denoted by a null pointer.
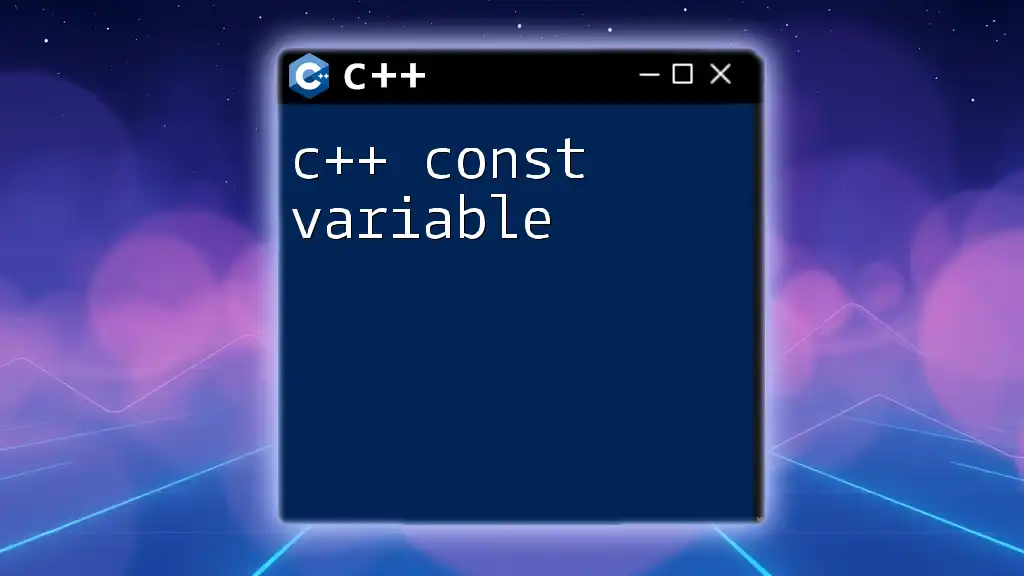
Important Considerations
Security Risks of Environment Variables
While using environment variables can be beneficial, it’s essential to consider potential security risks. Sensitive information (like API keys or passwords) could be exposed if not properly managed. To mitigate these risks:
- Use Only Required Variables: Limit the number of environment variables and the sensitivity of the data they contain.
- Null Checks: Always check for `nullptr` to avoid accessing non-existent variables that could lead to undefined behavior.
Limitations of Using `getenv()`
Even though `getenv()` is widely supported, there are some limitations to be aware of:
- Portability: Not all environments may set up specific variables, leading to inconsistencies.
- Character Encoding: Different platforms may have varying support for multi-byte character encodings, affecting how values are read.
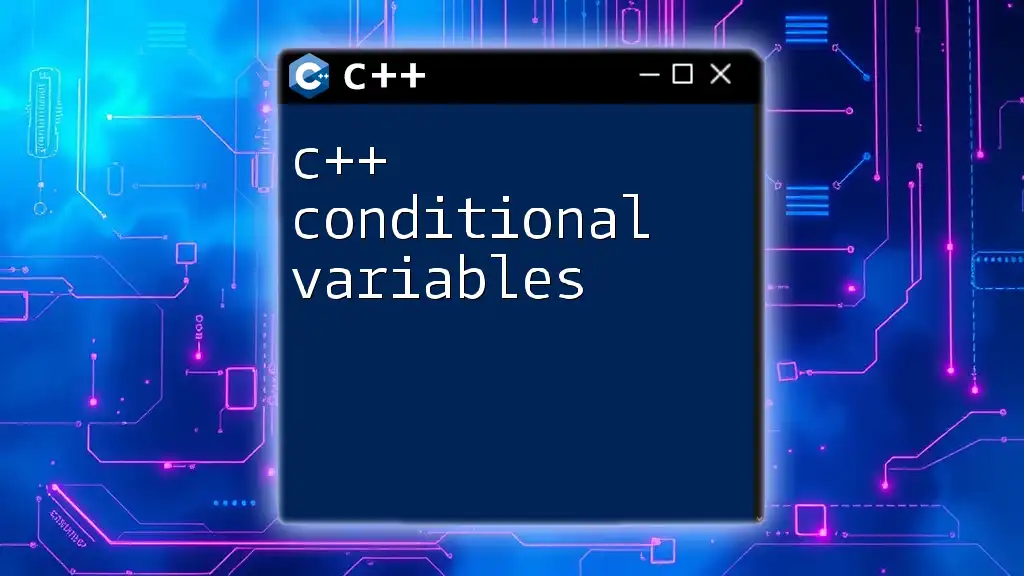
Conclusion
Accessing environment variables in C++ grants developers the ability to configure applications dynamically, enhancing flexibility and adaptability. By utilizing `getenv()` and the `environ` array, you can easily retrieve environment variables and use them in your applications. Experiment with these tools to see how they can streamline your development process and enrich your coding practices.
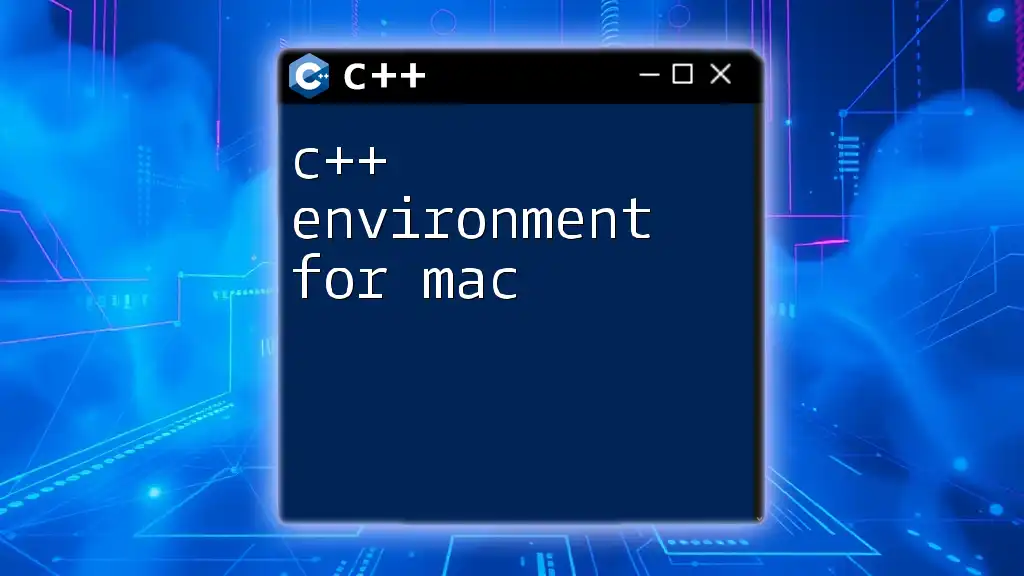
Additional Resources
Recommended Reading and Tutorials
- Books on C++: Consider reputable titles that delve deeply into C++ programming and best practices.
- Online Forums: Platforms like Stack Overflow and C++ communities on GitHub provide community support and assistance for C++ developers.
Community and Support
Engage in discussions within the programming community; sharing knowledge and experiences can significantly enhance your understanding and skills in working with C++ and environment variables.