A C++ union allows you to define a data type that can store different data types in the same memory location, saving space but only one member can hold a value at any given time.
#include <iostream>
using namespace std;
union Data {
int intValue;
float floatValue;
char charValue;
};
int main() {
Data data;
data.intValue = 10;
cout << "Int: " << data.intValue << endl;
data.floatValue = 220.5; // overwrites intValue
cout << "Float: " << data.floatValue << endl; // now shows float value
return 0;
}
What is a Union?
In C++, a union is a special data type that allows storing different data types in the same memory location. Unlike structures and classes, which allocate separate memory for each member, a union allocates a single memory block large enough to hold the largest member only. This means that all members share the same memory space, rendering unions a powerful tool for optimizing memory usage.
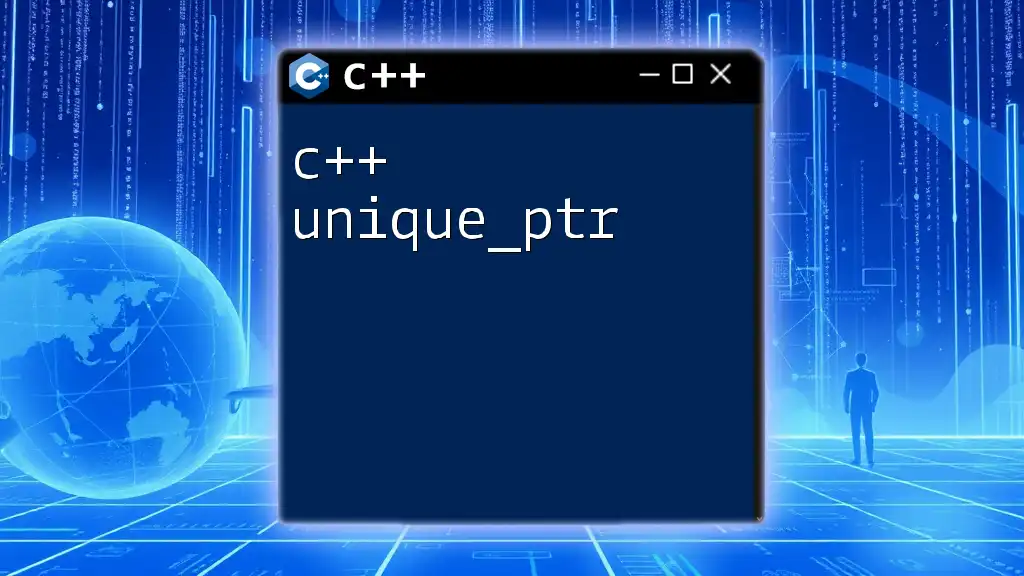
Why Use Unions?
Unions are particularly useful when you need to manage multiple types of data in a memory-efficient manner. They are ideal in cases where a variable may hold different types of data at different times, such as in low-level programming, embedded systems, or when working with hardware interfaces. Unions allow for flexibility and optimized resource usage, making them a favored choice among developers when appropriate.
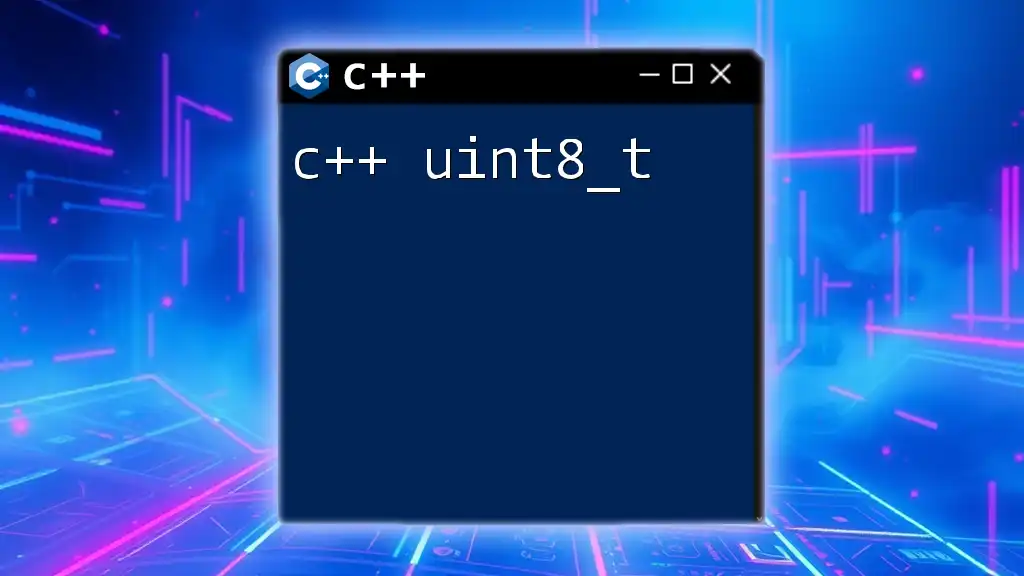
Basic Syntax and Structure of Unions
Declaring a Union
To declare a union in C++, use the following syntax:
union Data {
int integer;
float floating;
char character;
};
This example defines a union named `Data`, which can hold either an integer, a floating-point number, or a character. Only one of these can be active at any one time, and the memory used will be based on the largest member type listed.
Accessing Union Members
You can access members of a union in a similar manner to structures. Here is how to do it:
Data data;
data.integer = 10;
std::cout << "Integer: " << data.integer << std::endl; // Output: Integer: 10
In this snippet, we create an instance of the union `Data` and assign a value to the `integer` member. When we print it, we see the expected output.
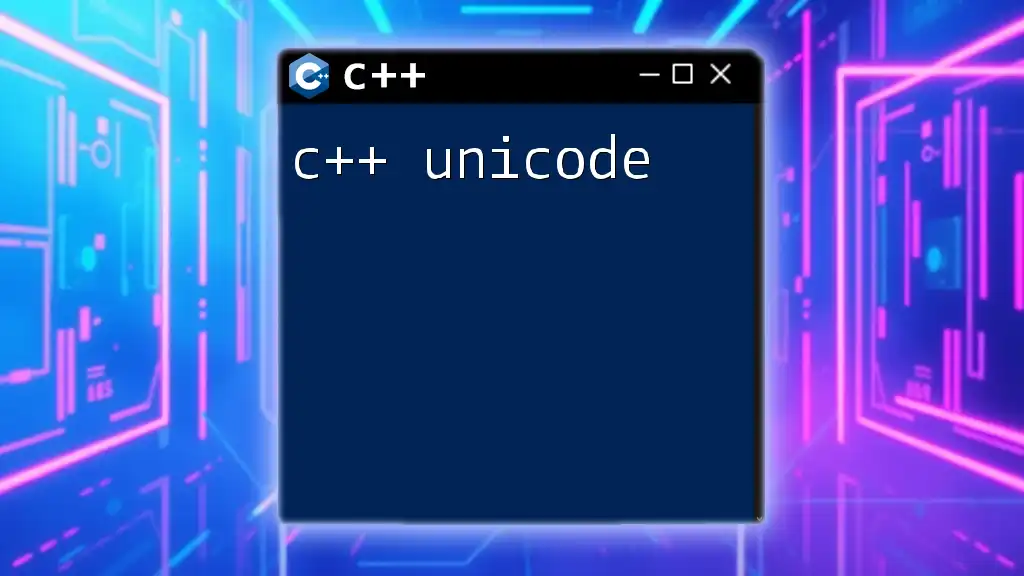
Key Characteristics of Unions
Size and Memory Allocation
One of the most important features of a union is how it allocates memory. The size of the union is determined by the size of its largest member. Consider this example:
Data data;
std::cout << "Size of union: " << sizeof(data) << std::endl; // Typically returns size of largest member
When you run this code, you will notice that the size of `data` matches the size of the largest type defined in the union, which is an `int` or potentially a `float`, depending on your system.
Type Safety Concerns
While unions are efficient, they come at the cost of type safety. Since only one member can be active at a time, accessing an inactive member results in undefined behavior. Therefore, it’s crucial to ensure that you are accessing the correct member at a given time, particularly when dealing with data that may change frequently.
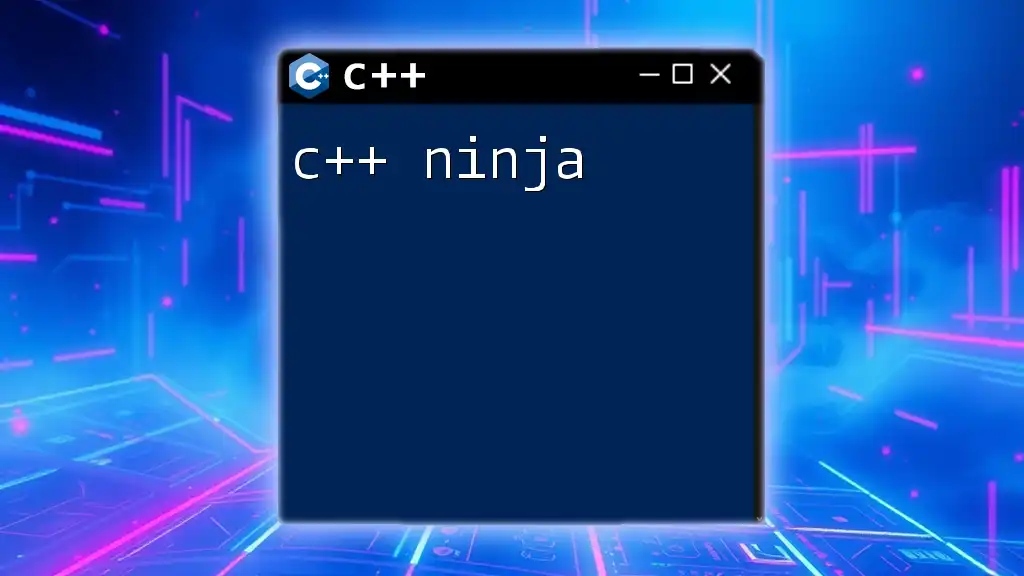
Union Initialization
Different Ways to Initialize Unions
You can initialize a union using aggregate initialization. Here's an example:
Data data = { .integer = 5 }; // C99 style, supported in C++17 and later
In this example, we directly initialize the union with an integer value.
Union Initialization Rules
When initializing unions, only the member that is set during initialization is considered active. Accessing another member without reassigning can lead to unexpected results and data corruption, as the contents of inactive members are not guaranteed.
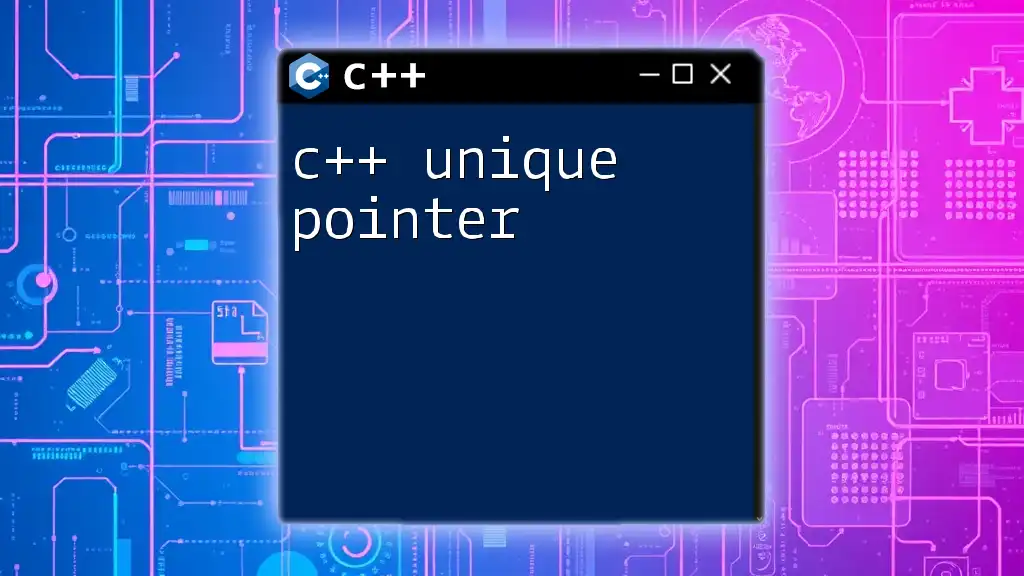
Practical Examples of Unions
Storing Different Types of Data
Unions are exceptionally useful for managing various data types effectively. Here’s a simple example using a union to store both integer and double values:
union Number {
int integer;
double decimal;
};
Number num;
num.integer = 42;
// Later...
num.decimal = 3.14; // overwrites integer
std::cout << "Decimal: " << num.decimal << std::endl; // Output: Decimal: 3.14
In this code, `num.integer` is first assigned a value, but when `num.decimal` is assigned, it overwrites the storage, showcasing how unions can hold only one value at a time.
Combining with Structs
You can incorporate unions within structs to create more complex data types. For instance:
struct Employee {
char name[50];
union {
int id;
float salary;
} info;
};
This structure `Employee` has a union `info` that can either hold an employee's ID or salary. This compact approach utilizes memory wisely by storing relevant information, depending on the current context of use.
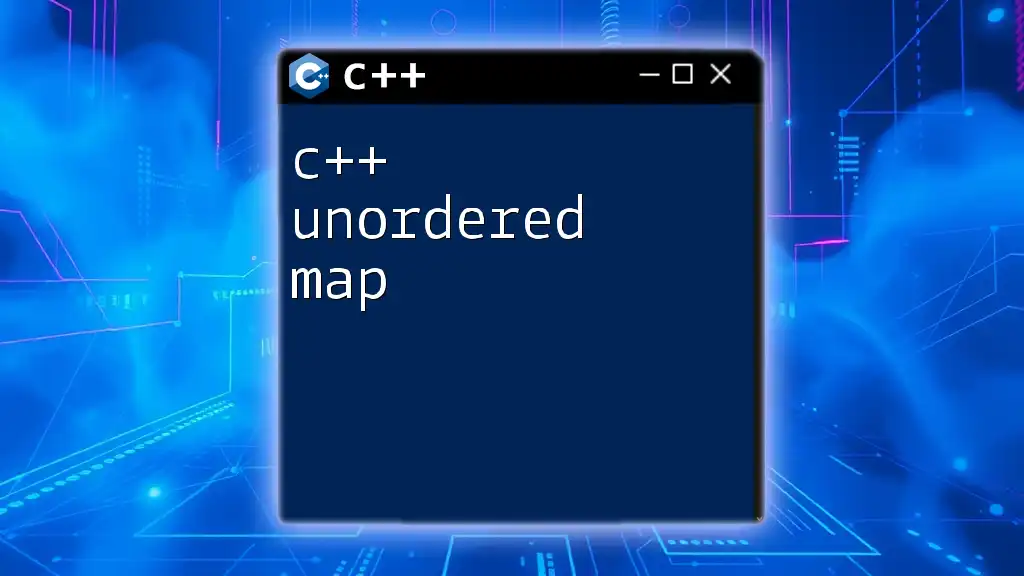
Limitations of Unions
Lack of Constructors and Destructors
Unions in C++ do not support constructors and destructors. This means that you cannot directly declare initialization logic for union members, leading to potential complications when managing complex data types. If you need to perform tasks like resource allocation or deallocation, consider using a struct or class instead.
Data Overwriting and Loss
One of the significant drawbacks of using a union is data overwriting. Since all members share the same memory segment, when one member is assigned a value, the others become inaccessible:
data.integer = 5;
data.floating = 3.14; // overwrites the integer value
std::cout << "Integer: " << data.integer << std::endl; // Output is now undefined
The value stored in `data.integer` is lost when `data.floating` is assigned. This risk demands vigilant tracking of what member is currently valid.
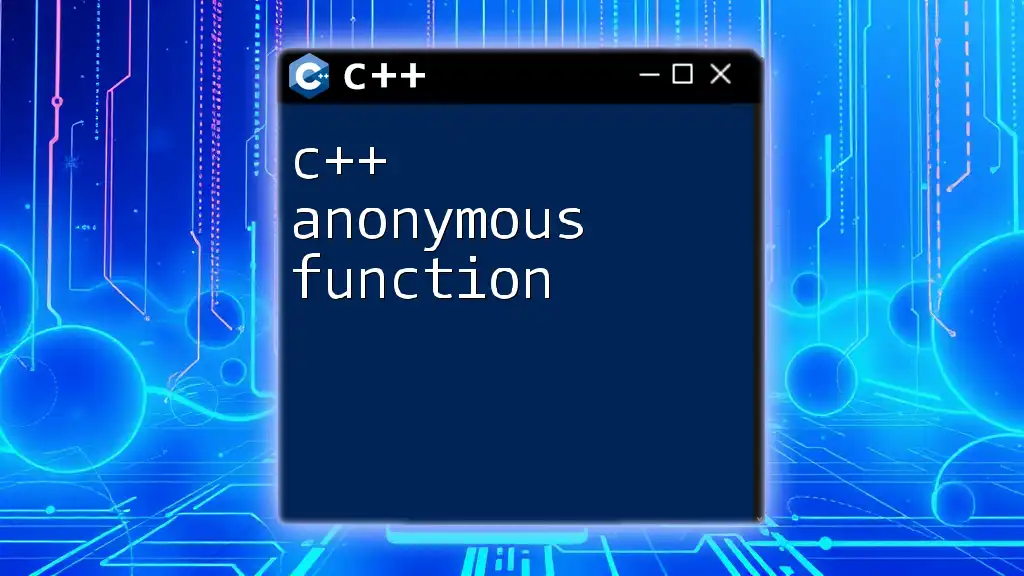
Best Practices for Using Unions
When to Use Unions
Unions are best utilized in scenarios where memory conservation is crucial. They are suitable for embedded systems, interpreters, or cases where a variable's type needs to change dynamically, but always remember to consider type safety.
Ensuring Safety in Union Usage
For safer usage of unions, consider wrapping them in a structured interface. For example, you could employ methods to explicitly manage which member is currently active and ensure proper initialization.
class SafeUnion {
public:
enum Type { NONE, INTEGER, FLOATING };
SafeUnion() : type(NONE) {}
void setInteger(int i) {
integer = i;
type = INTEGER;
}
void setFloating(float f) {
floating = f;
type = FLOATING;
}
int getInteger() {
if (type != INTEGER) throw std::runtime_error("Active member is not an integer");
return integer;
}
private:
union {
int integer;
float floating;
};
Type type;
};
This approach ensures that the user interacts with the union more safely while encapsulating functionality, enhancing both usability and maintainability.
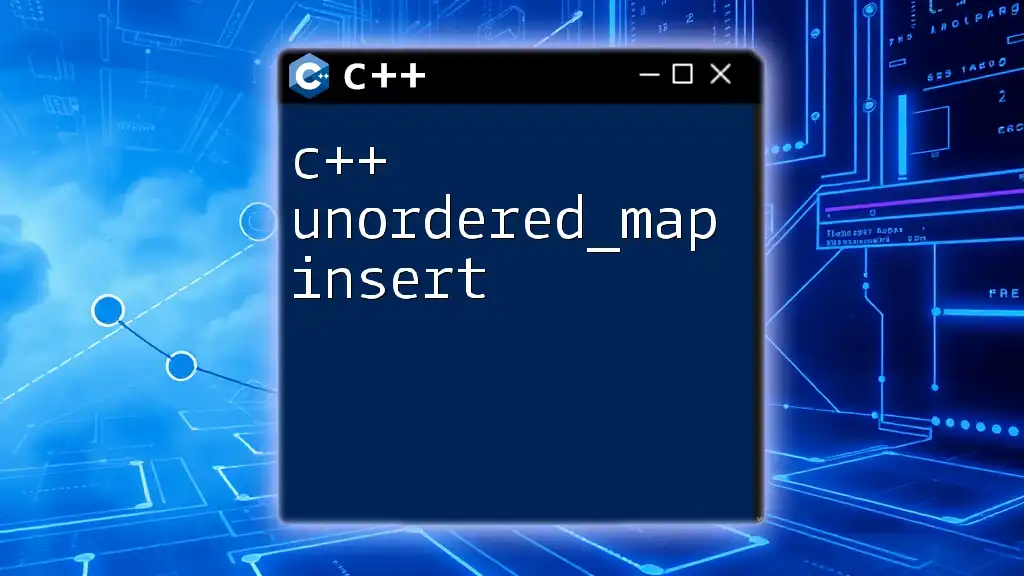
Conclusion
C++ unions provide an efficient way to handle multiple data types using a single memory block. They are versatile and memory-efficient, making them suitable for a variety of programming tasks. However, they come with caveats related to type safety and potential data loss through overwrites. By understanding their capabilities and limitations, developers can leverage unions effectively in their applications, ensuring optimal performance and resource usage. For those interested in mastering unions, further exploration into their use cases and safety practices is highly encouraged.