The `unordered_map::find` function in C++ is used to search for a specific key in an unordered map and returns an iterator to the element if found, or to the end if not found.
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
auto it = myMap.find(2);
if (it != myMap.end()) {
std::cout << "Found: " << it->second << std::endl; // Output: Found: two
} else {
std::cout << "Key not found" << std::endl;
}
return 0;
}
Understanding `unordered_map` Basics
What is `unordered_map`?
An `unordered_map` is a part of the C++ Standard Library designed to store elements in key-value pairs. It utilizes a hash table structure to allow for very efficient average-case time complexity for lookups, inserts, and deletes. The keys are hashed, and the values are stored in an unordered fashion, which makes accessing elements faster but does not maintain any specific order.
The primary distinction between `unordered_map` and a regular `map` is that `maps` store elements in a sorted order while `unordered_maps` do not. This unordered nature allows for average constant time complexity O(1) for search operations, making it a preferred choice for scenarios where quick retrieval is essential.
Key Properties of `unordered_map`
-
Average Time Complexity: The average-case complexity for search, insert, and delete operations is O(1) due to the underlying hash table structure.
-
Storage Method: `unordered_map` uses a hash table for storage, which means its performance heavily relies on the efficiency of the hash function used to compute the index for each key.
-
Hash Function and Equality Comparison: To use custom types as keys, appropriate hash functions and equality comparison operators must be defined.
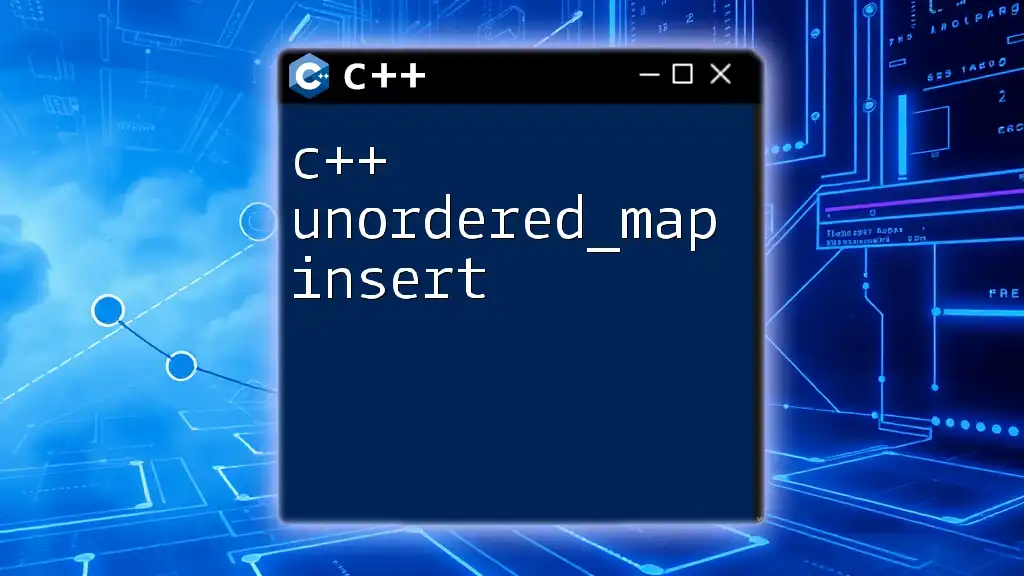
Using `find` with `unordered_map`
Introduction to `find` Function
The `find` function is an essential component of `unordered_map`, used to locate an element by its key. It allows you to check if a key exists in the map without triggering an insertion.
The syntax of the `find` function is as follows:
iterator find(const Key& k);
In this function, `k` is the key you're searching for, and the return value is an iterator pointing to the element associated with that key. If the key does not exist, the function returns an iterator to the end of the map.
Signature of the `find` Function
The signature of the `find` function is critical for understanding its behavior. It accepts one parameter, the key to search for, and returns an iterator:
-
Return Value: If the element is found, the returned iterator points to it; otherwise, it points to the end of the map.
-
Best Practices: Always compare the returned iterator with `unordered_map::end()` to check if the key was found:
std::unordered_map<int, std::string> myMap;
auto it = myMap.find(key);
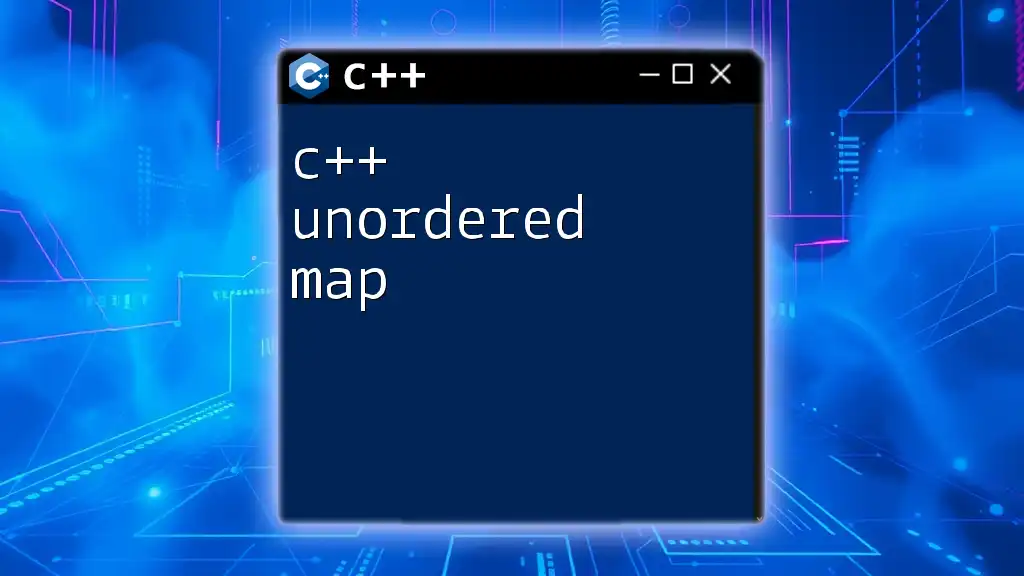
Examples of Using `find`
Basic Example of `find`
Let’s illustrate how to use the `find` function with a straightforward example using integers as keys:
std::unordered_map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
auto it = myMap.find(2);
if (it != myMap.end()) {
std::cout << "Found: " << it->second << std::endl;
} else {
std::cout << "Key not found" << std::endl;
}
In this example, we create an `unordered_map` and populate it with a few key-value pairs. We then search for the key `2`. If found, the program prints "Found: Two". If the key were absent, it would print "Key not found". This illustrates the fundamental use of the `find` function.
Handling Not Found Cases
Understanding how to handle cases where a key is not present is essential. Continuing from our previous example:
auto it = myMap.find(4);
if (it == myMap.end()) {
std::cout << "Key not found" << std::endl;
}
In this example, trying to find the key `4`, which does not exist in the `unordered_map`, will lead to the output "Key not found". Always ensure to verify the existence of the key before attempting to dereference the iterator.
Using `find` with Custom Types
`unordered_map` is flexible enough to work with user-defined types as keys, provided you implement a hash function and equality operator. Consider this example:
#include <iostream>
#include <string>
#include <unordered_map>
struct Person {
std::string name;
int age;
bool operator==(const Person& other) const {
return name == other.name && age == other.age;
}
};
namespace std {
template <>
struct hash<Person> {
size_t operator()(const Person& p) const {
return hash<string>()(p.name) ^ hash<int>()(p.age);
}
};
}
int main() {
std::unordered_map<Person, std::string> personMap;
personMap[{ "Alice", 30 }] = "Manager";
personMap[{ "Bob", 25 }] = "Developer";
Person keyToFind = { "Alice", 30 };
auto it = personMap.find(keyToFind);
if (it != personMap.end()) {
std::cout << "Found: " << it->second << std::endl;
}
}
In this example, a `Person` struct is defined, along with a specialized hash function to enable using `Person` objects as keys. The search for the key `Alice, 30` returns the associated title.
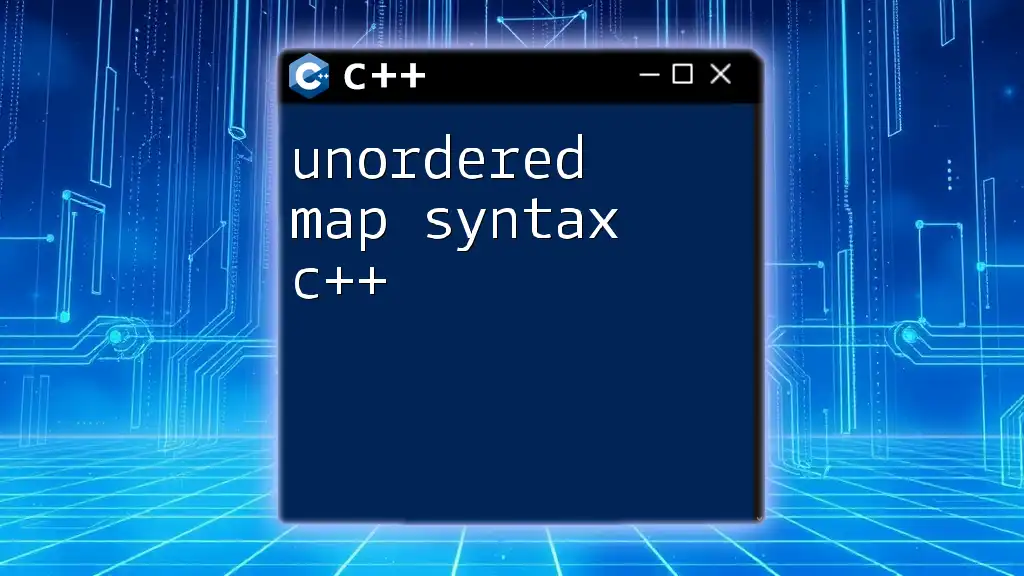
Common Errors and Debugging
Common Mistakes with `find`
When using `find`, several common errors may occur:
-
Key Not Found: Failing to check if the key exists can lead to dereferencing a non-valid iterator.
-
Incorrect Type for Key: Ensure that the key type passed to `find` matches the key type defined in the `unordered_map`.
-
Not Checking Iterator Validity: Always compare the result of `find` with `unordered_map::end()` to avoid runtime errors.
Debugging Tips
Debugging issues related to `find` can be accomplished through:
-
Using Logging: Print statements highlighting keys being searched and the results can aid in diagnosing issues.
-
Assertions: Utilize assertions to handle cases where expected outcomes are not met.
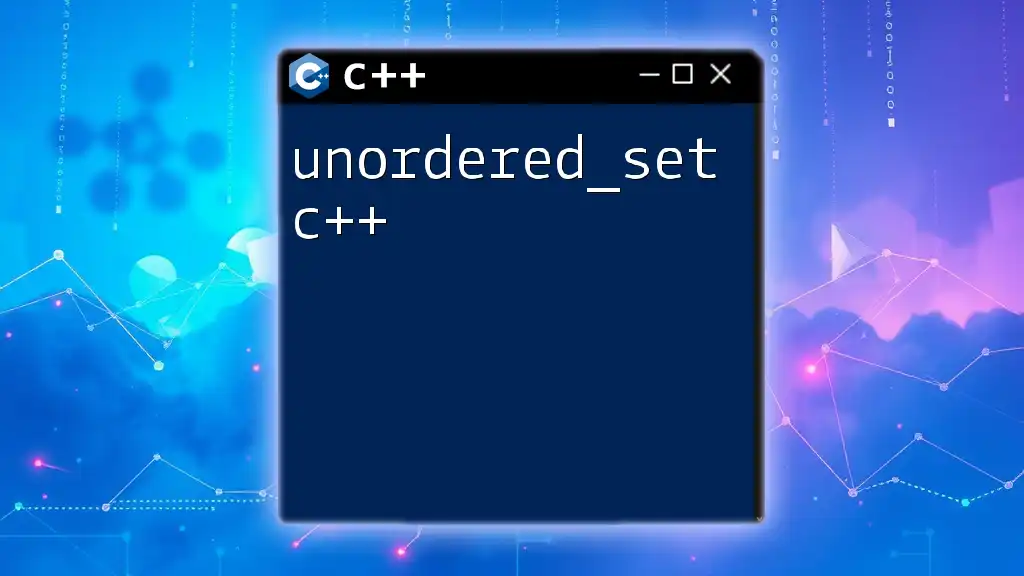
Performance Considerations
Complexity of `find`
Understanding the complexity of `find` is important for performance tuning:
-
Average-Case Time Complexity: The average-case time complexity is O(1), making it extremely efficient for search operations.
-
Worst-Case Time Complexity: In cases of high collision rates (when multiple keys hash to the same index), the complexity can degrade to O(n), but such cases are rare with a good hash function.
When to Use `unordered_map`
`unordered_map` is typically the best choice when:
- High-frequency key-based lookups are required, and the order of elements does not matter.
- The overhead of maintaining sorted elements (as in a `map`) is unnecessary.
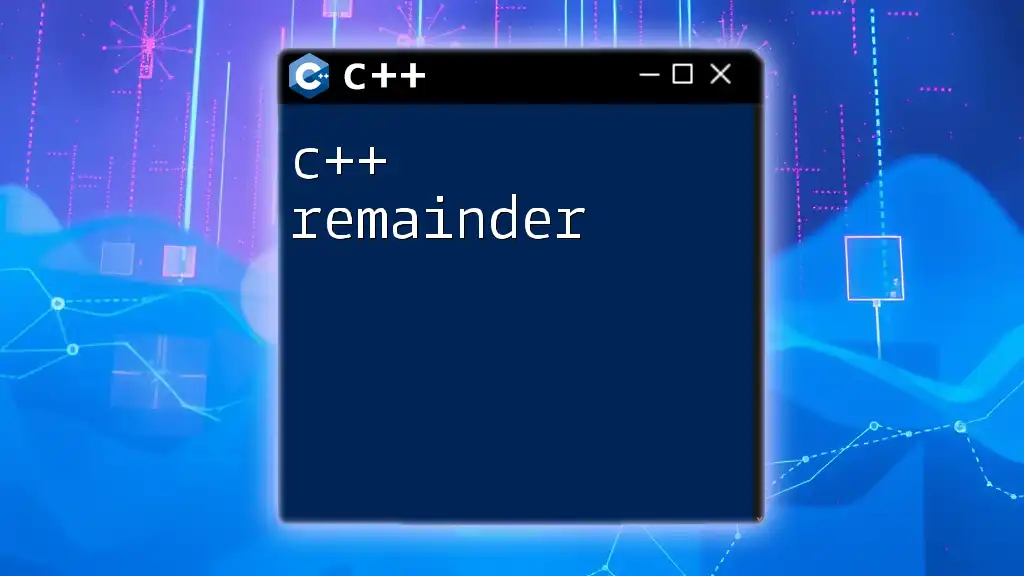
Conclusion
In summary, the `find` function of C++ unordered_map is a powerful tool that allows for efficient key searching within data collections. By grasping how to implement and utilize `find`, you can write efficient C++ programs that take full advantage of unordered associative containers.
For further learning, explore resources like C++ documentation, participate in coding forums, or consider online courses focused on STL and C++ data structures. Practice is essential to deepen your understanding of concepts like unordered_map and its many capabilities.
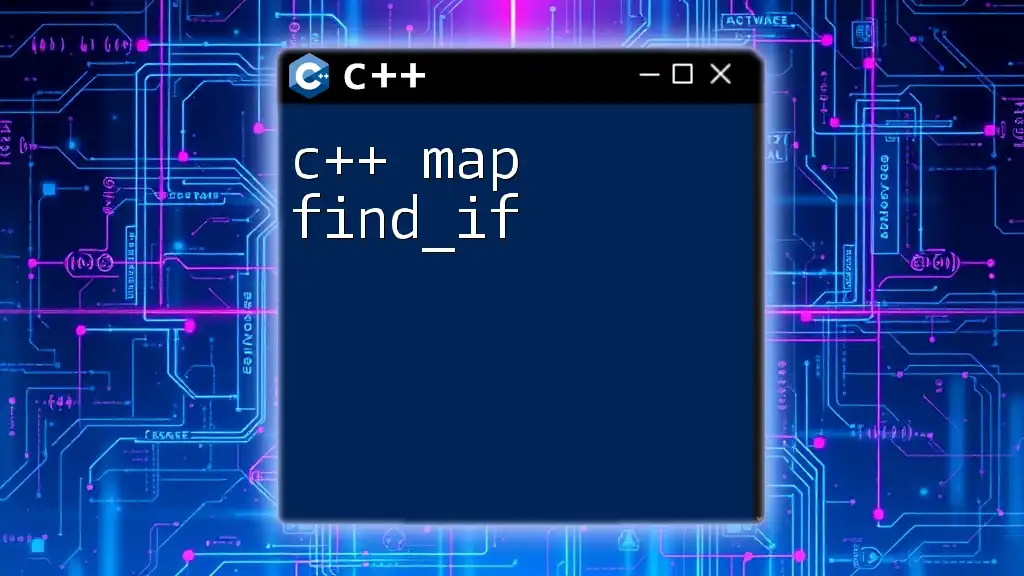
FAQs
-
What happens if I try to find a key not present in the `unordered_map`? The `find` function will return an iterator pointing to the `end()` of the map, indicating that the key was not found.
-
How does the `unordered_map` handle collisions? Collisions are managed through a technique called chaining or open addressing, allowing multiple entries to occupy the same index.
-
Can I use custom objects as keys in an `unordered_map`? If so, how? Yes, to use custom objects, you need to provide a hash function and an equality operator to define how the object should be compared.