In C++, an unordered map is a collection of key-value pairs that allows for fast retrieval and insertion using hash tables, and is defined using the `std::unordered_map` syntax.
Here's a simple example of how to declare and use an unordered map in C++:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> ages;
ages["Alice"] = 30;
ages["Bob"] = 25;
std::cout << "Alice's age: " << ages["Alice"] << std::endl;
return 0;
}
What is an Unordered Map?
An unordered map is a part of the C++ Standard Template Library (STL) that allows you to store and manage key-value pairs. Unlike ordered maps (like `std::map`), unordered maps do not maintain the order of elements; instead, they organize elements in a hash table which optimizes the speed of data retrieval.
The main features of unordered maps include:
- Fast average-time complexity: Unordered maps offer average time complexities of O(1) for operations like insertions, deletions, and lookups.
- Hashing: They use hash functions to convert keys into a unique index in a table where values can be stored.
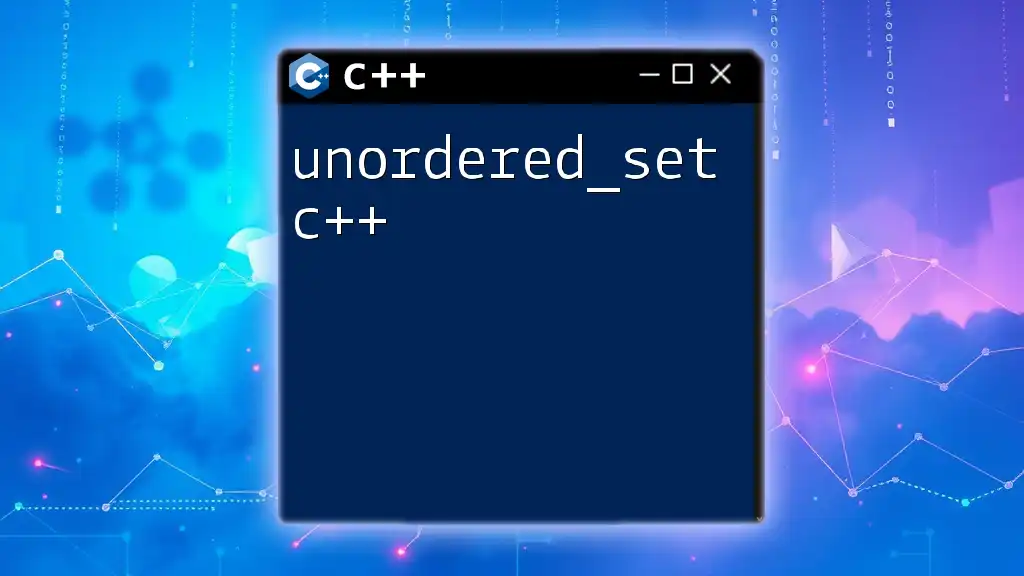
Benefits of Using Unordered Maps
Using unordered maps in your C++ applications can provide several advantages:
- Efficiency: With their O(1) average time complexity, unordered maps are generally faster for large datasets compared to other associative containers, making them an ideal choice for applications requiring frequent insertions and lookups.
- Flexibility: Unordered maps accommodate various data types for both keys and values, allowing for a wide range of applications.
- Ease of Use: The syntax for working with unordered maps is straightforward, making it an accessible data structure for both beginners and experienced developers.
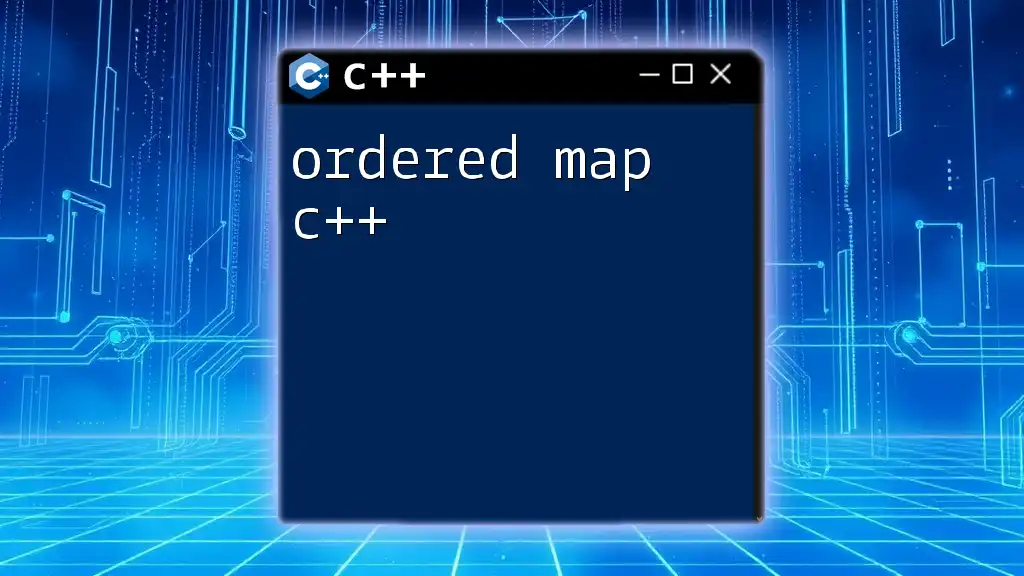
Understanding the Syntax of Unordered Maps
Basic Syntax of Unordered Maps in C++
To declare an unordered map, use the following syntax:
std::unordered_map<KeyType, ValueType> myMap;
Before using an unordered map, it’s essential to include the necessary header:
#include <unordered_map>
Initializing Unordered Maps
Unordered maps can be initialized in different ways:
-
With a specific size: You can initialize an unordered map with a predefined size and a specific bucket count, using the following syntax:
std::unordered_map<int, std::string> myMap(10);
-
Using initializer lists: You can also use initializer lists for a more succinct and convenient initialization:
std::unordered_map<int, std::string> myMap = { {1, "One"}, {2, "Two"}, {3, "Three"} };
Key Operations with Unordered Maps
Adding Elements
To insert elements into an unordered map, use the `insert` method:
myMap.insert({4, "Four"});
Alternatively, you can also use the `[]` operator for insertion. If the key doesn’t exist in the map, it will be created:
myMap[5] = "Five";
Accessing Elements
Accessing values in an unordered map is straightforward. You can retrieve the value associated with a key as follows:
std::string value = myMap[2]; // Returns "Two"
Modifying Elements
Updating the value associated with a specific key can be done easily:
myMap[2] = "Updated Two";
This syntax allows you to overwrite the existing value with a new one.
Removing Elements
To erase an element by its key, you can use the `erase` method:
myMap.erase(3); // Removes the element with key 3
If you attempt to erase a key that does not exist, it will have no effect, keeping the map intact.
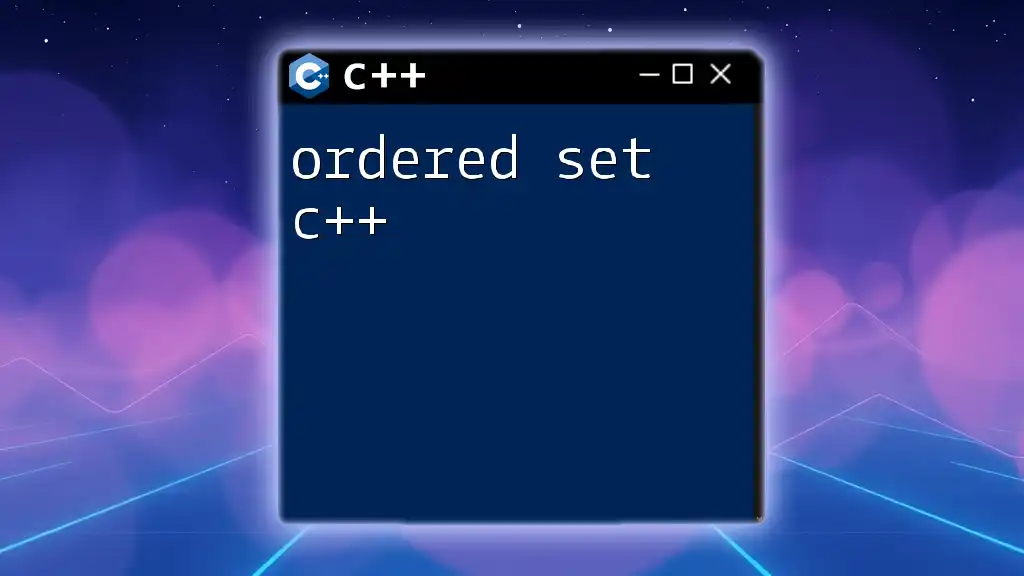
Iterating through Unordered Maps
Using Iterators
Iterators can be used to traverse through an unordered map. An example of this syntax is shown below:
for(auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << " : " << it->second << std::endl;
}
This allows you to access both keys and values during iteration.
Range-based For Loop
C++11 introduced range-based for loops, which provides a cleaner and more concise way to iterate through elements:
for (const auto& pair : myMap) {
std::cout << pair.first << " : " << pair.second << std::endl;
}
This method is more readable and simplifies the syntax, particularly for beginners.
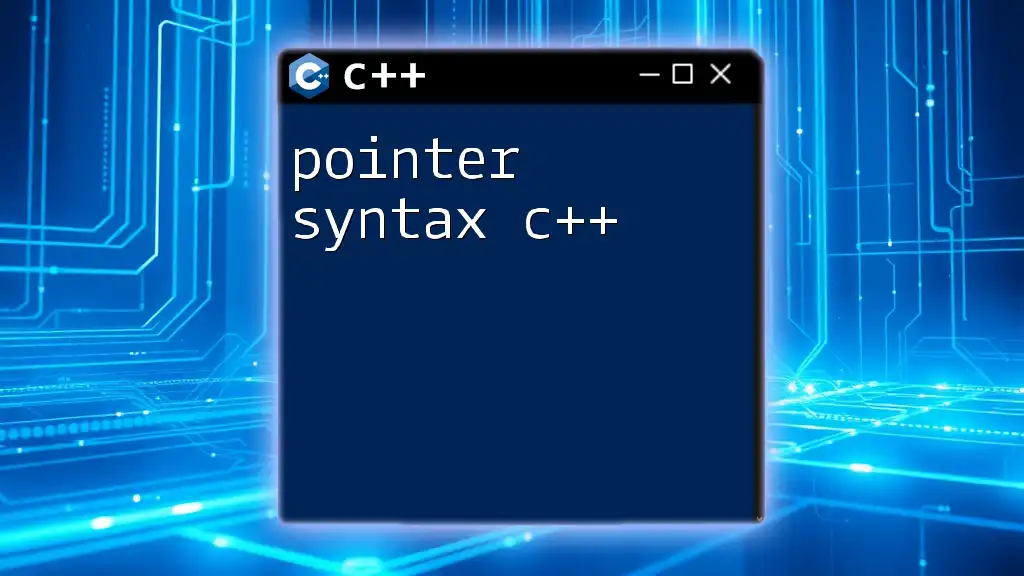
Common Functions and Methods
Size and Capacity
To obtain the number of elements in the map as well as the number of buckets, you can use the following methods:
size_t mapSize = myMap.size();
size_t bucketCount = myMap.bucket_count();
These methods quantitatively describe your unordered_map's current size and its capacity.
Checking for Element Existence
To check if a key exists in the unordered map, the `find` method serves as a reliable approach:
if (myMap.find(2) != myMap.end()) {
// Key exists
}
If `find` returns an iterator pointing to the end of the map, it means the key is not present.
Clearing the Unordered Map
To remove all elements from an unordered map, the `clear` method is your go-to solution:
myMap.clear();
This effectively resets the map, preparing it for new data entries.
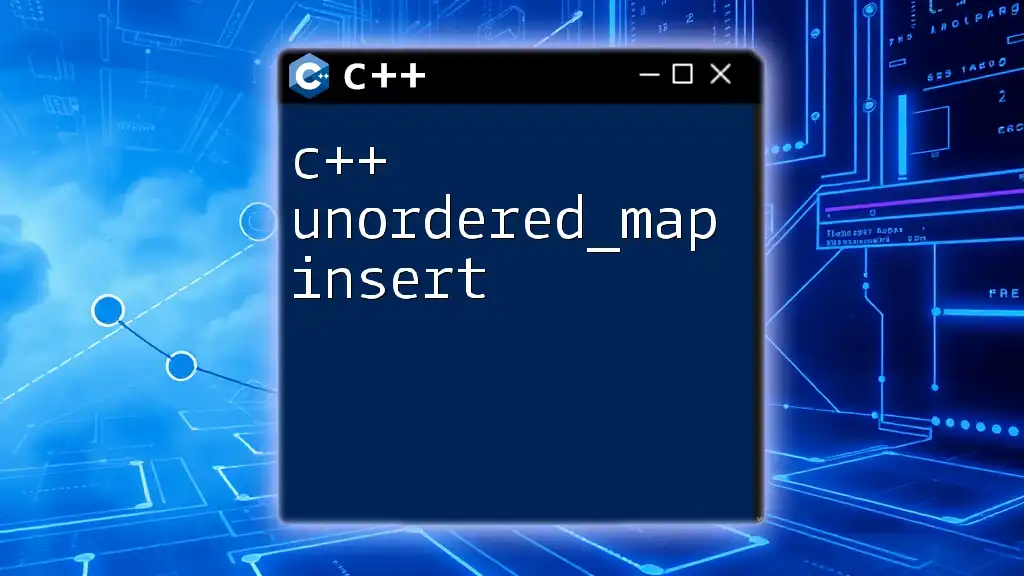
Performance Considerations
Time Complexity of Unordered Maps
The average and worst-case time complexities for unordered maps highlight their efficiency:
- Insertion: O(1) average, O(n) worst case
- Deletion: O(1) average, O(n) worst case
- Access: O(1) average, O(n) worst case
When performance is critical and order is not necessary, unordered maps are often the ideal choice.
Hash Functions and Collisions
Unordered maps rely on hash functions to store and retrieve keys effectively. However, collisions—instances where different keys produce the same hash value—can occur. C++ manages this by resolving collisions through chaining or open addressing, but understanding this concept is crucial for optimizing performance with unordered maps.
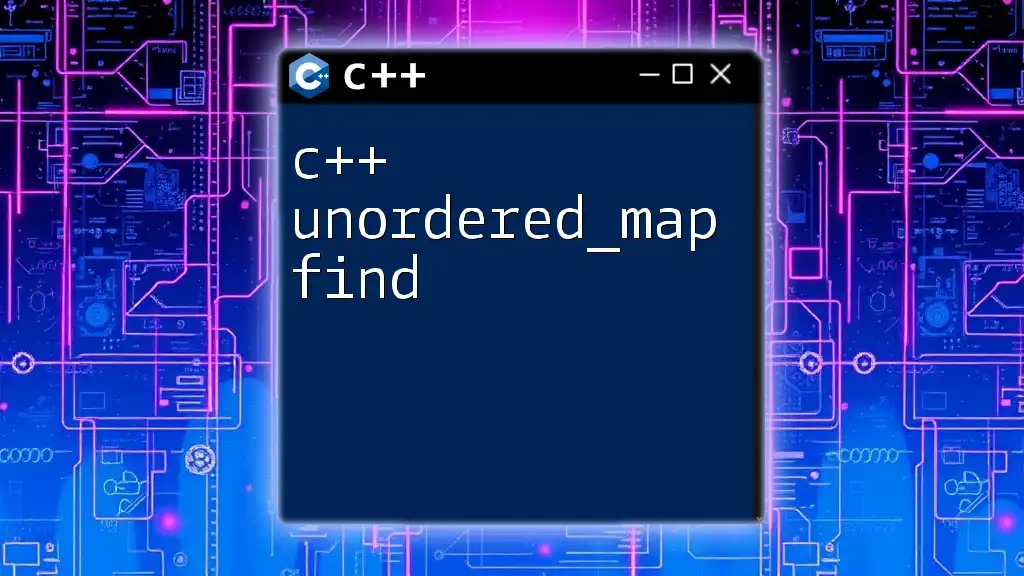
Conclusion
In summary, mastering the unordered map syntax in C++ empowers developers to create efficient, high-performance applications. With benefits like fast lookups, flexible data types, and simple syntax, unordered maps are a vital tool within the STL toolkit. Practice implementing these structures to gain a deeper understanding and enhance your programming capabilities.