Standard input in C++ allows users to read data from the console using the `cin` object, which is commonly utilized in interactive programs.
Here’s a simple example of using standard input in C++:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << "You entered: " << num << endl;
return 0;
}
What is Standard Input?
Standard input is a fundamental concept in C++ that allows a program to receive data from external sources, such as the keyboard. This feature is crucial for interactive applications where user input is required. Understanding how to utilize standard input effectively can significantly enhance the functionality and interactivity of your C++ programs.
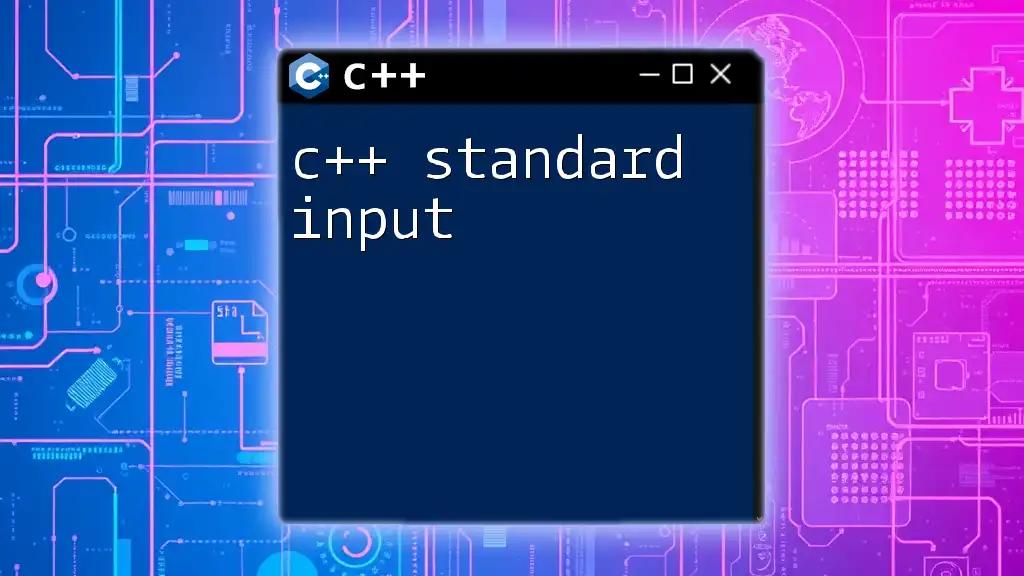
How Does Standard Input Work in C++?
In C++, standard input primarily utilizes input streams, which are abstractions that represent a source of data. The `cin` object is a core component of standard input, allowing developers to read data from the keyboard. When you use `cin`, you can gather various types of information from users, making your programs responsive and dynamic.
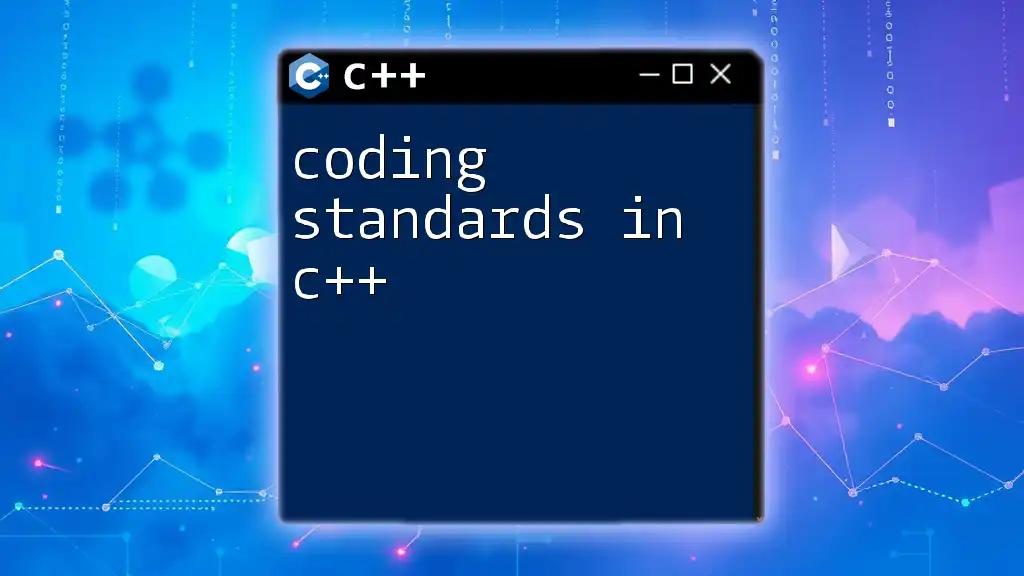
Setting Up Your C++ Environment for Input
Required Libraries
To work with standard input in C++, you'll need to include the necessary library at the beginning of your program:
#include <iostream>
This library contains the definitions for `cin`, `cout`, and other standard stream objects.
Compiling and Running C++ Programs
When you're ready to test your C++ code, you can compile it using g++, a widely-used C++ compiler. The basic command to compile your code is:
g++ -o my_program my_program.cpp
After compilation, you can run your program with the command:
./my_program
![Understanding Unsigned Int in C++ [Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fu%2Funsigned-int-cpp.webp&w=1080&q=75)
Using `cin` for Standard Input
Basic Syntax of `cin`
The syntax for using `cin` is straightforward. A simple command like the following reads user input and stores it in a variable:
std::cin >> variable;
This line waits for user input and assigns it to `variable`, making it an essential part of interactive C++ applications.
Reading Different Data Types
Reading Integers
Reading integers from standard input is simple using `cin`. Here's a basic example:
int num;
std::cin >> num;
In this case, when a user inputs a number, it is stored in the variable `num`. The program will wait until the user provides a value followed by the Enter key.
Reading Floating-Point Numbers
To read floating-point numbers, the procedure is similar:
double decimalNum;
std::cin >> decimalNum;
It is essential to note that if the user inputs a non-numeric value, the input operation will fail, which brings us to the topic of error handling.
Reading Strings
For reading single words, `cin` functions well:
std::string word;
std::cin >> word;
However, if you want to capture a whole line, including spaces, you should use `getline()`:
std::string fullString;
std::getline(std::cin, fullString);
This command ensures that everything the user inputs until they hit Enter is captured, which is particularly useful for gathering complete sentences or phrases.
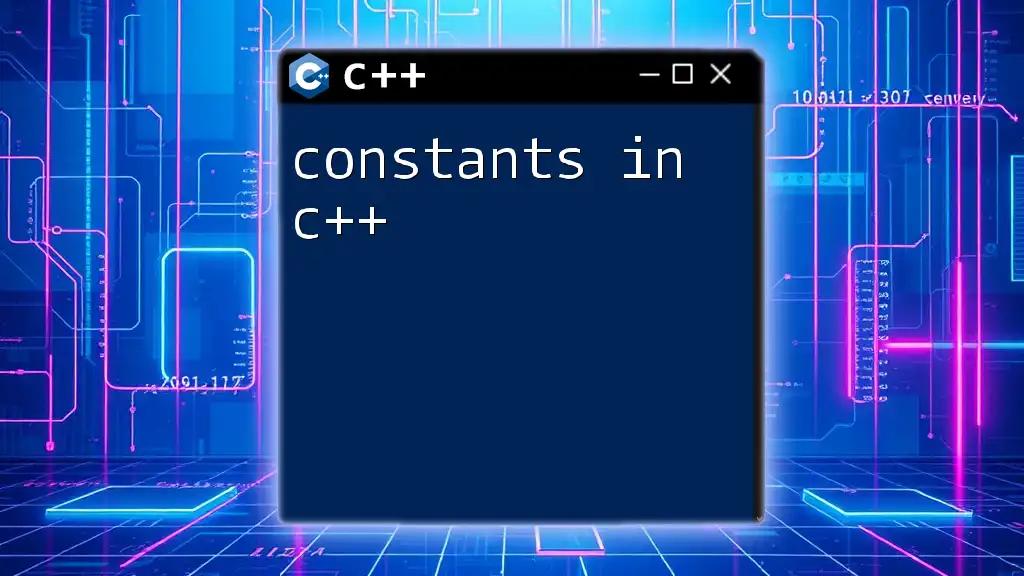
Error Handling with Standard Input
Common Input Errors
When dealing with user input, errors are inevitable. One common error is a type mismatch, where the user inputs data that does not match the expected type (e.g., entering a letter when a number is expected).
Using `cin.fail()`
To handle input failures gracefully, you can use the `cin.fail()` method. After an input attempt, check if it succeeded. If it didn't, you can clear the error state and ignore the invalid input:
if (std::cin.fail()) {
std::cin.clear(); // Clears the error flag
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Discard invalid input
}
This approach allows your program to remain responsive and adaptable, prompting the user for input again if something goes wrong.

Advanced Standard Input Techniques
Input Validation
Input validation ensures that the data users provide is both appropriate and usable. You can enforce restrictions, like ensuring a value falls within a certain range. For example:
int age;
do {
std::cout << "Enter your age: ";
std::cin >> age;
} while (age < 0 || age > 120);
In this snippet, the program repeatedly prompts the user until a valid age is entered, improving the robustness of your application.
Using Input with Loops
You can also incorporate loops to repeatedly read user input until the user requests to stop. Here’s an example:
std::string input;
while (true) {
std::cin >> input;
if (input == "exit") break; // Exits the loop if the user types "exit"
}
This method provides a simple way to continuously gather input, making your application more interactive.
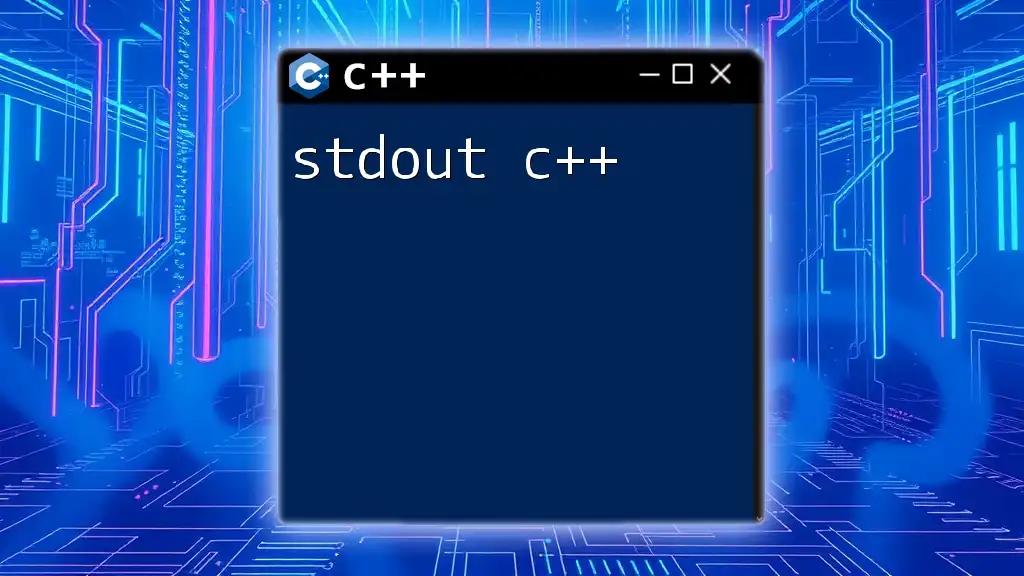
Standard Input vs. Other Input Methods
Comparing `cin` with `getchar()` and `getline()`
While `cin` is a popular choice for reading input, other methods such as `getchar()` and `getline()` are also available.
-
`getchar()`: Best for reading single characters. It can be useful in specific scenarios where you only need one character at a time.
Example:
char ch; ch = getchar();
-
`getline()`: Best for reading entire lines of text, especially those with spaces. Unlike `cin`, which stops at whitespace, `getline()` captures everything until the line break.
Choosing the right input method depends on the needs of your application and the expected user input.

Conclusion
Understanding standard input in C++ is crucial for creating interactive applications that are responsive to user input. By mastering the use of `cin`, input validation, and error handling, you can enhance the quality and robustness of your programs. Continually practicing these concepts will enable you to handle various input scenarios gracefully, making your software more user-friendly and efficient.
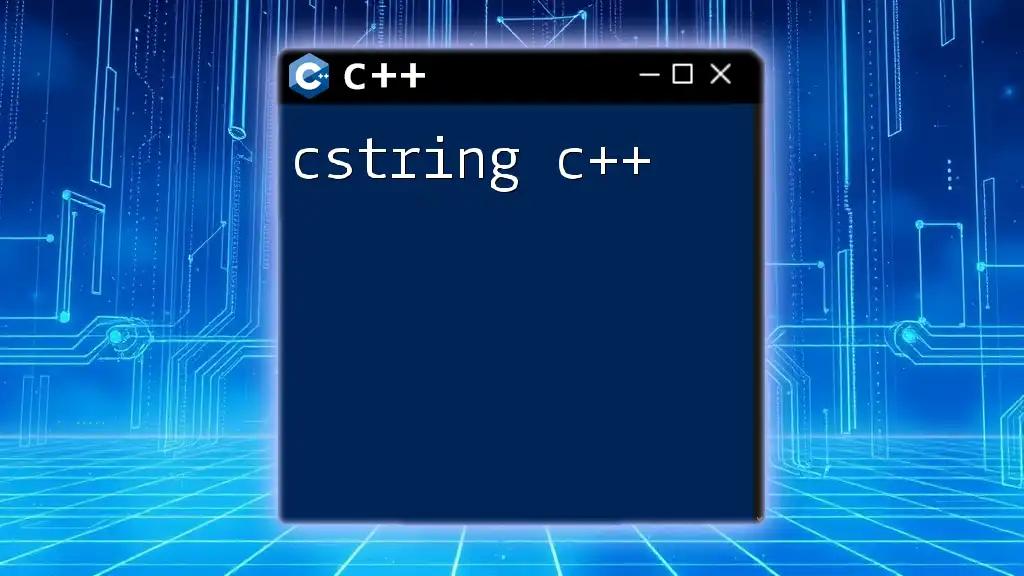
Additional Resources
To delve deeper into C++ programming and standard input handling, consider exploring recommended books and online courses that can further sharpen your skills and broaden your understanding of this powerful language.