UE5 Enhanced Input in C++ provides a robust framework for implementing player input handling, allowing developers to easily customize and extend input mappings.
Here's a simple example demonstrating how to set up an action mapping using Enhanced Input in C++:
#include "EnhancedInputComponent.h"
#include "EnhancedInputSubsystems.h"
// Assuming this is within your Player Controller or Character class
void AMyPlayerController::SetupInputComponent()
{
Super::SetupInputComponent();
// Enable Enhanced Input system
UEnhancedInputLocalPlayerSubsystem* EnhancedInputSubsystem = GetLocalPlayer()->GetSubsystem<UEnhancedInputLocalPlayerSubsystem>();
if (EnhancedInputSubsystem)
{
EnhancedInputSubsystem->AddMappingContext(MyMappingContext, 0);
}
// Bind action
InputComponent->BindAction(MyAction, ETriggerEvent::Triggered, this, &AMyPlayerController::MyActionMethod);
}
Make sure to define `MyMappingContext` and `MyAction` prior to this, and implement `MyActionMethod()` to handle the input response.
Understanding Input Systems in Unreal Engine 5
What is Input in Unreal Engine?
Input handling is a fundamental aspect of game development, enabling player interaction with the game environment. In Unreal Engine, input management allows developers to capture and respond to player actions effectively. Understanding how to access and process input is essential for creating engaging gameplay.
Traditionally, input handling in Unreal Engine has relied on pre-set key bindings and responses. However, this can be limiting, especially in complex games. This is where the Enhanced Input system comes into play, offering a more flexible and powerful approach.
Overview of Enhanced Input System
The Enhanced Input system introduces several key features that significantly improve input management in Unreal Engine. This system allows developers to create more responsive and adaptable input structures that can streamline gameplay experiences.
Key advantages of Enhanced Input include:
- Dynamic Input Mappings: Easily switch inputs based on the game context (e.g., switching controls during combat or exploration).
- Composite Actions: Combine multiple input actions into a single response, allowing for more complex gameplay mechanics.
- Improved Input Debugging: Access detailed input state information, making it easier to troubleshoot issues and refine gameplay.
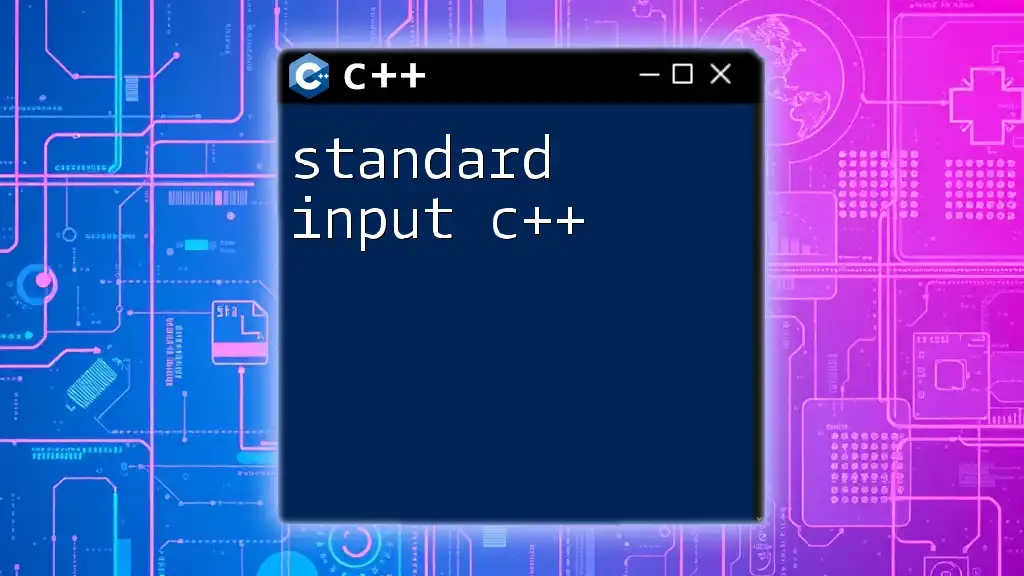
Getting Started with Enhanced Input in C++
Setting Up Your UE5 Project
To begin, you’ll want to create a new Unreal Engine 5 project. Follow these steps:
- Launch Unreal Engine and select "New Project."
- Choose a template (e.g., First Person or Third Person) that suits your game style.
- Name your project and create it.
Once your project is set up, enable the Enhanced Input Plugin:
- Go to Edit -> Plugins in the Unreal Engine menu.
- Search for “Enhanced Input” and check the box to enable it.
- Restart the Unreal Engine editor to apply changes.
To confirm the plugin is enabled, navigate to Edit -> Project Settings -> Plugins, and ensure that the Enhanced Input Plugin appears in your enabled plugins list.
Creating Input Actions and Mappings
Defining Input Actions
Input actions represent specific player commands, such as jumping, moving, or shooting. To create an Input Action, follow these steps:
- Go to Content Browser, right-click in the directory, and select Input -> Input Action.
- Name your action (e.g., `JumpAction`) and set its properties to fit your design needs.
The following code snippet demonstrates how to create an Input Action in C++:
#include "InputAction.h"
UInputAction* JumpAction = NewObject<UInputAction>();
JumpAction->SetName(TEXT("JumpAction"));
JumpAction->ValueType = EInputActionValueType::Boolean;
Setting Up Input Mapping Contexts
Input mapping contexts allow you to segregate various types of input based on the game state. To manage input mapping effectively:
- In Content Browser, right-click and select Input -> Input Mapping Context.
- Name the context (e.g., `DefaultMappingContext`).
- Add your created actions to the context with the respective keys or buttons.
For example, mapping an action to the space bar for jumping:
DefaultMappingContext->AddActionMapping(JumpAction, EKeys::Space);
![Understanding Unsigned Int in C++ [Quick Guide]](/images/posts/u/unsigned-int-cpp.webp)
Implementing Enhanced Input in C++
Writing Your First Enhanced Input Code
Adding Input Components to Actors
Every Actor that requires input must have the Enhanced Input capability. Generally, this is accomplished within your Character class.
Create an Input Component in your Player Character class as follows:
#include "EnhancedInputComponent.h"
void AMyCharacter::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
Super::SetupPlayerInputComponent(PlayerInputComponent);
UEnhancedInputComponent* EnhancedInputComponent = Cast<UEnhancedInputComponent>(PlayerInputComponent);
if (EnhancedInputComponent)
{
EnhancedInputComponent->BindAction(JumpAction, ETriggerEvent::Triggered, this, &AMyCharacter::Jump);
}
}
Responding to Input Events
Handling input events requires binding actions to functions in your class. Here’s an example of responding to a jump input action:
void AMyCharacter::Jump()
{
if (!bIsJumping)
{
Jump();
bIsJumping = true;
}
}
Complex Input Handling
Combining Input Actions
You can combine multiple input actions using Composite Actions, making your characters respond to a sequence of inputs.
For example, implementing a combined sprint and jump ability can be configured as follows:
EnhancedInputComponent->BindAction(SprintAction, ETriggerEvent::Triggered, this, &AMyCharacter::StartSprinting);
EnhancedInputComponent->BindAction(JumpAction, ETriggerEvent::Triggered, this, &AMyCharacter::Jump);
Handling Input Triggers and Conditions
Triggers and conditions can control how and when actions are executed. For instance, if you want a player to jump only while sprinting, this can be enforced with conditions:
void AMyCharacter::Jump()
{
if (bIsSprinting)
{
Super::Jump();
}
}
This logic ensures that the jump action only activates during a sprint.
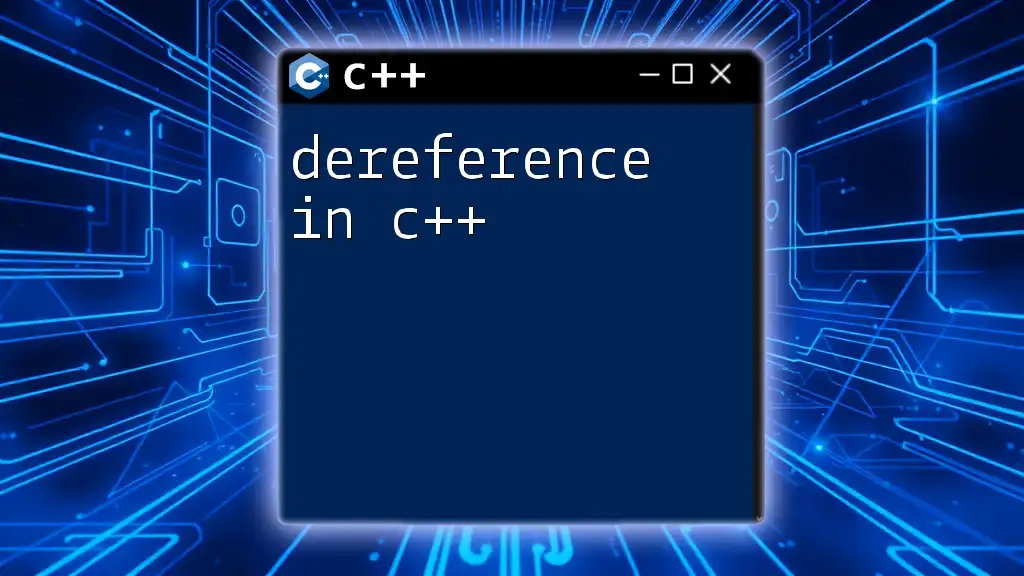
Best Practices for Enhanced Input Management
Structuring Your Input Code
For clean, maintainable code, it’s essential to structure your input handling well. Use separate classes or components for defining input actions and mappings, thereby decoupling input management from game logic.
This not only improves readability but also makes it simpler to modify input settings without needing to change core gameplay mechanics.
Debugging Input Issues
When you encounter issues with input, leverage Unreal Engine’s debugging tools. Common problems include conflicting key bindings or improperly set actions. The Enhanced Input system provides detailed state information, allowing you to diagnose problems more effectively.
Use the following strategies for effective debugging:
- Enable input debugging in the editor to visualize input actions.
- Test each input action in isolation to ensure it works as expected.
- Utilize logging to track input events during gameplay.
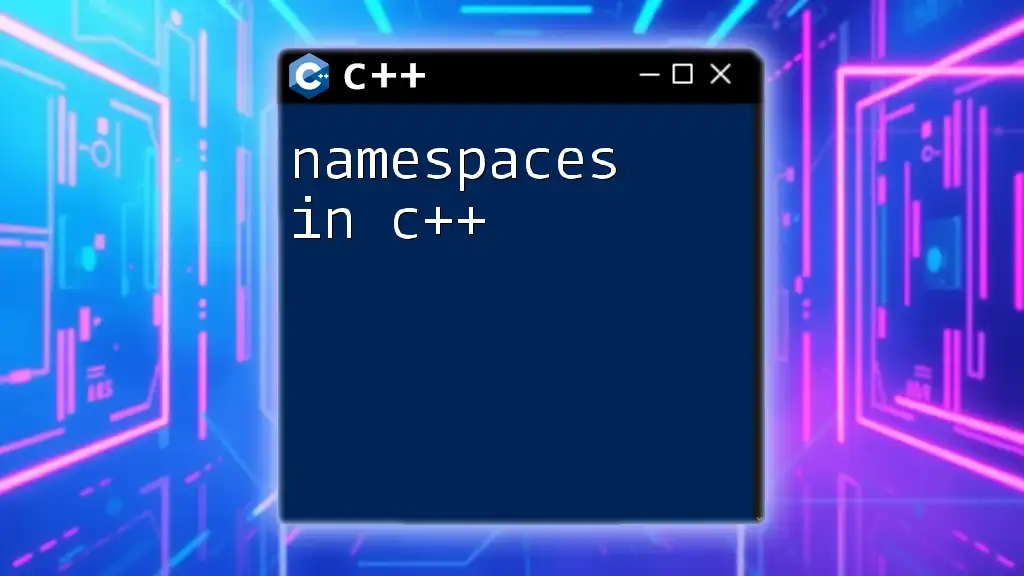
Advanced Topics in Enhanced Input
Customizing Input Debugging
To improve input debugging, you can enable additional input state diagnostics. To do this, ensure that you've set the input component’s debugging property:
EnhancedInputComponent->bDebugInput = true;
This will allow you to see live input statuses in the UE5 console.
Creating Custom Input Behaviors
For unique gameplay experiences, creating bespoke input behaviors can greatly enhance how players interact with your game. This might include multi-step input sequences or special configurations for abilities.
For example, implementing a toggleable input mode can be achieved through a simple check:
void AMyCharacter::ToggleAbilityInput()
{
bAbilityInputEnabled = !bAbilityInputEnabled;
}
This would allow players to switch between different control schemes dynamically, enriching gameplay options.
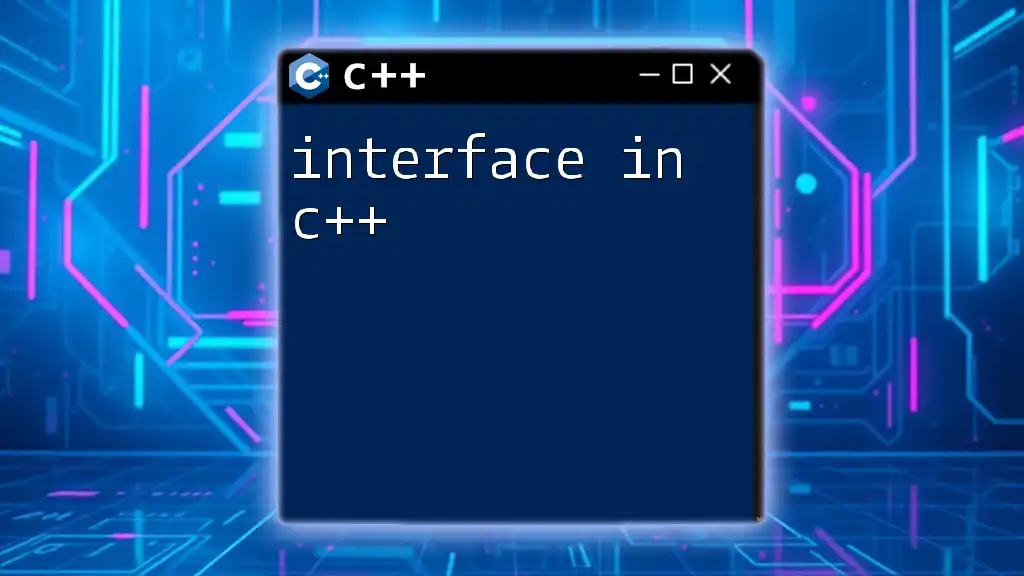
Conclusion
In this comprehensive guide on UE5 Enhanced Input C++, we explored the intricacies of effective input management in Unreal Engine. With the Enhanced Input system, you can create more responsive and adaptable game controls, enhancing the overall player experience. By utilizing the structures and practices outlined, you can provide both your players and your development team with a seamless interface for interactions—one that adjusts effortlessly to fit gameplay scenarios.
Additional Resources
For more insights and detailed information, refer to the official Unreal Engine documentation on Enhanced Input. Engage with community forums, tutorials, and discussions to keep abreast of best practices and innovations in game input management.