The Stanford C++ Library is a collection of useful C++ classes and functions designed to simplify programming tasks for students and educators at Stanford University.
Here’s an example of using the Stanford C++ Library to create and manipulate a simple string:
#include "simplecpp"
int main() {
// Create a simple string
string greeting = "Hello, World!";
cout << greeting << endl; // Output the greeting
return 0;
}
What is the Stanford C++ Library?
The Stanford C++ Library is a collection of functionalities and resources designed specifically for learning and implementing C++ programming concepts. It enhances standard C++ by providing additional classes and functions that ease the learning curve and facilitate more efficient programming practices. This library is particularly popular among students and educators as it simplifies the process of writing C++ code, making it more approachable for beginners.
The main features of the Stanford C++ Library include a variety of data structures such as `Vector`, `Map`, and `Set`, as well as utilities for handling input and output efficiently. Unlike standard libraries, which can be overwhelming due to their complexity, the Stanford C++ Library focuses on teaching core programming principles while providing essential features.
The Target Audience
The Stanford C++ Library is tailored for a diverse audience, primarily:
- Students: Those learning the fundamentals of programming can benefit immensely from its simplified syntax and structure.
- Educators: Instructors can use the library as a tool to teach C++ concepts more effectively in classrooms.
- Early Career Developers: Individuals looking to deepen their understanding of C++ will find it a valuable resource.
Additionally, the library can be applied in various fields including software development, game programming, and scientific computing.
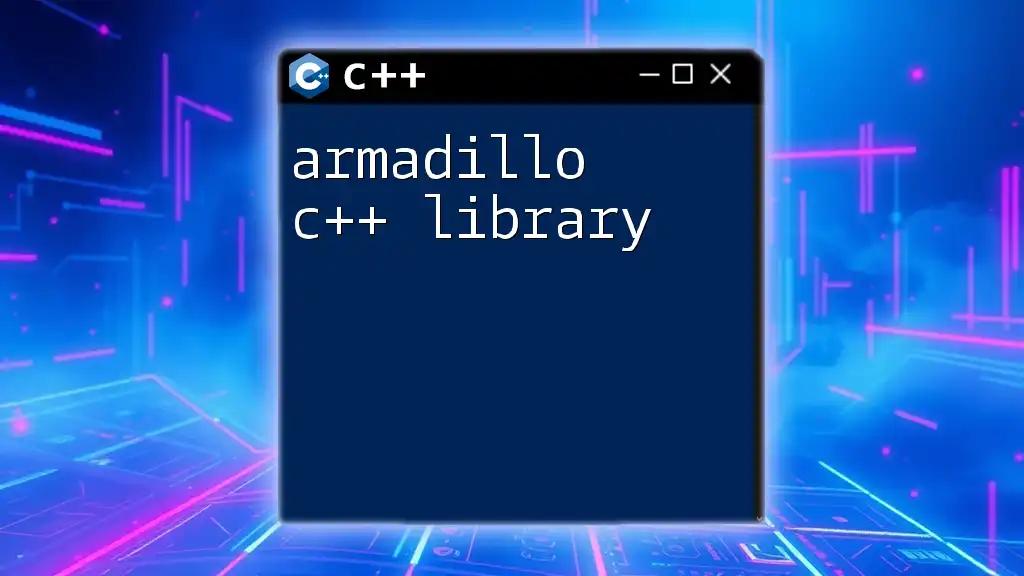
Getting Started with the Stanford C++ Library
Installation Process
Installing the Stanford C++ Library is straightforward, allowing users to quickly access its features. Here’s a detailed guide to get started:
System Requirements
Before installation, ensure that your system meets the following requirements:
- Operating Systems: Windows, macOS, or Linux.
- Development Tools: A compatible C++ compiler (such as GCC or Visual Studio).
Step-by-Step Installation Guide
- Downloading the Library: Visit the official Stanford C++ Library website to download the latest version of the library files.
- Configuring the Environment: Follow the installation guide relevant to your operating system. This typically involves setting environment variables and including library paths in your IDE.
- Verification of Installation: To confirm that the library is installed correctly, run the following test code snippet:
#include "vector.h" #include <iostream> using namespace std; int main() { Vector<int> testVector; testVector.add(1); testVector.add(2); cout << testVector; // Should output: 1, 2 return 0; }
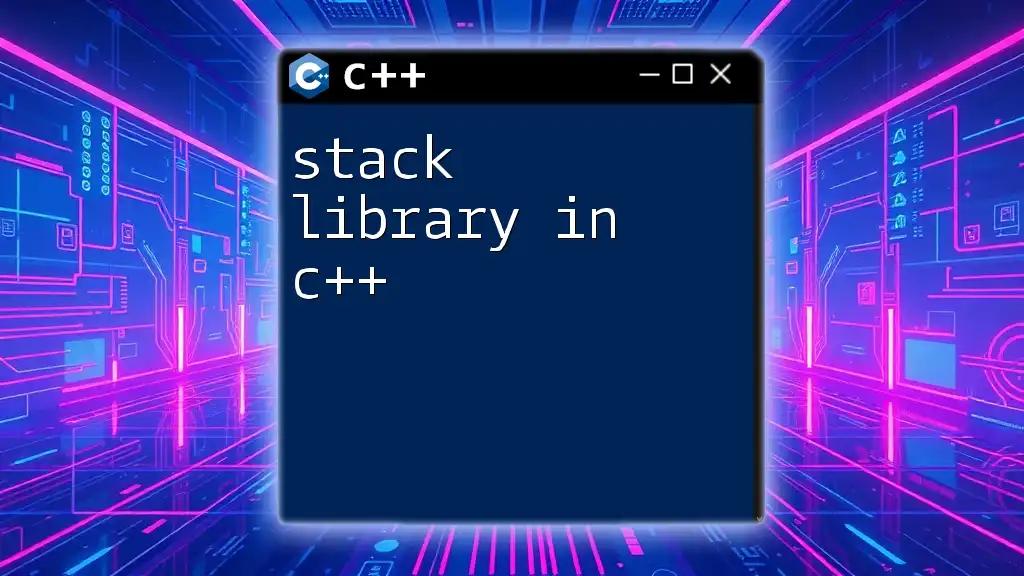
Core Components of the Stanford C++ Library
The Stanford C++ Library is rich in components that facilitate various programming tasks. Understanding these core components will empower users to write effective C++ code.
Basic Data Types and Structures
The library provides several data structures that are easy to use and essential for managing data:
- Vector: A dynamic array that can grow or shrink in size.
- Map: A collection of key-value pairs which allows for efficient data retrieval.
These data structures underpin many operations in programming, enabling users to manipulate data effortlessly.
Handling Input and Output
The Stanford C++ Library enhances input and output operations, providing utilities that simplify file handling.
For example, the following code snippet demonstrates how to read data from a file into a `Vector`:
#include "vector.h"
#include "filelib.h"
#include <iostream>
using namespace std;
int main() {
Vector<int> numbers;
ifstream infile("numbers.txt");
if (infile.is_open()) {
infile >> numbers; // Read numbers from a file
cout << numbers; // Output numbers to console
} else {
cout << "Error opening file." << endl;
}
infile.close();
return 0;
}
This example showcases how the library simplifies file handling, enabling users to focus on logic rather than syntax.
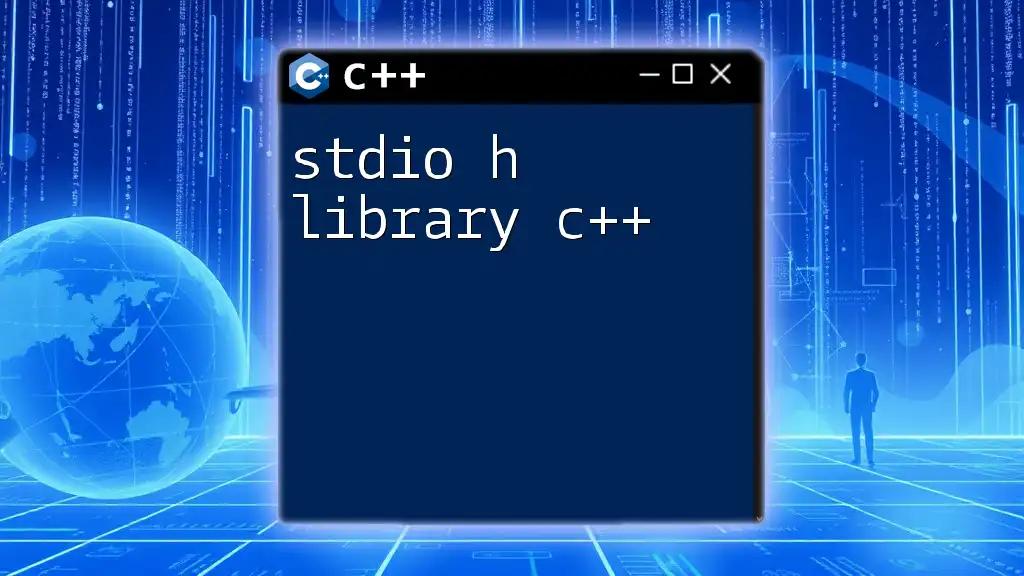
Key Features of the Stanford C++ Library
The robustness of the Stanford C++ Library lies in its key features that facilitate optimized programming.
Memory Management
Effective memory management is crucial in programming. The Stanford C++ Library provides smart pointers, such as `shared_ptr`, to help manage memory more effectively. Using smart pointers reduces the likelihood of memory leaks and dangling pointers, which are common pitfalls in C++ programming.
For instance, you can use `shared_ptr` as shown below:
#include "memory.h"
#include <iostream>
using namespace std;
void example() {
shared_ptr<int> sp = make_shared<int>(20);
cout << *sp << endl; // Outputs: 20
}
This code snippet illustrates how to handle dynamic memory seamlessly, making coding safer and more efficient.
Graphical User Interface (GUI) Components
For those interested in graphical programming, the Stanford C++ Library offers easy-to-use GUI components. Creating a simple graphical window can be accomplished with minimal code:
#include "simplegraphics.h"
#include <iostream>
int main() {
initGraphics("My Window", 500, 500);
drawCircle(250, 250, 50);
waitForClick();
closeGraphics();
return 0;
}
With just a few lines, users can create an interactive window and handle events, which is ideal for developing educational software and simple games.
Data Structures and Algorithms
The library includes built-in support for various data structures and common algorithms, allowing users to focus on solving problems rather than implementing complex functionalities from scratch.
For example, sorting a `Vector` can be done as follows:
#include "vector.h"
#include <algorithm>
#include <iostream>
void sortExample() {
Vector<int> v = {5, 3, 2, 8, 1};
sort(v.begin(), v.end());
cout << v; // Will output: 1, 2, 3, 5, 8
}
This capability reflects how the library’s features align with real-world programming needs, enhancing learning and application.
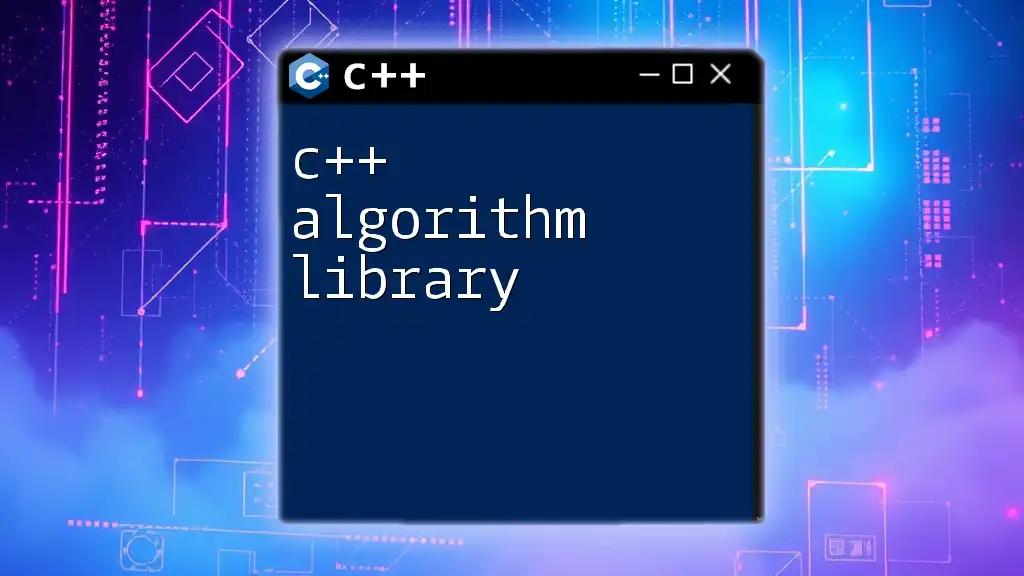
Practical Applications of the Stanford C++ Library
Educational Context
The Stanford C++ Library has become a cornerstone in many computer science curriculums. Its straightforward design allows educators to introduce concepts like data structures, algorithms, and software engineering principles efficiently. Courses leveraging this library report improved student engagement and understanding.
Industry Applications
In addition to educational settings, the Stanford C++ Library finds its place in the industry, particularly in fields such as software development, game design, and scientific research. By providing a solid foundation in C++, many developers launch their careers with a thorough grasp of programming principles.
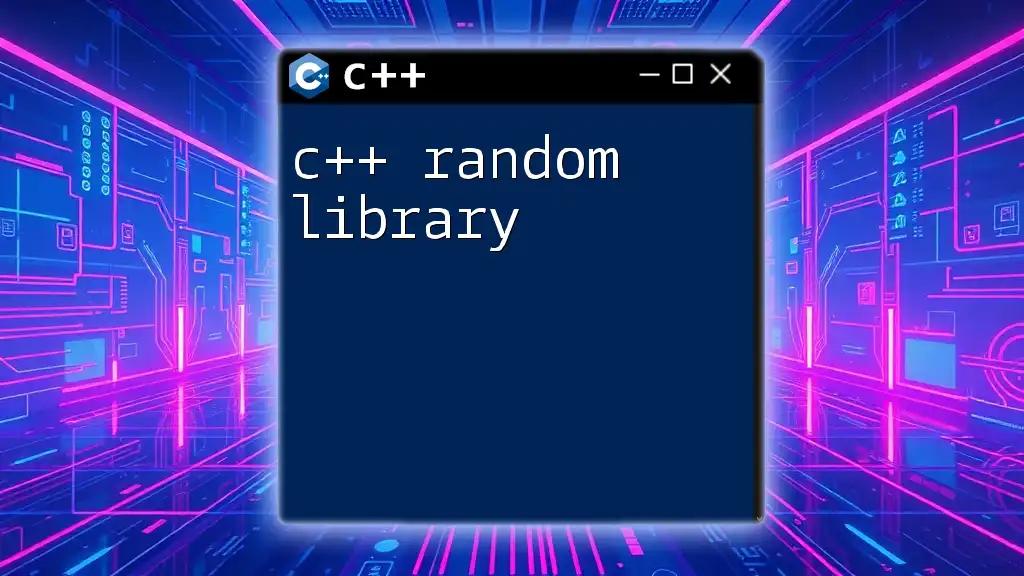
Tips and Best Practices
To maximize your experience with the Stanford C++ Library, consider the following tips and best practices:
- Common Pitfalls to Avoid: Beginners often make errors related to memory management. Be vigilant about initializing pointers and track ownership using smart pointers.
- Optimization Techniques: When coding, focus on using provided data structures and algorithms to avoid reinventing the wheel. Familiarize yourself with the library documentation for specific functionalities.
Documentation and Resources
The official documentation for the Stanford C++ Library is extensive and serves as the best resource for users looking to explore the library's full capabilities. Additionally, numerous online courses and tutorials can supplement your learning, providing hands-on experience.
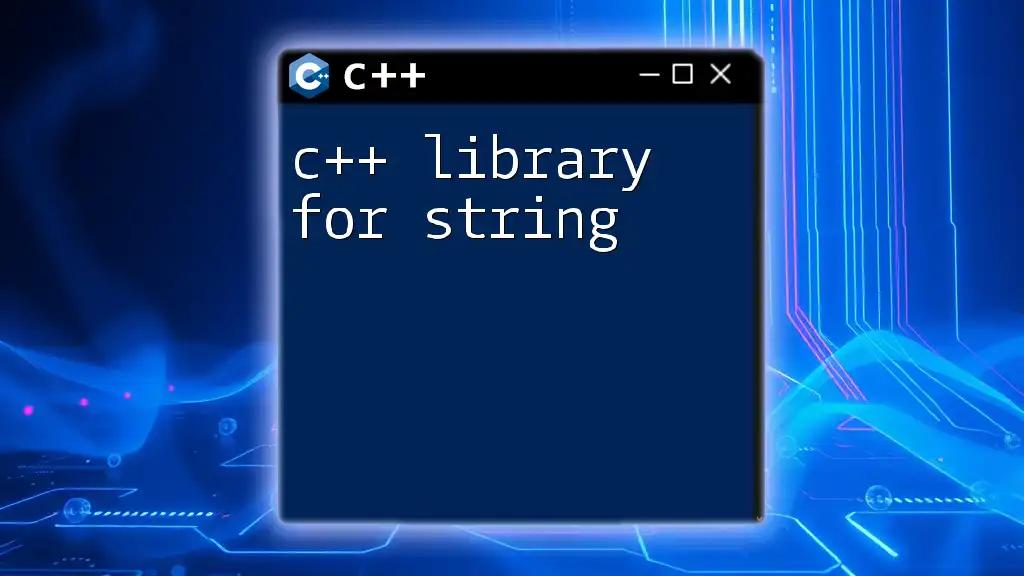
Conclusion
In summary, the Stanford C++ Library serves as an invaluable tool for students, educators, and novice developers alike. With its user-friendly design, comprehensive functionalities, and practical applications, it stands out as a premier resource for anyone engaged in learning or utilizing C++. Whether you are coding a simple program or embarking on more complex projects, this library will enhance your programming journey. Embrace the power of the Stanford C++ Library and elevate your coding skills!
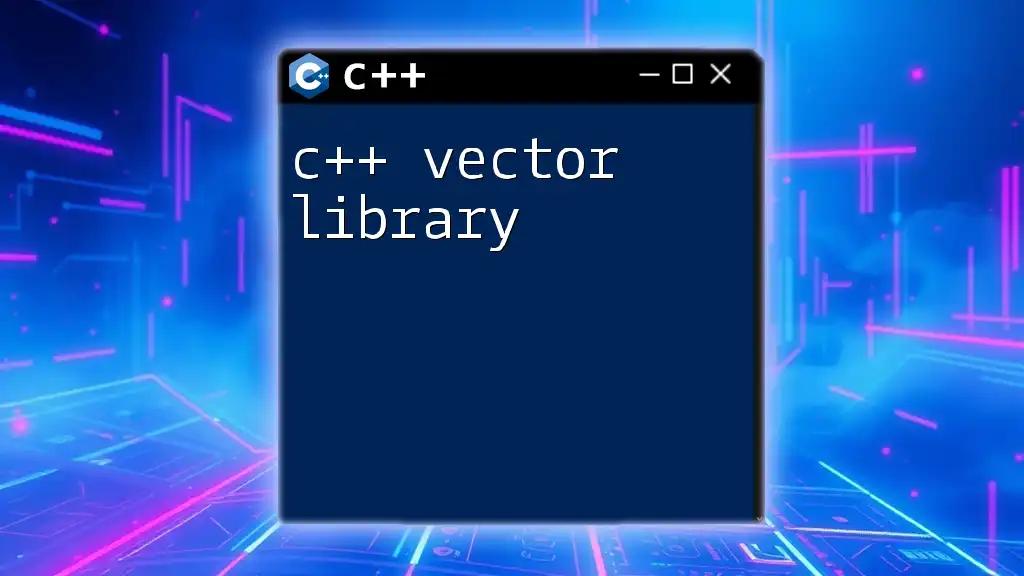
Further Reading
To deepen your understanding of the Stanford C++ Library and C++ programming in general, look into the following resources:
- Books: Find recommended titles focused on C++ programming and the university's specific curriculum.
- Online Resources: Access the official documentation and various online tutorials that provide a structured learning path.