C++ provides the `<string>` library, which allows for efficient manipulation of strings using the `std::string` class, creating a seamless way to handle character data.
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
Understanding String Data Types in C++
The C++ library for string manipulation begins with the `std::string` class, which offers a powerful and flexible way to work with textual data. Understanding this class and how it differs from C-style strings is crucial for efficient programming in C++.
The `std::string` Class
The `std::string` class encapsulates a sequence of characters, providing functionality for manipulating them easily.
Key Properties:
- Dynamic Size: Unlike character arrays, `std::string` automatically adjusts its size as needed.
- Memory Management: It manages memory on its own, reducing the risk of buffer overflows common in C-style string handling.
Comparison with C-style strings
C-style strings are essentially arrays of characters ending with a null character (`'\0'`). Their usage can lead to pitfalls, including:
- Manual memory management
- Risk of buffer overflows
- Complexity in string manipulation
Advantages of `std::string`:
- Automated memory management
- Extensive built-in functions for easier string manipulation
- Type safety, reducing bugs related to string handling
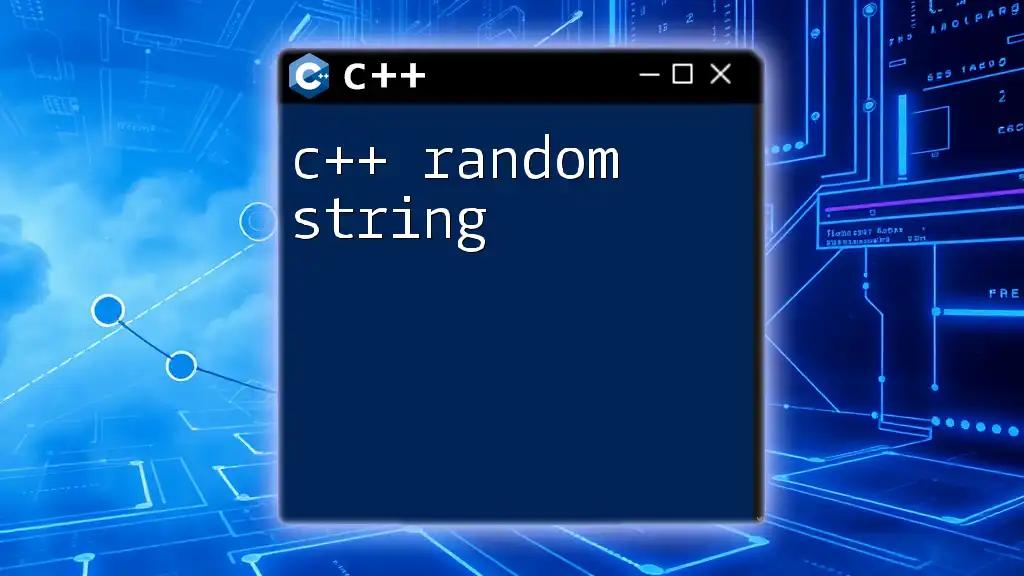
C++ Standard Library: The String Class
The C++ standard library provides the `<string>` header, which contains the `std::string` class. This inclusion in the standard library makes it widely supported and easy to use across different compilers and platforms.
Key Features of the `std::string` Class
The `std::string` class comes with several key features, such as:
- Mutable and Immutable strings: While `std::string` itself is mutable, string literals are immutable.
- String Literals and Initialization: You can initialize a string using quotes, for example, `std::string str = "Hello, World!";`.
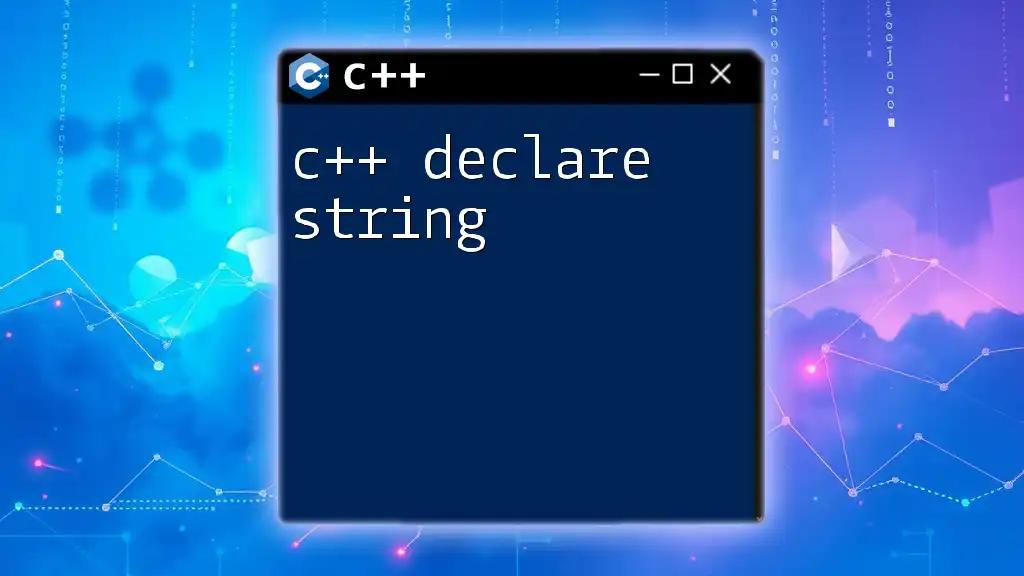
Common Operations with the C++ String Library
Creating and Initializing Strings
Creating a string in C++ is straightforward. Here are a few common methods of initialization:
std::string defaultStr; // Default (empty) string
std::string literalStr = "Hello"; // String literal
std::string charArrayStr = {'H', 'e', 'l', 'l', 'o', '\0'}; // From character array
Modifying Strings
Once you have initialized your strings, modifying them is the next step.
Appending Strings
You can append to a string using the `+=` operator or the `append()` method:
std::string str = "Hello";
str += " World"; // Output: Hello World
str.append("!"); // Output: Hello World!
Inserting and Erasing Characters
You can insert or erase characters at specific positions using `insert()` and `erase()`:
std::string str = "Hello World";
str.insert(5, ", Everyone"); // Inserting after "Hello"
str.erase(5, 10); // Output: Hello!
Replacing Substrings
Using `replace()`, you can replace parts of a string with new text:
std::string str = "Hello World";
str.replace(6, 5, "C++"); // Output: Hello C++!
Accessing String Elements
You can access individual characters using the `operator[]` or the `at()` method:
std::string str = "Hello";
char firstChar = str[0]; // 'H'
char secondChar = str.at(1); // 'e'
String Size and Capacity
Understanding string size is important for effective memory usage.
Use `size()`, `length()`, and `capacity()` to manage string sizes:
std::string str = "Hello";
size_t len = str.size(); // Length of string
size_t cap = str.capacity(); // Allocated memory space
Adjust the size dynamically with `resize()`:
str.resize(10); // Resize string to 10 characters
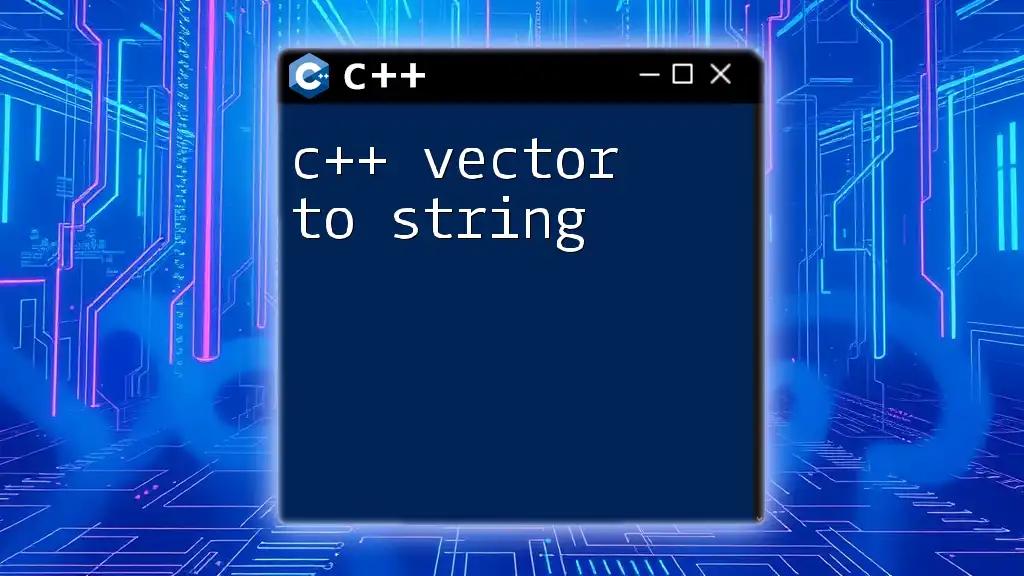
String Manipulation Functions
Conversion Functions
String conversion functions allow you to seamlessly interchange between strings and numbers:
std::string numberStr = "42";
int number = std::stoi(numberStr); // String to int
std::string convertedStr = std::to_string(number); // int to string
Searching and Finding Substrings
Utilize `find()` and `rfind()` to locate substrings:
std::string str = "Hello World";
size_t position = str.find("World"); // Returns position of "World"
String Comparison
With `compare()`, you can verify if two strings are the same:
std::string str1 = "Hello";
std::string str2 = "Hello";
if (str1.compare(str2) == 0) {
// Strings are equal
}
String Stream: A Powerful Tool
The `std::stringstream` class lets you handle string input and output seamlessly.
#include <sstream>
std::stringstream ss;
ss << "Score: " << 100;
std::string result = ss.str(); // "Score: 100"

Advanced Features of the C++ String Library
String Algorithms
The `<algorithm>` header offers several useful algorithms for string manipulation, enhancing functionality.
Common functions include `std::find`, `std::count`, and `std::replace` for efficient string processing.
Regular Expressions
The `<regex>` library facilitates advanced string operations, such as pattern matching:
#include <regex>
std::regex pattern("Hello");
std::string text = "Hello World";
bool found = std::regex_search(text, pattern); // Returns true

Performance Considerations
When using the C++ library for string, consider performance implications.
`std::string` vs. C-style strings:
- Use `std::string` for dynamic and safer handling.
- Opt for C-style strings in performance-critical applications or when interfacing with C libraries.
Tips for Optimization
Utilize string reserves (`reserve()`) to minimize memory reallocations when dealing with large strings or concatenations.
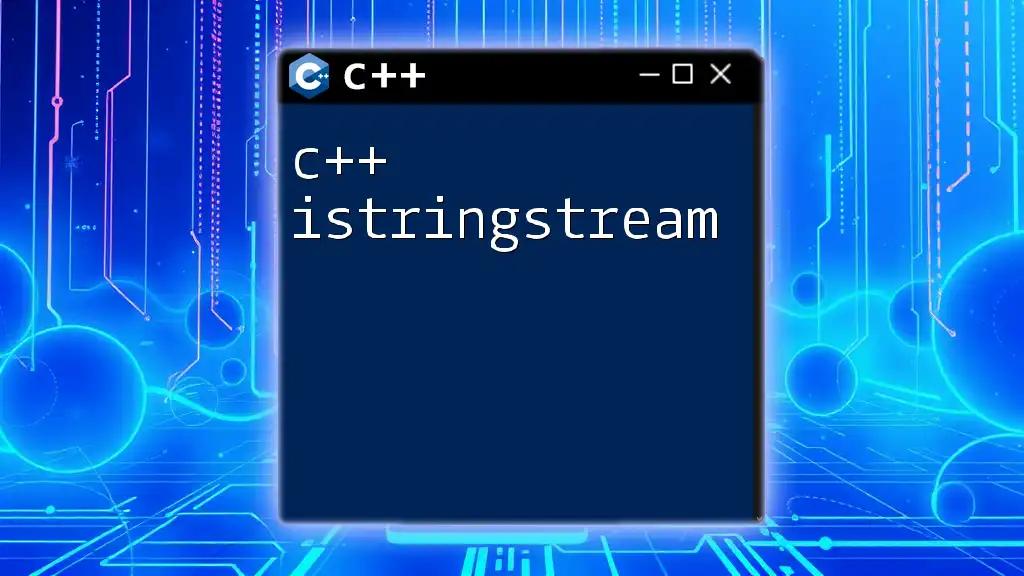
Conclusion
Mastering the C++ library for string manipulation opens doors to efficient and effective programming within the C++ ecosystem. Understanding and practicing the features outlined in this guide not only enhances your coding skills but also prepares you for real-world applications where string handling is crucial. To deepen your knowledge, explore community resources, documentation, and tutorials available online.