C++ memory ordering refers to the rules that determine how memory operations (reads and writes) are seen by different threads in concurrent programming, allowing developers to manage synchronization and prevent data races effectively.
Here’s a simple code snippet illustrating the use of memory ordering with atomic operations:
#include <atomic>
#include <iostream>
#include <thread>
std::atomic<int> counter(0);
void increment() {
for (int i = 0; i < 1000; ++i) {
counter.fetch_add(1, std::memory_order_relaxed);
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Counter: " << counter.load(std::memory_order_relaxed) << std::endl;
return 0;
}
Understanding Concurrency in C++
Defining Concurrency
Concurrency refers to the ability for multiple threads or processes to execute sequences of operations independently. In modern applications, leveraging concurrency can significantly improve performance, particularly for tasks that are computationally intensive or I/O bound. However, this also introduces challenges, particularly in terms of managing shared resources.
The Role of Memory in Concurrency
In concurrent programming, multiple threads often access shared data. This shared nature can lead to issues such as data races, where two or more threads read and write shared data simultaneously without proper synchronization, potentially leading to inconsistent or unpredictable states. Understanding C++ memory ordering is critical to successfully managing these shared resources.
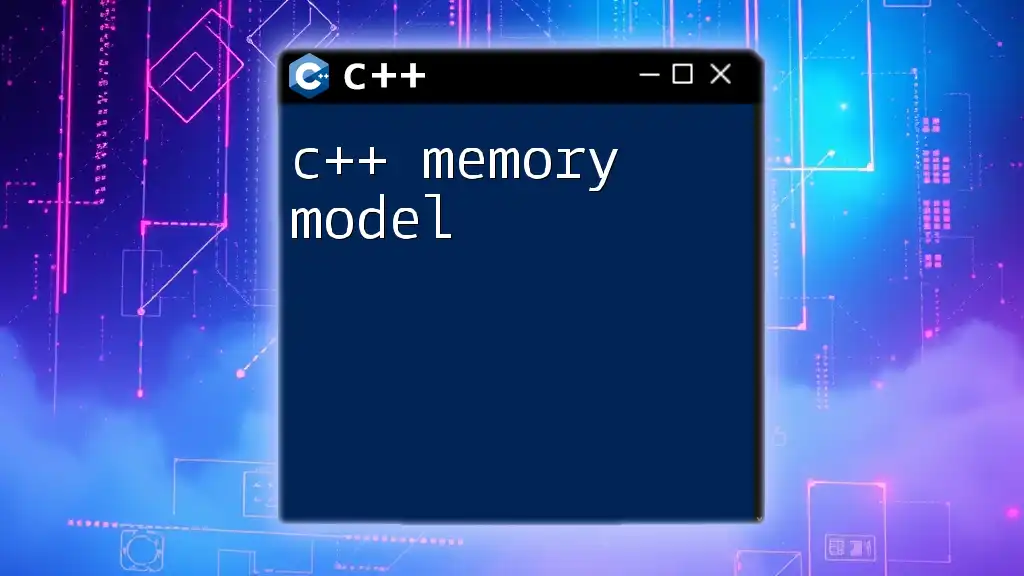
The C++ Memory Model
Overview of the C++ Memory Model
Introduced in C++11, the C++ memory model provides a set of guarantees about how operations on data can be perceived by different threads. This is essential for defining safe parallel programming practices. The new memory model ensures that operations perform consistently across different executions, adapting to the complexities of multi-core processors.
Key Concepts of the C++ Memory Model
-
Thread Safety refers to how code behaves when accessed concurrently by multiple threads without leading to data races or inconsistencies. To ensure thread safety, developers must use appropriate synchronization mechanisms, including memory orders.
-
Happens-before relationship establishes a sequence of operations where one operation is guaranteed to be visible to another. Understanding this relationship is key to reasoning about the correctness of concurrent programs.
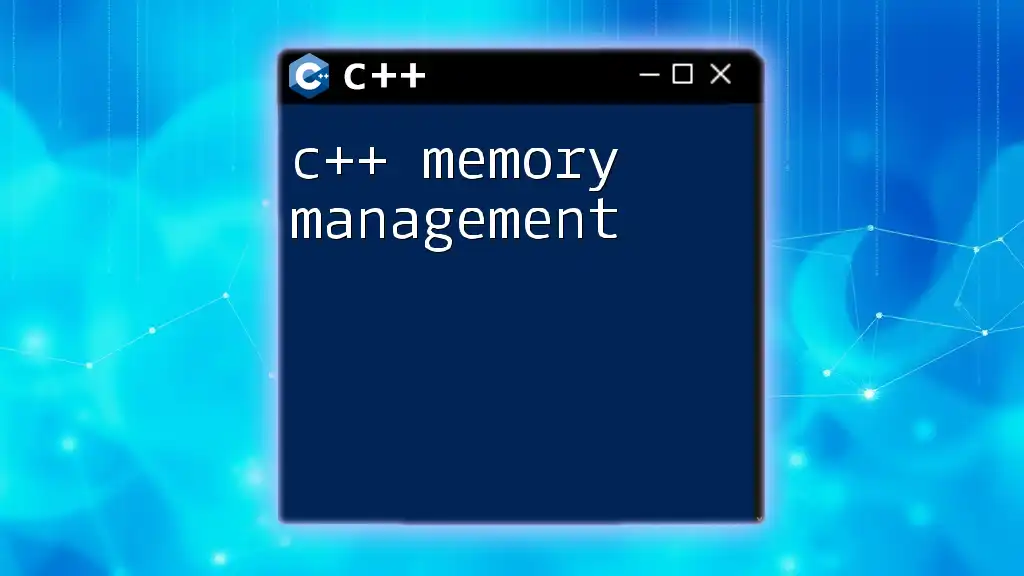
Memory Order in C++
What is Memory Order?
In C++, the term memory order refers to how operations on shared variables are perceived in a multithreaded environment. It affects the ordering of atomic operations, influencing both predictability and performance. Making the correct choice in memory order can improve performance while ensuring safety and correctness.
Types of Memory Orders in C++
-
Relaxed Memory Order (`std::memory_order_relaxed`): This allows maximal performance with minimal guarantees about visibility between threads. It is suitable when atomic operations do not depend on the happens-before relationship. For example:
std::atomic<int> counter(0); void increment() { counter.fetch_add(1, std::memory_order_relaxed); }
-
Acquire Memory Order (`std::memory_order_acquire`): This guarantees that all preceding reads and writes in the current thread are visible after an atomic load. It establishes a happens-before relationship. For example:
int data; std::atomic_flag flag = ATOMIC_FLAG_INIT; void producer() { data = 42; flag.clear(std::memory_order_release); // Release } void consumer() { while (flag.test_and_set(std::memory_order_acquire)) {} // Acquire std::cout << data; // Safe to read data now }
-
Release Memory Order (`std::memory_order_release`): This provides visibility guarantees for writes before the release point. It ensures that all previous writes are visible to threads that acquire the same atomic object. An example involves producer-consumer scenarios where flags indicate data readiness.
-
Acquire-Release Memory Order: Often used together; for instance, in a scenario where one thread updates a flag and another thread waits on this flag before reading the updated data, as shown above.
-
Sequentially Consistent Memory Order (`std::memory_order_seq_cst`): This is the strictest type of memory order, providing a total ordering of all sequentially consistent operations across all threads. It simplifies reasoning about the program but may hinder performance:
std::atomic<int> value(0); void write() { value.store(1, std::memory_order_seq_cst); } void read() { int temp = value.load(std::memory_order_seq_cst); std::cout << temp; }
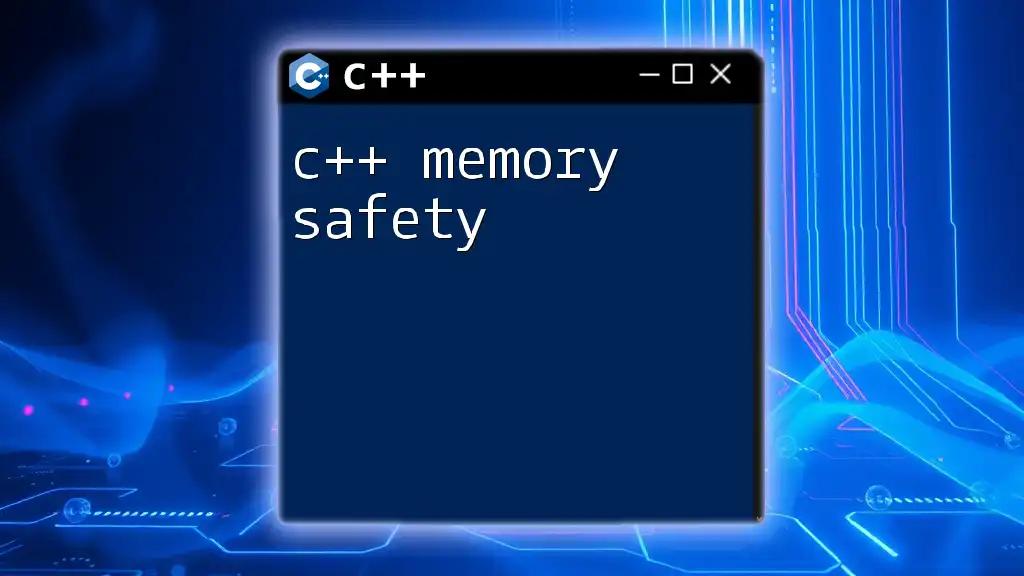
How to Use Memory Order Effectively
Choosing the Right Memory Order
It is essential to understand application requirements when selecting a memory order. Use relaxed when performance is critical and ordering is not a concern. Use acquire and release for synchronization and sequentially consistent when correctness is paramount. Always weigh the trade-offs between performance and safety.
Common Pitfalls and How to Avoid Them
Developers often make the mistake of using sequentially consistent memory order out of habit, which can lead to performance bottlenecks. Failing to understand the implications of acquire-release pairs can also lead to subtle bugs. To avoid these pitfalls, always analyze the specific needs of your operations and consider starting with relaxed when safe.
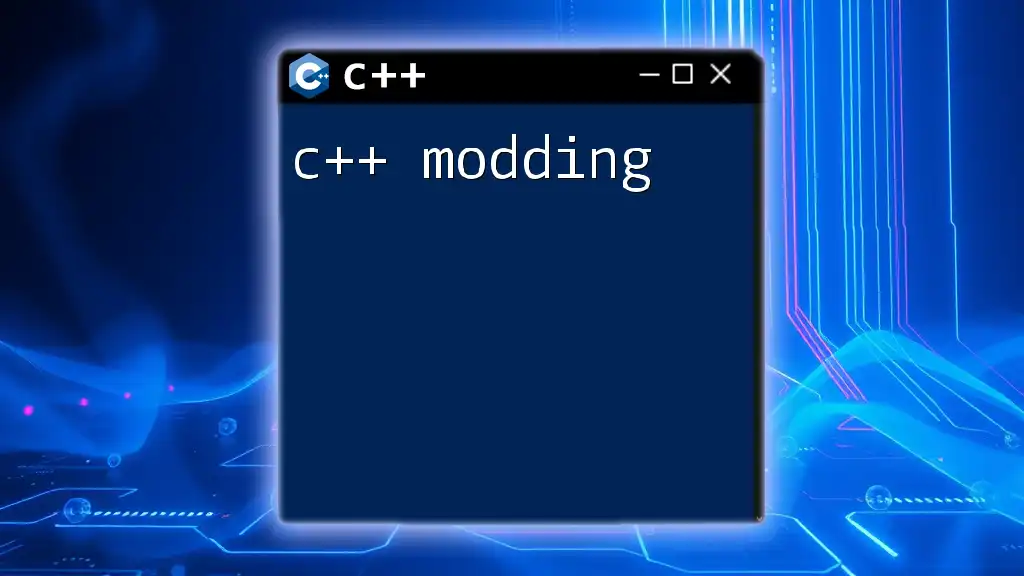
Practical Examples and Best Practices
Implementation of C++ Memory Ordering
Consider a simple producer-consumer scenario involving two threads: one producing data and another consuming it:
std::atomic<int> data(-1);
std::atomic<bool> ready(false);
void producer() {
data.store(42, std::memory_order_relaxed);
ready.store(true, std::memory_order_release);
}
void consumer() {
while (!ready.load(std::memory_order_acquire)); // Wait until data is ready
std::cout << data.load(std::memory_order_relaxed) << std::endl; // Read data
}
This example shows how C++ memory ordering can be effectively used to coordinate access to shared data without causing data races.
Performance Considerations
Memory ordering affects not just correctness but also performance. Relaxed memory order can yield higher throughput in certain applications due to reduced overhead. However, the right choice should always be based on an understanding of both the shared data's usage and how threads interact with it.
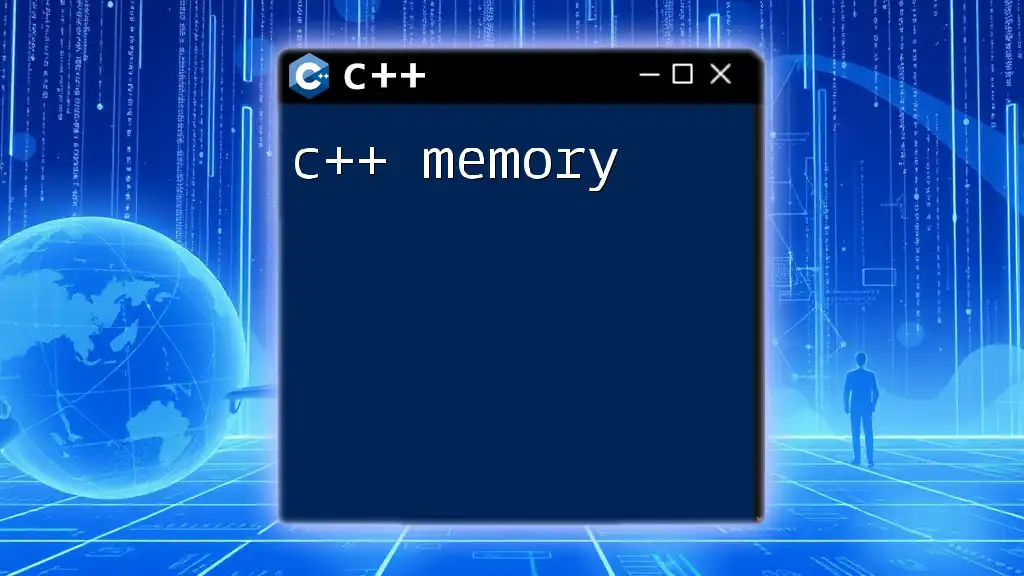
Debugging Memory Order Issues
Tools for Debugging
Several tools can assist in debugging memory order-related issues. These include thread sanitizers and static analysis tools that can identify potential data races or improper synchronization patterns. Examples of such tools include:
- ThreadSanitizer: Detects data races in C++ applications.
- Valgrind: Provides additional insights into heap memory issues.
Code Analysis Techniques
One efficient technique for analyzing memory order is to conduct regular code reviews, focusing on the interactions between threads. Employing static analysis tools during development can help catch potential problems early on.
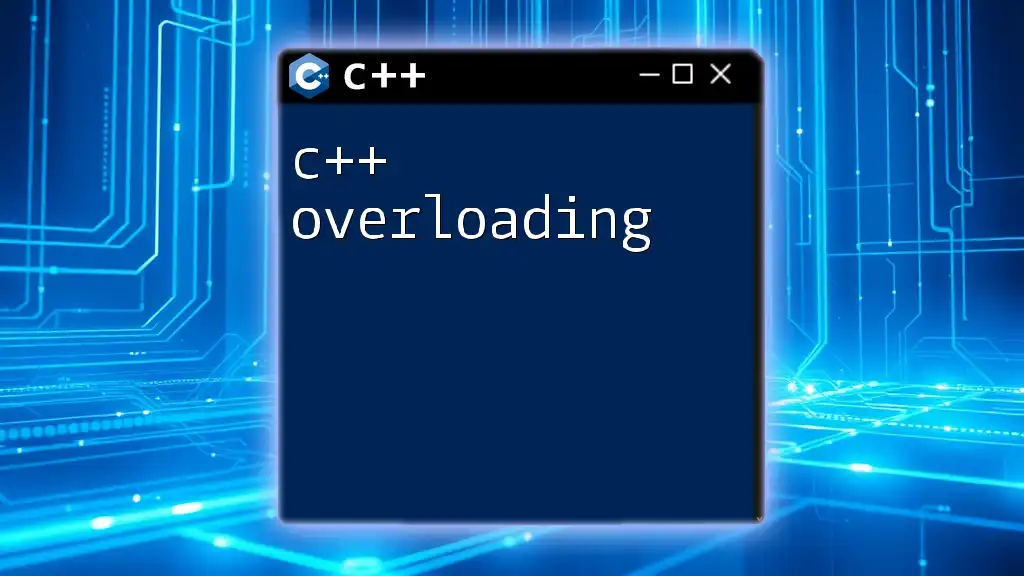
Conclusion
Understanding c++ memory ordering is vital for developing safe and efficient concurrent applications. By grasping the concepts of memory models, types of memory orders, and their practical applications, developers can effectively manage shared data and ensure the robustness of their multi-threaded applications. The journey toward mastering concurrency in C++ is ongoing, and keeping abreast of new developments in the language will inspire further growth and understanding in this critical area.
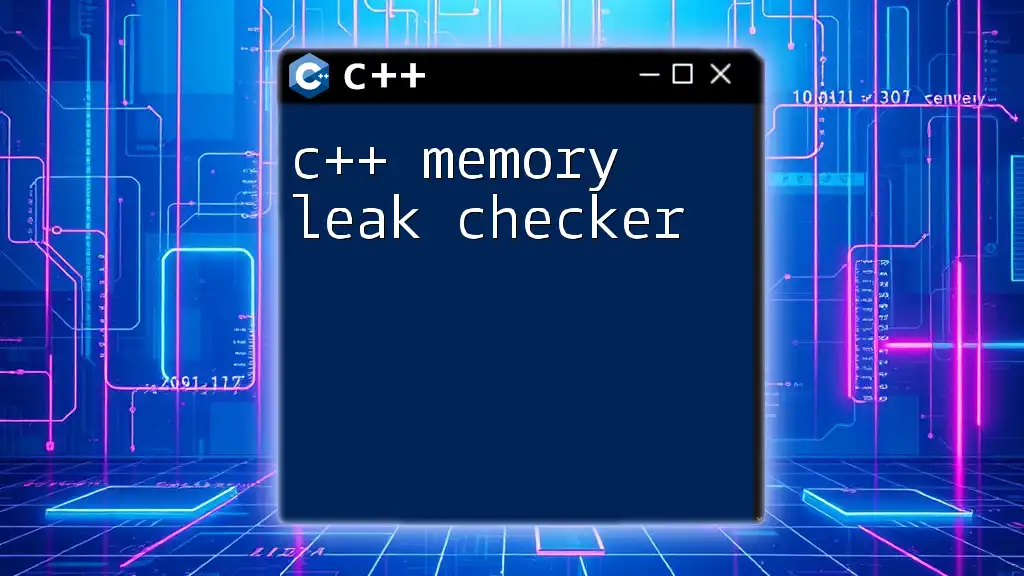
Additional Resources
Recommended readings and references include comprehensive texts on C++ concurrency and the specificities of memory order. Joining communities and forums can provide ongoing support and knowledge-sharing opportunities to enhance your understanding of these advanced concepts.