C++ memory safety refers to the practices and techniques used to prevent common memory-related errors, such as buffer overflows and memory leaks, ensuring that a program correctly manages memory allocations and deallocations.
Here's a simple example demonstrating memory safety with smart pointers:
#include <iostream>
#include <memory>
void safeMemoryUsage() {
std::unique_ptr<int> ptr = std::make_unique<int>(42); // Automatically handles memory cleanup
std::cout << "The value is: " << *ptr << std::endl;
} // Memory is automatically freed when ptr goes out of scope
Understanding Memory Safety in C++
What is Memory Safety?
Memory safety refers to the prevention of programming errors that can lead to unpredictable behavior in a program. In the context of C++, memory safety is crucial as improper memory management can lead to issues such as buffer overflows, dangling pointers, and memory leaks. These issues not only compromise the integrity of applications but can also lead to security vulnerabilities in software.
Why Memory Safety Matters
The impact of memory-related bugs extends beyond simple crashes; they can cause data corruption, software failures, and security breaches. Many well-documented software failures can be traced back to memory safety issues. Examples include:
- Heartbleed: A buffer overflow vulnerability in OpenSSL that exposed sensitive information.
- Yahoo data breach: Exploits due to inadequate memory management allowed attackers to steal user data.
In C++, ensuring memory safety isn't just about writing code; it's about writing robust and secure applications that can withstand unexpected scenarios.
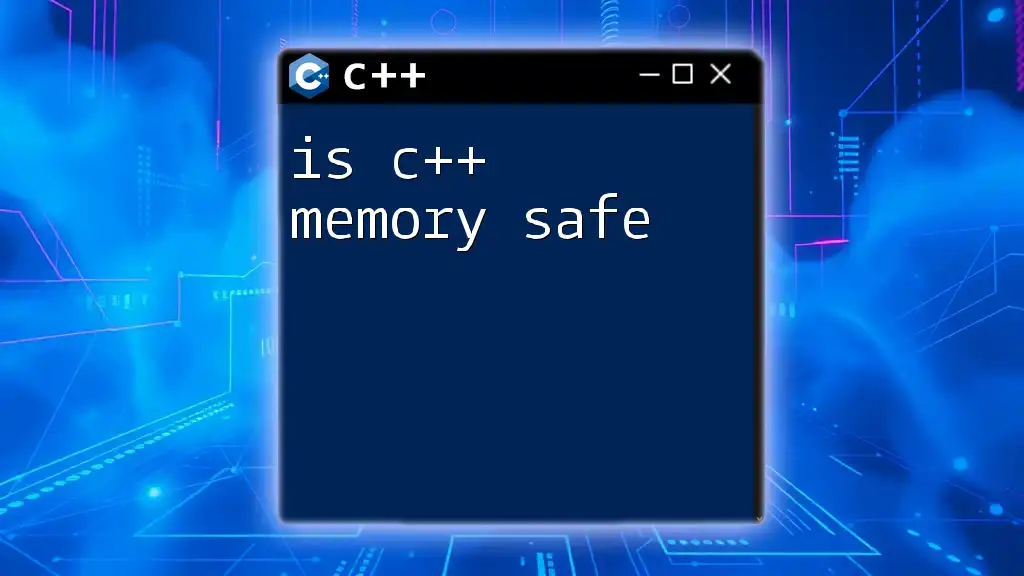
Key Concepts in Memory Management
The C++ Memory Model
C++ employs a unique memory model that divides memory into static (stack) and dynamic (heap) allocations.
- Stack Memory: This is where local variables are stored. It is automatically managed; when variables go out of scope, memory is reclaimed automatically.
- Heap Memory: This part of memory is used when we need dynamic memory allocation. Programs explicitly request memory from the heap, and failure to free this memory can lead to leaks.
Pointers and References
Pointers and references are fundamental components of C++ memory management but come with their own pitfalls.
Pointers can point to any memory address and can be manipulated directly. This flexibility can be dangerous:
int* p = nullptr; // Declare a pointer
*p = 10; // Dereferencing a null pointer leads to undefined behavior
In contrast, references must be initialized and cannot be null, making them safer to use. However, it is still possible to fall into the trap of dangling references if the underlying object goes out of scope.
Dynamic Memory Management
Dynamic memory is allocated using the `new` operator and should be freed using `delete`.
However, forgetting to free memory can lead to leaks:
int* arr = new int[10];
// ...
delete[] arr; // Important to prevent memory leaks
Understanding this necessity is essential for managing memory safely.
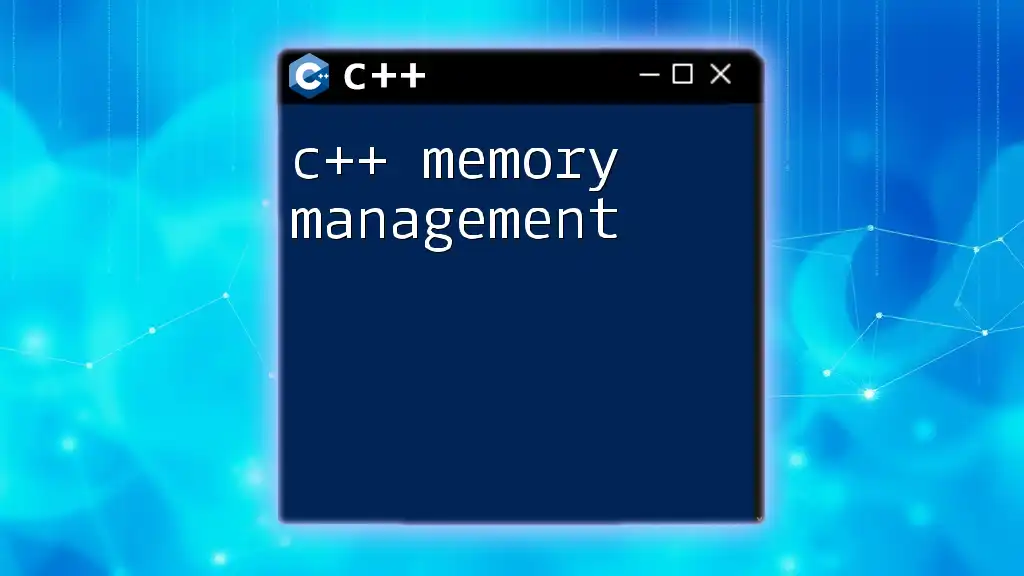
Common Memory Safety Issues in C++
Buffer Overflows
A buffer overflow occurs when data is written beyond the boundaries of allocated memory. This can corrupt data and lead to unpredictable behavior.
Consider the following example:
char buffer[10];
strcpy(buffer, "This string is too long"); // Buffer overflow
To prevent buffer overflows, always prefer safer functions that check bounds, such as `strncpy` or `snprintf`.
Use After Free
A dangling pointer occurs if an application accesses memory after it has been deallocated. Here’s an illustration:
int* ptr = new int(42);
delete ptr;
// ptr is now dangling; accessing it leads to undefined behavior
To prevent such issues, always set pointers to `nullptr` after deletion:
ptr = nullptr; // Safe cleanup
Memory Leaks
Memory leaks happen when allocated memory is not freed. They lead to a gradual increase in memory usage and can severely affect system performance:
void leakMemory() {
int* leakedPtr = new int(42);
// No delete statement means leakedPtr is never freed
}
Tools like Valgrind can help identify leaks in an application, giving developers the visibility needed to write more memory-safe code.
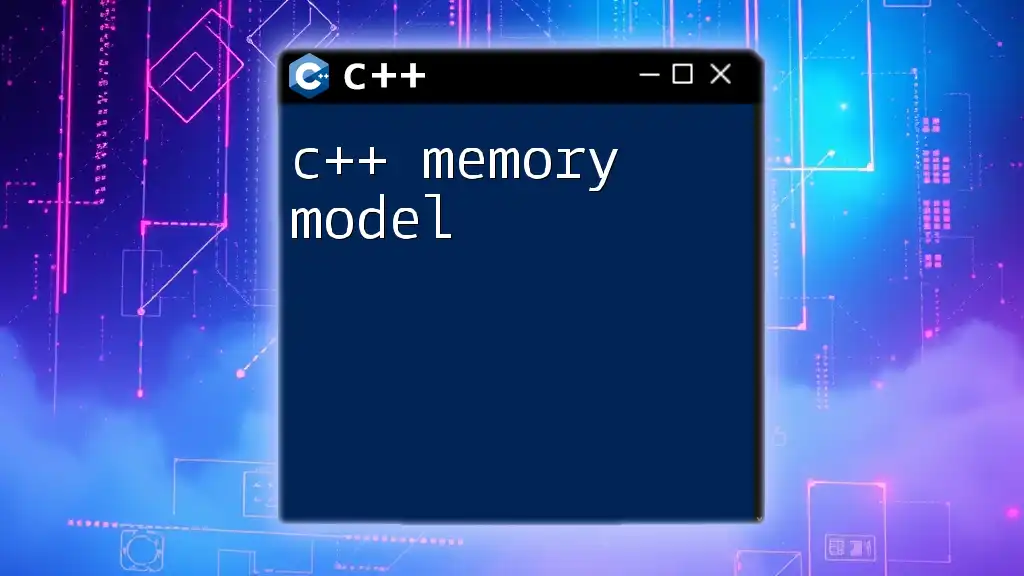
Best Practices for Memory Safety in C++
Smart Pointers
Smart pointers, introduced in C++11, provide an excellent mechanism for automatic memory management. They help avoid common pitfalls associated with raw pointers.
-
`std::unique_ptr` manages memory such that there’s only one owner at a time, ensuring automatic cleanup:
std::unique_ptr<int> smartPtr = std::make_unique<int>(42);
-
`std::shared_ptr` allows multiple owners of a memory block and automatically cleans up when the last owner goes out of scope.
Using smart pointers effectively minimizes memory leaks and dangling pointers, enhancing overall memory safety.
RAII (Resource Acquisition Is Initialization)
The RAII principle states that resources should be tied to object lifetimes. For instance, if you allocate memory in a constructor, free it in the destructor. This way, the memory management is handled automatically as an object goes out of scope.
Example:
class Resource {
public:
Resource() { ptr = new int(42); }
~Resource() { delete ptr; }
private:
int* ptr;
};
This approach minimizes error-prone manual memory management, making your C++ code safer and cleaner.
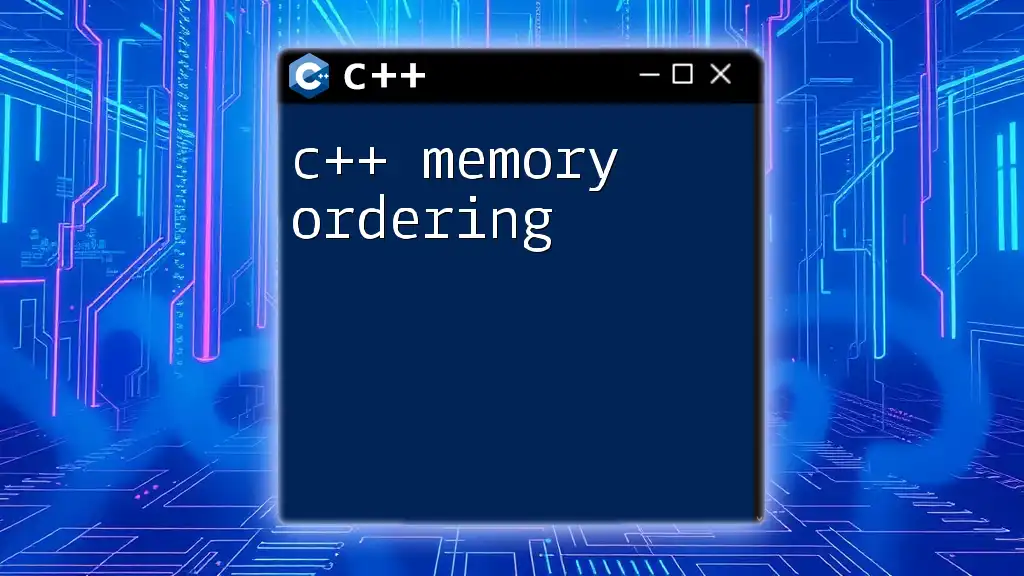
Advanced Memory Safety Techniques
Static Analysis Tools
Static analysis tools such as Clang Static Analyzer or Coverity are effective at spotting potential memory issues during compilation. They analyze the code without executing it, thus identifying vulnerabilities proactively and helping developers maintain memory safety in their applications.
Address Sanitizer
The Address Sanitizer (ASan) is a powerful debugging tool that detects memory corruption bugs at runtime. By enabling ASan during compilation, developers can catch a variety of memory safety issues including buffer overflows and use-after-free errors quickly.
Example of using ASan:
To compile a C++ program with ASan:
g++ -fsanitize=address -g your_program.cpp -o your_program
When executed, ASan will report any memory safety violations, allowing for immediate debugging.
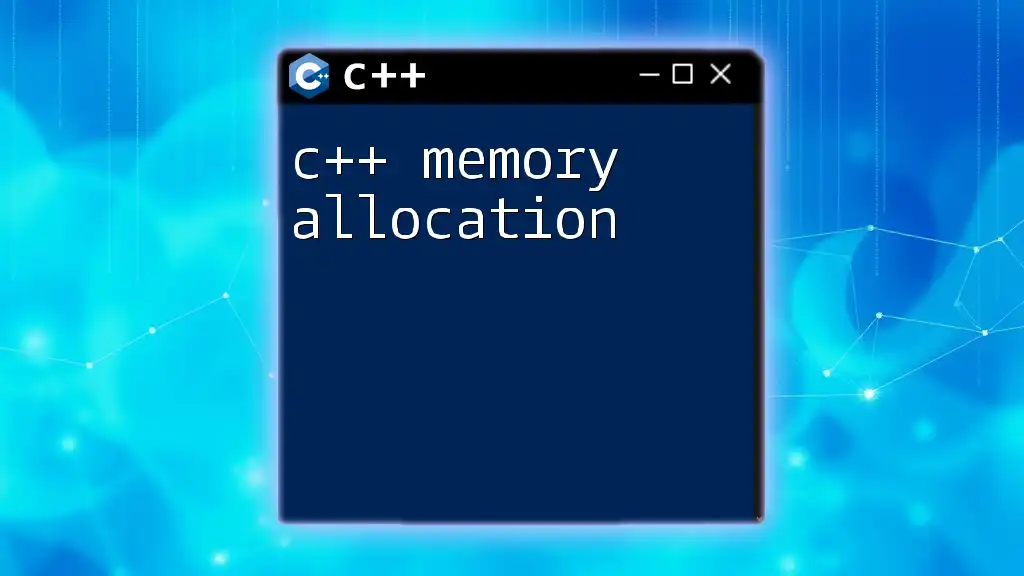
Conclusion
In C++, memory safety is paramount to ensuring the reliability and security of applications. By understanding the intricacies of memory management, employing practices like using smart pointers, and leveraging tools such as static analyzers and address sanitizers, developers can significantly improve their memory safety.
As with any skill, continuous learning and adapting are essential. Embrace safe memory management techniques, and always stay vigilant against potential pitfalls in your C++ programming journey.