A C++ memory leak occurs when allocated memory is not properly deallocated, leading to wasted memory resources and potential program instability.
Here’s a simple example to illustrate a memory leak:
#include <iostream>
void createMemoryLeak() {
int* leak = new int(42); // dynamically allocated memory
// Memory is not freed, causing a leak
}
int main() {
createMemoryLeak();
// Program terminates without deleting the allocated memory
return 0;
}
Understanding Memory Leaks in C++
What is a Memory Leak?
A memory leak occurs when a program allocates memory but fails to release it back to the operating system after use. This means that even though the program may no longer need the allocated memory, it remains unusable for other processes. In C++, dynamic memory management is often necessary when allocating memory during runtime, typically through functions like `new` and `malloc`, but failing to deallocate these allocations with `delete` or `free` leads to leaks.
Why Memory Leaks are a Concern
Impact on Performance: Memory leaks can significantly affect an application’s performance. If memory is not released, the operating system has less available memory, leading to potential slowdowns, increased paging, and even crashes in severe cases.
Debugging Challenges: Debugging memory leaks can be arduous, especially in large codebases where tracking down every allocation and deallocation is difficult. This can lead to frustration and increased development time.
Long-Term Effects: Over time, applications with memory leaks may consume more resources, making them less efficient and more prone to crashes. This is particularly detrimental for long-running processes, such as servers or embedded systems.
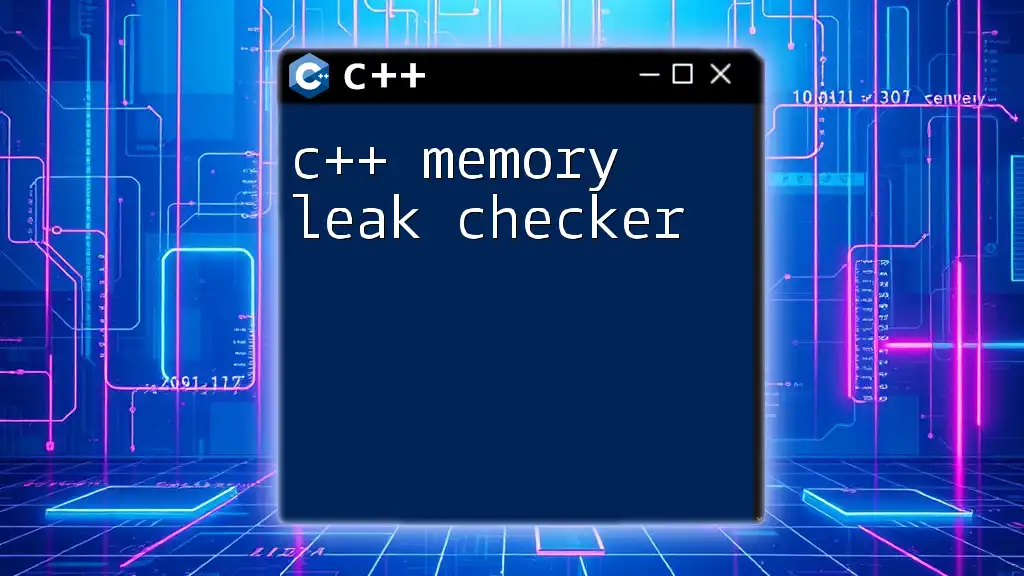
Common Causes of Memory Leaks in C++
Improper Memory Management
One of the most common causes of memory leaks is not deallocating memory that has been dynamically allocated. Consider the following example:
void exampleFunction() {
int* array = new int[10]; // Allocate memory for an array of 10 integers
// Do something with the array
// Forgetting to delete the array leads to a memory leak
}
In this case, the memory allocated for `array` is never deallocated with `delete[]`, resulting in a leak.
Lost References
Memory leaks can also happen when pointers to allocated memory are overwritten. Here's an example:
void lostReferenceExample() {
int* ptr = new int(42); // Memory allocated
ptr = new int(55); // New memory allocated; previous memory lost
delete ptr; // Memory is freed, but the initial allocation is not handled
}
In this scenario, we lose the reference to the original integer before freeing it, creating a leak.
Circular References
Circular references can also happen, particularly with smart pointers. Consider this example:
#include <memory>
struct Node {
std::shared_ptr<Node> next;
};
void circularReferenceExample() {
std::shared_ptr<Node> node1 = std::make_shared<Node>();
std::shared_ptr<Node> node2 = std::make_shared<Node>();
node1->next = node2;
node2->next = node1; // circular reference
// When node1 and node2 go out of scope, memory is not freed
}
In the example above, both `node1` and `node2` reference each other, preventing the memory from being deallocated when they go out of scope.
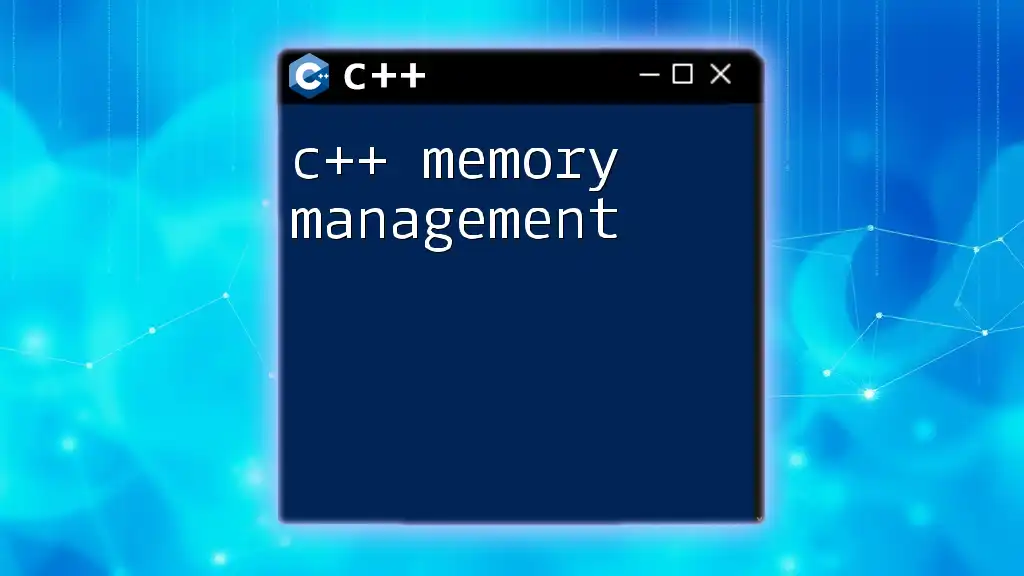
Identifying Memory Leaks in Your Code
Tools for Detecting Memory Leaks
To identify memory leaks effectively, several tools are available for C++ programmers:
Valgrind: This popular tool can help detect memory leaks, invalid memory access, and other memory-related issues. Running a program with Valgrind yields a report of memory allocations that have not been freed.
AddressSanitizer: Integrated into many compilers, AddressSanitizer is a powerful tool for detecting memory corruption and leaks. Compile your code with the `-fsanitize=address` flag and run your application to get detailed diagnostics.
Manual Detection Techniques
Using Debugging Methods: If you wish to identify leaks manually, consider inserting reasonable checks into your code. Logging allocation and deallocation can provide insights:
void* operator new(size_t size) {
std::cout << "Allocating " << size << " bytes\n";
return malloc(size);
}
void operator delete(void* ptr) noexcept {
std::cout << "Freeing memory\n";
free(ptr);
}
Code Review Practices: Regular code reviews focusing on proper memory management practices can catch potential leaks before they go live. Encourage team members to utilize best practices and knowledge-sharing.
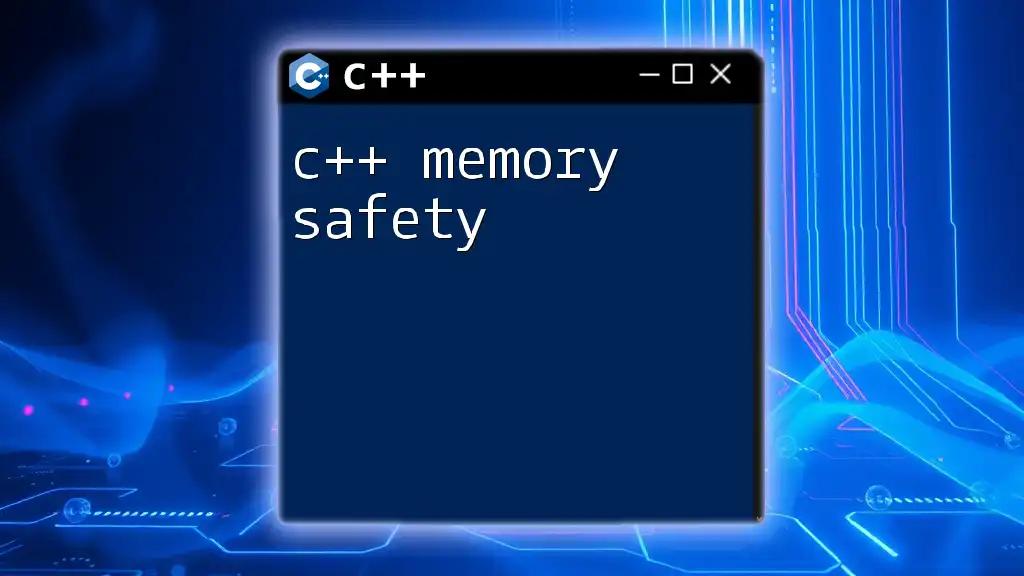
Preventing Memory Leaks in C++
Best Practices for Memory Management
A few best practices can help prevent memory leaks effectively.
Always Match `new` with `delete`: Always ensure that every block of memory allocated with `new` has a corresponding `delete`. This principle sounds simple but is critical in avoiding memory leaks.
void practiceMemoryManagement() {
int* number = new int(10);
// Use number
delete number; // Correctly deallocate memory
}
Utilizing Smart Pointers: By using smart pointers such as `std::unique_ptr` and `std::shared_ptr`, C++ programmers can reduce the risk of memory leaks. These smart pointers automatically manage memory and ensure proper deallocation when they go out of scope:
#include <memory>
void smartPointerExample() {
std::unique_ptr<int> smartPtr(new int(20)); // Automatically managed memory
// No need for delete; memory will be freed when smartPtr goes out of scope
}
Coding Conventions
Clear Ownership Rules: Establish clear ownership rules in your codebase to help delineate which parts of the code are responsible for freeing memory.
RAII (Resource Acquisition Is Initialization): The RAII pattern should be a core principle of C++ programming. This approach models resource management as part of object lifetime, whereby resources are acquired during object construction and released during destructors.
class Resource {
public:
Resource() { memory = new int[10]; }
~Resource() { delete[] memory; } // Automatically released
private:
int* memory;
};
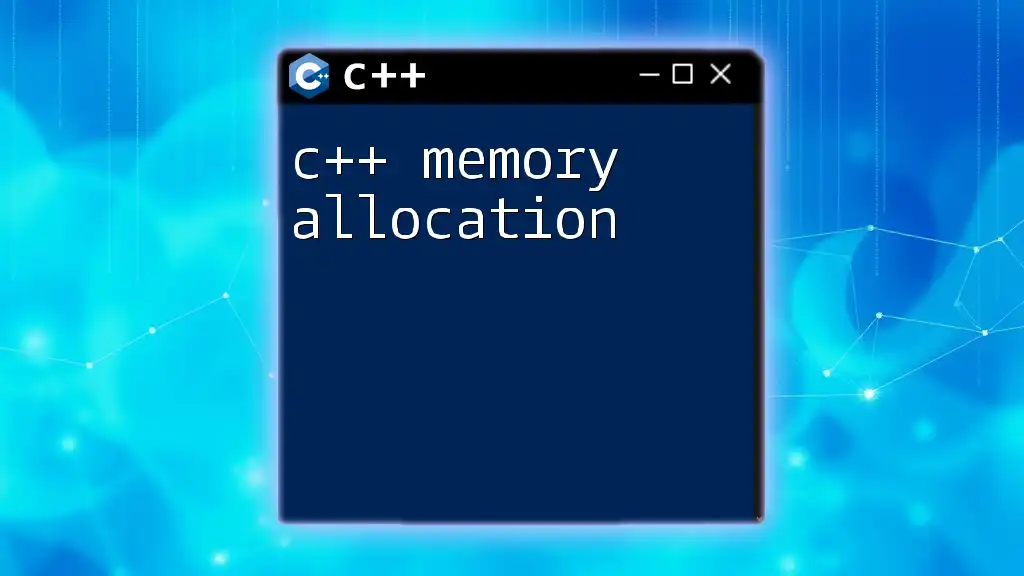
Troubleshooting Memory Leaks
Analyzing Leak Reports
Understanding leak reports is fundamental to troubleshooting memory leaks. Valgrind and AddressSanitizer produce detailed output that indicates where memory was allocated and not freed. Interpreting these outputs correctly can lead you directly to the source of the leak.
Real-World Scenarios
Consider case studies where developers encountered memory leaks—often leading to increases in memory usage or application crashes. By analyzing these instances, developers can learn to diagnose their own memory management issues.
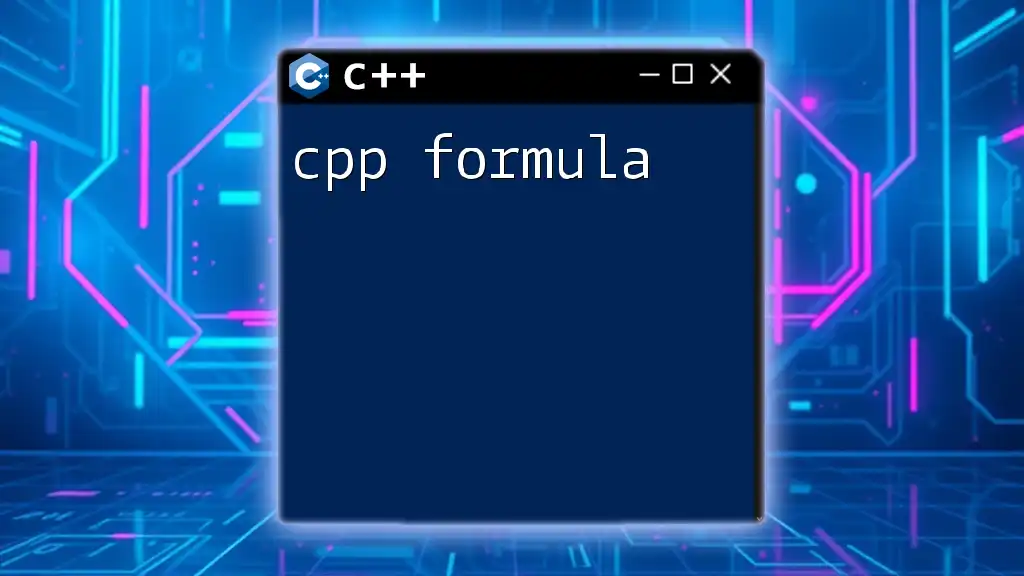
Conclusion
Key Takeaways
To recap, addressing cpp memory leaks involves a solid understanding of memory allocation and deallocation practices. Ensure you always match your `new` with `delete`, prefer smart pointers when handling dynamic memory, and enforce coding conventions that promote clear ownership models.
Encouragement to Practice
Encourage continuous practice of memory management techniques to build better habits, improving both the reliability of code and your effectiveness as a C++ developer.
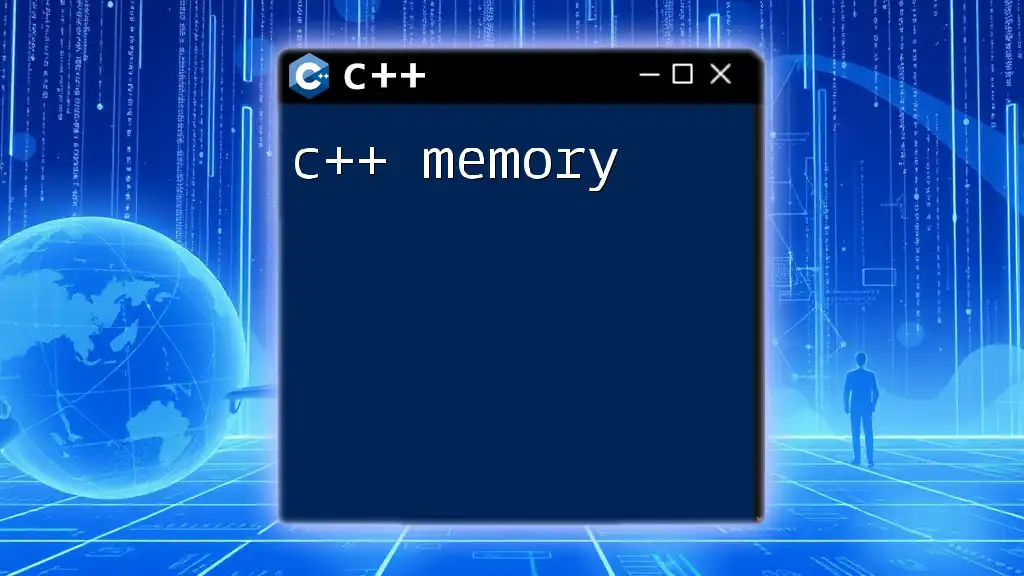
Additional Resources
Recommended Reading and Tools
For further learning, consider digging into books that specialize in C++ memory management, engaging with online tutorials, and participating in forums dedicated to advanced C++ topics.
Call to Action
Join our community where you can engage with peers, share knowledge, and expand your understanding of effective C++ command usage while avoiding pitfalls like memory leaks.