A C++ formula is a structured expression that combines variables, operators, and functions to perform calculations or manipulate data efficiently.
Here’s a simple code snippet demonstrating a C++ formula that calculates the area of a rectangle:
#include <iostream>
using namespace std;
int main() {
float length = 5.0; // Length of the rectangle
float width = 3.0; // Width of the rectangle
float area = length * width; // C++ formula for area calculation
cout << "Area of the rectangle: " << area << endl;
return 0;
}
What is C++?
C++ is a powerful and versatile programming language that enables developers to write efficient and high-performance applications. With its origins dating back to the early 1980s, C++ has become a staple in various domains, including systems programming, game development, and real-time simulation. The language allows for both procedural and object-oriented programming, making it adaptable for a wide range of programming paradigms.
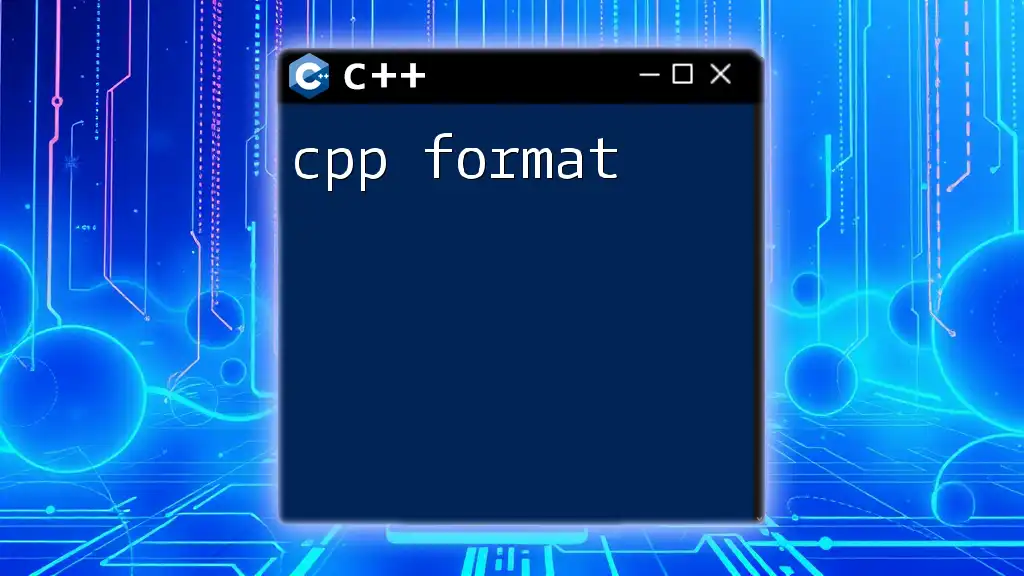
Understanding C++ Formulas
In the context of C++, a formula typically refers to a mathematical expression or computation that employs various data types and operators to produce a result. CPP formulas are essential for performing calculations, algorithms, and logic in programming. They form the backbone of applications by allowing developers to manipulate data and yield meaningful outputs from processes.
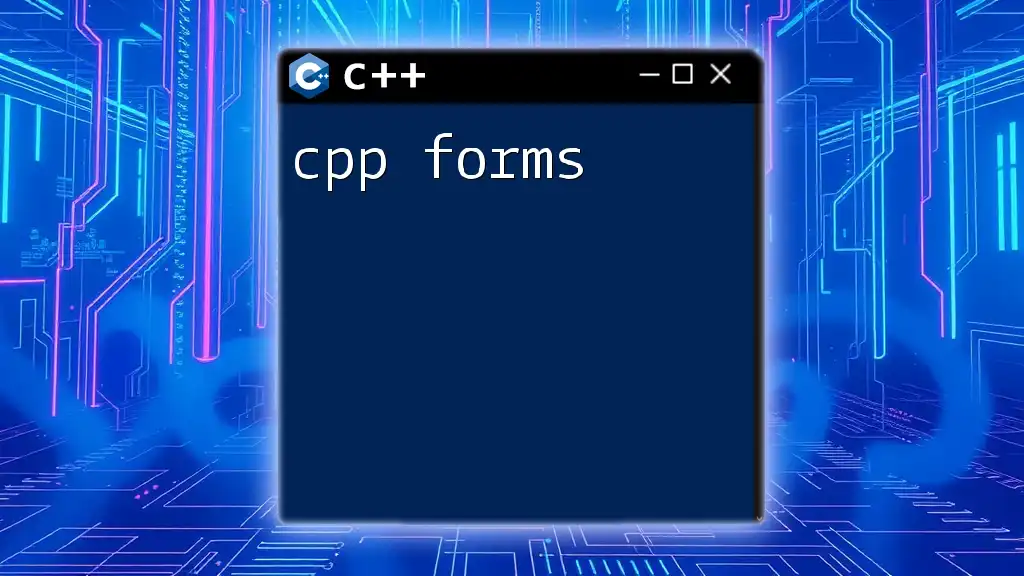
C++ Syntax Fundamentals
Basic Structure of a C++ Program
Every C++ program has a basic structure, comprised of key components, such as headers, namespaces, functions, and the `main()` function. Within this structure, various operations and formula applications occur.
Here is a simple illustration of a basic C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Variables and Data Types
C++ offers several data types, allowing for diverse applications of formulas. You can define variables of different types:
- int for integers
- float and double for floating-point representations
- char for individual characters
- bool for boolean values (true or false)
Choosing the correct data type is crucial as it affects the performance and capabilities of your applications. For instance:
int age = 25;
float salary = 80000.50;
char grade = 'A';
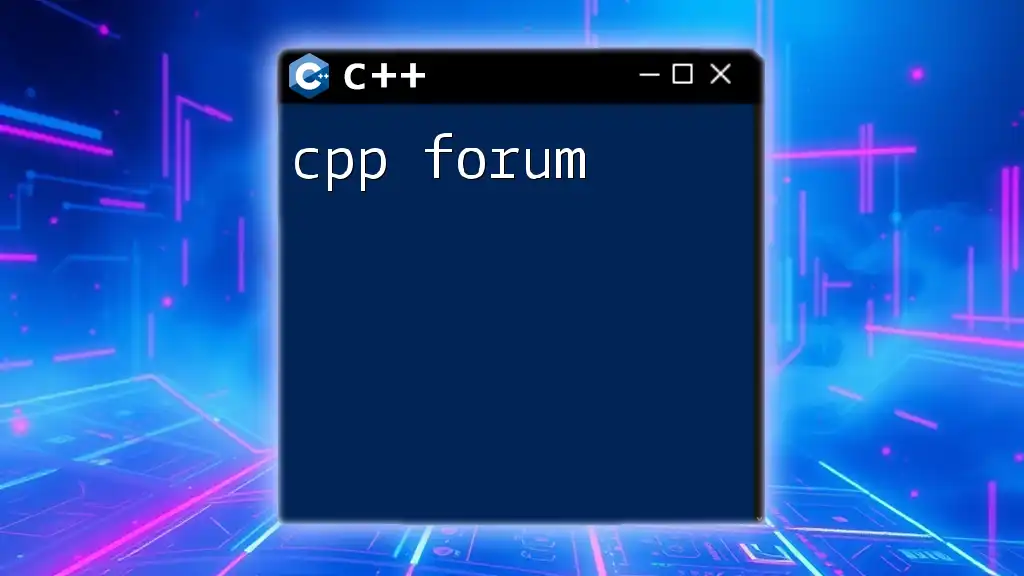
Creating Formulas in C++
Using Operators in C++
Operators are the building blocks of formulas in C++. They facilitate the manipulation of variables and execution of calculations. C++ supports various types of operators:
- Arithmetic Operators: `+`, `-`, `*`, `/`, `%`
- Relational Operators: `==`, `!=`, `<`, `>`, `<=`, `>=`
- Logical Operators: `&&`, `||`, `!`
Here’s how we can use these operators to create a simple formula to calculate the sum and product of two integers:
int a = 10, b = 5;
int sum = a + b; // Addition
int product = a * b; // Multiplication
Implementing Mathematical Functions
C++ also provides the `<cmath>` library, which includes a plethora of mathematical functions, enhancing your ability to create formulas. Functions like `sqrt`, `pow`, and trigonometric functions are invaluable in various calculations.
For example:
#include <cmath>
double result = sqrt(16); // result will be 4.0
double power = pow(2, 3); // power will be 8.0
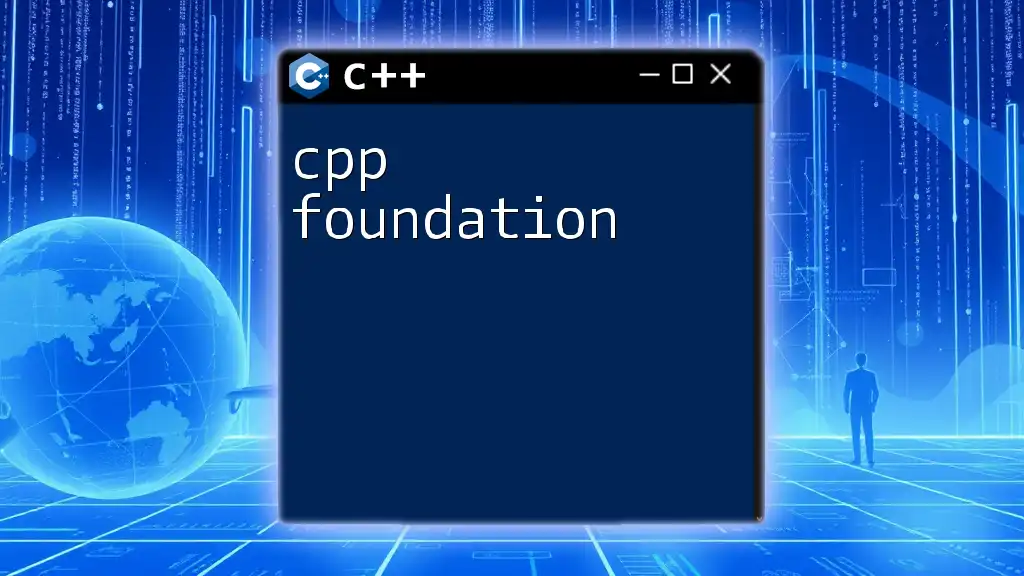
Functions: Making Formulas Reusable
Creating User-Defined Functions
Functions play a key role in C++ by promoting code reusability and organization. You can create your own functions to execute specific formulas and reuse them throughout your program. Here’s an example of a simple addition function:
double add(double x, double y) {
return x + y;
}
Function Overloading
C++ offers a powerful feature known as function overloading, which allows you to define multiple functions with the same name but different parameter types or counts. This flexibility can simplify the code and enhance readability.
Here’s a quick example:
double add(int a, int b) { return a + b; }
double add(double a, double b) { return a + b; }
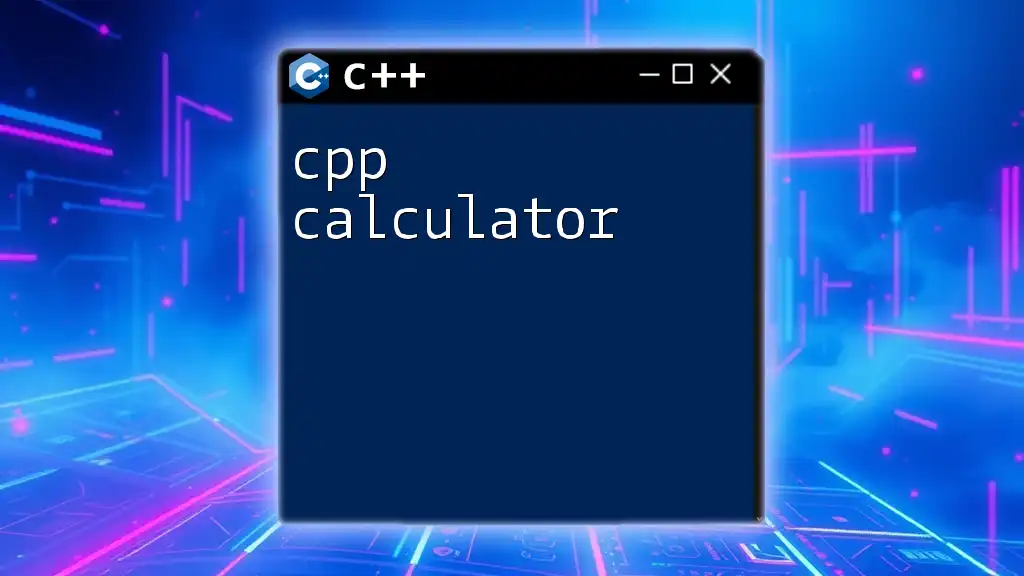
Advanced Concepts in C++ Formulas
Templates: Generic Programming
Templates allow you to write generic functions capable of operating on different data types without having to redefine the functions multiple times. This aspect is crucial when creating formulas that can accommodate various data types dynamically. Below is a simple template for an addition function:
template <typename T>
T add(T a, T b) {
return a + b;
}
Lambda Functions for Short Formulas
C++11 introduced lambda functions, which provide a concise way to create anonymous functions. They are particularly useful for short formulas that don’t need to be reused across the program.
For example:
auto sum = [](int a, int b) { return a + b; };
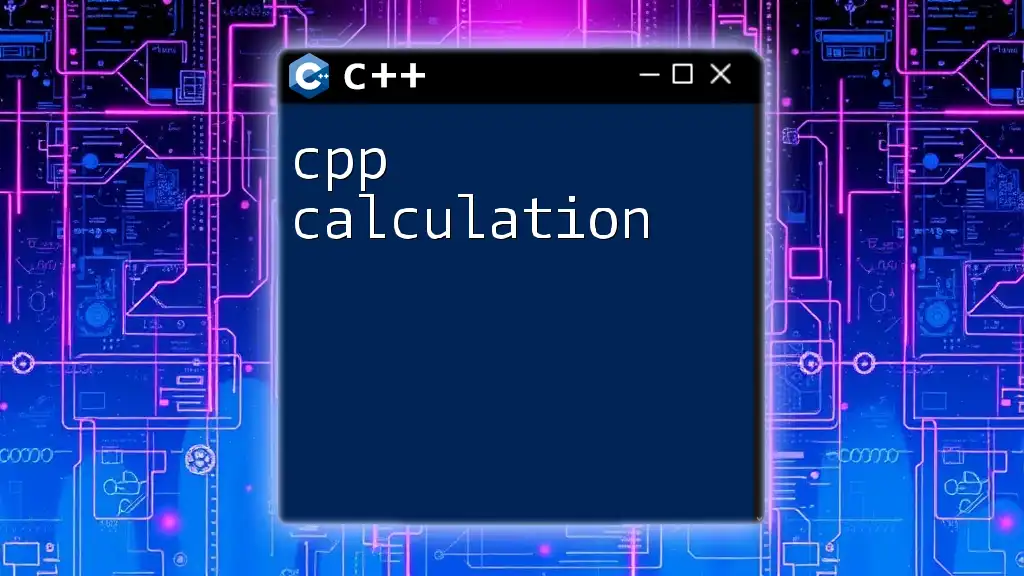
Error Handling in C++
Handling Errors for Robust Formulas
Ensuring that your formulas are robust is vital, especially when dealing with user input or external data sources. C++ provides exception handling through `try` and `catch` blocks, allowing you to manage errors gracefully and maintain control over your application’s flow.
Here’s an example of how to handle exceptions when performing calculations:
try {
throw "An error occurred!";
} catch (const char* msg) {
cerr << msg << endl;
}
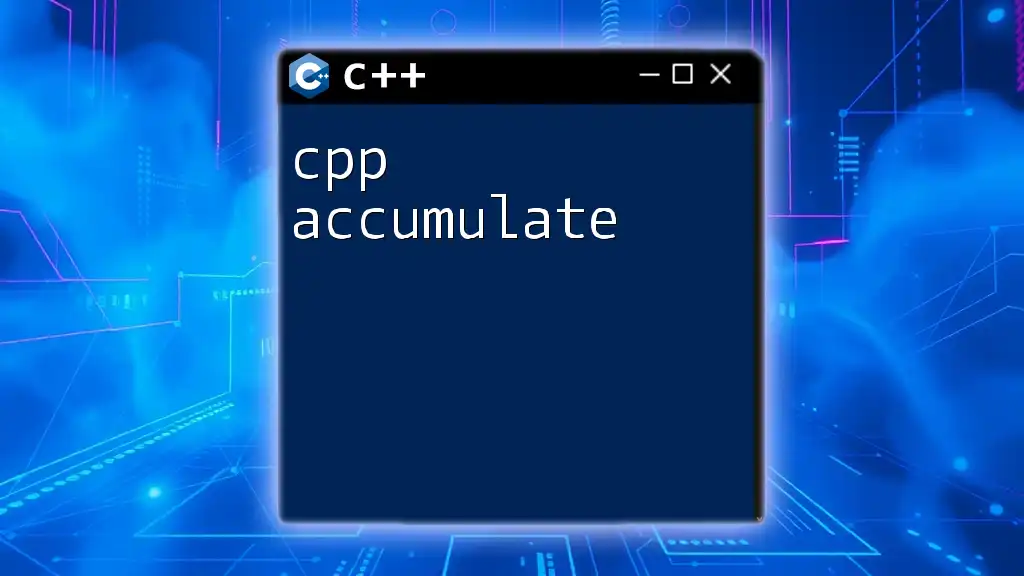
Practical Applications of C++ Formulas
Real-World Example: Scientific Calculators
C++ is commonly used to build scientific calculators that implement a variety of mathematical formulas and functionality. You might define functions to handle common calculations involving square roots, powers, and more. Here’s a simple representation:
double calculateSquareRoot(double number) {
return sqrt(number);
}
double calculatePower(double base, double exponent) {
return pow(base, exponent);
}
Developing Simple Games Using Formulas
Formulas are extensively utilized in game development. They can govern scoring systems, player statistics, collision detections, and physics simulations. For instance, updating a score within a game can easily be expressed as:
int score = 0;
score += 10; // Updating score in-game
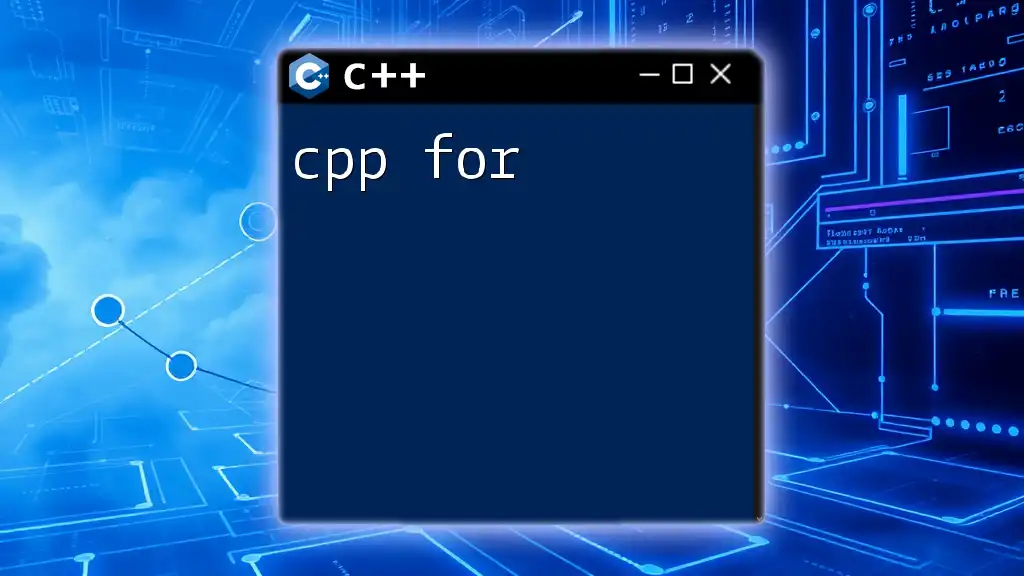
Conclusion
In summary, mastering C++ formulas is essential for creating effective and efficient programs. By leveraging variables, operators, functions, and advanced concepts within C++, developers can tackle complex calculations and enhance application performance. As you delve deeper into the realm of C++, remember to practice frequently and challenge yourself with more intricate topics.
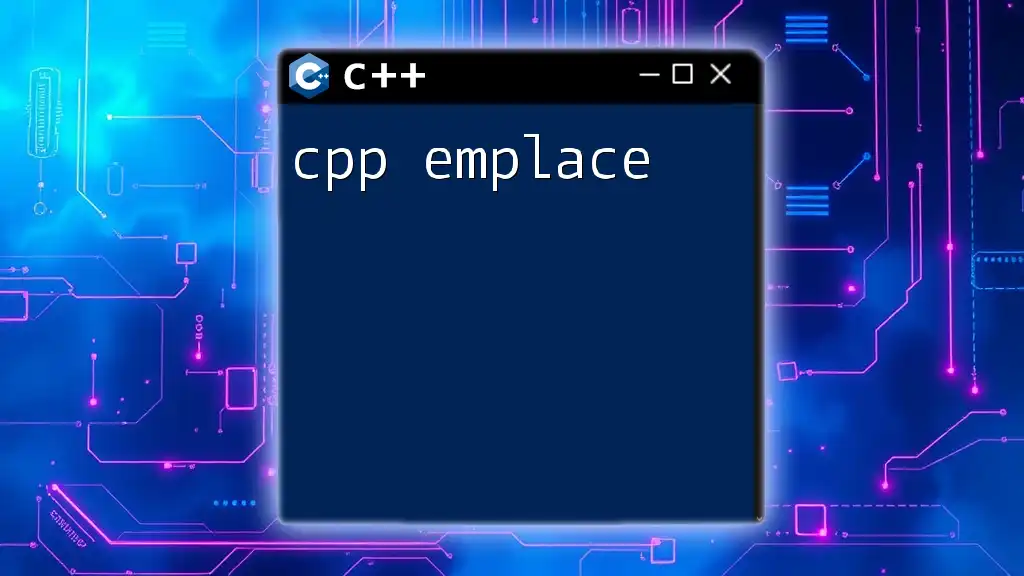
Further Resources
For those eager to expand their understanding of C++, consider exploring comprehensive programming books, online courses, and interactive coding platforms that focus on C++.
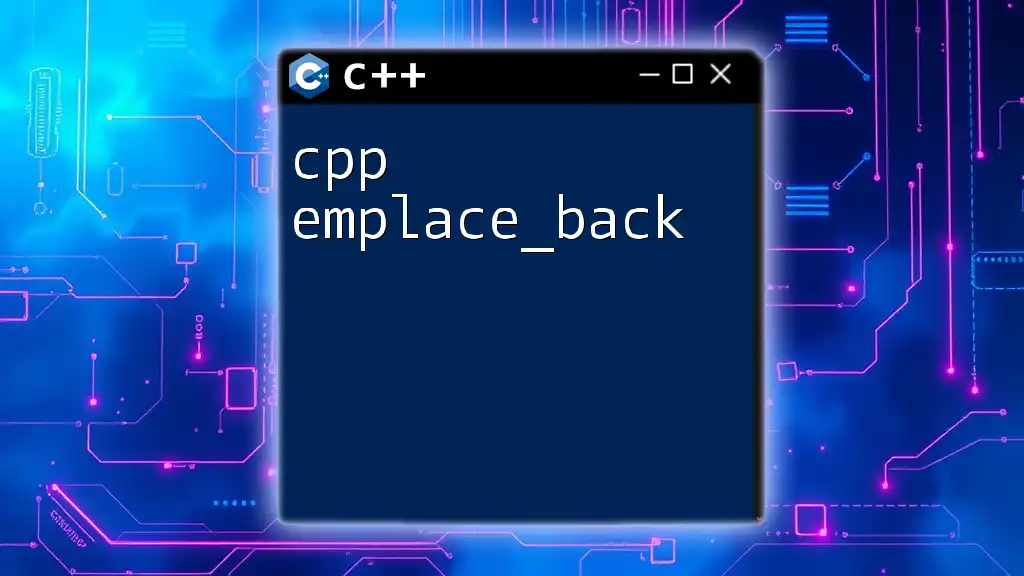
Call-to-Action
We’d love to hear about your experiences or queries related to C++ formulas. Feel free to share your thoughts or ask questions in the comments section below. Your input is invaluable as we grow this community devoted to mastering C++!