A C++ forum can be a valuable platform for developers to share knowledge, ask questions, and discuss best practices in using C++ commands effectively.
Here’s a simple code snippet demonstrating a basic C++ command to output text:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Forum?
A C++ forum is an online platform where programmers and enthusiasts gather to engage in discussions about C++ programming. Such forums serve multiple purposes: they facilitate learning, provide a space for sharing knowledge, and assist with troubleshooting coding issues. By participating in a C++ forum, individuals can gain insights into best practices, explore solutions to complex problems, and enhance their overall understanding of the language.
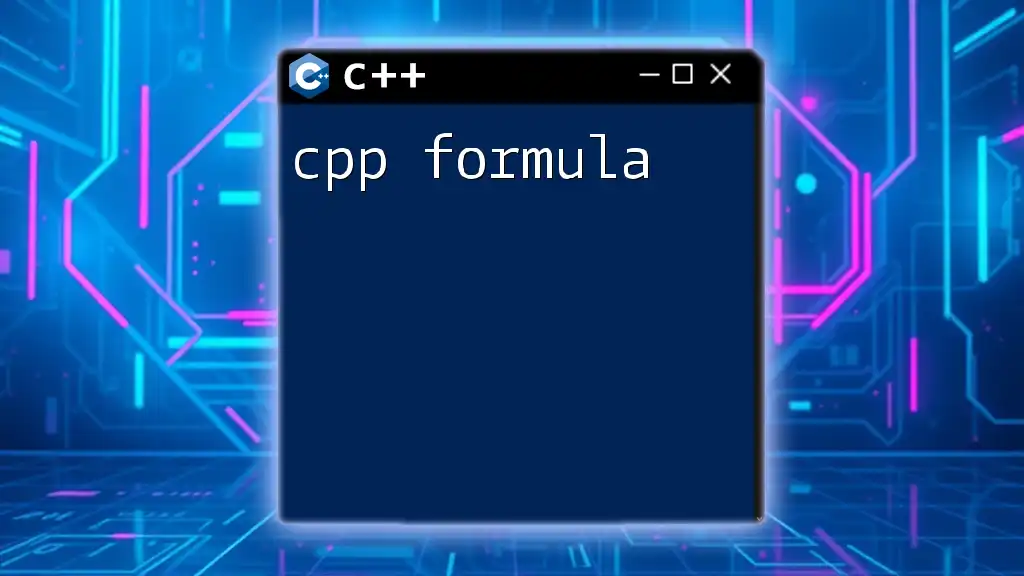
Benefits of Engaging in C++ Forums
Engaging in a C++ forum offers a range of benefits:
Networking Opportunities: One of the greatest advantages of C++ forums is the ability to connect with other programmers, from beginners to experts. This can lead to opportunities for collaboration, mentorship, and even job offers.
Knowledge Sharing: A wealth of resources exists within C++ forums. Members often share code snippets, project ideas, and tutorials, which can be invaluable for learning and improving coding skills.
Problem Solving: Forums are excellent places to seek help with specific coding challenges. By detailing the issue at hand, forum members can provide targeted advice and potential solutions, making troubleshooting easier.
Continuous Learning: C++ is a language that evolves over time. By engaging in forums, programmers can stay updated on new features, industry trends, and best practices, fostering a culture of continuous learning.
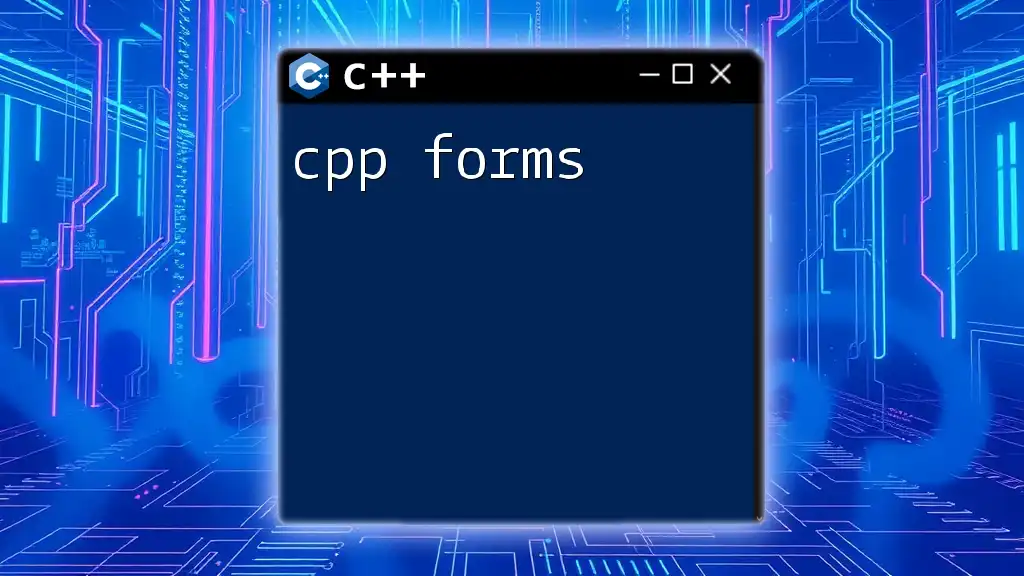
Popular C++ Forums
Several popular C++ forums have become go-to resources for developers:
Stack Overflow: This widely recognized Q&A platform hosts a vast community of professionals and enthusiasts. Users can ask specific questions and receive detailed responses from experienced C++ developers. For instance, a common question such as, "How do I implement a copy constructor in C++?" may yield multiple well-researched answers with various examples.
Cplusplus.com Forums: Focused specifically on C++, this forum allows for dedicated discussions. Members frequently share their projects and ask for feedback, while others post code snippets and usage examples regarding different C++ features.
Reddit - r/cpp: This subreddit offers a more casual atmosphere where members can share news articles, project showcases, and discussion threads. Topics often include new library releases or performance tips, making it a vibrant community for C++ enthusiasts.
C++ Forum: Another excellent resource, this forum provides threads on a range of topics from beginner queries to advanced techniques. Members can engage in discussions about specific challenges or delve into broader topics like best coding practices.
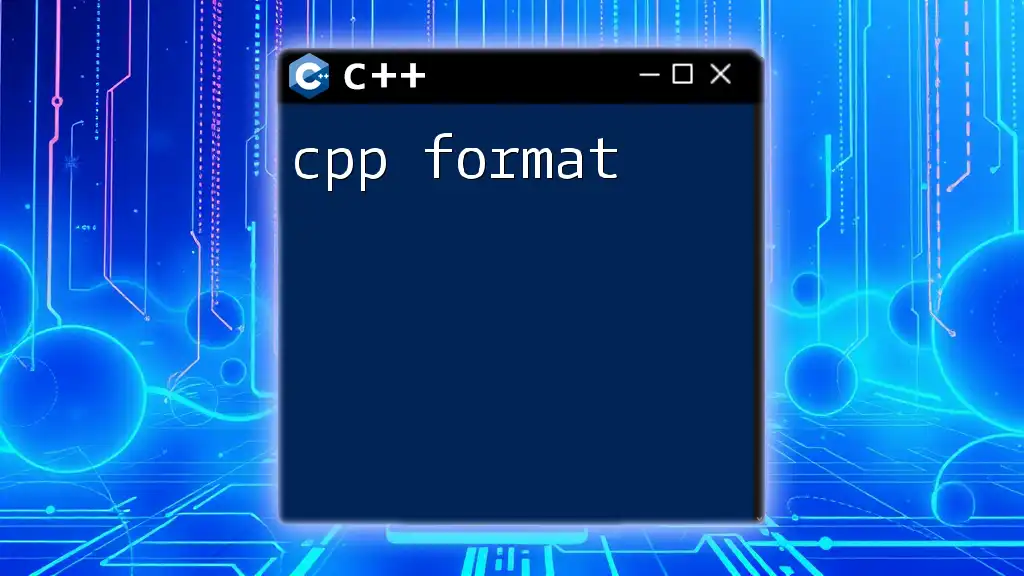
How to Participate in a C++ Forum
Creating an Account: Most forums require users to sign up for an account. The process is usually straightforward, involving providing a username, email, and password.
Reading the Forum Rules: Before diving in, it's essential to understand the community guidelines. Each forum has unique rules that guide interactions and posting behavior.
Posting Questions: For effective communication, it's crucial to ask questions concisely. A well-structured question often includes:
- A clear title that summarizes the issue.
- Relevant context and any attempted solutions.
- Code snippets to illustrate the problem.
For example, a question about a segmentation fault might be phrased as:
Title: "Segmentation Fault when using a Pointer in C++"
Content: "I'm encountering a segmentation fault when trying to access an element of a dynamically allocated array. Here’s the code I’m using:"
#include <iostream>
int main() {
int* arr = new int[5];
std::cout << arr[5]; // Attempting to access out-of-bounds
delete[] arr;
return 0;
}
Answering Questions: When providing answers, ensure they are helpful and well-reasoned. Start by acknowledging the questioner’s issue, then provide a solution, which might include a code example. For instance, you could explain how to safely access array elements to avoid segmentation faults.
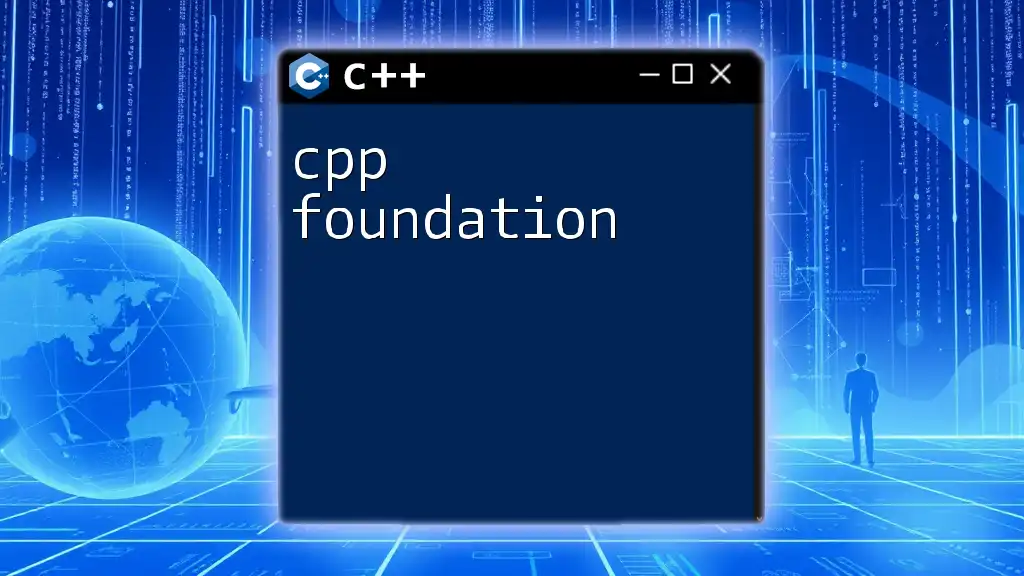
Best Practices for Engaging in C++ Forums
Be Respectful and Courteous: Maintaining a respectful tone is vital in any community. Politeness encourages open communication and creates a positive environment.
Use Proper Formatting: Clear and readable posts are more likely to receive responses. Utilize markdown to format code snippets and emphasize critical points. For instance:
#include <iostream>
// Function to add two numbers
int add(int a, int b) {
return a + b;
}
This formatting enhances readability and helps others quickly understand your code.
Research Before Asking: Before posing a question, do some preliminary research. Searching for similar problems may lead you directly to an answer, saving you time and effort.
Stay on Topic: Keeping discussions relevant to C++ is essential. Off-topic posts can dilute the focus of the forum, making it less effective for all members.
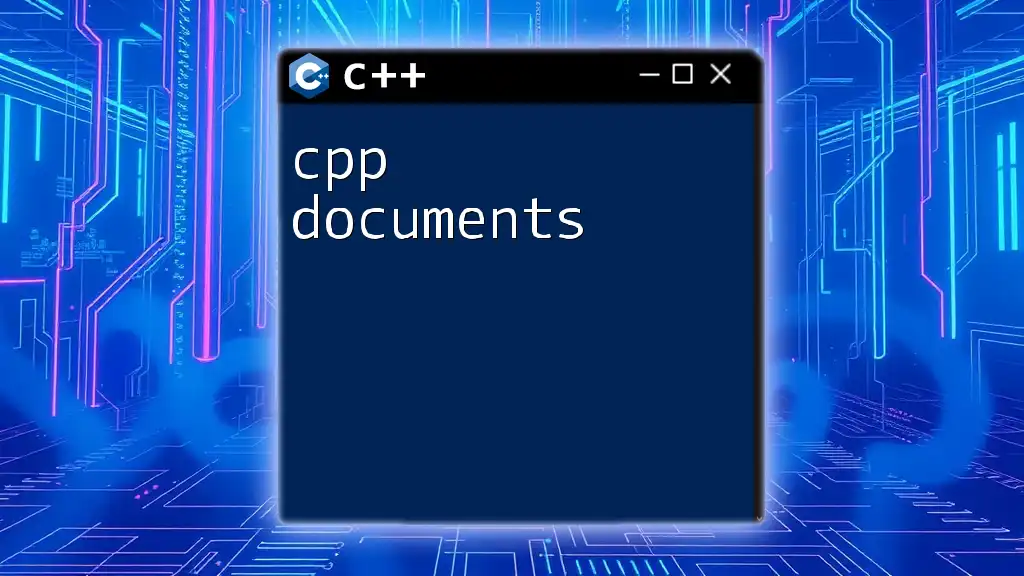
Common Topics Discussed in C++ Forums
C++ forums cover a wide variety of topics, reflecting the language's complexity and versatility:
C++ Basics: Questions about syntax and language constructs are common among beginners. For example, queries about using loops or conditionals often arise.
Memory Management: Discussions surrounding pointers, memory leaks, and resource management are frequently explored, given their critical role in C++ programming.
Object-Oriented Programming: Threads related to classes, inheritance, and polymorphism abound. For instance, developers often seek advice on implementing interfaces in C++.
Templates and STL: The Standard Template Library (STL) is a frequent topic, with users sharing insights about using functions like `std::vector` and `std::map`.
Performance Optimization: Developers frequently ask for advice on optimizing code performance, whether through algorithm improvements or efficient memory usage.
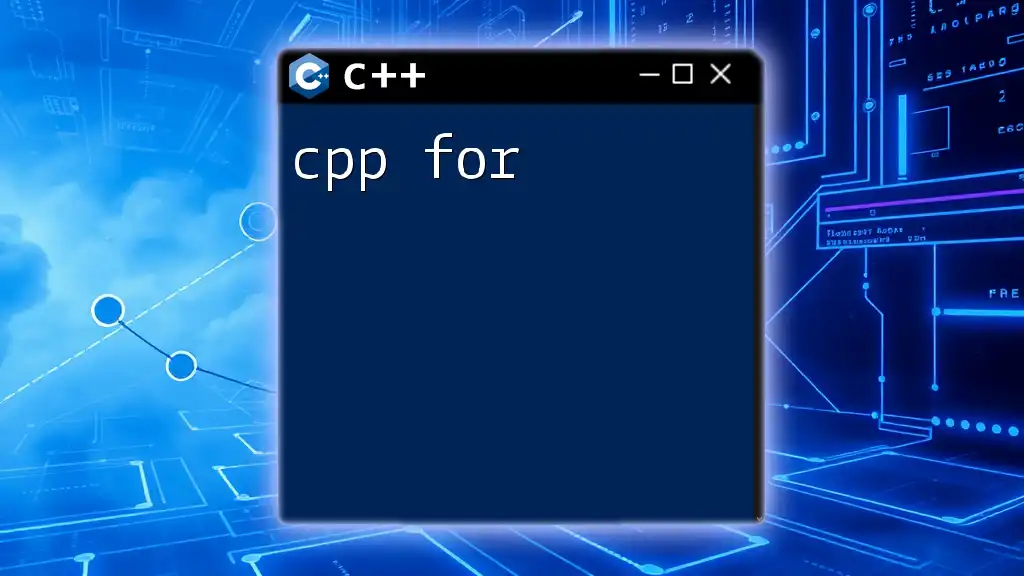
The Role of Moderators in C++ Forums
Enforcing Rules: Moderators play a key role in maintaining the quality of discussions. They ensure that members adhere to the community guidelines, preventing spam and abusive behavior.
Guidance for New Members: Moderators often assist newcomers, providing advice on how to navigate the forum. They may even create introductory threads to guide users in making effective posts.
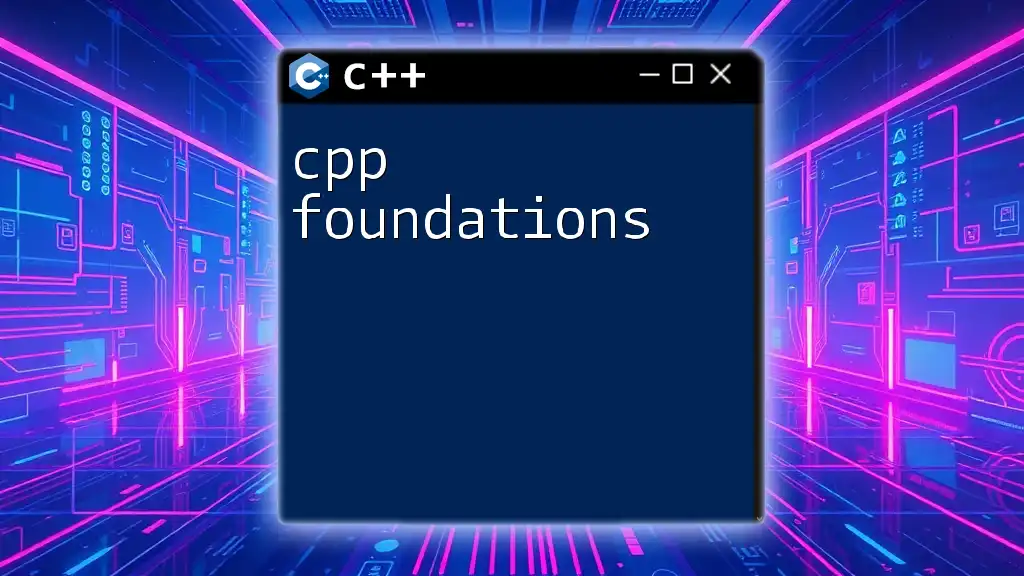
How to Leverage C++ Forums for Personal Growth
Engaging in C++ forums can significantly contribute to your personal growth as a programmer. By conversing with others, sharing experiences, and tackling challenges together, you develop a deeper understanding of C++.
Building Skills Through Interaction: By actively participating in discussions and seeking feedback on your code, you'll gain practical insights that can elevate your skills. Each interaction presents an opportunity for learning.
Contributing to the Community: Sharing your knowledge reinforces your learning and offers valuable resources to others. Answering questions and contributing tutorials can enhance your reputation in the community while diversifying your expertise.
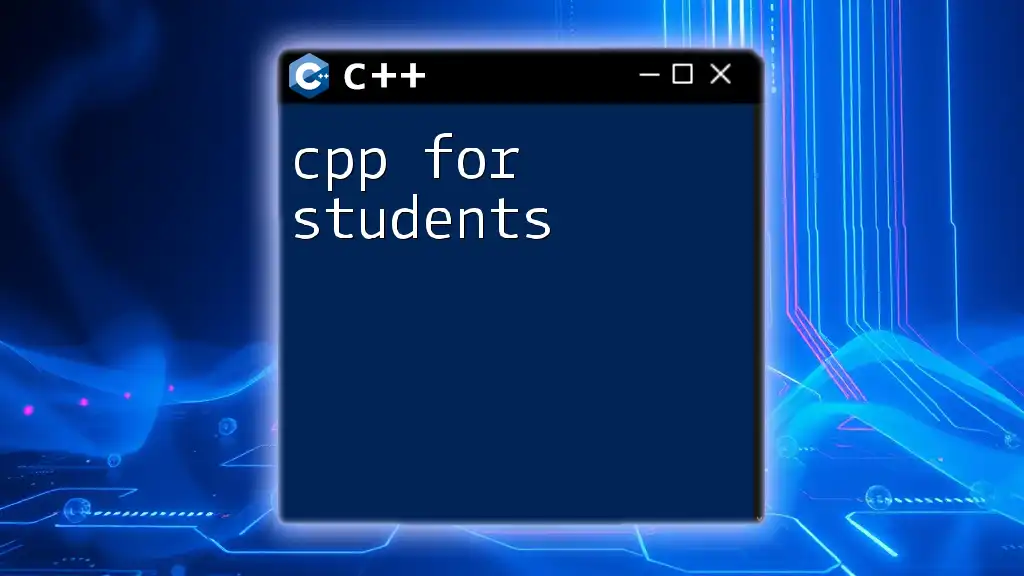
Conclusion
Participating in a C++ forum provides unparalleled opportunities for growth, learning, and community engagement. By sharing knowledge, asking questions, and contributing to discussions, you can significantly enhance your C++ programming journey. Embrace the chance to connect with fellow developers and explore the countless resources available in these vibrant online communities.
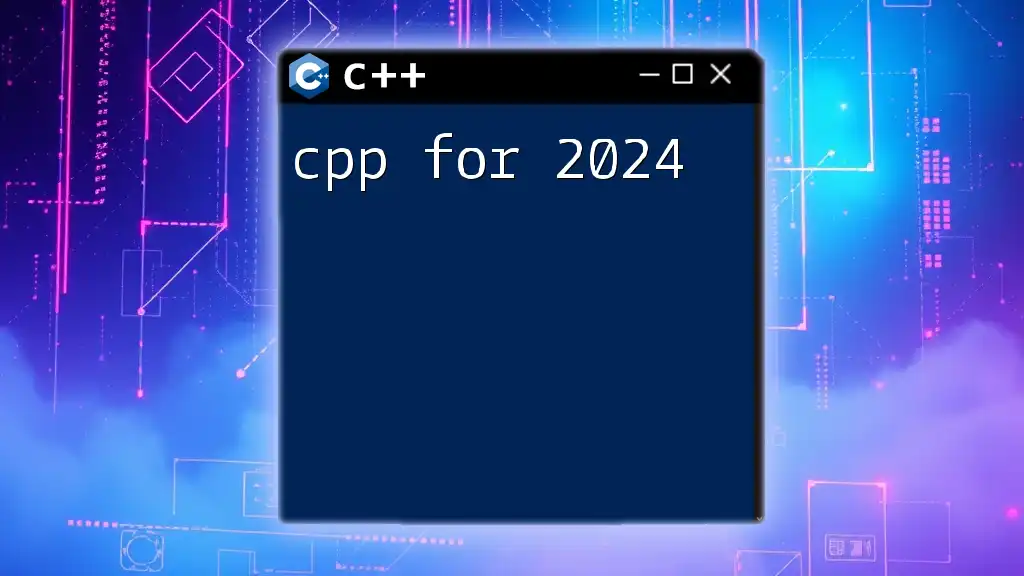
Additional Resources
To supplement your learning, consider exploring additional resources such as C++ tutorials, documentation, and recommended reading lists. Joining relevant forums or communities can further enhance your engagement with the programming landscape.