"CPP for 2024 focuses on empowering learners with quick, concise commands to enhance their C++ programming skills effectively."
Here's a simple code snippet showcasing a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What’s New in C++23 and Beyond
Overview of C++23 Features
C++23 introduces several enhancements and features that significantly improve the language's usability and performance. Some key updates include:
-
Lambdas with implicit captures: This feature allows for easier use of local variables within lambda expressions, leading to cleaner code.
-
New standard library features: C++23 has improved support for ranges, making it easier to process sequences of values without heavy overhead.
-
Static-asserts improvements: The language now supports more complex expressions in static assertions, enabling developers to catch errors at compile-time more effectively.
These updates enable developers to write more expressive and efficient code, helping to address common pain points in modern software development.
Future of C++: Anticipated Changes in C++24 and Beyond
The C++ community is always evolving, and the ISO committees continue to propose new enhancements. Although C++24 is still in the proposal stage, several exciting features are on the table:
-
Pattern matching: This feature aims to simplify code that requires complex branching based on data types.
-
Systematic error handling: Improved error handling mechanisms could significantly reduce runtime errors and improve resilience.
Being aware of these anticipated changes helps programmers prepare to adopt new paradigms and improve their codebases going forward.
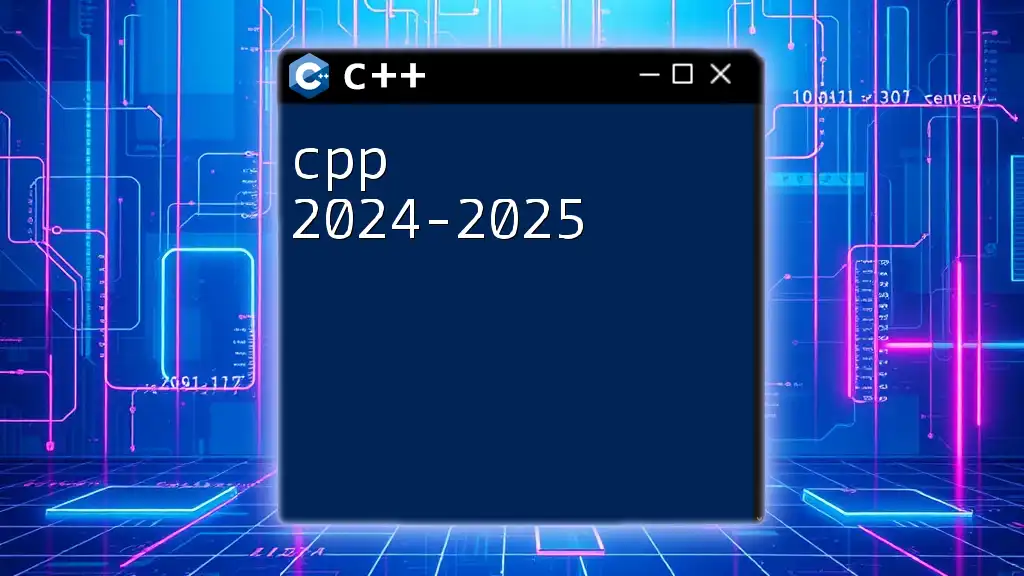
Getting Started with C++ Basics
Setting Up Your C++ Environment
To begin coding in C++, you'll need to set up your development environment. Start by choosing a suitable IDE or text editor. Popular options include:
- Visual Studio: Excellent for Windows users with robust debugging features.
- Code::Blocks: A free, cross-platform IDE that is lightweight and user-friendly.
- CLion: A powerful IDE from JetBrains that supports CMake and comes with various productivity tools.
Next, it’s necessary to install a C++ compiler. You can choose from:
- GCC (GNU Compiler Collection)
- Clang
- Microsoft Visual C++
Once your environment is set, you're ready to start coding.
Basic Syntax and Structure
Understanding C++ syntax and structure is essential for beginners. Here's a classic "Hello, World!" example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code:
- `#include <iostream>` includes the Input/Output stream library, enabling standard console input and output.
- The `main` function serves as the entry point of the program.
- `std::cout` is used to print output to the console.
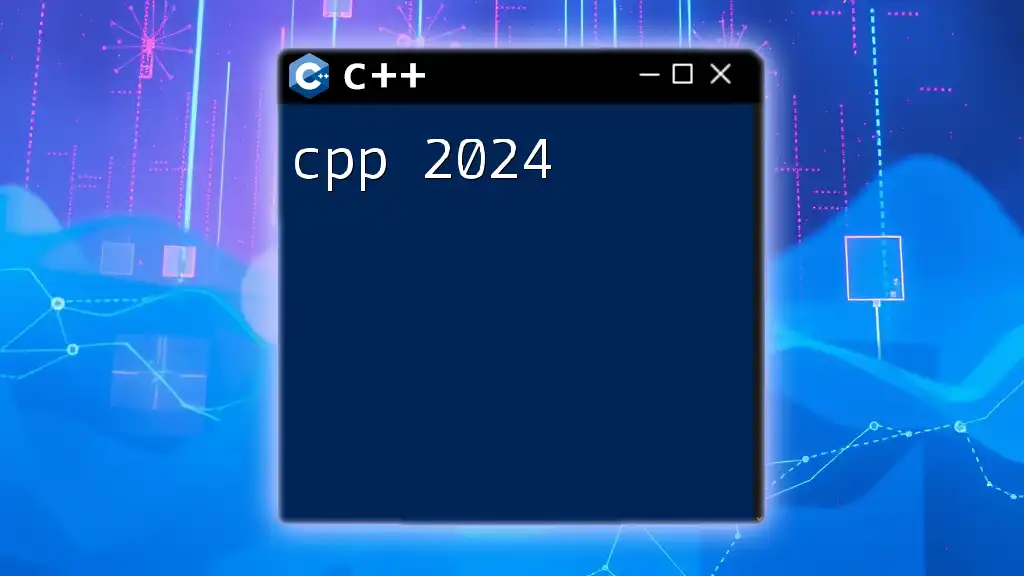
Essential C++ Concepts
Data Types and Variables
C++ supports various data types, both primitive (like `int`, `char`, `float`) and user-defined (like `struct`, `class`). Variable declaration in C++ is straightforward:
int age = 30; // Integer type
float salary = 55000.0; // Float type
char grade = 'A'; // Character type
Control Structures
Control structures allow for decision-making and loops in programming. Use if-else statements for conditional execution, and for and while loops for repetition:
if (age > 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
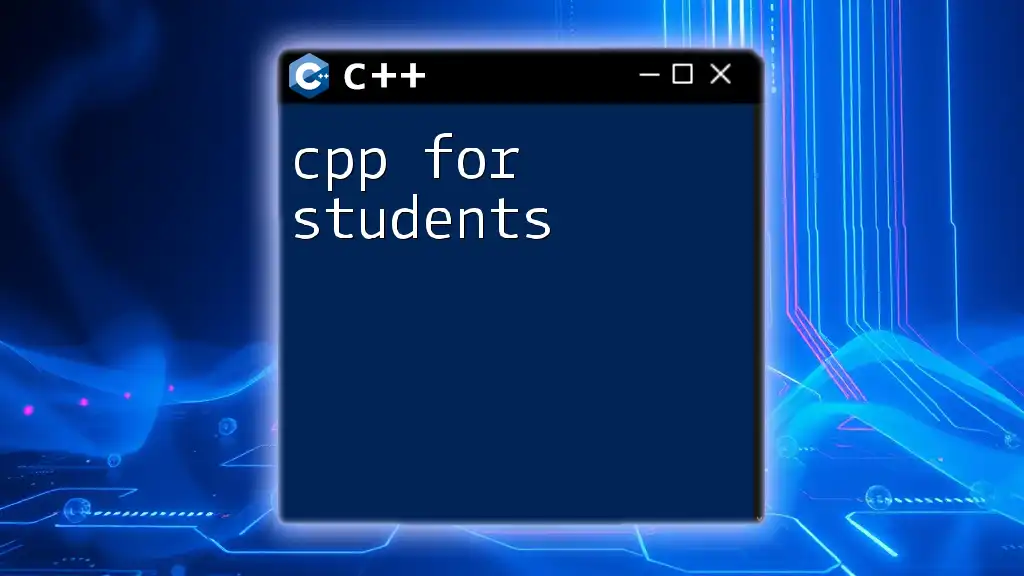
Object-Oriented Programming in C++
Understanding the OOP Paradigm
C++ is known for its support for object-oriented programming (OOP), which enables developers to use classes and objects. Key concepts include:
- Classes: Blueprints for creating objects. They can encapsulate data and functions.
- Objects: Instances of classes that hold state and behavior.
Creating Classes and Objects
Here's an example of creating a simple class:
class Animal {
public:
void speak() {
std::cout << "Animal speaks!" << std::endl;
}
};
Animal dog;
dog.speak();
In this example, we define an `Animal` class and a method `speak()`, which can be called by any instance of the class.
Encapsulation, Inheritance, and Polymorphism
- Encapsulation: Keeping data and functions together, allowing better modularization.
- Inheritance: One class can inherit characteristics from another, promoting code reusability.
- Polymorphism: Allows methods to do different things based on the object calling them.
An example of inheritance:
class Dog : public Animal {
public:
void speak() {
std::cout << "Bark!" << std::endl;
}
};
Dog dog;
dog.speak(); // Outputs: Bark!
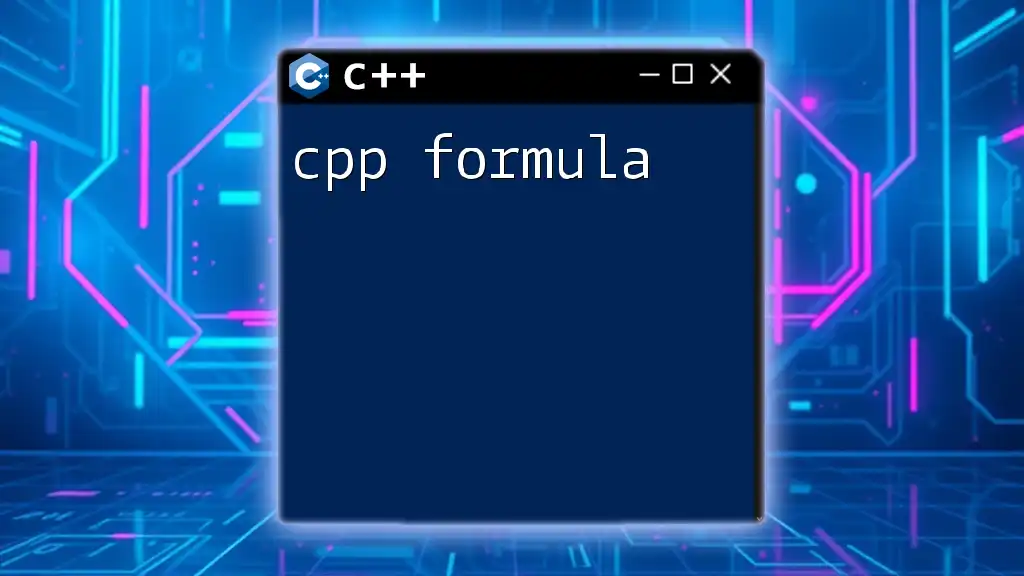
Advanced C++ Features
Templates and Generic Programming
C++ supports templates, allowing functions and classes to operate with generic types. Here’s an example of a simple template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can take parameters of any type that supports the `+` operator.
Smart Pointers and Memory Management
Smart pointers are crucial for effective memory management in modern C++. They help prevent memory leaks and improve performance. Here's an example of using smart pointers:
#include <memory>
std::unique_ptr<int> smartPtr(new int(5));
std::cout << *smartPtr << std::endl; // Outputs: 5
Concurrency in C++
With the rise of multi-core processors, concurrency in C++ has become vital. Here’s how you can create a new thread:
#include <thread>
void threadFunction() {
std::cout << "Thread is working!" << std::endl;
}
std::thread myThread(threadFunction);
myThread.join();
This code illustrates simple threading, allowing your application to perform multiple operations simultaneously.
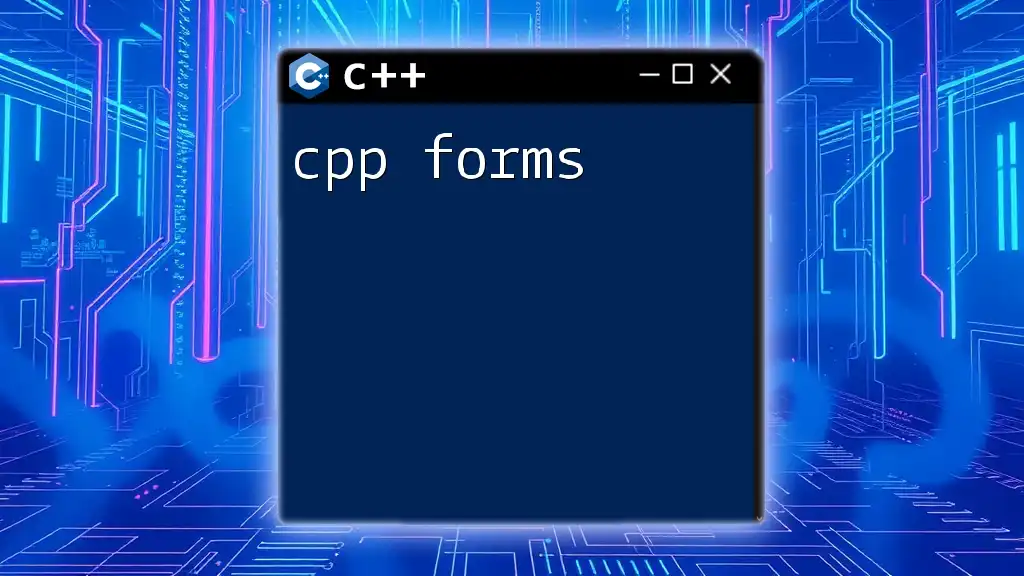
Best Practices for Writing C++ in 2024
Code Style and Formatting
Following a consistent code style improves readability and maintainability. Adhering to principles such as proper indentation, naming conventions, and comment usage is essential.
Debugging Tips and Tools
Utilizing debugging tools like gdb and valgrind aids significantly in identifying and fixing bugs in your code. Familiarity with these tools will save you time and headaches during development.
Performance Optimization Techniques
Performance is crucial in C++. Optimizing your code can involve various techniques like:
- Minimizing memory allocations
- Using algorithmic efficiencies
- Profiling and iterating on code
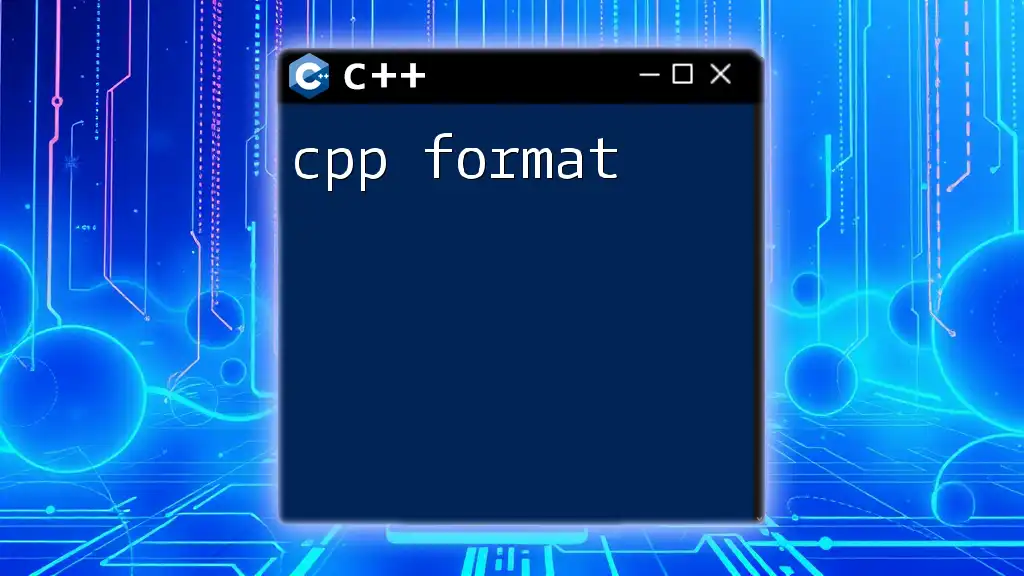
Resources for Further Learning
Top Online C++ Courses and Tutorials
Many platforms offer quality C++ courses for different experience levels. Look for well-reviewed options that cover C++ fundamentals, advanced topics, and industry best practices.
Books for C++ Developers
A few must-read books include:
- The C++ Programming Language by Bjarne Stroustrup
- Effective Modern C++ by Scott Meyers
- C++ Primer by Stanley Lippman
Online Communities and Forums
Engagement with online communities such as Stack Overflow, Reddit’s r/cpp, or various C++ mailing lists is beneficial. Sharing knowledge and seeking help from experienced developers can significantly boost your learning journey.
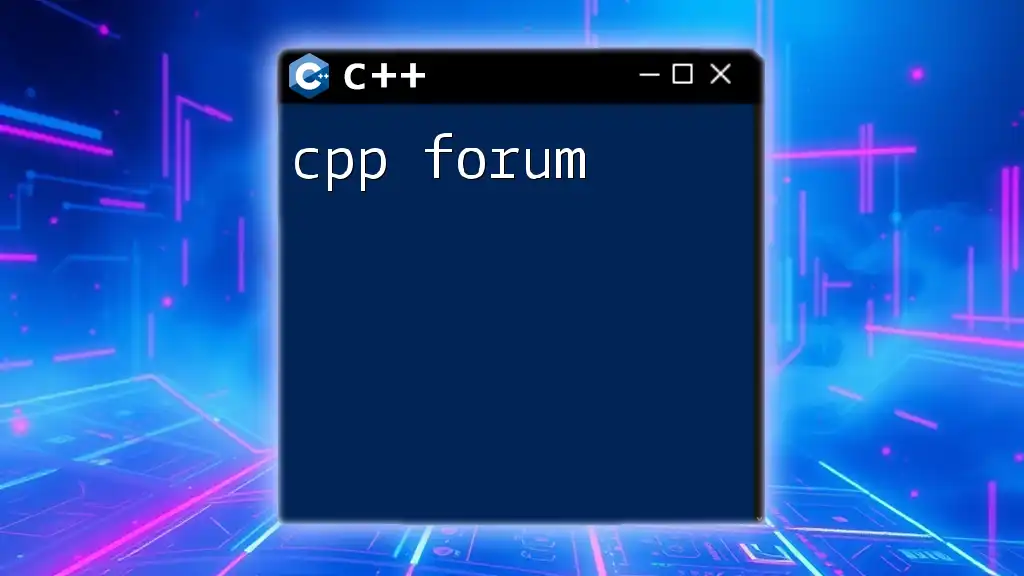
Conclusion
C++ continues to be a powerful language with immense practical applications in software development. With the updates in C++23 and anticipated changes in C++24, now is a great time to dive into C++ for 2024. As you enhance your coding skills, remember that the journey of learning never ends. Join a community or enroll in a course to further deepen your knowledge and expertise in this versatile language.