A C++ for loop allows you to execute a block of code multiple times with a specified initialization, condition, and increment, making it ideal for iterating over arrays or performing repetitive tasks.
for (int i = 0; i < 5; ++i) {
std::cout << "Iteration: " << i << std::endl;
}
Understanding the Basics of For Loops in C++
What is a For Loop?
A for loop in C++ is a fundamental programming structure that allows for the repeated execution of a block of code as long as a specified condition remains true. Loops are essential in programming, enabling developers to automate repetitive tasks efficiently without having to write the same code multiple times.
The Structure of a For Loop in C++
Understanding the for loop syntax in C++ is crucial for mastering loop constructs. A typical for loop consists of three primary components:
-
Initialization: This is where you define and initialize a loop variable. This variable will control the loop iteration.
-
Condition: This expression is evaluated before each iteration. If it evaluates to true, the loop will execute. If it's false, the loop terminates.
-
Increment/Decrement: After each iteration of the loop, the loop variable is updated, which will potentially change the result of the condition for the next iteration.
The basic structure of a C++ for loop can be illustrated as follows:
for (initialization; condition; increment/decrement) {
// Code to be executed during each iteration
}
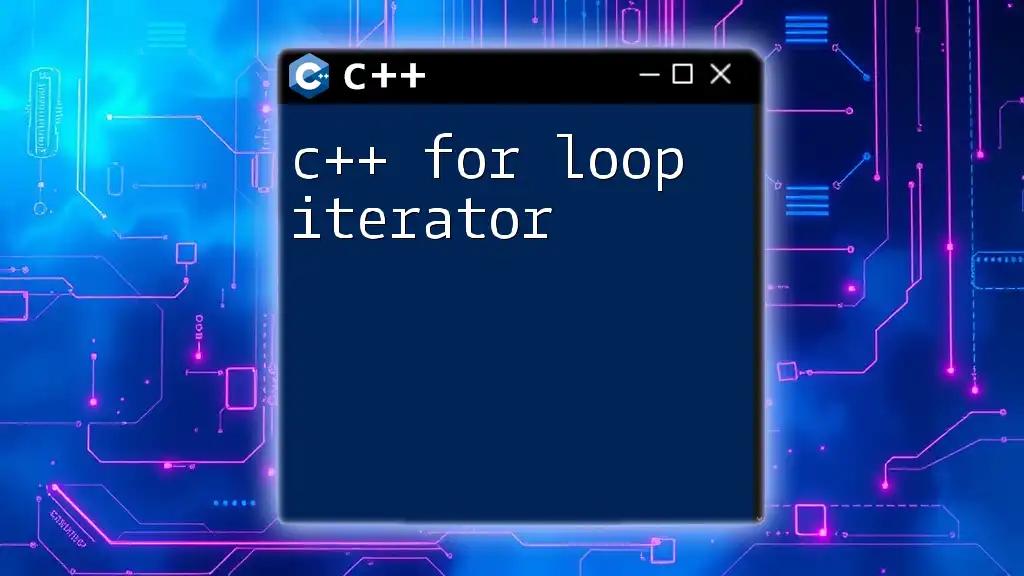
The C++ For Loop Syntax Explained
Basic For Loop Syntax
The most straightforward C++ for loop looks like this:
for (int i = 0; i < 10; i++) {
// Code to be executed
}
In this example:
- `int i = 0;` initializes the loop variable `i` to zero.
- `i < 10` is the condition that dictates how long the loop will run. As long as `i` is less than 10, the loop continues executing.
- `i++` increments `i` by one after each iteration.
Advanced For Loop Variations in C++
Nested For Loops in C++
Sometimes, a single loop isn't sufficient when we have multi-dimensional data. This is where nested for loops come in handy. A nested for loop involves placing one for loop inside another. For example:
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
// Code to be executed in nested loop
}
}
In this case, for every iteration of the outer loop (indexed by `i`), the inner loop (indexed by `j`) runs to completion. Nested loops can be particularly useful for working with two-dimensional arrays or matrices.
Using For Loop with Different Data Types
For loops are not limited to integers alone. They can also iterate over arrays, strings, and other data structures. Here’s how you can use a for loop with an array:
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < sizeof(arr)/sizeof(arr[0]); i++) {
// Accessing array elements
}
Here, `sizeof(arr)/sizeof(arr[0])` calculates the number of elements in the array `arr`, allowing for proper iteration without hardcoding the size.
For Loop with a List or Vector (C++ STL)
The Standard Template Library (STL) includes a variety of useful data structures, such as vectors. A for loop can efficiently iterate through these structures. For example, you can use a for loop in combination with a `std::vector` like this:
#include <vector>
std::vector<int> vec = {1, 2, 3, 4, 5};
for (int i : vec) {
// Code to process vector elements
}
This range-based for loop simplifies iterations over collections, making your code cleaner and more intuitive.
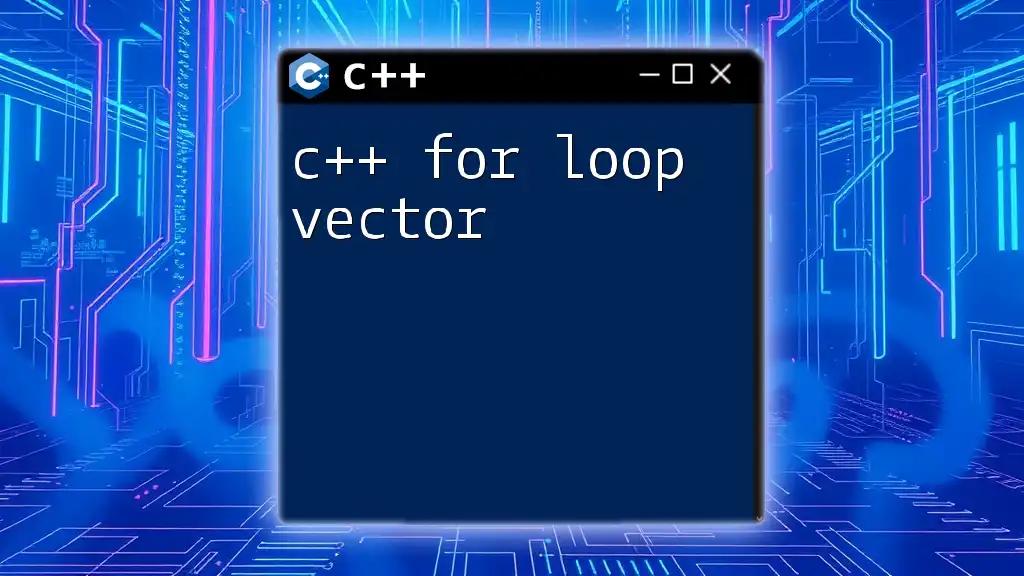
Common Use Cases for For Loops in C++
Iterating Over Collections
For loops are widely used to iterate through collections of data, whether they are arrays, vectors, or other container classes. This capability simplifies tasks such as searching for an element or performing operations on each item in the collection.
Customizing For Loop Behavior
For loops can also be modified to run in various ways. You can easily change the increment step to skip certain elements. For instance, if you want to iterate through even numbers:
for (int i = 0; i < 10; i += 2) {
// Process only even i values
}
This allows you to customize how your loop operates based on specific requirements.
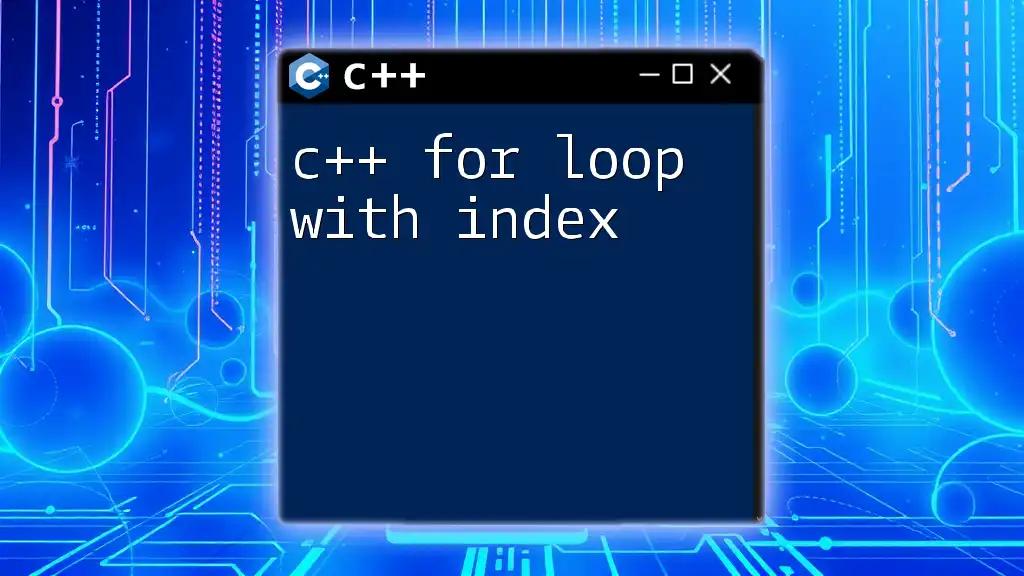
Best Practices for Using For Loops in C++
Writing Clean and Efficient For Loops
When writing C++ for loops, aim for clean, readable, and maintainable code. Always choose clear variable names and structure your loops in a straightforward manner. This enhances the understandability of the code, especially for others who may read it.
Performance Considerations
While for loops are powerful, understanding their impact on performance is also crucial. The complexity of algorithms that utilize for loops can greatly affect the efficiency of your program. Always strive to ensure that your loops do not grow unnecessarily complicated. In some scenarios, it may be more appropriate to use other types of loops, such as `while` or `do-while`, depending on the use case.
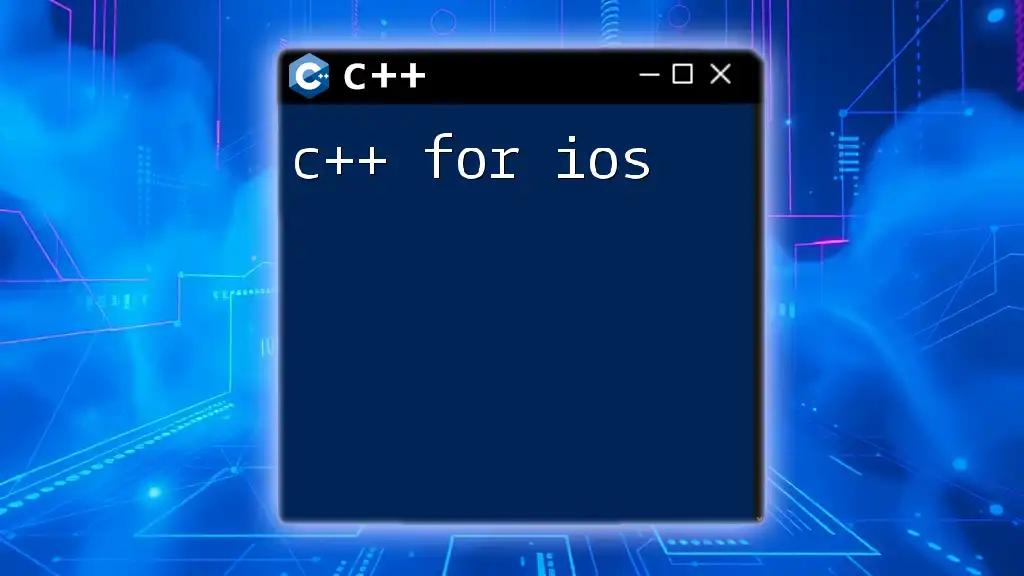
Practical Examples of For Loops in C++
Summing Numbers with a For Loop
A prevalent use case for for loops is summing a series of numbers. Here's a simple example illustrating how to sum numbers from 1 to 100 using a for loop:
int sum = 0;
for (int i = 1; i <= 100; i++) {
sum += i;
}
// sum now holds the total of the first 100 integers
This example effectively demonstrates the cumulative addition of the integers.
Finding Maximum/Minimum Values in an Array
For loops can also be employed to find the maximum or minimum values in an array. Consider the following example of finding the maximum value:
int arr[] = {10, 5, 3, 25, 7};
int max = arr[0];
for (int i = 1; i < sizeof(arr)/sizeof(arr[0]); i++) {
if (arr[i] > max) {
max = arr[i];
}
}
// max now holds the maximum value found
This efficiently scans through the array and updates the maximum value whenever a larger element is found.
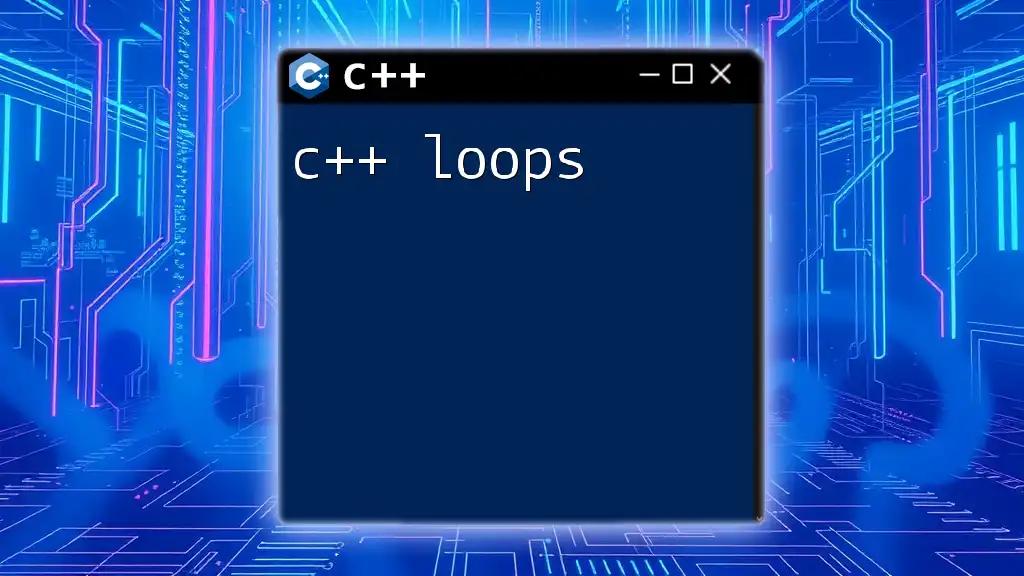
Common Mistakes to Avoid with For Loops
Off-by-One Errors
Off-by-one errors are some of the most common pitfalls encountered when using for loops. These occur when the loop's condition is incorrectly set, leading to either one extra or one fewer iterations than intended. Always double-check your loop conditions to prevent these types of errors.
Forgetting the Increment/Decrement
Another frequent mistake is omitting the increment or decrement statement, which can lead to infinite loops. Always ensure that your loop's execution will eventually lead to the termination of the loop.
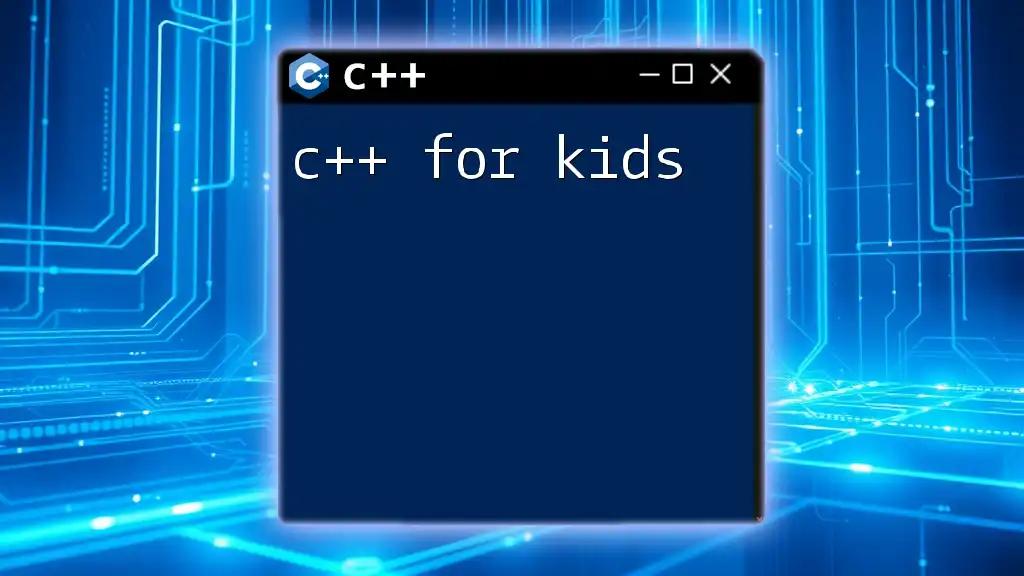
Conclusion
The c++ for loops serve as a cornerstone in efficient programming, enabling the execution of repetitive tasks with ease. Understanding their syntax and structure empowers developers to write better code while reducing redundancy in programming.
By practicing and applying the principles laid out in this guide, you will develop a strong foundation for effectively utilizing for loops in your C++ projects. Happy coding!