"C++ for kids is an engaging way to introduce young learners to programming concepts using simple commands and fun projects, fostering creativity and problem-solving skills."
Here's a basic example of a C++ program that prints "Hello, World!" to the screen:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
C++ Basics
Understanding What Programming Is
Programming is essentially the art of providing instructions to a computer, telling it exactly what to do step by step. When you learn to code, you're essentially learning a new language to communicate with machines.
C++ is a powerful programming language that can help kids unlock the potential of technology. By learning C++, children enhance their problem-solving skills, logical thinking, and creativity—all valuable traits in today’s technology-driven world.
Setting Up Your Environment
To begin learning C++, you'll need to set up a programming environment. Here's how:
Installing a C++ Compiler
-
Choose an IDE: Download an integrated development environment (IDE) like Code::Blocks or Visual Studio.
-
Installation Steps: Follow the instructions specific to the IDE you choose. Each environment will usually guide you through the setup process.
Getting Started with a Simple Program
Once you've set everything up, let's write your very first C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code:
- `#include <iostream>` includes the input-output stream library, which is necessary for displaying text.
- `int main()` is the starting point of every C++ program.
- `std::cout` is used to print text to the console, and `std::endl` adds a new line.
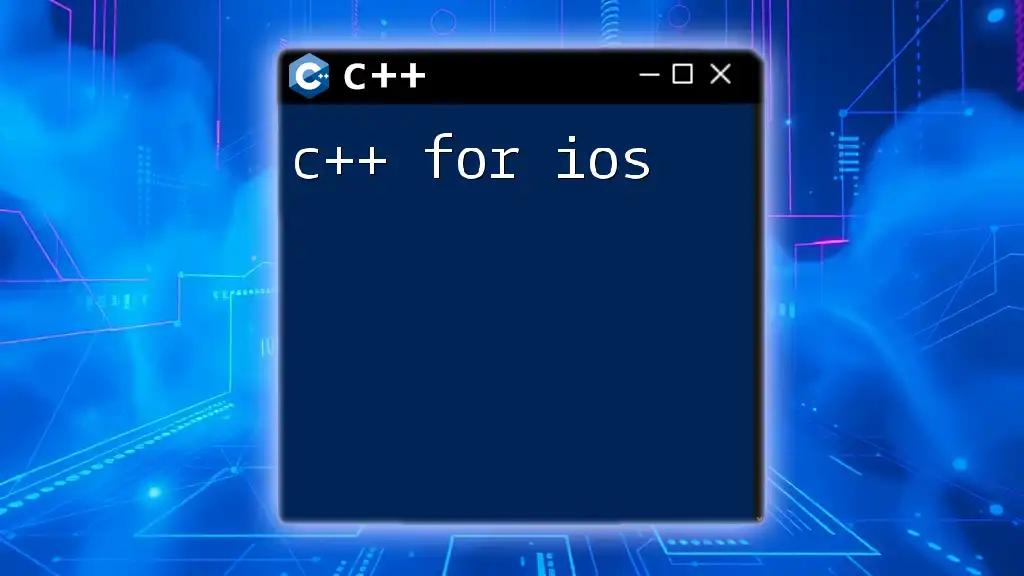
Core Concepts of C++
Variables and Data Types
What are Variables?
Variables act like containers that hold data. Imagine you have a box labeled ‘Fruit’, and inside you can store different types of fruit. Similarly, a variable can hold different types of data depending on its type.
Common Data Types in C++
C++ has several basic data types. Here are a few common ones:
- int: Used to store whole numbers (e.g., 5, -3).
- float: Used for numbers with decimals (e.g., 5.5, -2.3).
- char: Used for single characters (e.g., 'A', 'b').
- string: Used for text (e.g., "Hello").
Here's a quick overview with examples:
int age = 10;
float height = 1.5;
char grade = 'A';
std::string name = "Alice";
Control Structures
Conditional Statements
Understanding If Statements
Conditional statements allow your program to make decisions. Think of it like determining what you want to wear based on the weather—if it’s raining, grab an umbrella!
Here's an example of an if statement:
if (age >= 13) {
std::cout << "You are a teenager!" << std::endl;
} else {
std::cout << "You are a child!" << std::endl;
}
In this case:
- If the variable `age` is 13 or older, the program tells you that you are a teenager.
- If not, it indicates that you are a child.
Loops
Why Do We Use Loops?
Loops are incredibly useful when you want to repeat a certain task multiple times, like counting or creating patterns.
Different Types of Loops include While Loops and For Loops. Let’s look at the For Loop:
for (int i = 1; i <= 5; i++) {
std::cout << "This is loop number " << i << std::endl;
}
In the code above, the program will print statements for each number from 1 to 5.
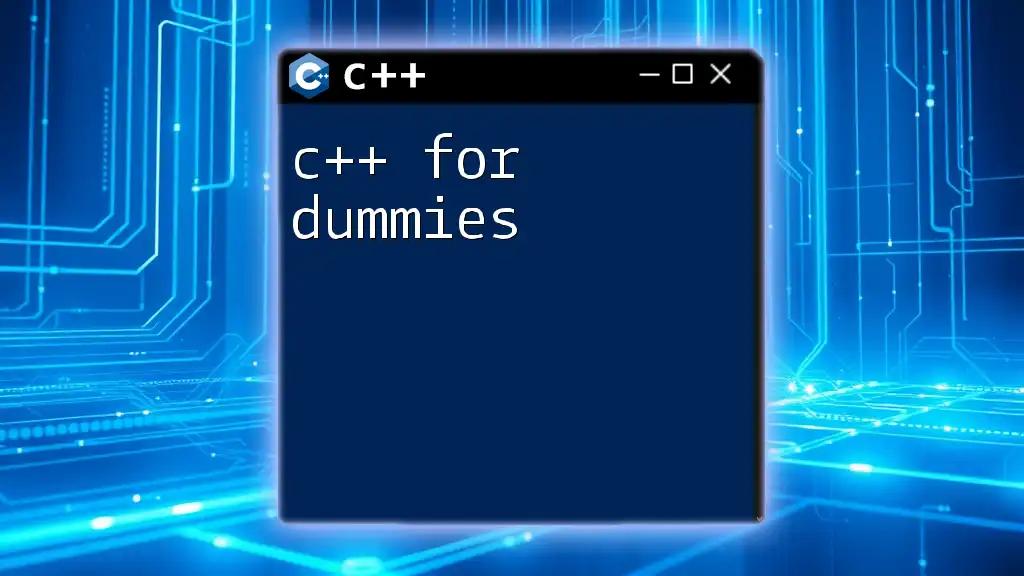
Functions in C++
Understanding Functions
What is a Function?
A function is like a recipe. Just as you follow a recipe to create a dish, you can call a function to perform a specific task in a program. Once defined, you can use this function repeatedly without rewriting the code.
How to Declare and Define a Function
Here’s how you can create a simple greet function:
void greet() {
std::cout << "Welcome to C++!" << std::endl;
}
int main() {
greet(); // Call the greet function
return 0;
}
Scope of Variables
Understanding the difference between Local and Global Variables is crucial.
- Local Variables are declared inside a function and cannot be accessed outside of it.
- Global Variables are declared outside any function and can be used throughout the program.
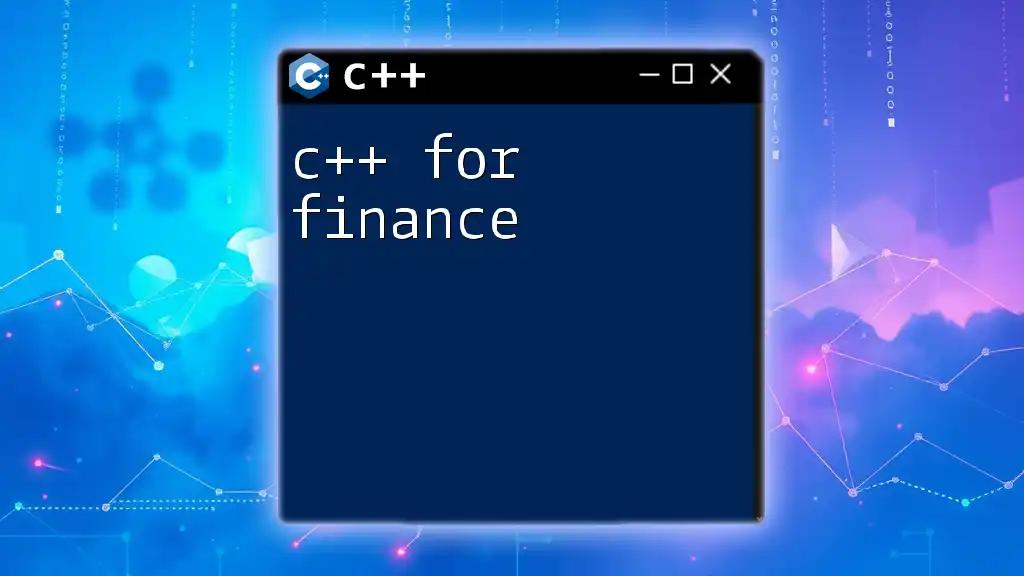
Object-Oriented Programming (OOP) Basics
Introduction to OOP
What is Object-Oriented Programming?
OOP allows you to model real-world things using code. Think of it as arranging your toys in a box, where each toy represents an object with specific properties and functions.
Creating a Simple Class
To understand classes, consider this simple example of an `Animal` class:
class Animal {
public:
void speak() {
std::cout << "Roar!" << std::endl;
}
};
int main() {
Animal lion; // Create an object of class Animal
lion.speak(); // Call the speak method
return 0;
}
In this code, the class `Animal` has a method `speak`. When you create an instance of `Animal` called `lion`, you can call its `speak` method.
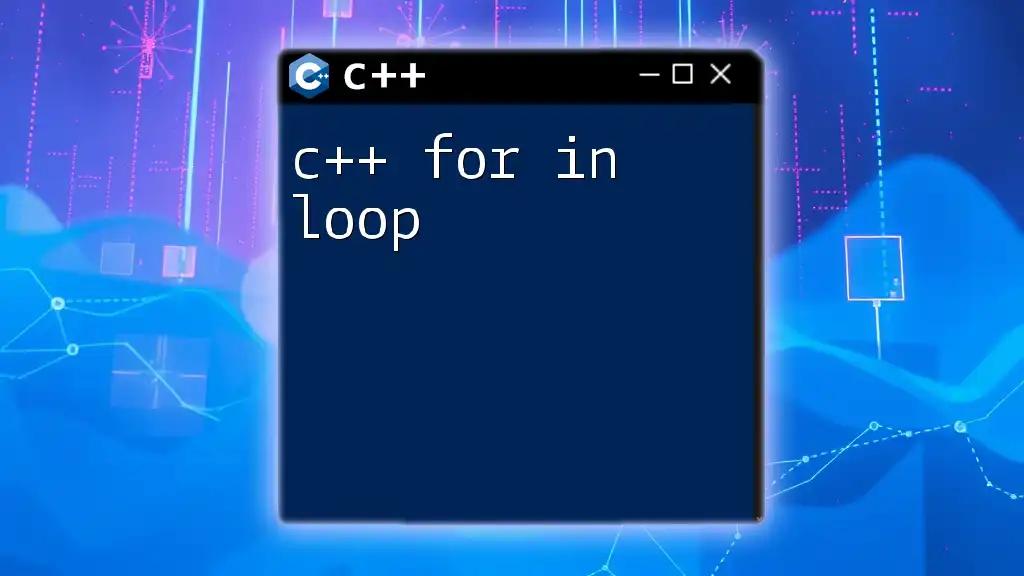
Practical C++ Projects
Creating a Simple Game
Now that you have learned the basics, let’s create a simple text adventure game. It can be structured with if-else statements to guide the player through choices.
int main() {
std::cout << "You're at a crossroad. Do you want to go left or right?" << std::endl;
// More game logic can follow here
}
Your game can become as complex as you want, adding more choices and scenarios!
Building a Math Quiz Application
Designing a quiz application can be a fun, interactive way to practice your coding skills. Here’s a simple code snippet to get you started:
int main() {
int answer;
std::cout << "What is 5 + 3? ";
std::cin >> answer; // Takes user input
if (answer == 8) {
std::cout << "Correct!" << std::endl;
} else {
std::cout << "Try again!" << std::endl;
}
}
In this quiz, the program prompts for a math question, evaluates the answer, and provides feedback.
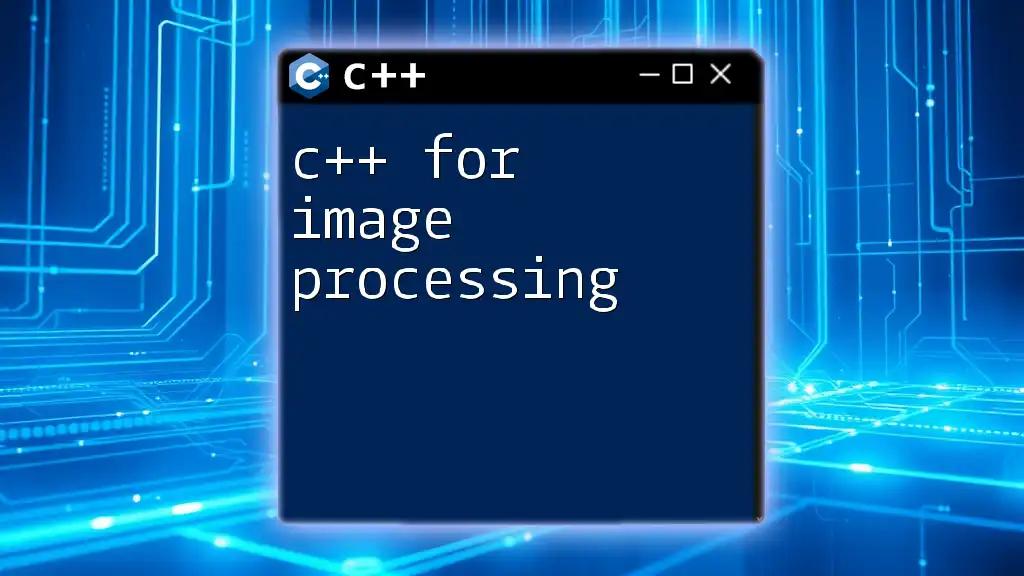
Conclusion
Learning C++ for Kids can be an exciting adventure that helps develop essential thinking skills. It's a foundation that opens up numerous opportunities in technology and beyond. Remember, practice is key! Keep experimenting with coding, and don’t hesitate to tackle more complex projects as you grow confident in your C++ skills.
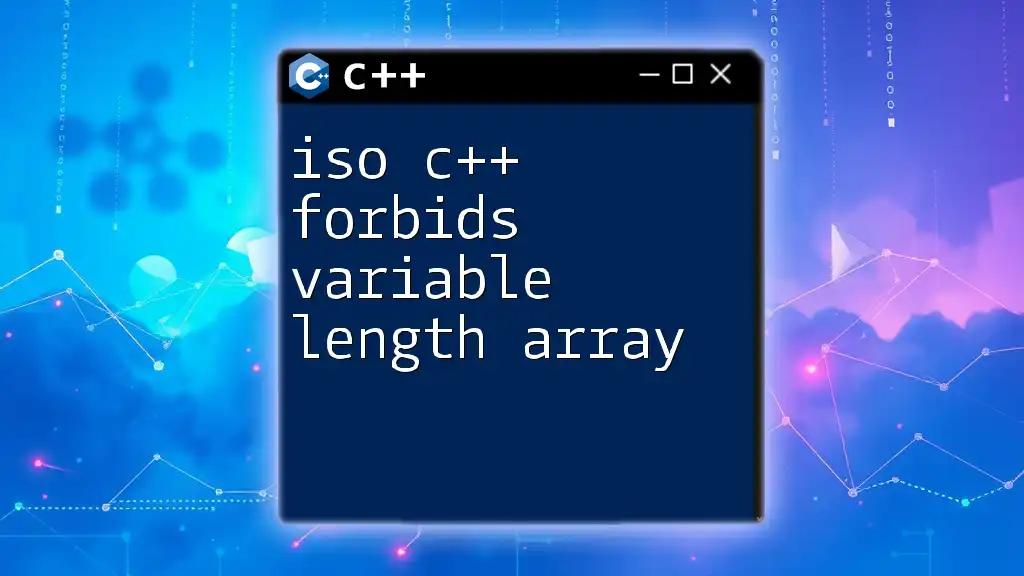
Resources for Further Learning
To ensure you continue your journey in coding, consider exploring additional resources:
- Look for books specifically aimed at kids learning C++.
- Explore online courses and interactive platforms to practice coding.
- Engage with coding forums and community groups to find peers and mentors.
- Participate in coding challenges tailored for younger audiences.
By nurturing your programming skills, you’re taking valuable steps towards a bright future in the digital world. Happy coding!