In ISO C++, variable length arrays (VLAs) are not allowed, meaning that you cannot declare an array with a size that is determined at runtime; instead, you can use dynamic memory allocation via pointers.
Here's a code snippet demonstrating the use of dynamic memory allocation:
#include <iostream>
int main() {
int size;
std::cout << "Enter the size of the array: ";
std::cin >> size;
// Using dynamic memory allocation instead of a variable length array
int* array = new int[size];
// Example usage
for (int i = 0; i < size; ++i) {
array[i] = i;
std::cout << array[i] << " ";
}
// Freeing the allocated memory
delete[] array;
return 0;
}
Understanding Variable Length Arrays (VLAs)
Variable Length Arrays (VLAs) are a feature primarily associated with the C programming language. They allow you to create arrays whose size is determined at runtime, rather than at compile time. For instance:
int n;
scanf("%d", &n);
int arr[n]; // Size of the array is determined by user input
This flexibility can be convenient but comes with drawbacks, particularly in the context of memory management and program efficiency.
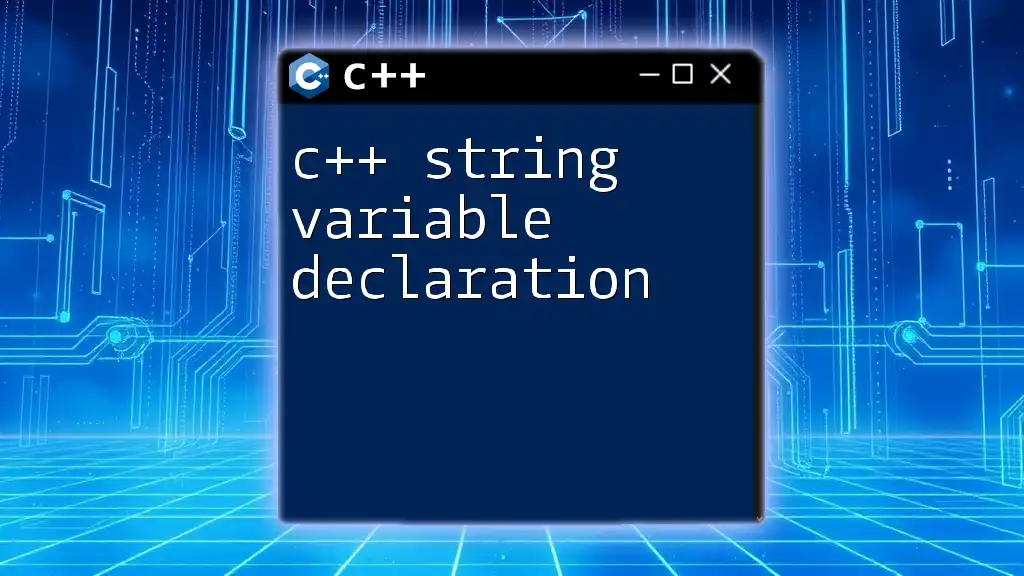
The Evolution of C++ Standards
C++ has evolved significantly from its inception. Understanding this evolution is essential, especially when discussing why ISO C++ forbids variable length arrays.
The major iterations of C++ standards include:
- C++98: This was the first standardized version.
- C++03: A bug-fix release with no new features.
- C++11: Introduced notable features like `auto`, `std::unique_ptr`, and `nullptr`.
- C++14/C++17: Added enhancements, but VLAs were not included.
These changes reflect ongoing efforts to enhance performance, safety, and usability in C++.
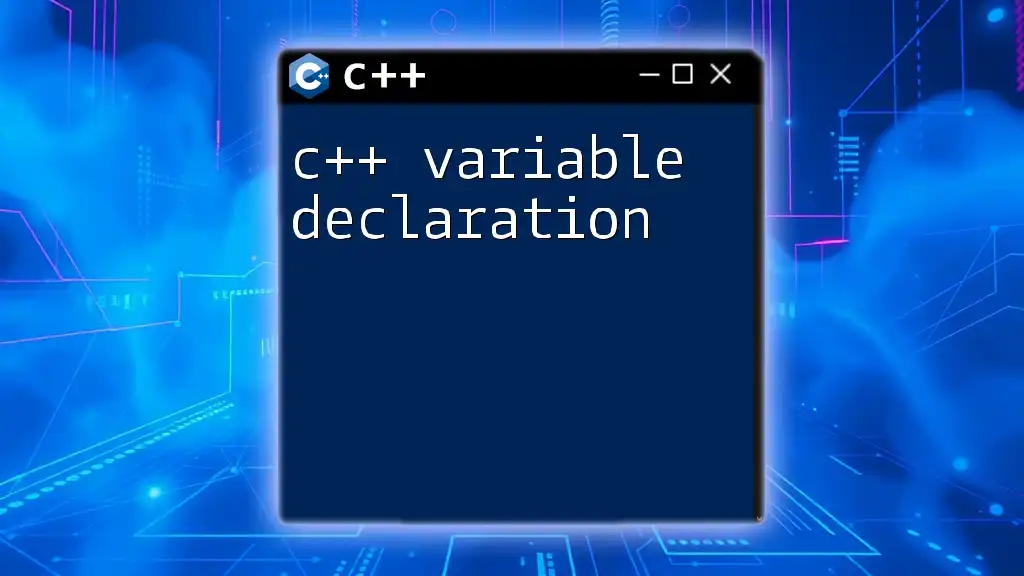
ISO C++ and Its Restrictions
When discussing the ISO C++ forbids variable length arrays, it is crucial to understand the rationale behind this decision.
Why Variable Length Arrays are Forbidden
The primary reason is rooted in memory allocation and efficiency. VLAs can lead to:
- Unpredictable Memory Usage: Since VLAs allocate memory on demand, they can cause stack overflow, which is a risk for large arrays.
- Undefined Behavior: If a VLA is declared in a scope where the variable defining its size goes out of scope, it can lead to unpredictable program behavior.
In contrast, fixed-size arrays, as shown below, have a constant size known at compile time, leading to safer and more efficient memory usage:
int arr[10]; // Fixed-size array, size known at compile time
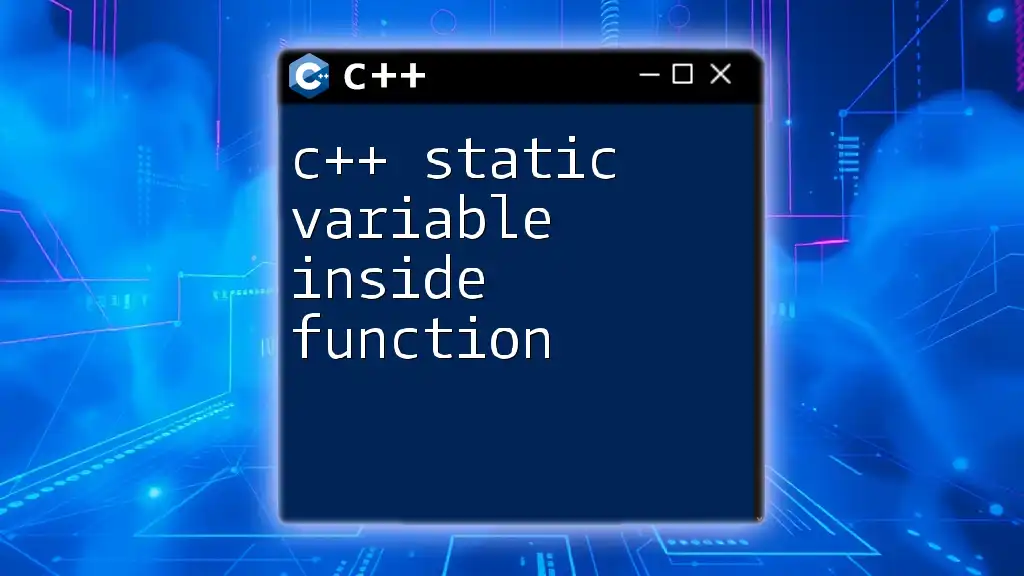
Exploring Variable Length Arrays in C
The context of VLAs is primarily within C programming. Here's what makes them stand out:
Understanding VLAs in C Programming
In C, VLAs provide flexibility that can be quite useful:
void exampleFunction() {
int n;
printf("Enter size: ");
scanf("%d", &n);
int arr[n]; // VLA example
}
Advantages and Drawbacks of Using VLAs
Advantages:
- Flexibility with array size
- Allowing efficient use of memory based on runtime data.
Drawbacks:
- Potential for memory overflow
- Harder to manage than fixed arrays.
Transitioning to C++
When moving to C++, developers are encouraged to abandon VLAs given the language's emphasis on strong type safety and memory management.
Limitations of C-style VLAs in C++
C++ offers a more robust memory management system, and using VLAs can lead to issues during compilation and execution. Developers face potential bugs and runtime errors that are typically avoided with static arrays.
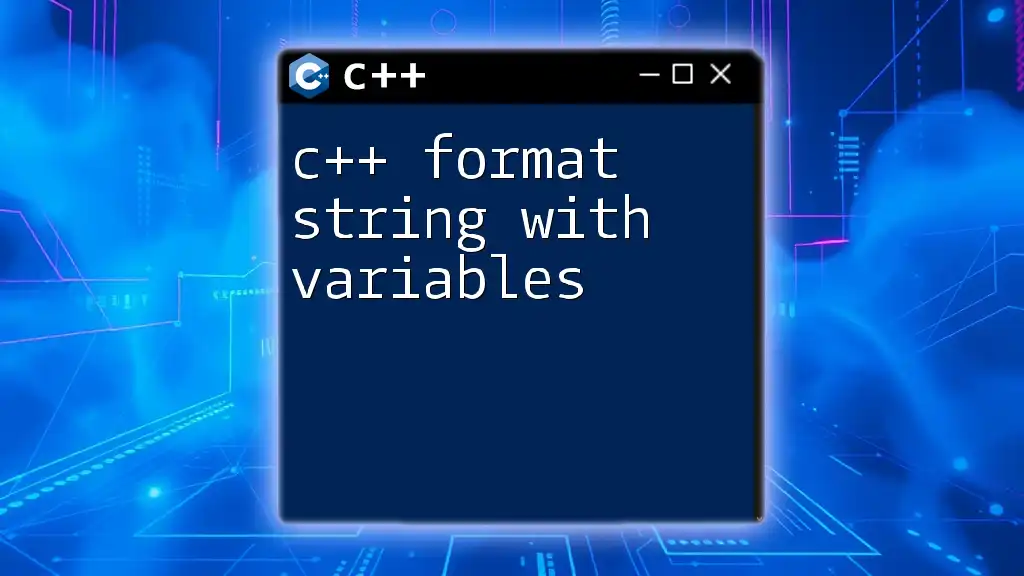
Alternatives to Variable Length Arrays in C++
When VLAs are off the table in C++, what options are available?
Fixed-Size Arrays
Fixed-size arrays are declared with a specified size known at compile time:
int arr[10]; // Fixed-size array
While they lack the flexibility of VLAs, they ensure that memory management remains predictable.
Dynamic Memory Allocation
Dynamic memory allocation through `new` and `delete` allows developers to create arrays whose size is determined at runtime while retaining control over memory management:
int* arr = new int[n]; // Dynamic array
// Use the array
delete[] arr; // Release memory
This method ensures precise control over memory usage, preventing issues associated with stack overflow.
Using `std::vector`
The modern C++ standard library offers containers that make dynamic memory management effortless. `std::vector` adapts its size dynamically. Here’s how you could use it:
#include <vector>
#include <iostream>
void exampleFunction() {
int n;
std::cout << "Enter size: ";
std::cin >> n;
std::vector<int> vec(n); // Vector with size specified at runtime
// Use vec as an array
}
Advantages:
- Automatic memory management
- Resizable array that handles internal memory allocations.
Modern Alternatives
C++ also provides `std::array` and `std::list`, which can serve as alternatives depending on specific use cases:
#include <array>
// Example of std::array
std::array<int, 10> arr; // Size fixed at compile time
These containers instill safer memory usage principles into C++ programs, so developers can maintain efficiency and speed.
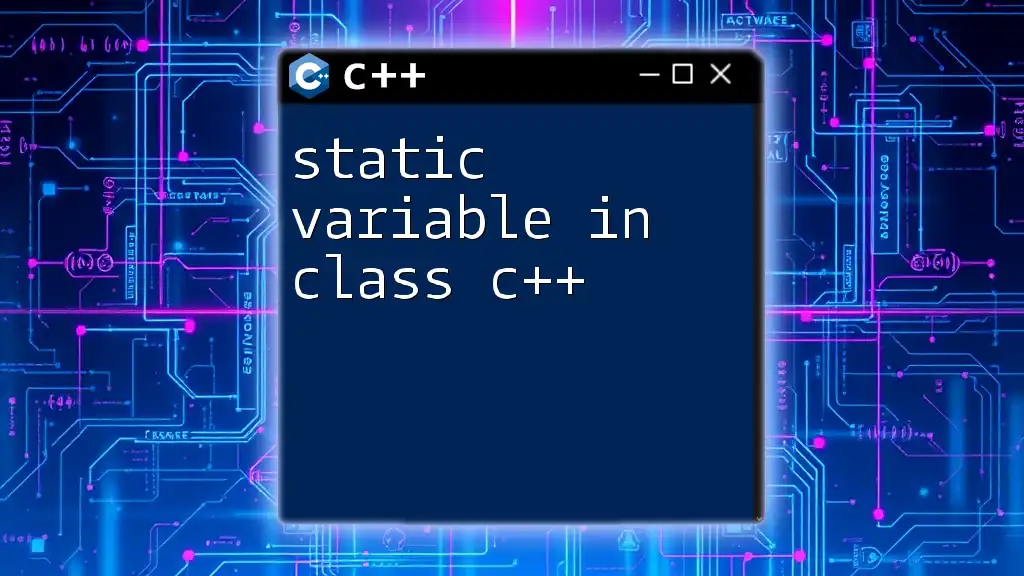
Common Misconceptions
Why Some Developers Prefer VLAs
Despite ISO C++ forbids variable length arrays, there are developers who hesitate to move away from them. The appeal lies in their flexibility and ease of use, particularly in constructing quick algorithms where performance may not initially be a concern.
Clarifying Misunderstandings about ISO C++
One common myth surrounding VLAs is the belief that they offer significant performance improvements over standard array types. In reality, adhering to C++ standards enhances maintainability and performance for larger, complex applications.
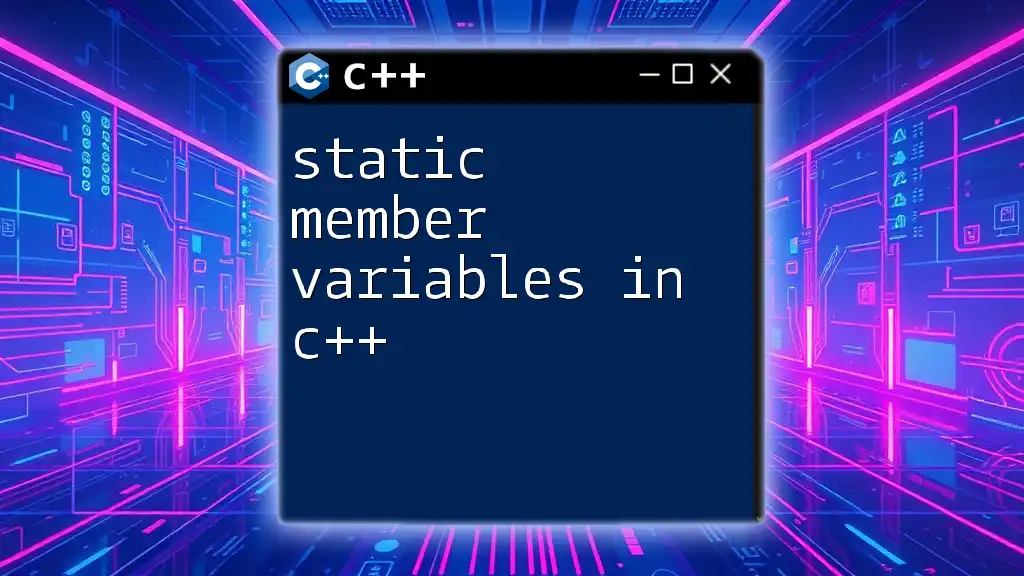
Conclusion
In summary, understanding why ISO C++ forbids variable length arrays is essential for any C++ developer. VLAs introduce potential pitfalls in memory management and code maintainability. Instead, developers are encouraged to embrace fixed-size arrays, dynamic memory allocation, and STL containers like `std::vector`, which provide safer and more effective solutions.
Ultimately, aligning practices with modern C++ standards ensures better performance, safety, and maintainability. By moving away from VLAs, developers can focus on enhancing their code quality and embracing the rich features C++ offers.