A static member variable in C++ is shared among all instances of a class, meaning there's only one copy of that variable for the class rather than for each object.
#include <iostream>
using namespace std;
class MyClass {
public:
static int staticVar;
void increment() {
staticVar++;
}
};
int MyClass::staticVar = 0;
int main() {
MyClass obj1, obj2;
obj1.increment();
obj2.increment();
cout << "Static variable value: " << MyClass::staticVar << endl; // Outputs: 2
return 0;
}
The Basics of Static Member Variables
What is a Static Member Variable?
A static member variable in C++ is a class-level variable that has the same value for all instances of the class. Unlike regular member variables, which are tied to individual objects, static member variables belong to the class itself. This means they are shared among all instances, providing a way to maintain a single shared state across different objects.
How Static Member Variables are Shared
Static member variables allow you to share data among all instances of a class. For example, if you want to keep track of how many objects of a class have been created, you can use a static member variable to count the instances.
Consider the following code snippet:
class MyClass {
public:
static int instanceCount;
MyClass() {
instanceCount++;
}
};
int MyClass::instanceCount = 0;
In this example, every time a new `MyClass` object is created, the `instanceCount` is incremented. Thus, even if you have multiple instances of `MyClass`, they will all share the same `instanceCount` variable, which reflects the total number of instances created.
Memory Allocation for Static Member Variables
The memory for static member variables is allocated when the class is loaded, and since they are shared across instances, they exist for the lifetime of the program. This is different from instance variables, which are created and destroyed with each object.
To visualize this, consider the following code example:
#include <iostream>
class MyClass {
public:
static int count;
MyClass() {
count++;
}
};
int MyClass::count = 0;
int main() {
MyClass obj1;
MyClass obj2;
std::cout << "Number of instances: " << MyClass::count << std::endl; // Output: 2
return 0;
}
Here, `count` is initialized to `0`, and every time an object of `MyClass` is created, the static member variable reflects the total number of created instances.
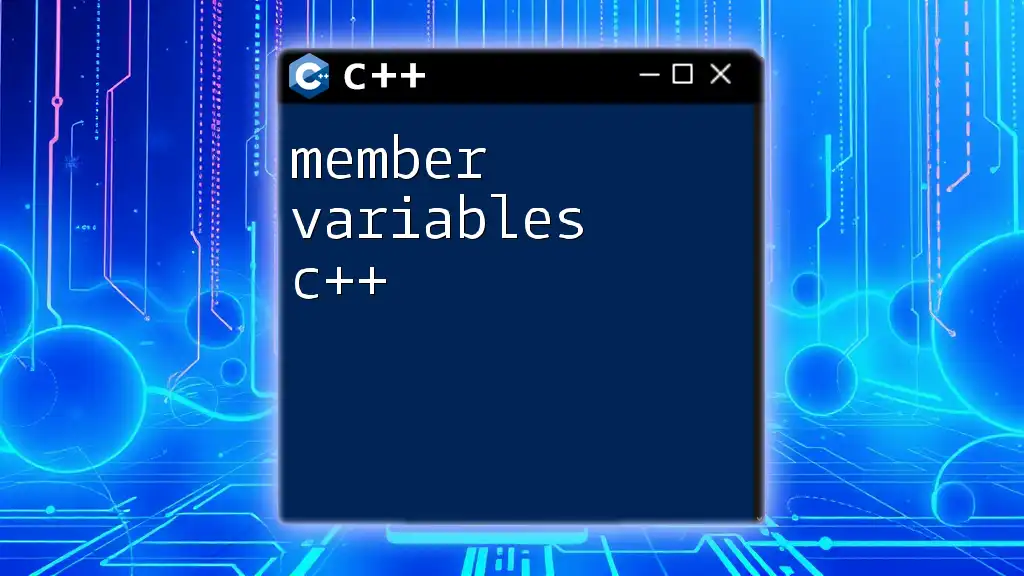
Defining and Accessing Static Member Variables
Syntax for Declaring Static Member Variables
To declare a static member variable, you need to specify the `static` keyword within the class definition. However, the actual definition must be provided outside of the class, typically in a `.cpp` file. Here’s how you can do this:
class Example {
public:
static int value; // Declaration
};
// Definition (usually in a .cpp file)
int Example::value = 0; // Initialization
Accessing Static Member Variables
Access via Class Name
Static member variables are generally accessed using the class name, which improves code clarity and communicates that the variable belongs to the class, not to any individual object.
For instance:
std::cout << Example::value << std::endl; // Accessing static member variable
Access via Object Instances
While you can access static member variables using an object instance, it is not the recommended way. This can mislead being interpreted as the variable being tied to the instance. Here is an example:
Example obj;
obj.value = 5; // Not recommended but valid
std::cout << Example::value << std::endl; // Output: 5
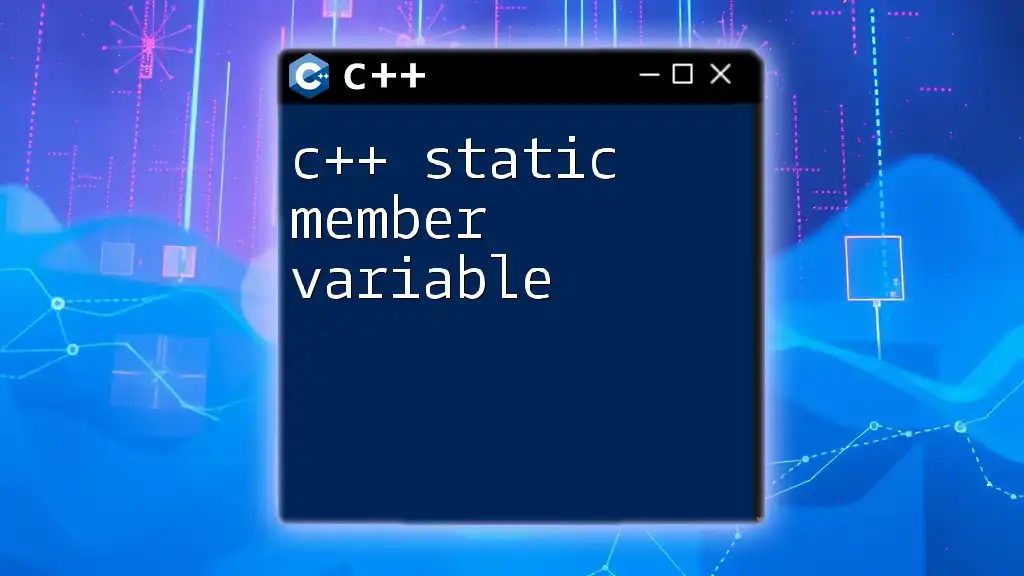
Static Member Function and Its Relationship with Static Member Variables
What are Static Member Functions?
A static member function is a function that can be called on the class itself, rather than on instances of the class. This function can only access static member variables and cannot access non-static members directly.
Accessing Static Member Variables in Static Member Functions
You can manipulate static member variables directly through static member functions, which also reinforces the concept of shared state. Here’s an example:
class Counter {
public:
static int count;
static void increment() {
count++;
}
};
int Counter::count = 0;
int main() {
Counter::increment();
Counter::increment();
std::cout << "Count: " << Counter::count << std::endl; // Output: 2
return 0;
}
In this example, `increment` is a static member function that modifies the shared static variable `count`. This illustrates the relationship between static member functions and static member variables effectively.
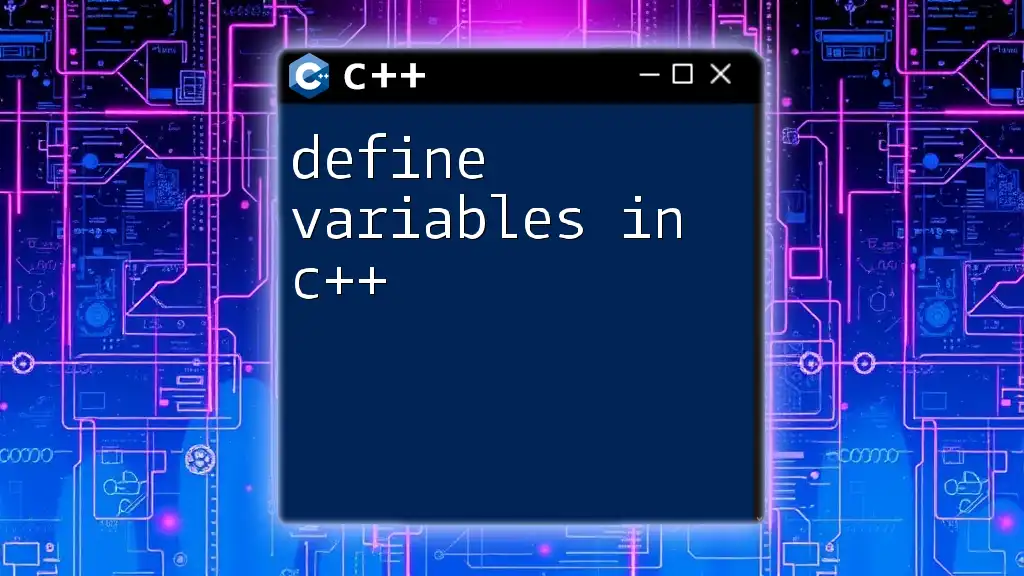
Use Cases for Static Member Variables
Single Instance of a Variable for All Objects
One common use case for static member variables is when you need a single instance of a variable for all objects. This can help maintain global state or settings across all instances.
Implementing Counter for Class Instances
Static member variables can also be useful for tracking the number of instances of a class. For example, in a game, you might want to keep track of how many enemies have been created. Using a static member variable to maintain this count can simplify your management of game state.
class Enemy {
public:
static int enemyCount;
Enemy() {
enemyCount++;
}
};
int Enemy::enemyCount = 0;
int main() {
Enemy e1;
Enemy e2;
std::cout << "Total enemies: " << Enemy::enemyCount << std::endl; // Output: 2
return 0;
}
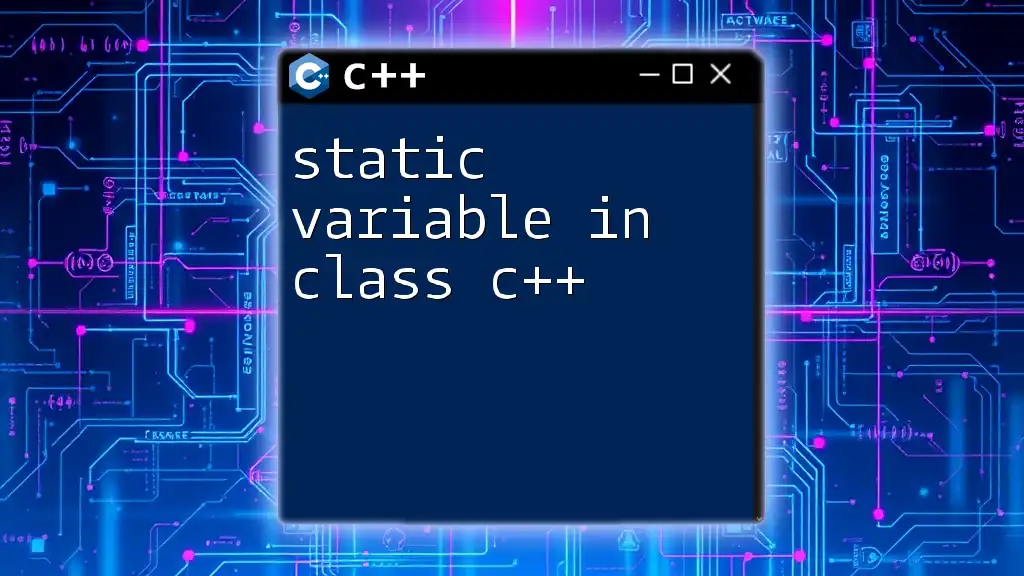
Best Practices for Using Static Member Variables
When to Use Static Member Variables
Use static member variables judiciously—only when you genuinely need to share information across all instances of a class. They can provide efficiency and save memory if used properly. However, overusing static variables can lead to code that is difficult to maintain and debug due to shared state.
Alternative Approaches to Consider
In certain scenarios, you might choose instance variables or other design patterns like the Singleton pattern if the goal is to control the instantiation of a class while maintaining global access. Always consider the implications of using static member variables on the design and structure of your application.
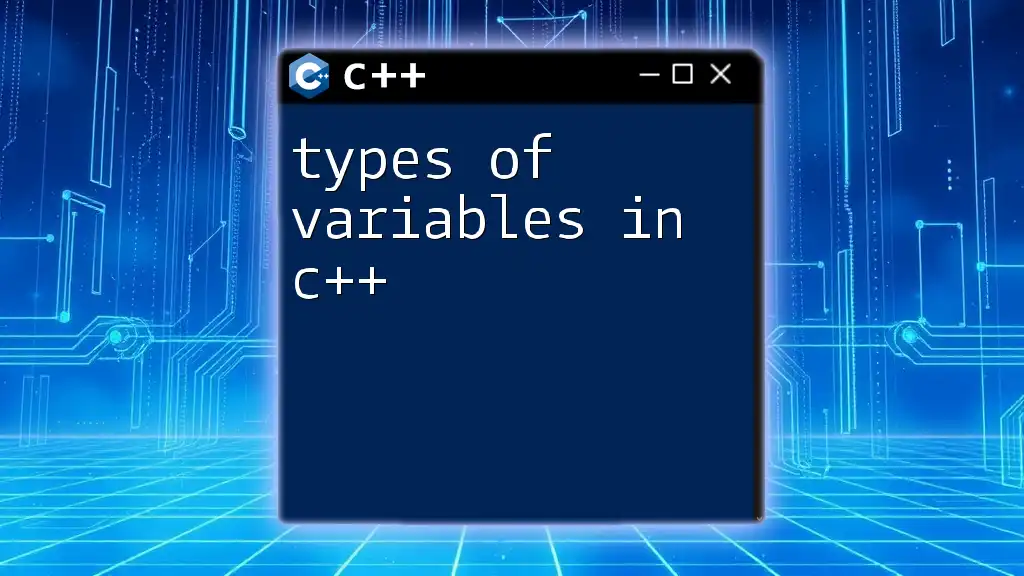
Conclusion
Understanding static member variables in C++ is critical for managing shared state across class instances efficiently. They provide a powerful way to track information that is global to a class while maintaining a clear structure within the code. Properly utilizing static member variables can lead to better code organization and streamlined functionalities. Remember to weigh the pros and cons before deciding to use them in your classes.