The `string` header file in C++ provides a versatile and powerful way to work with strings through the `std::string` class, enabling dynamic string manipulation and memory management.
Here's a simple example of how to include the `string` header and use the `std::string` class:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
What is the C++ String Header?
The string header file in C++, `<string>`, is a crucial component of the C++ Standard Library that provides powerful tools for handling text-based data. The string class encapsulates a sequence of characters and offers an intuitive way to manage string-related operations, significantly simplifying what was traditionally a cumbersome process with C-style strings. Unlike C-style strings, which are arrays of characters terminated by a null character, the C++ string class provides features and functionality that allow for safer and more efficient string manipulation.
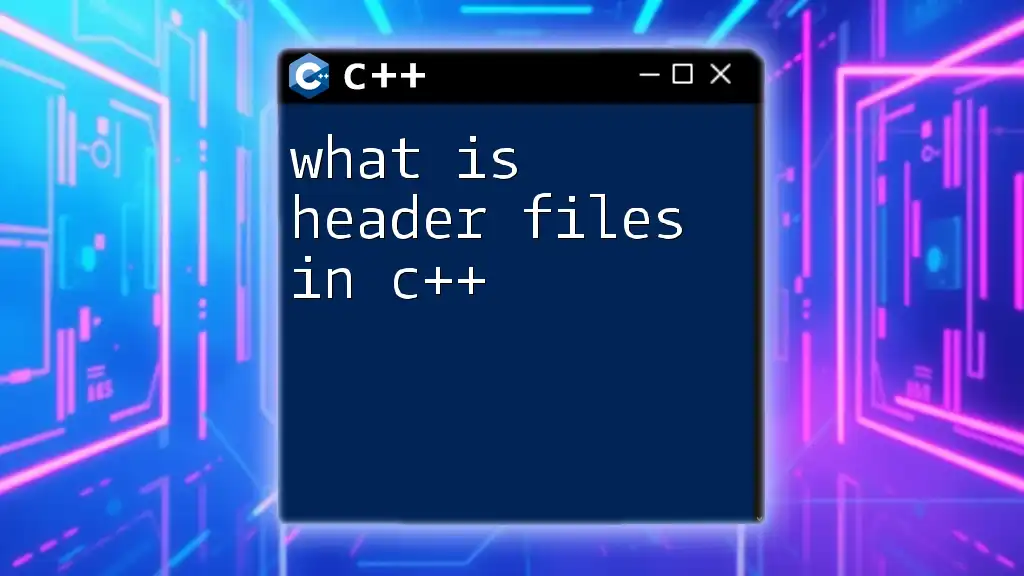
Including the String Header in C++
To utilize the string class and its features, you must include the string header in your program. This can be done with the following syntax:
#include <string>
This simple line makes all the functionalities of the string class available in your program. Here's a brief example demonstrating the inclusion and use of strings:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
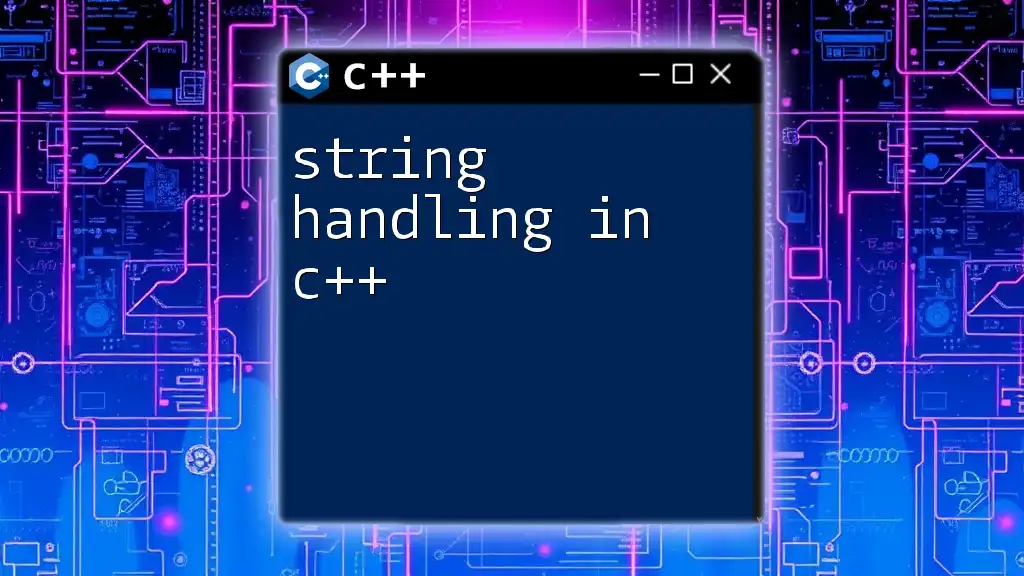
Creating and Initializing Strings
Creating and initializing strings in C++ is straightforward. You can declare string variables in several ways:
- Default Initialization: This will create an empty string.
std::string myString; // Default initialization
- Initialization with a C-string: You can initialize a string directly from a C-style string (null-terminated character array).
std::string anotherString = "Hello"; // Initialized with a C-string
Additionally, the string class provides constructors that allow for more advanced initialization. For instance, you can create a string containing a specified number of repeated characters:
std::string str1(10, 'A'); // Creates a string of length 10 with 'A'
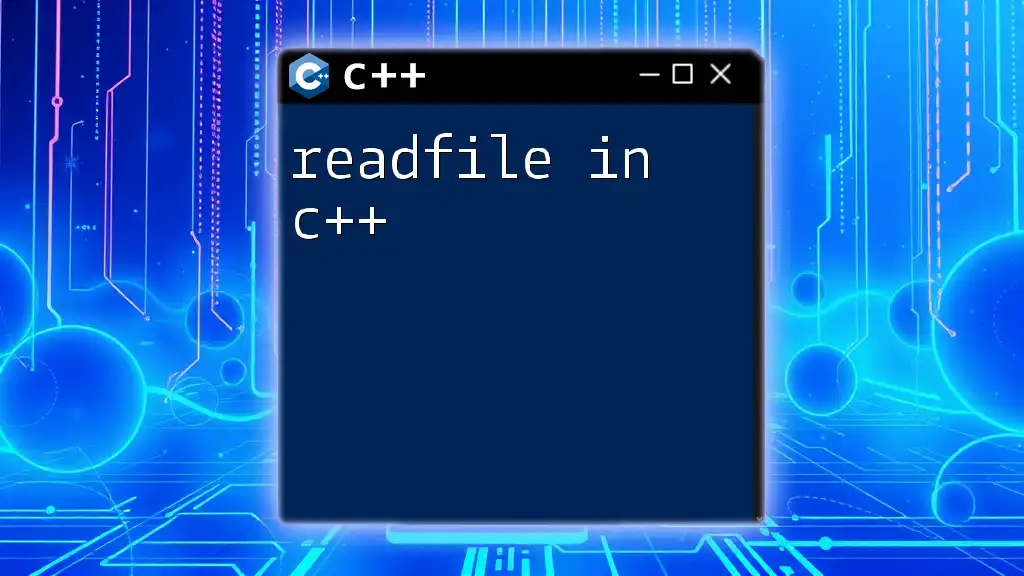
Basic String Operations
Understanding basic string operations is essential for effective string manipulation in your programs. Let's explore some common operations.
Concatenation of Strings
String concatenation is a common operation that combines two or more strings into one. In C++, you can achieve this using the `+` operator or the `+=` operator.
std::string firstName = "John";
std::string lastName = "Doe";
std::string fullName = firstName + " " + lastName; // Concatenation
Accessing String Characters
The string class allows you to access individual characters using the `[]` operator and the `at()` method, which provides bounds checking.
char firstChar = fullName[0]; // Accessing the first character
char secondChar = fullName.at(1); // Accessing the second character (with bounds checking)
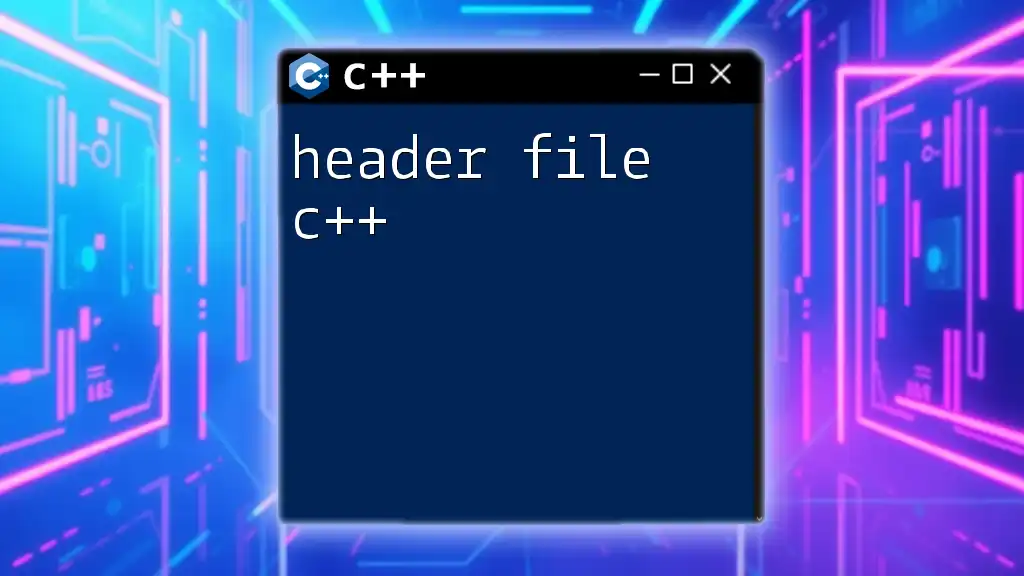
Common String Functions
The C++ string class offers an array of functions that make string manipulation easy and intuitive.
Length and Size of a String
To find the length or size of a string, you can use the `length()` or `size()` methods. Both methods yield the same result as they are interchangeable in this context.
std::cout << "Length of fullName: " << fullName.length() << std::endl; // Outputs 8 for "John Doe"
Substring Extraction
The `substr()` function allows you to extract a substring from a string, specified by a starting index and a desired length.
std::string sub = fullName.substr(0, 4); // Extracts "John"
Finding and Replacing
You can locate substrings using the `find()` method and replace portions of strings with the `replace()` method. Note that `find()` returns the index of the first occurrence, or `std::string::npos` if not found.
std::size_t pos = fullName.find("Doe");
if (pos != std::string::npos) {
fullName.replace(pos, 3, "Smith"); // Replaces "Doe" with "Smith"
}
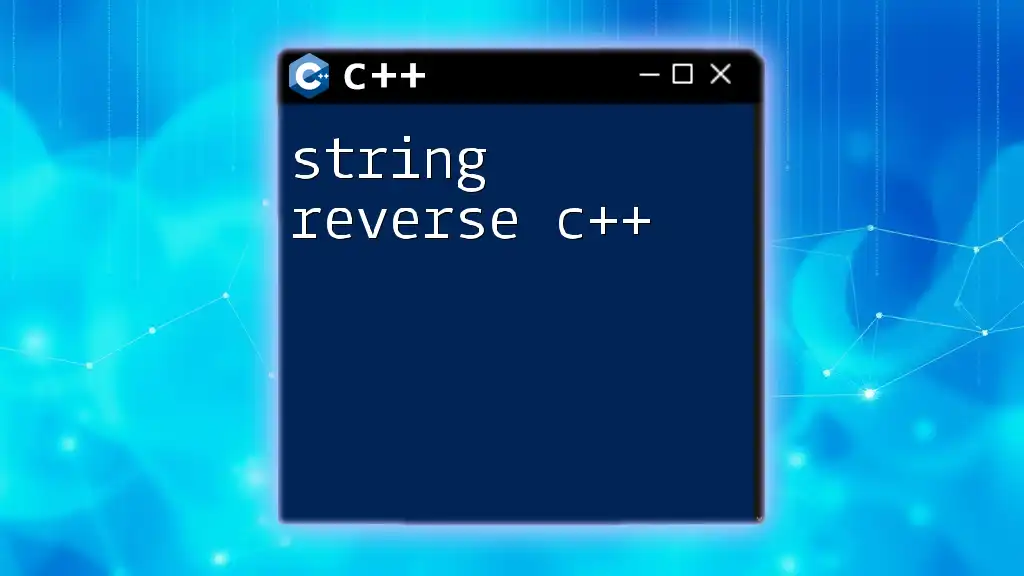
Input and Output with Strings
Strings are inherently useful for handling user input and output. You can easily read and display string data using standard input and output streams.
Using Strings with Input/Output Streams
To read and output strings, make use of `std::cin` and `std::cout`. For example:
std::string userInput;
std::cout << "Enter your name: ";
std::cin >> userInput; // Reads a string from user input
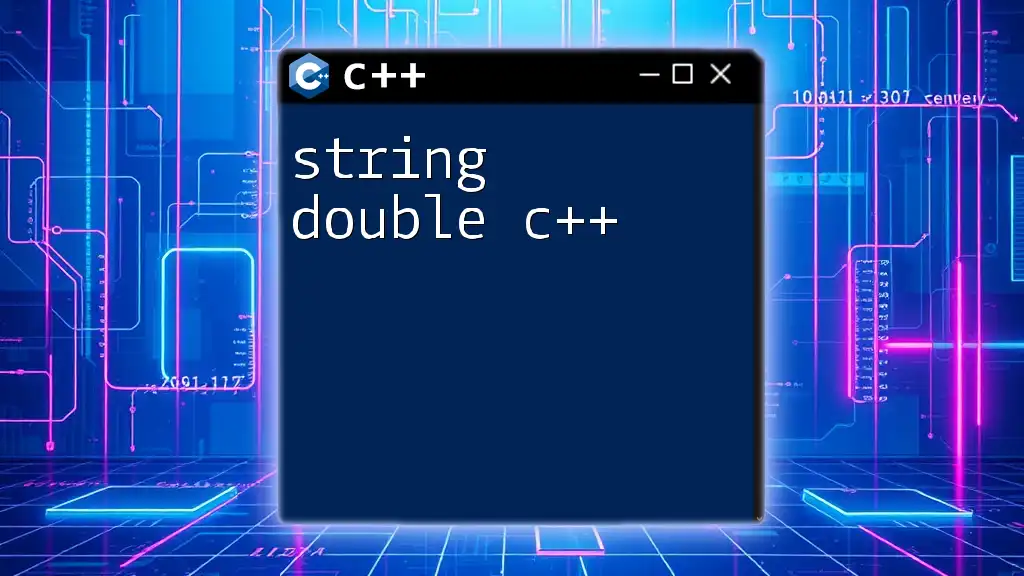
String Comparison
Comparing strings is straightforward in C++. C++ provides operators such as `==`, `!=`, `<`, `>`, etc., that work comparing strings lexicographically, just like comparing standard data types.
if (firstName == lastName) {
std::cout << "Same names";
} else {
std::cout << "Different names";
}
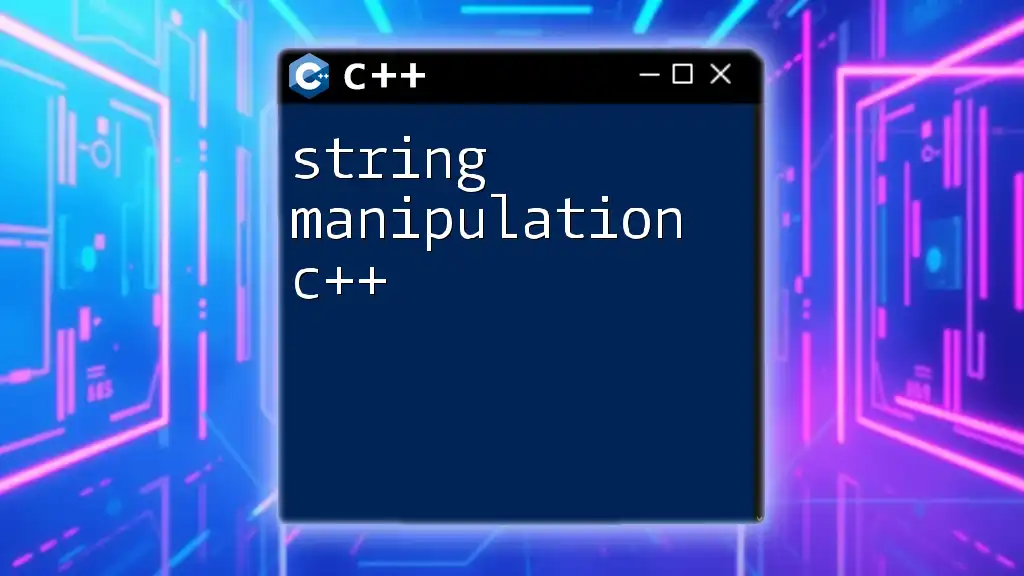
Advanced String Manipulations
For more advanced string manipulations, the string class provides iterators and string streams.
String Iterators
Iterators allow you to traverse through the characters in a string. This can be more flexible than using indexing.
for (std::string::iterator it = fullName.begin(); it != fullName.end(); ++it) {
std::cout << *it << " "; // Prints each character in fullName
}
String Stream (sstream)
For complex input and output operations involving strings, C++ offers the `sstream` library, which is useful for mixing strings with other streams.
#include <sstream>
std::stringstream ss;
ss << "Hello " << userInput; // Combining strings
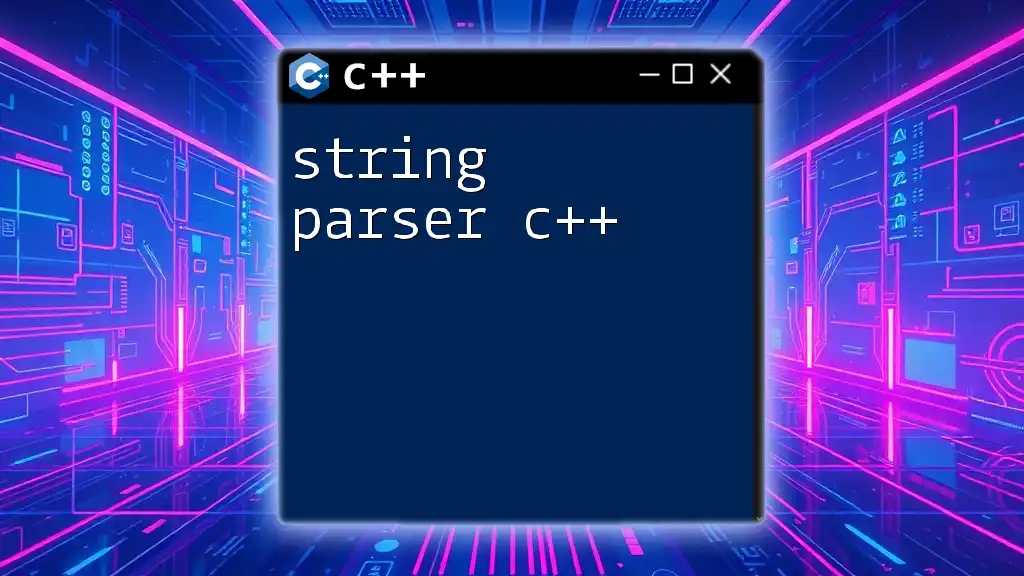
Conclusion
The string header file in C++ is an indispensable tool for any programmer working with text. It provides a wealth of functionality that simplifies the process of string manipulation, making it both efficient and intuitive. By mastering these string operations and functions, you can handle complex string tasks with ease. Practice the examples provided in this guide, and consider exploring more advanced topics associated with string manipulation to deepen your understanding of this fundamental C++ feature.
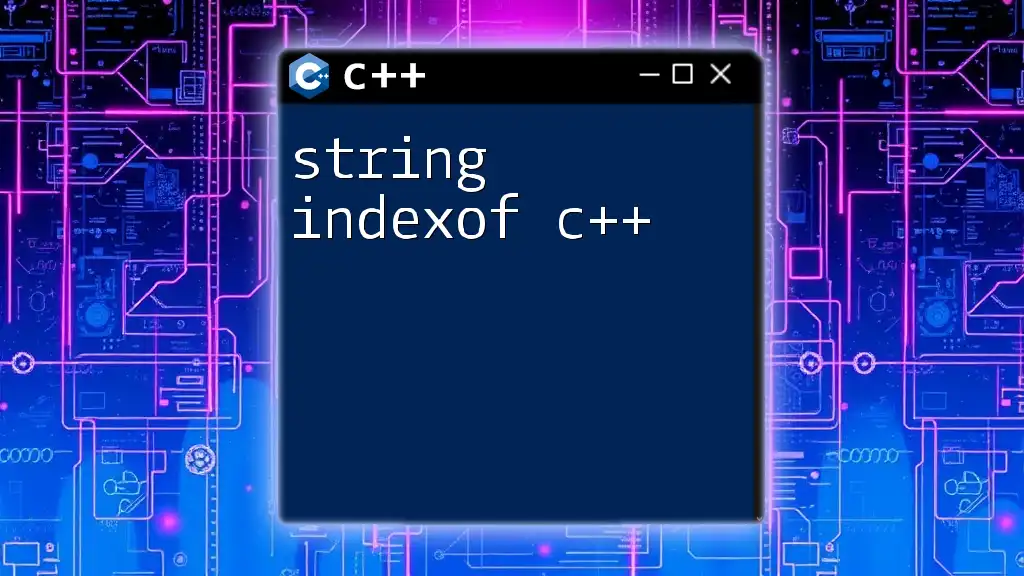
Resources for Further Reading
For additional knowledge beyond this guide, consider exploring recommended books, articles, and online resources that delve into C++ strings and the broader C++ Standard Library.