Header files in C++ are used to declare functions, classes, and variables that can be shared across multiple source files, helping to organize code and promote reusability.
Here’s an example of a simple header file and its usage:
// example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
void sayHello();
#endif // EXAMPLE_H
// example.cpp
#include <iostream>
#include "example.h"
void sayHello() {
std::cout << "Hello, World!" << std::endl;
}
// main.cpp
#include "example.h"
int main() {
sayHello();
return 0;
}
What is a Header File?
A header file in C++ is essentially a file containing declarations and definitions that can be shared across multiple program files. These files usually have the `.h` extension and are crucial for organizing code, promoting reusability, and separating interface from implementation.
The purpose of header files is to allow programmers to declare functions, classes, and variables that can be defined later in corresponding `.cpp` source files. This helps in keeping the code modular and manageable, allowing different teams or individuals to work on different parts of a program simultaneously without conflict.
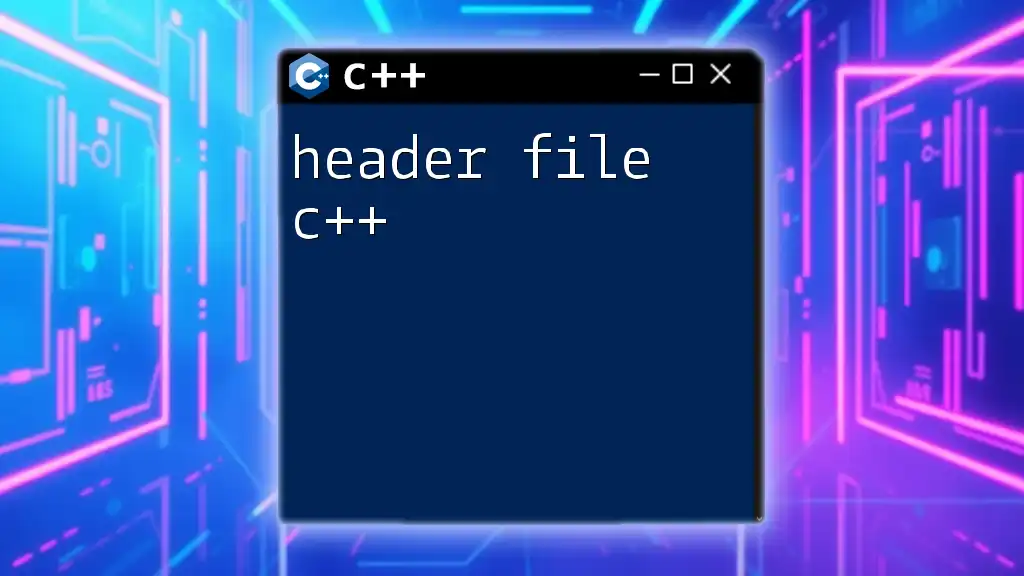
Types of Header Files in C++
C++ offers a variety of header files:
-
Standard Header Files: These come with the C++ standard library and include common utilities and functionalities. Examples are `<iostream>` for input and output operations and `<vector>` for handling dynamic arrays.
-
User-defined Header Files: These are custom header files created by programmers to encapsulate specific functionalities related to their programs. They allow programmers to define their own functions, classes, and constants that can be reused in different files.
-
External Header Files: These refer to headers that are part of third-party libraries or frameworks, enabling the use of additional functionalities developed by others.
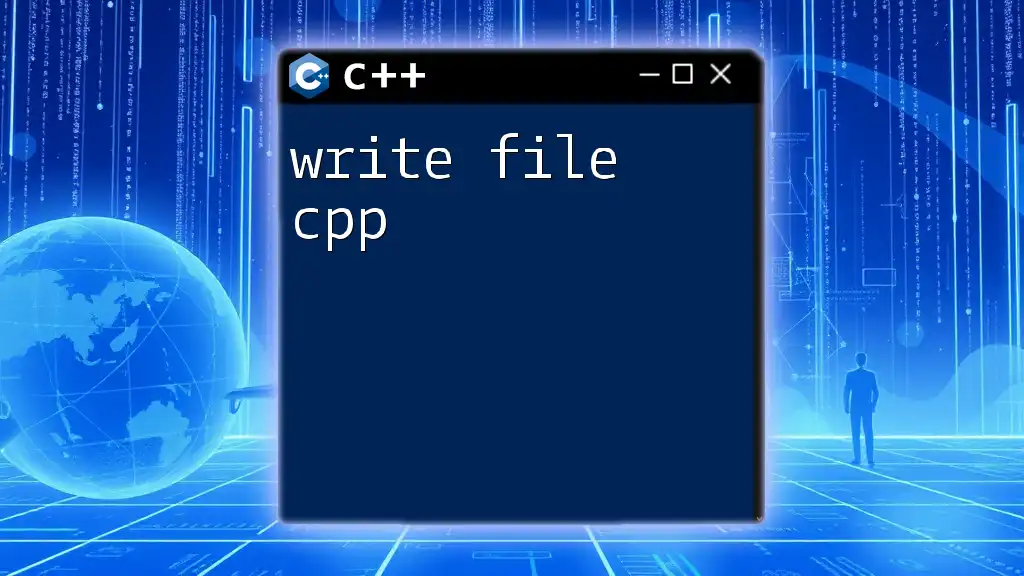
Structure of a C++ Header File
Basic Components of a Header File
A typical C++ header file consists of:
-
Preprocessor Directives: These include statements that tell the compiler which files to include before compilation starts. The `#include` directive is commonly used to include both standard and user-defined headers.
-
Function Declarations: Each function's prototype is declared within the header file, allowing its definition to reside in a `.cpp` file.
-
Class Definitions: For object-oriented programming, header files serve to define the structure of classes - their attributes and methods.
Example Header File in C++
Here is a basic example of how a header file can be structured:
#ifndef MYHEADER_H
#define MYHEADER_H
// Function declaration
void myFunction();
// Class definition
class MyClass {
public:
void display();
};
#endif // MYHEADER_H
In this example, the header guard (encapsulated within `#ifndef`, `#define`, and `#endif`) prevents multiple inclusions of the same header file, which could otherwise lead to compilation errors.
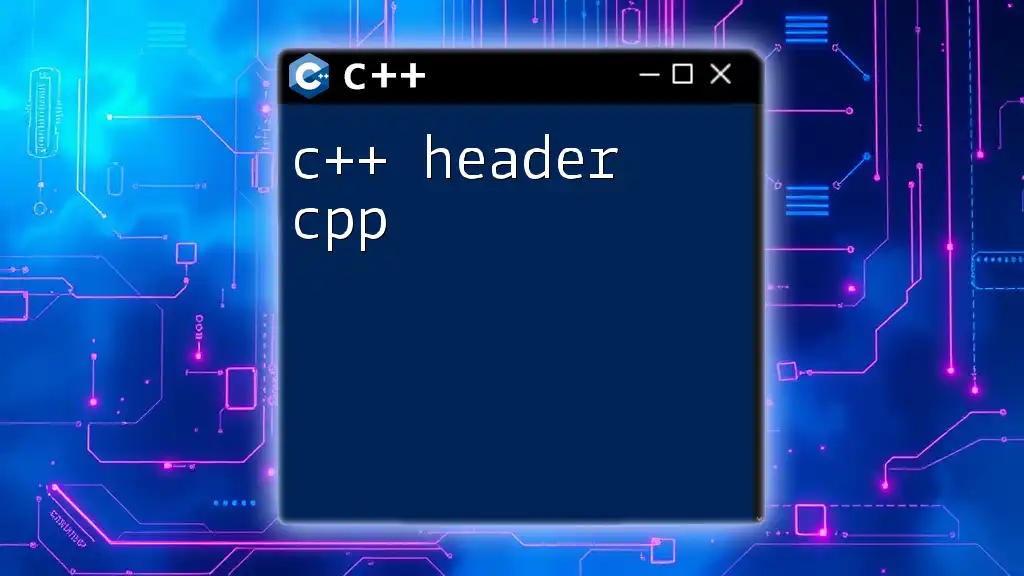
How to Create and Use Header Files in C++
Creating a Header File
Creating a `.h` file is straightforward. You simply create a new text file and give it a name that typically ends with `.h`. For example, you can name your file `myheader.h`.
When naming header files, it's good practice to use a descriptive name to indicate the contents or purpose, helping to maintain code organization.
Including Header Files in C++
To use a header file in your C++ source files, you utilize the `#include` directive. There are two primary ways to include a header file:
-
Using Angle Brackets: This method is used for standard library files or system-specific header files, like:
#include <iostream>
-
Using Quotes: When including user-defined header files, you would typically use quotes to specify the path:
#include "myheader.h"
Example of Including a Header File
The following example demonstrates how to include a user-defined header file in a source file:
#include "myheader.h"
int main() {
MyClass obj;
obj.display();
return 0;
}
In this case, `myheader.h` is included in the implementation file where the `main()` function resides. The class `MyClass` declared in the header file can now be instantiated and utilized here.
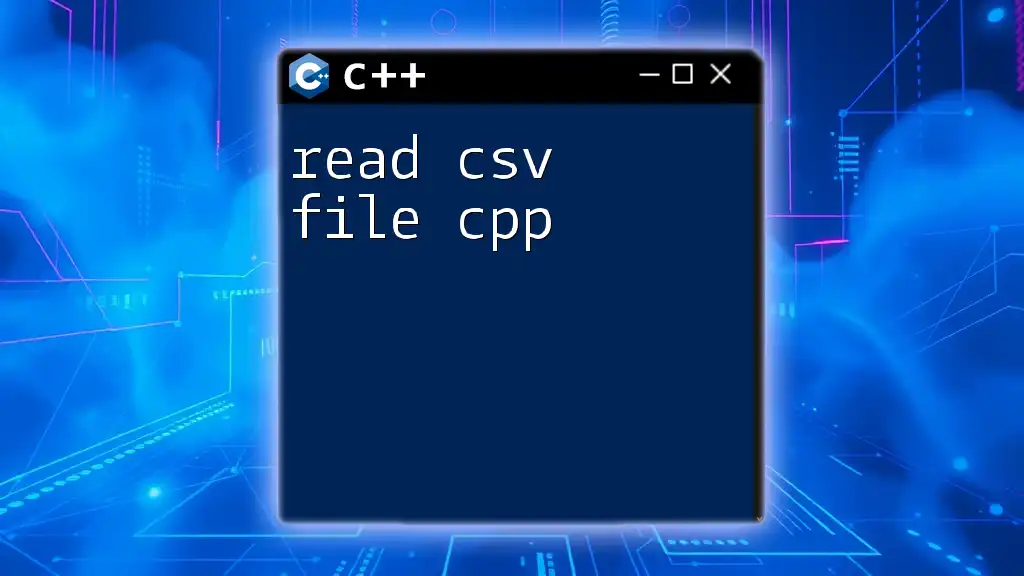
Best Practices for Using Header Files in C++
Organizing Header Files
A well-structured project with organized header files leads to greater maintainability. Consider the following best practices:
-
Place header files in a separate directory, perhaps named `include`, to differentiate them from implementation files located in a `src` directory.
-
Utilize namespaces to encapsulate your code within specific logical areas, reducing potential naming conflicts with other libraries.
Avoiding Common Pitfalls
Common pitfalls include circular dependencies, which occur when two header files reference each other. This can cause issues during compilation and should be resolved by refactoring your code to eliminate these dependencies.
Additionally, strive to keep your header files lightweight by avoiding excessive includes, which can lead to longer compilation times and potential conflicts.
Header Guards and `#pragma once`
To prevent multiple inclusions of header files, it's essential to employ header guards. These guards can be set up using the traditional `#ifndef`, `#define`, and `#endif` directives.
Alternatively, you can simplify this with `#pragma once`, which is supported by most modern compilers. This single line effectively prevents a file from being included more than once:
#pragma once
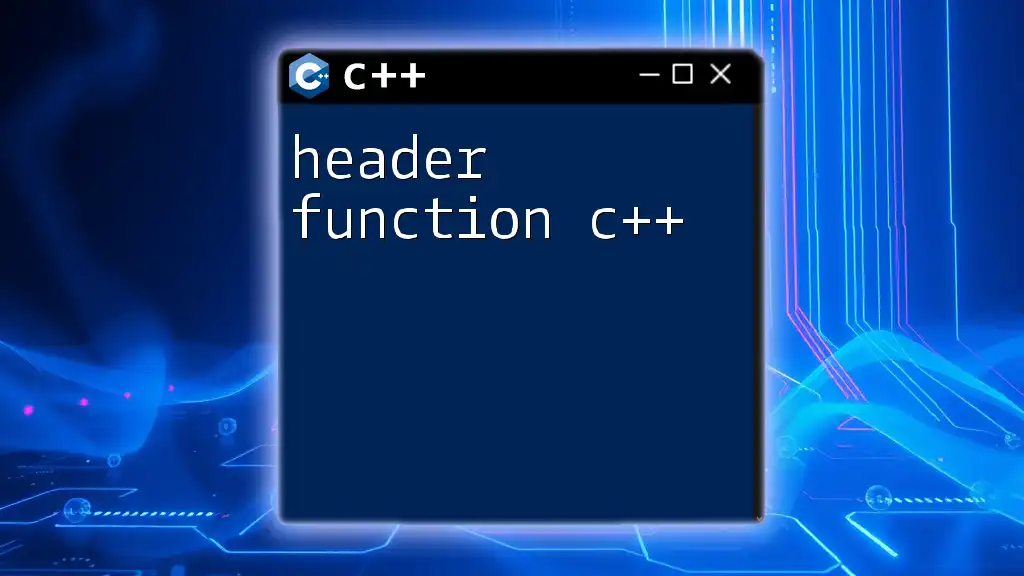
Common Examples and Patterns
Basic Example of a C++ Header File
Here’s a straightforward example of a header file:
#ifndef EXAMPLE_H
#define EXAMPLE_H
void exampleFunction();
#endif // EXAMPLE_H
In this case, `exampleFunction()` is declared in `example.h`, permitting it to be defined in a corresponding `.cpp` file.
Advanced Header File with Templates
Header files can also be used to define templated functions or classes, promoting code reuse through generics. Here’s an example:
#ifndef TEMPLATE_H
#define TEMPLATE_H
template <typename T>
void print(T value) {
std::cout << value << std::endl;
}
#endif // TEMPLATE_H
In this example, `print()` can handle any data type due to its templated nature.
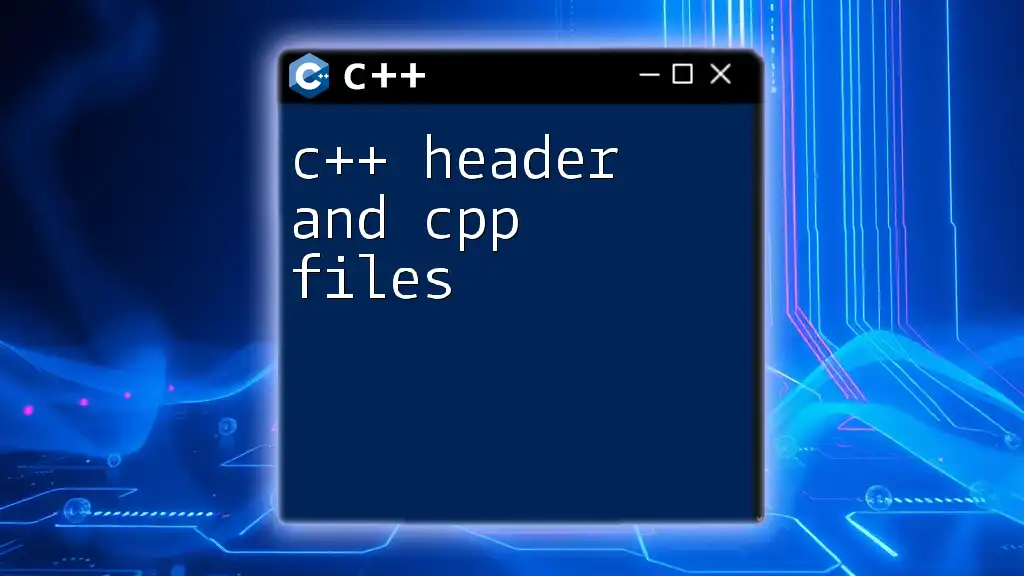
Conclusion
Understanding and effectively utilizing header files in C++ is fundamental for writing organized, modular, and maintainable code. By separating declarations and definitions, header files enable teams to collaborate efficiently and facilitate the reusability of code.
By following best practices, structuring your projects wisely, and avoiding common pitfalls, you will improve not only the readability of your code but also its robustness. Always take the time to experiment and practice with header files, as hands-on experience is immensely beneficial for mastery in C++.
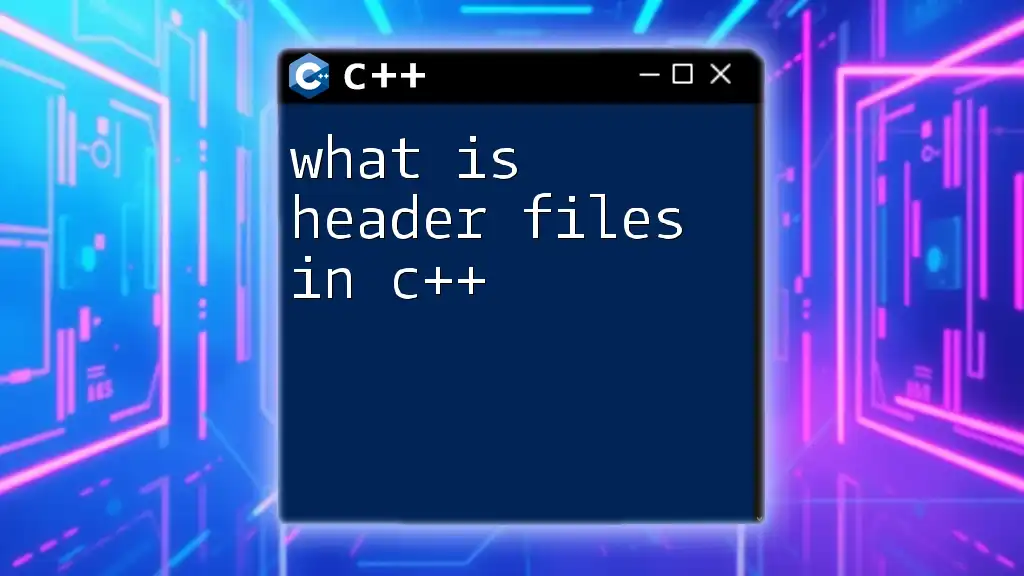
Additional Resources
As you continue your journey in mastering C++, consider exploring recommended books, online tutorials, and open-source projects that demonstrate effective use of header files. These resources will provide deeper insights and practical examples to enhance your learning experience.