To read a CSV file in C++, you can use the ifstream class to open the file and then parse its contents line by line.
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
int main() {
std::ifstream file("data.csv");
std::string line;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string value;
std::vector<std::string> row;
while (std::getline(ss, value, ',')) {
row.push_back(value);
}
// Process the row data as needed
}
file.close();
return 0;
}
Understanding CSV Files
What is a CSV File?
A CSV (Comma-Separated Values) file is a simple text-based format used to store tabular data. Each line in a CSV file corresponds to a row in the table, and each value in that row is separated by a comma. The versatility and ease of use make CSV files an excellent choice for data interchange between different systems. Common uses of CSV files include data export from databases, spreadsheets, and other applications that handle structured data.
Why Use C++ to Read CSV Files?
C++ is a powerful programming language known for its efficiency and control over system resources. When it comes to reading CSV files in C++, you can leverage the language's strong file handling capabilities and speed to manage potentially large datasets. Moreover, learning to read CSV files in C++ can significantly enhance your data processing skills, especially if you are dealing with performance-critical applications.
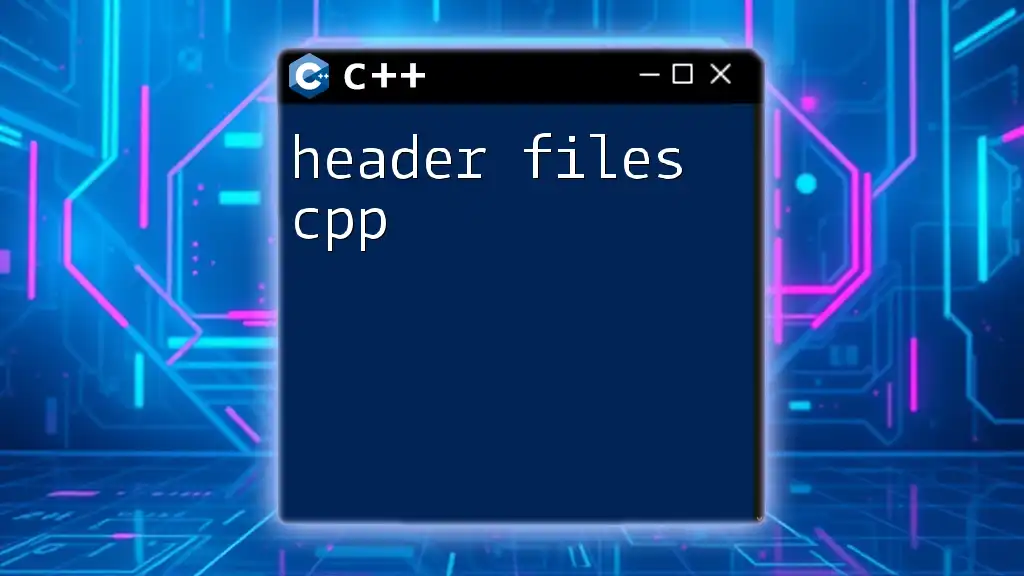
Setting Up Your C++ Environment
Choosing Your IDE
To begin working with C++ and reading CSV files, you need to choose an Integrated Development Environment (IDE). Recommended IDEs include:
- Visual Studio: Feature-rich, great for Windows development.
- Code::Blocks: Lightweight and portable, suitable for various platforms.
- CLion: Powerful but requires a subscription, ideal for professionals.
Setting Up Your Compiler
Having a properly configured C++ compiler is crucial. You can use:
- GCC (GNU Compiler Collection) for Linux and Windows via MinGW.
- MSVC (Microsoft Visual C++) for Windows development.
After installation, you can verify that the compiler is set up correctly by writing a simple C++ program that prints "Hello, World!" to the console.
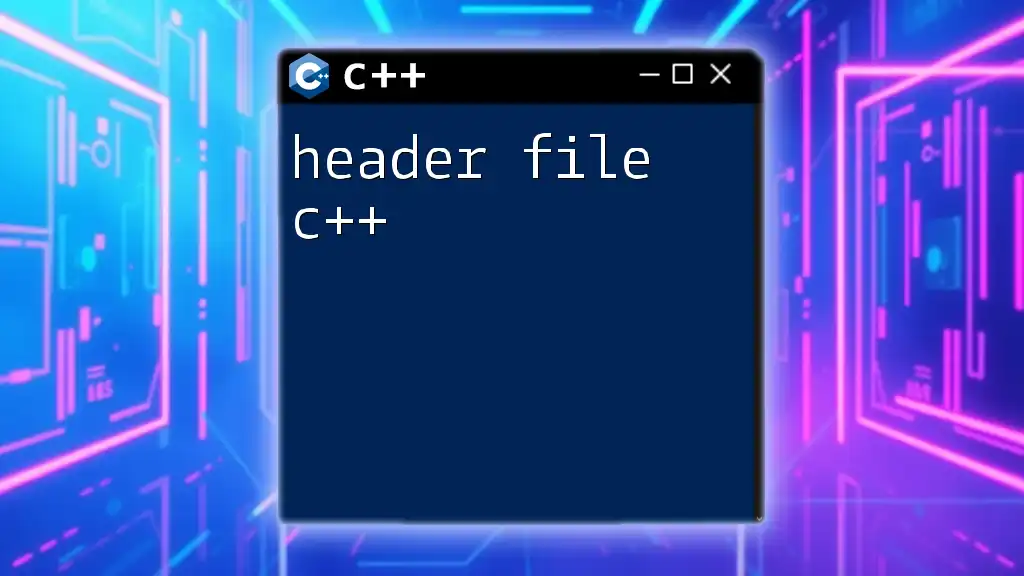
Basic File Input/Output in C++
File Streams in C++
Understanding file streams is fundamental when learning to read CSV files in C++. In C++, file handling is done using streams, specifically `ifstream` for input file streams and `ofstream` for output file streams. Here's a simple syntax to open and close files:
#include <fstream> // Required to use file streams
std::ifstream inputFile("example.csv"); // Opening a file for reading
// Perform file operations
inputFile.close(); // Don’t forget to close the file
Reading From a File
To read from a CSV file, you often use the `getline()` function, which allows you to read each line until the end of the file. Here’s a basic code snippet demonstrating this:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.csv");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::cout << line << std::endl; // Prints each line
}
file.close();
}
return 0;
}
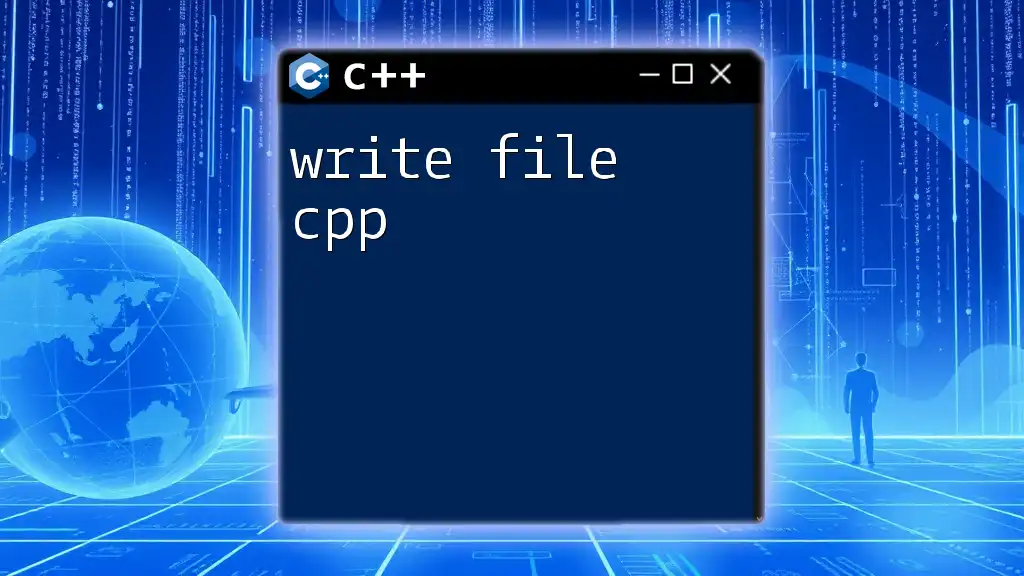
Parsing CSV Data in C++
Understanding CSV Format
When reading CSV files, it is vital to understand their format. Each value in a line is separated by a delimiter, commonly a comma. However, issues can arise, such as when commas appear within quoted fields. Thus, effective parsing is essential for accurately extracting the data.
Tokenizing CSV Lines
To extract individual values from each line, you can use `std::stringstream` to tokenize the line. The `getline()` function, in conjunction with a `stringstream`, enables you to separate the fields based on the specified delimiter. Here’s how you can do it:
#include <sstream>
std::stringstream ss(line);
std::string token;
while (getline(ss, token, ',')) {
std::cout << token << std::endl; // Prints each token
}
Example: Reading and Parsing a CSV File
Combining the aforementioned elements, here’s a comprehensive example of reading a CSV file, parsing its contents, and printing them to the console. This code demonstrates how to read a file line by line and tokenize it.
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
int main() {
std::ifstream file("example.csv");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::stringstream ss(line);
std::string token;
while (getline(ss, token, ',')) {
std::cout << token << " "; // Print each token with a space
}
std::cout << std::endl; // New line for each row
}
file.close();
}
return 0;
}
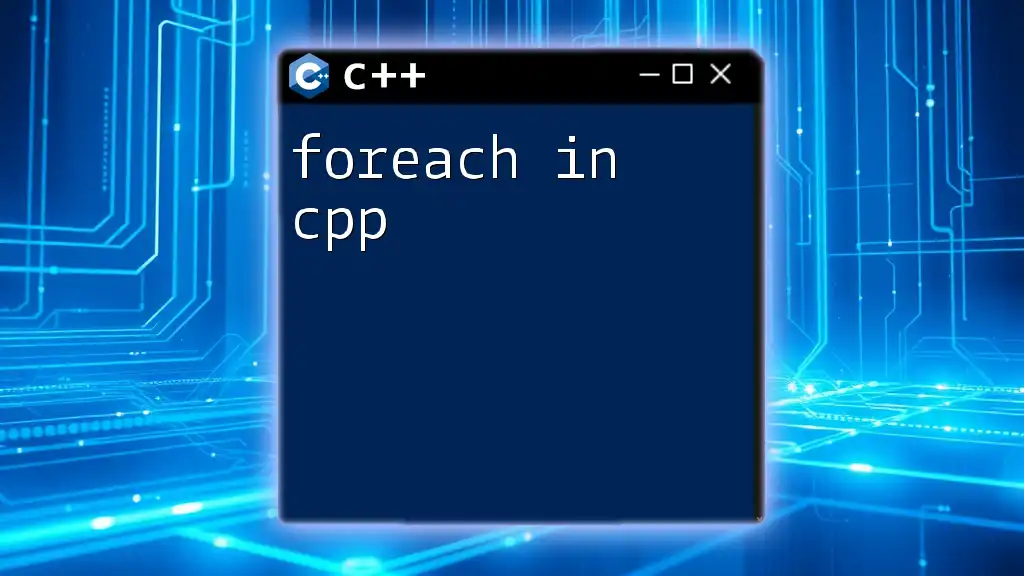
Advanced CSV Handling in C++
Dealing with Quoted Fields
Caution is required when dealing with quoted fields that may contain commas. Such cases need special handling to ensure that your parser correctly interprets them. You may need to write a custom parser that can recognize when to treat commas as part of a value.
Error Handling and Validation
Implementing error handling is crucial in any file-processing application. Always check if the file opens successfully and handle scenarios where file reading might fail. Additionally, validate the data read to ensure its integrity before processing.
Using Libraries for Enhanced CSV Handling
If you prefer to focus on the logic of your application rather than the nitty-gritty of file parsing, consider using C++ libraries designed for handling CSV files. Libraries like `csv.h` or `RapidCSV` can simplify the process significantly. They provide built-in methods to read and parse CSV files effectively, reducing the complexity of your code:
// Example using a library
// #include "csv.h"
// csv::CSVReader csvReader("example.csv");
// for (auto& row : csvReader) {
// std::cout << row[0] << ", " << row[1] << std::endl;
// }
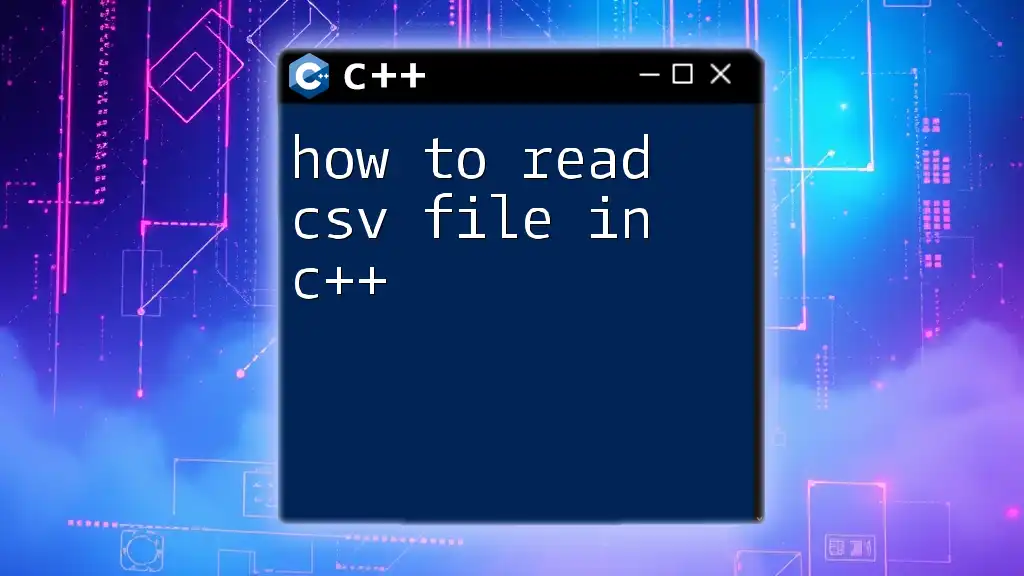
Conclusion
Learning how to read a CSV file in C++ is a valuable skill that empowers you to handle structured data efficiently. This guide has navigated through the complexities of CSV file formats, basic file handling, parsing techniques, and advanced strategies. By practicing and implementing these techniques, you can enhance your programming proficiency in C++ and utilize it for various data-related tasks. Don't hesitate to share your experiences and challenges as you continue to explore the world of C++.