PThreads, or POSIX Threads, provide a standardized way to create and manage multiple threads in C++, allowing for concurrent execution within a program.
Here’s a simple code snippet demonstrating the creation of a thread using PThreads in C++:
#include <pthread.h>
#include <iostream>
void* printMessage(void* message) {
std::cout << static_cast<char*>(message) << std::endl;
return nullptr;
}
int main() {
pthread_t thread;
const char* message = "Hello, PThreads!";
pthread_create(&thread, nullptr, printMessage, (void*)message);
pthread_join(thread, nullptr);
return 0;
}
Understanding pthreads in C++
What are pthreads?
Pthreads, or POSIX threads, are a standardized C library for thread management and synchronization. They enable developers to create and manage threads in a portable manner across various platforms. The design goal of pthreads is to provide a lightweight process-oriented threading model that allows multiple threads within a single process to run concurrently.
Benefits of using pthreads in C++
Using pthreads in C++ offers several key benefits:
- Performance Advantages: Pthreads directly map to kernel threads, allowing for scheduling and execution that can effectively utilize multicore processors.
- Resource Utilization: Memory consumption is reduced as threads share the same address space, allowing easier communication between them.
- Management of Complex Interactions: Pthreads provide various synchronization mechanisms that help manage interactions between threads, minimizing race conditions and deadlocks.
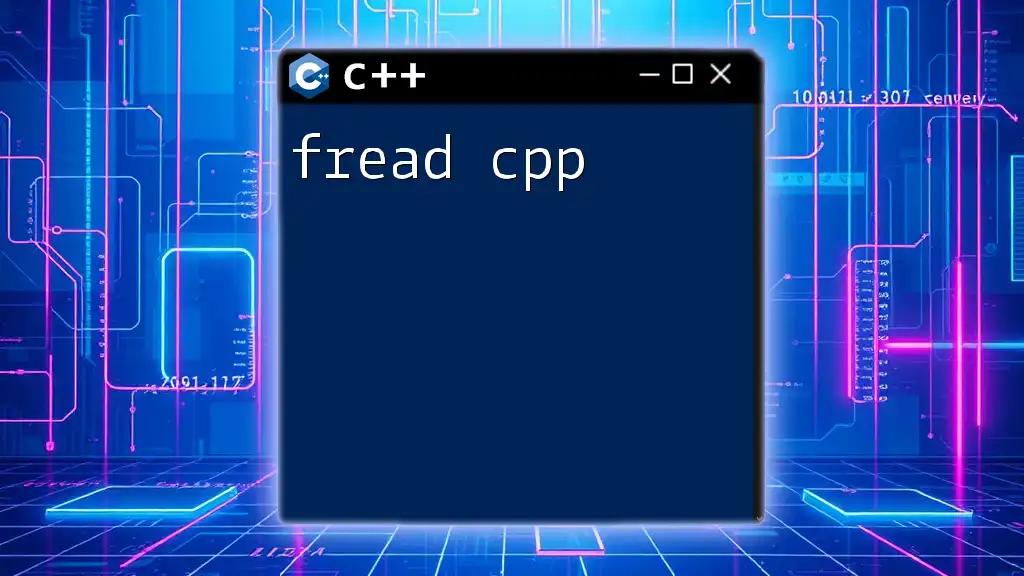
Setting Up Your Environment
To utilize pthreads efficiently, ensure that your development environment is set up correctly.
Required libraries for pthread usage in C++
Most modern C++ compilers include support for pthreads out of the box, but confirm that you have the pthread library available. This might require libraries such as `libpthread` on Linux systems.
Installing pthreads on popular operating systems
-
Windows Setup: Use MinGW or Cygwin, which provide access to pthreads on Windows.
-
Linux Setup: On most Linux distributions, `libpthread` comes pre-installed. You can install additional development headers using your package manager. For example:
sudo apt-get install build-essential
-
macOS Setup: Pthreads are also included natively. Ensure that Xcode command-line tools are installed.
Example Code: Checking for pthread Availability
To check for pthread support, you can write a simple program that compiles and runs with pthreads. If it compiles successfully, pthreads are available:
#include <pthread.h>
#include <iostream>
int main() {
std::cout << "Pthreads are available!" << std::endl;
return 0;
}

Basic pthread Commands
Overview of pthread Commands
Pthreads offer a set of commands for thread management. Understanding the function signatures and usage is essential for effective programming in C++ with pthreads.
Creating Threads with pthread_create
The `pthread_create` function is fundamental for creating threads. It requires that you specify attributes of the thread, a starting routine (function that the thread will execute), and an argument to pass into the started routine.
Syntax:
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine)(void *), void *arg);
Example: A Simple Program Creating a Thread
The following code demonstrates how to create and execute a thread using `pthread_create`:
#include <iostream>
#include <pthread.h>
void* myThreadFun(void* arg) {
std::cout << "Hello from thread!" << std::endl;
return nullptr;
}
int main() {
pthread_t thread;
pthread_create(&thread, nullptr, myThreadFun, nullptr);
pthread_join(thread, nullptr);
return 0;
}
In this example, the `myThreadFun` function is executed in a separate thread, displaying a message to the console.
Managing Thread Lifecycle
Understanding `pthread_join`: This function is called to wait for a thread to finish execution. It is essential for resource cleanup as it allows the parent thread to synchronize with child threads.
Syntax and Usage:
void pthread_join(pthread_t thread, void **retval);
Example: Joining Threads
To ensure that the main thread waits for `myThreadFun` to complete, we make use of `pthread_join`:
pthread_join(thread, nullptr);
Detaching Threads with pthread_detach
Sometimes, you may not need to join threads, especially for fire-and-forget type threads. In such cases, you detach the thread to allow it to clean up resources independently.
Syntax and Example:
pthread_detach(thread);
Detaching means that once the thread finishes, its resources are automatically reclaimed by the system, making it an effective choice for non-critical background tasks.

Synchronization Mechanisms in pthreads
Mutex Locks with pthread_mutex
Using `pthread_mutex` allows multiple threads to manage access to shared resources, preventing race conditions. A mutex provides a lock that can be used for synchronization purposes.
Example of Using pthread_mutex:
pthread_mutex_t lock;
pthread_mutex_init(&lock, nullptr);
pthread_mutex_lock(&lock);
// Critical section
pthread_mutex_unlock(&lock);
This code snippet shows a basic use of mutexes for thread synchronization.
Conditional Variables with pthread_cond
Condition variables provide a mechanism for threads to block until a particular condition occurs, often used with mutexes.
Example Code:
pthread_cond_t cond_var;
pthread_cond_wait(&cond_var, &lock);
This snippet demonstrates how a thread can wait on a condition variable until it receives a signal that the condition has been met.
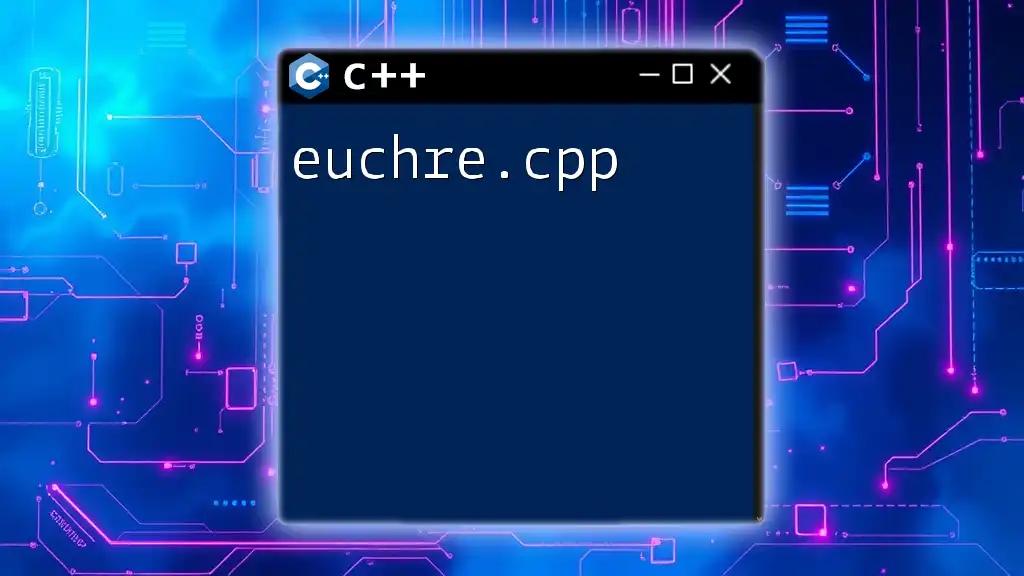
Advanced Topics in pthreads
Thread Safety
Thread safety is critical in multi-threaded applications. This refers to the property that enables multiple threads to operate on shared data without causing inconsistencies. Strategies include:
- Using Mutexes: To guard critical sections of code.
- Thread Local Storage: Use thread-specific variables to avoid shared data issues.
Error Handling in pthreads
When working with pthreads, it's important to handle errors gracefully. Each pthread function typically returns an error code indicating success or failure.
Common Error Codes in Pthread Functions:
- `EAGAIN`: Insufficient resources to create another thread.
- `EINVAL`: Invalid settings in `pthread_create`.
Example of Error Handling:
if (pthread_create(&thread, nullptr, myThreadFun, nullptr)) {
std::cerr << "Error creating thread!" << std::endl;
}
This simple check informs the developer about the status of thread creation.
Real-World Applications of pthreads in C++
Pthreads are invaluable in various scenarios. Some common applications include:
- Multi-threaded Servers: To handle multiple client connections simultaneously, improving responsiveness.
- Concurrent Data Processing: Ideal for high-performance applications where large datasets need processing in parallel.
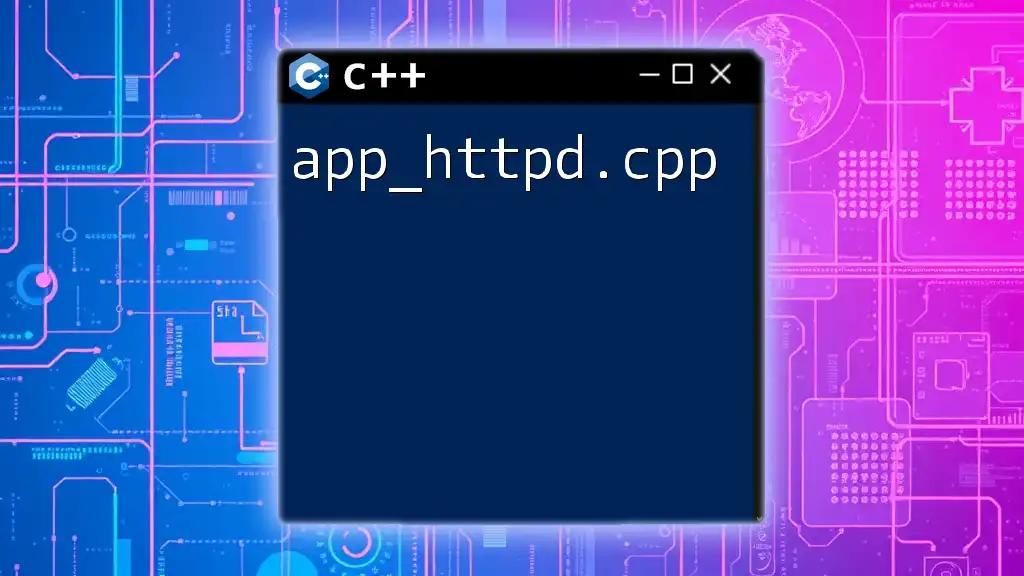
Best Practices for Using pthread in C++
To make the most of pthreads in C++, consider the following best practices:
- Use Mutexes judiciously to protect shared resources.
- Regularly test your multi-threaded applications to catch race conditions and deadlocks.
- Keep the threads' responsibilities clear and singular to lower complexity and improve maintainability.
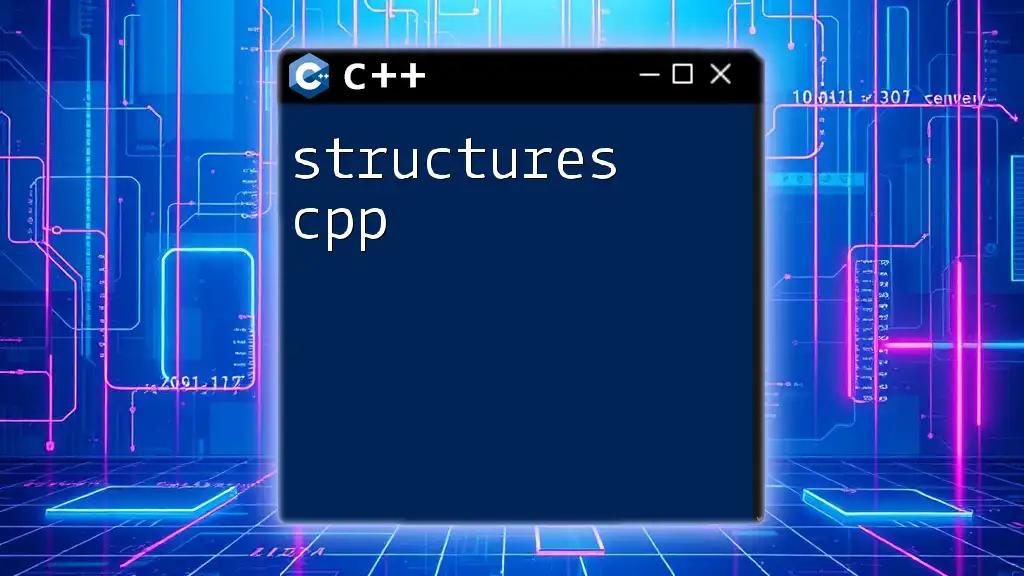
Conclusion
Mastering `pthread cpp` not only enhances your C++ programming skills but also equips you with the tools to effectively utilize multiple cores and improve application responsiveness. Practice implementing examples and explore advanced features to further deepen your understanding of threading and concurrency in C++.

Additional Resources
For further exploration, consider looking into specialized books or online courses about pthreads and synchronization techniques. The official pthread documentation is also a fantastic resource for in-depth function descriptions and additional details. Engaging with online communities can provide practical insights and support for your threading journey.