The `euchre.cpp` file is a C++ program that implements the classic card game Euchre, facilitating gameplay mechanics and player interactions.
Here’s a basic code snippet that demonstrates how you might start setting up classes and functions for the game:
#include <iostream>
#include <vector>
#include <string>
class Card {
public:
std::string suit;
std::string rank;
Card(std::string s, std::string r) : suit(s), rank(r) {}
};
class Player {
public:
std::string name;
std::vector<Card> hand;
Player(std::string n) : name(n) {}
};
void dealCards(std::vector<Player>& players) {
// Function logic to deal cards to players
}
int main() {
std::vector<Player> players = { Player("Alice"), Player("Bob") };
dealCards(players);
// Rest of the game logic
return 0;
}
Understanding Euchre
What is Euchre?
Euchre is a trick-taking card game that originated in Europe and has become particularly popular in North America. Generally played by four players in two partnerships, the game revolves around teams trying to win the most tricks, ultimately scoring points. A standard deck is shortened to 24, 28, or even 32 cards, depending on the format of play.
Key Concepts in Euchre
Understanding Euchre means grasping several fundamental concepts that govern the game:
-
Deck of Cards: Rather than using a full 52-card deck, Euchre utilizes just the higher cards, specifically the Aces, Kings, Queens, Jacks, Tens, and Nines of each suit.
-
Players: The game is played by four players divided into two teams. Each player occupies a specific position, and communication between partners is vital for strategic play.
-
Scoring System: Points are awarded based on the number of tricks a team wins. The winning team can gain additional points by declaring "trump" or "going alone," challenging the opposing team more aggressively.
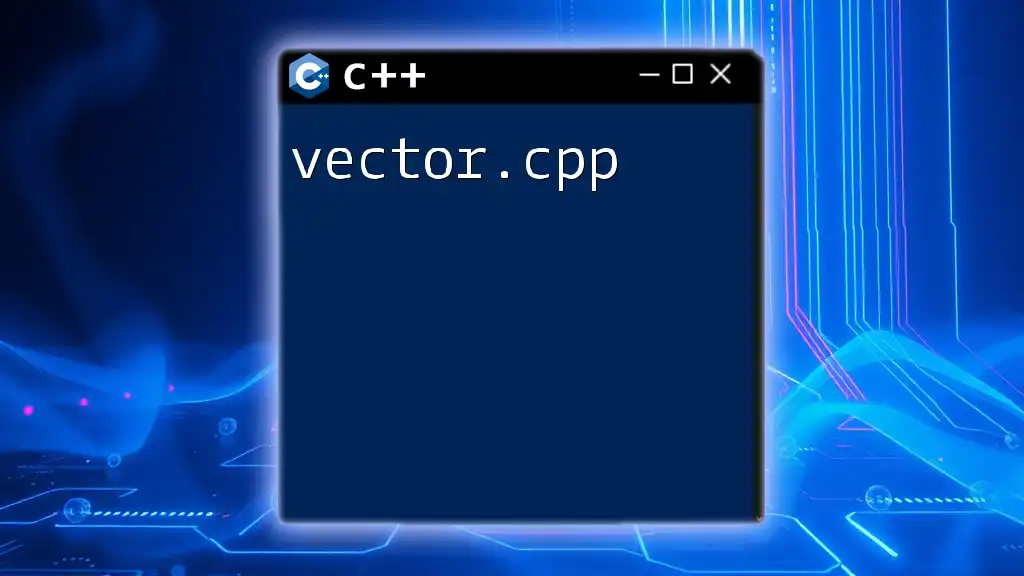
Setting Up the Euchre Game in C++
Creating a euchre.cpp project requires careful setup and organization.
Project Structure
Establish a clear project structure for ease of development:
-
src/: This folder contains the main source files where your game logic will reside.
-
include/: Here, you will define your header files with class declarations and any required libraries.
-
assets/: If you opt for visual components, like card images, create an assets directory to manage these resources effectively.
Required Libraries
For a complex project like euchre.cpp, consider using libraries that can enhance functionality. The SFML (Simple and Fast Multimedia Library) may be beneficial if you plan to implement graphics. The Standard Template Library (STL) can optimize data management, helping with vectors and queues to manage your game’s structure.
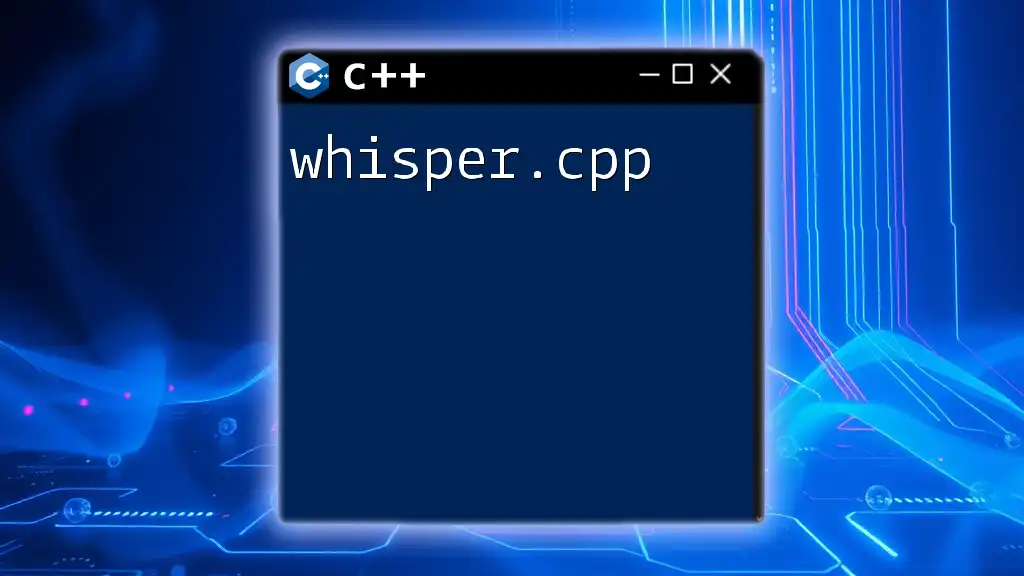
Core Components of euchre.cpp
When programming euchre.cpp, defining classes and managing game objects is fundamental.
Defining Classes and Objects
Card Class
Start with creating a `Card` class that represents the individual cards in Euchre.
class Card {
public:
enum Suit { HEARTS, DIAMONDS, CLUBS, SPADES };
enum Value { NINE, TEN, JACK, QUEEN, KING, ACE };
Card(Suit suit, Value value);
Suit getSuit() const;
Value getValue() const;
private:
Suit suit;
Value value;
};
This class includes enumerations for suits and values, making card management predictable and straightforward. Define getter methods to access card attributes.
Deck Class
Next, manage your card collection with a `Deck` class:
class Deck {
public:
Deck();
void shuffle();
Card deal();
private:
std::vector<Card> cards; // STL vector to hold the cards
};
In this class, the `shuffle()` method randomizes the deck, and `deal()` distributes cards to players when a new game begins.
Player Class
A `Player` class encapsulates details about the players:
class Player {
public:
void addCard(const Card& card);
void playCard(int index);
private:
std::vector<Card> hand; // Player's hand of cards
int score;
};
The `addCard()` method adds cards to a player’s hand, while `playCard()` allows them to play a card based on the index in their hand. This setup makes player interactions intuitive.
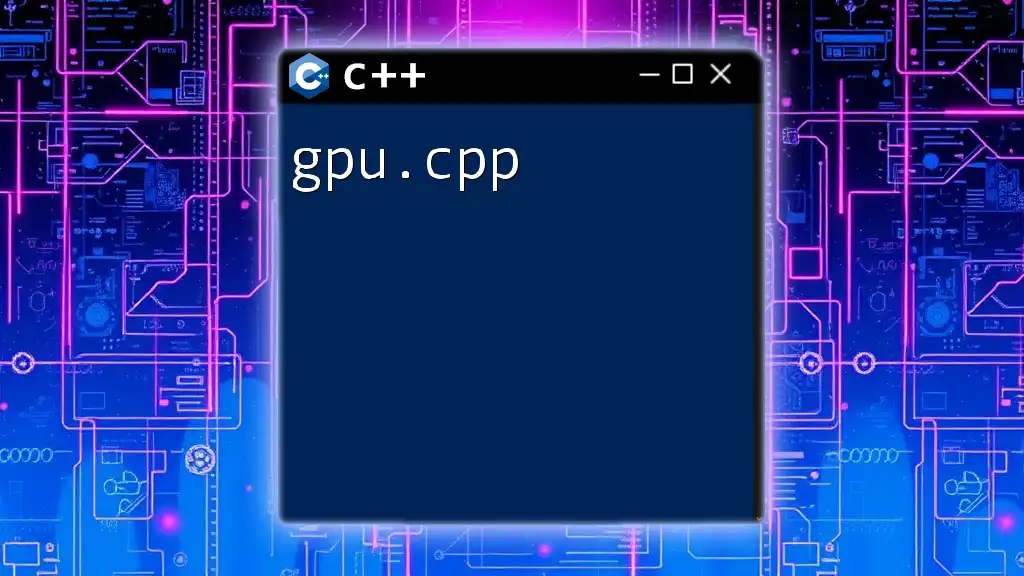
Game Logic
Initialization
Upon starting the game, it’s crucial to initialize the necessary elements, including shuffling and dealing cards. The initial game setup code should involve creating instances of the `Deck`, shuffling the cards, and dealing to every player, preparing the game for play.
Turn Management
Game Flow
A robust turn-based system is essential for managing player actions. Each turn might involve checking if the player can play a card:
while (!gameOver) {
// Handle player turns and game logic
}
This loop continues until the game's conclusion, ensuring each player has a chance to act.
Mechanisms for Scoring
Develop methods to calculate and apply scores based on the number of tricks won. This involves checking conditions each round to determine point allocation:
void calculateScore(Player& player, int points) {
player.incrementScore(points); // Example of incrementing score
}
This code snippet ensures accuracy in point allocation as players engage in rounds.
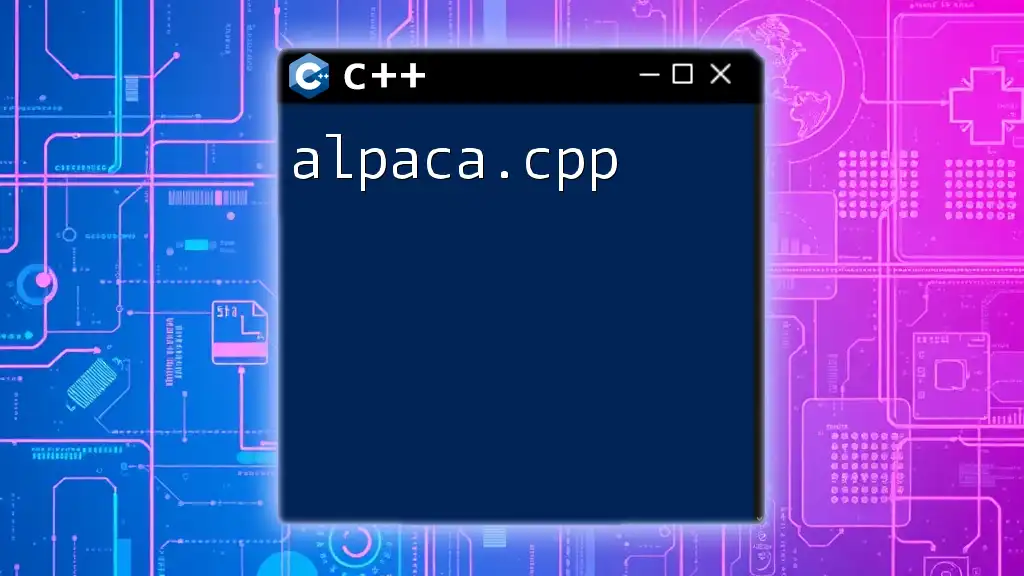
Implementing Gameplay
Sample Game Loop
The heart of the euchre.cpp application lies in its game loop. Players will interact with the game as it runs continuously. Here’s a basic setup for the game loop:
while (!gameOver) {
// Manage player turns
// Update scores
// Check for winning conditions
}
This loop keeps the game alive, processing player actions until a victor emerges.
Handling User Input
Managing user interaction is vital. Utilizing `std::cin` for command-line input allows players to make decisions through text inputs or commands. You might check the validity of input, ensuring players understand their available actions.
std::string input;
std::cin >> input; // Capture user input for card play or other actions
This method keeps gameplay interactive and engaging.
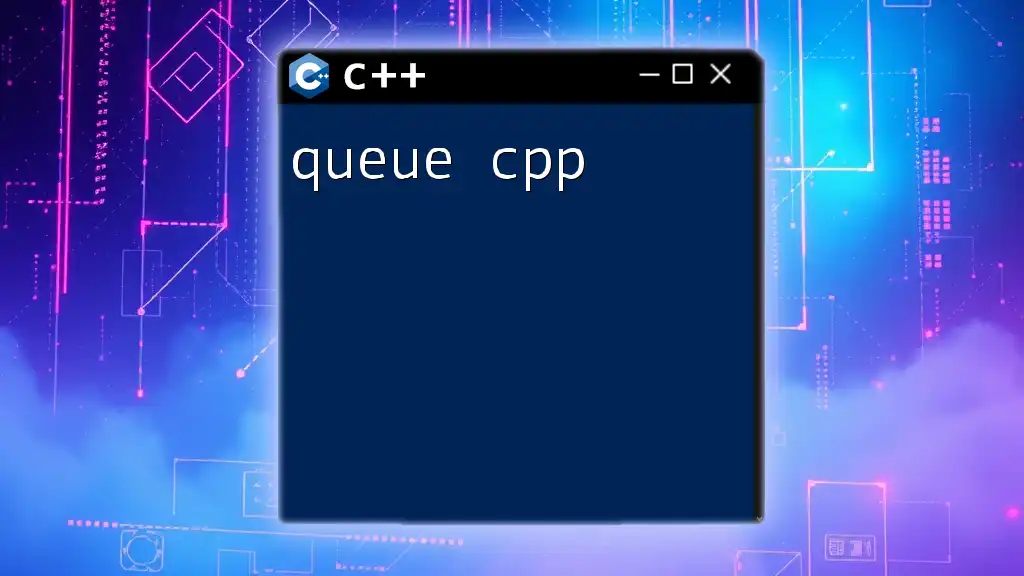
Conclusion
Implementing euchre.cpp has you traversing through the fascinating world of C++ programming while recreating an engaging card game experience. With defined classes for cards, players, and game logic, you’re set to adapt and enhance your application further. Future suggestions could involve adding features such as computerized opponents or online multiplayer modes to expand your game’s reach.
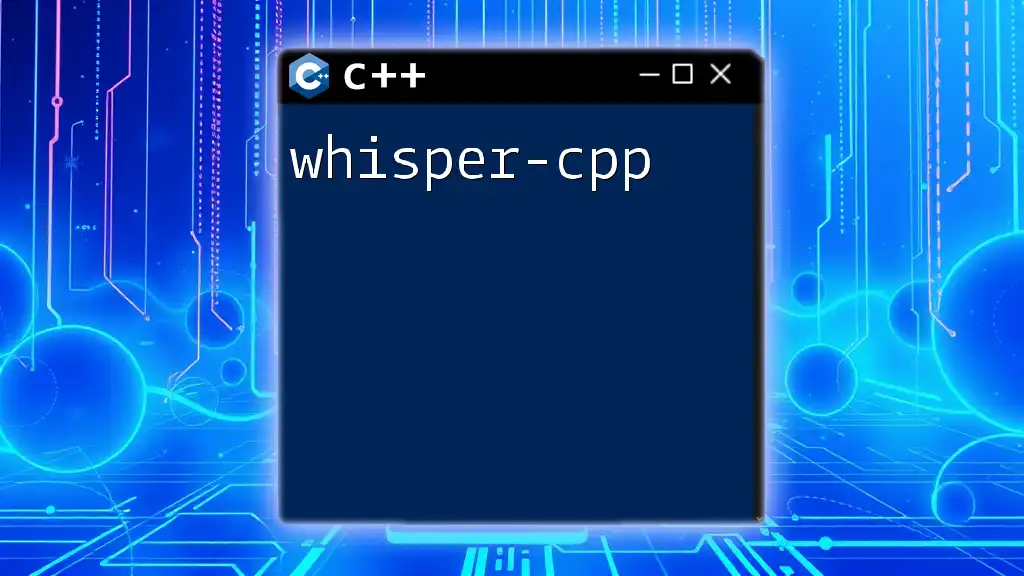
Additional Resources
For further learning, consider delving into C++ programming books, joining online forums like Stack Overflow, or participating in GitHub projects. These outlets will enhance your understanding and provide valuable insights as you grow your skills.
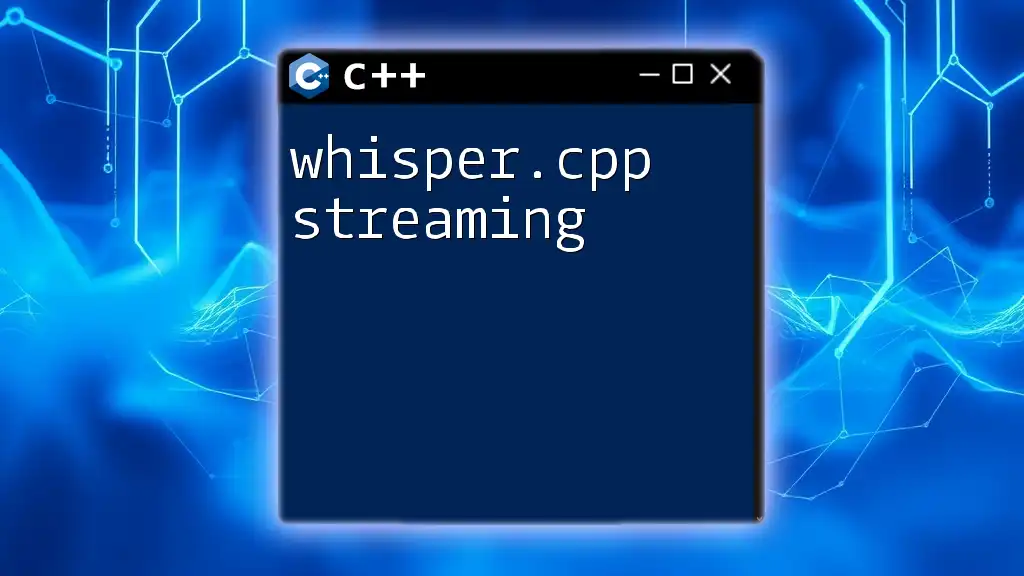
Call to Action
Don't forget to follow us for more concise and effective programming tutorials. Sign up for our newsletter or connect through social media to stay updated on future posts and guides in the world of C++.