"whisper.cpp" is a lightweight C++ implementation designed to facilitate easy integration of Whisper speech recognition capabilities in applications.
#include <whisper.h>
int main() {
Whisper whisper;
whisper.load("model_path");
std::string transcription = whisper.recognize("audio.wav");
std::cout << transcription << std::endl;
return 0;
}
What is `whisper.cpp`?
Whisper.cpp is a highly efficient and versatile component in the realm of C++ programming. It serves as a simplified interface that allows developers to execute various commands quickly and effectively. Historically, whisper.cpp emerged as a response to the need for more intuitive programming solutions, making it accessible to both beginners and seasoned developers alike.
In practical terms, whisper.cpp finds its applications in a myriad of scenarios—from basic scripting to more advanced software development tasks. By utilizing whisper.cpp, developers can achieve high performance without sacrificing code clarity and productivity.

Setting Up Your Environment
Prerequisites
Before diving into whisper.cpp, it’s essential to have the right tools in place. You'll need:
- A C++ compiler (such as GCC or Clang)
- An Integrated Development Environment (IDE) like Visual Studio, Code::Blocks, or any text editor of your choice
Installation Steps
To install the necessary packages for whisper.cpp, follow these steps:
-
Install a C++ compiler: Choose your operating system’s package manager to install it. For example, on Ubuntu, you can use:
sudo apt-get install g++
-
Set up your IDE: Download and install your preferred IDE. For instance, you can download Visual Studio Community Edition from Microsoft's official site.
-
Verify your installation: After installation, open your terminal or command prompt and type:
g++ --version
This command will confirm that your compiler is correctly installed.

Understanding the Core Concepts of `whisper.cpp`
Key Features
One of the standout aspects of whisper.cpp is its simplicity and speed. This allows for rapid command execution, which is particularly valuable in time-sensitive development environments.
Versatility is another important feature. Whisper.cpp can adapt to various programming frameworks and styles, making it suitable for diverse projects—whether you're dealing with embedded systems, game development, or software applications.
Core Components
Functions
At the core of whisper.cpp are its functions. These functions are designed to streamline operations and provide a straightforward interface. Here's a simple function declaration and execution:
void greet() {
std::cout << "Hello from whisper.cpp!" << std::endl;
}
In this example, the function `greet()` serves to demonstrate the basic syntax and usage of functions within whisper.cpp.
Objects and Classes
Whisper.cpp also supports object-oriented programming (OOP). You can create classes and instantiate objects effortlessly. Here’s an example of defining and using a class:
class Whisper {
public:
void speak() {
std::cout << "Whispering technique!" << std::endl;
}
};
In this snippet, the `Whisper` class has a method `speak()`, which outputs a message when called.
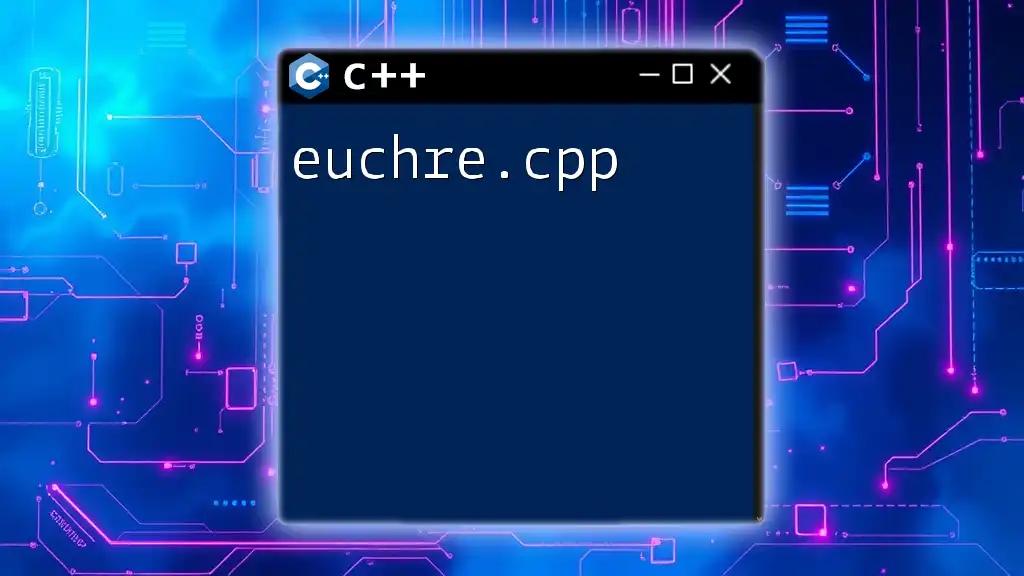
How to Use `whisper.cpp`
Basic Commands
The basic commands available in whisper.cpp allow you to harness its capabilities effectively. One primary command you might encounter is:
whisper("This is a whisper command.");
This simple command enables you to execute a whisper function, showcasing the streamlined nature of whisper.cpp.
Advanced Usage
For those looking to delve deeper, advanced usage of whisper.cpp allows for more complex command chaining and efficient scripting. Here’s an example of combining commands for enhanced functionality:
whisper("Advanced command usage");
whisper("Chaining commands for efficiency");
In this example, you can see how multiple commands can be executed in sequence, which is particularly beneficial for scripting tasks that require consolidated actions.
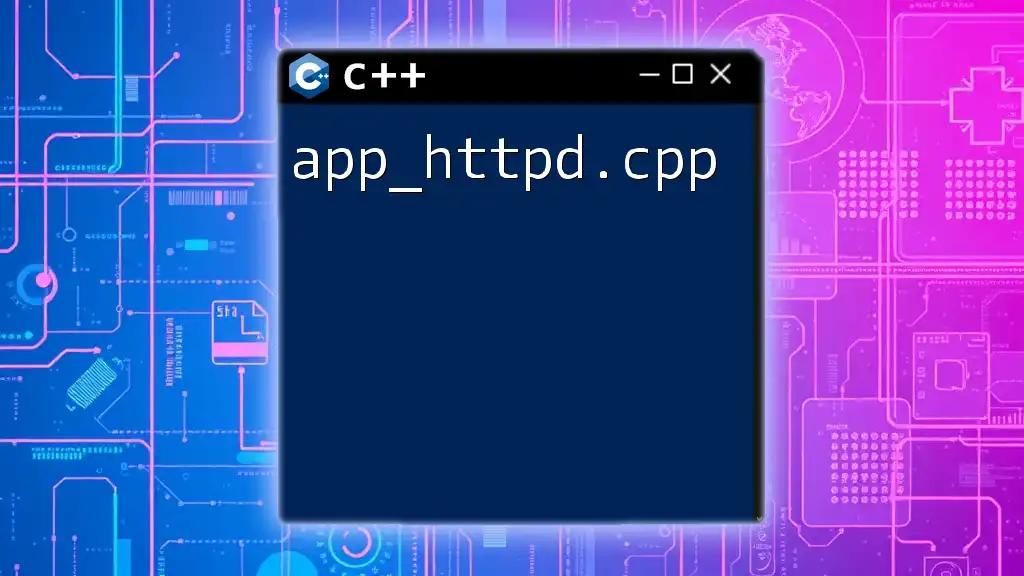
Error Handling in `whisper.cpp`
While working with whisper.cpp, you may encounter common errors. These could range from syntax issues to runtime exceptions. Here are some typical issues along with their solutions:
- Syntax errors: Ensure your command structure adheres to the prescribed syntax.
- Runtime exceptions: Implement try-catch blocks around your commands to manage errors gracefully.
Debugging Tips
To debug effectively in whisper.cpp, consider the following strategies:
- Use print statements: Output variable values to trace the flow of your code.
- Leverage debugging tools: IDEs often come with built-in debuggers that can step through your code and help identify problematic areas.
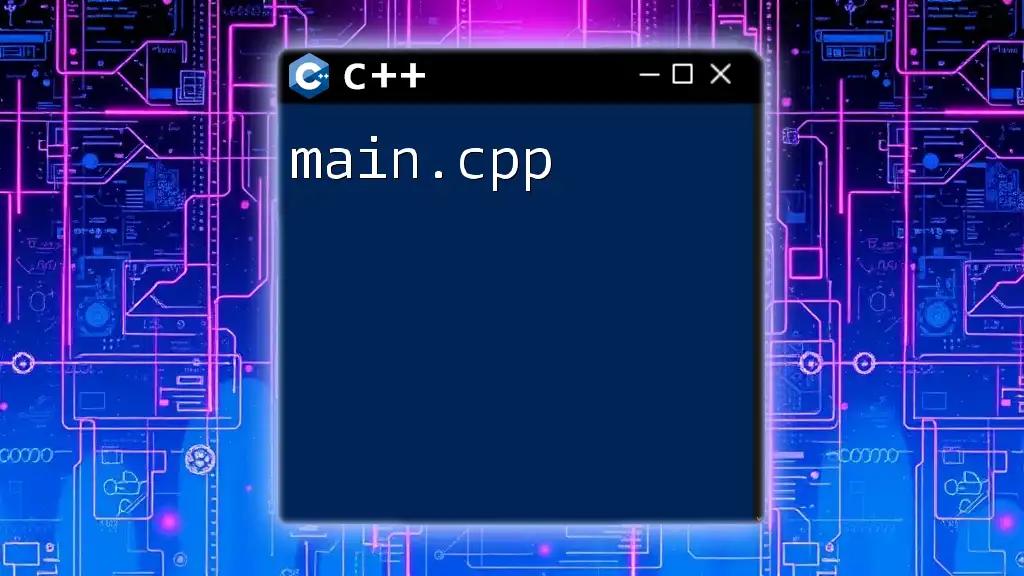
Performance Optimization
To ensure your whisper.cpp code runs efficiently, consider the best practices outlined below:
- Minimize unnecessary computations: Optimize loops and conditional statements to avoid redundancy.
- Profile your code: Utilize profiling tools such as Valgrind or gprof to measure performance and identify bottlenecks.
Following these tips will help you harness the full potential of whisper.cpp while maximizing speed and efficiency.

Real-World Examples
Several projects have successfully integrated whisper.cpp, showcasing its practical applications. One example might be a simple chat application that utilizes whisper commands to send messages seamlessly between users. Here's an illustrative code snippet:
int main() {
Whisper w;
w.speak();
return 0;
}
In this minimal example, we create an instance of the `Whisper` class and invoke `speak()`, allowing for effective communication within the application context.
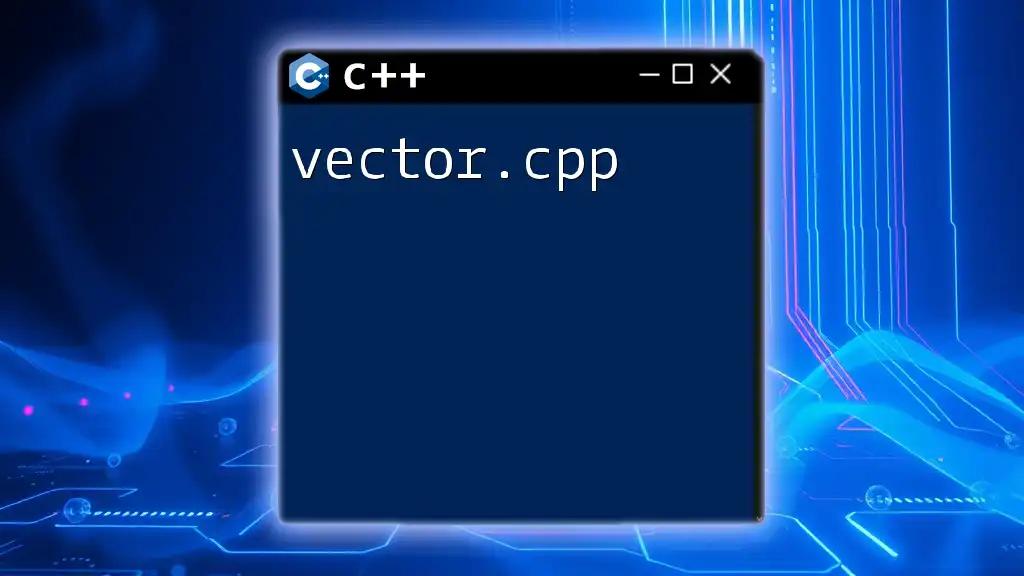
Conclusion
Mastering whisper.cpp not only enhances your C++ skills but also empowers you to create efficient and effective solutions. By embracing its simplicity and robust features, you can significantly improve your development workflow. We encourage you to dive into whisper.cpp experiments and share your insights and experiences with the community!
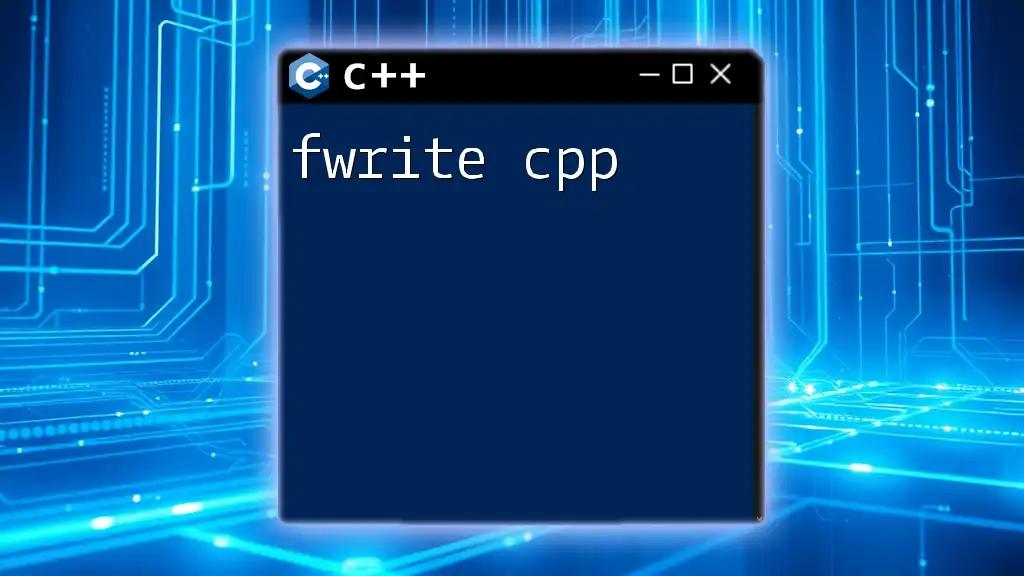
Additional Resources
To further enhance your understanding of whisper.cpp and C++, consider exploring the following additional resources:
- Recommended Books: "The C++ Programming Language" by Bjarne Stroustrup
- Online Courses: Platforms like Coursera or Udemy offer numerous C++ courses that dive deeper into the language.
- Community Forums: Engage in forums like Stack Overflow or Reddit’s r/cpp to connect with developers and gain insights into common practices.
With this guide, you are well-equipped to embark on your journey with whisper.cpp and optimize your programming endeavors!