In C++, `this` is a special pointer that refers to the object for which a member function is called, allowing access to the object's members and functions.
Here is a code snippet demonstrating its usage:
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "The value is: " << this->value << std::endl;
}
void setValue(int v) {
this->value = v;
}
private:
int value;
};
int main() {
MyClass obj;
obj.setValue(42);
obj.display();
return 0;
}
Understanding "this" Keyword
What is "this"?
The `this` keyword in C++ is a special pointer that refers to the calling object within a member function or a method. It allows developers to differentiate between member variables and parameters with the same name and provides access to the object's own data members. Understanding `this` is crucial for writing robust C++ code, as it provides context to the object instance and enables clear and efficient coding practices.
Context of "this"
In C++, `this` is primarily used within non-static member functions of a class. A non-static member function can manipulate the data members (variables) of the object from which it was called. The `this` pointer gives you a way to reference the current object's instance, enabling effective management of its attributes.
You cannot use `this` in static member functions since these functions don't operate on an instance of the class but rather belong to the class itself. This limitation is important to remember, as it marks a significant distinction between static and non-static contexts.

Using "this" Reference in Classes
Accessing Member Variables
One of the primary uses of the `this` pointer is to resolve naming conflicts between member variables and parameters passed into a constructor or method. Here’s an example:
class Example {
private:
int value;
public:
Example(int value) {
this->value = value; // Using 'this' to distinguish between parameter and member variable
}
};
In this example, the constructor's parameter, `value`, is shadowing the class member variable with the same name. By using `this->value`, you clearly indicate that you are referring to the member variable, not the parameter. This practice promotes code clarity and helps avoid bugs caused by variable shadowing.
Chaining Member Function Calls
Chaining member function calls, or method chaining, allows for more readable and concise code. The `this` pointer plays a pivotal role in achieving this by returning the current object instance. Here’s how it works:
class Builder {
private:
int data;
public:
Builder& setData(int data) {
this->data = data; // Assigning the input data to the member variable
return *this; // Returning this for chaining
}
void show() {
std::cout << data << std::endl; // Displaying the member variable
}
};
// Usage
Builder builder;
builder.setData(10).show(); // Method chaining
In this `Builder` class, the `setData` method assigns a value to `data` and returns a reference to the current instance. This allows you to call `show()` immediately after `setData()`, enhancing the fluidity and readability of your code.

"this" in Lambda Expressions
Lambda expressions in C++ allow you to create anonymous functions, and understanding how to capture `this` within these lambdas can significantly enhance your coding capabilities, especially when dealing with callbacks.
Capturing "this"
When you capture `this` inside a lambda, you gain access to the instance variables and methods of the enclosing class. This is particularly useful in asynchronous programming or when passing functions as arguments.
Consider the following example:
class Counter {
private:
int count = 0;
public:
void increment() {
auto lambda = [this]() { this->count++; }; // Capturing 'this'
lambda(); // Calling the lambda function
}
void display() {
std::cout << count << std::endl; // Displaying the count value
}
};
// Usage
Counter counter;
counter.increment();
counter.display(); // Outputs: 1
In this scenario, the lambda captures the `this` pointer, allowing it to increment the `count` member variable directly. This encapsulation simplifies the code and keeps the context intact, which is vital for maintaining state in complex applications.
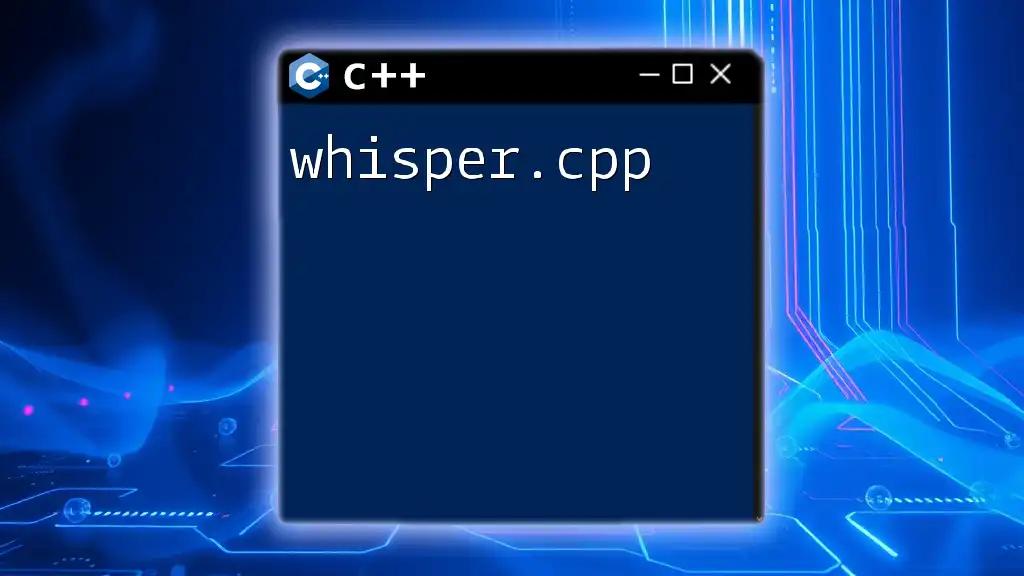
"this" in Operator Overloading
Another significant use of `this` occurs within operator overloading. When you overload operators, your functions begin to manipulate class objects, and `this` serves as the conduit through which these instances are accessed.
Facilitating Operator Functions
Here’s how you can overload the addition operator for a simple `Point` class:
class Point {
private:
int x, y;
public:
Point(int x, int y) : x(x), y(y) {}
Point operator+(const Point& other) {
return Point(this->x + other.x, this->y + other.y); // Using 'this' to access members
}
};
// Usage
Point p1(1, 2);
Point p2(3, 4);
Point p3 = p1 + p2; // Overloaded + operator
In this example, when you use `p1 + p2`, it invokes the overloaded `operator+`, which utilizes `this` to refer to the first `Point` object and access its member variables clearly. This allows for intuitive and concise mathematical operations on objects, elevating code readability and expressiveness.

Common Misconceptions About "this"
Despite its utility, various misunderstandings surrounding `this` can lead to coding errors.
Misuse of "this" Keyword
Common pitfalls include:
- Using `this` in static member functions: Since these functions do not have access to class instance data, they cannot use `this`.
- Overuse in trivial cases: While `this` can enhance clarity, its unnecessary use in straightforward code can clutter logic.
Best Practices
To effectively utilize `this`, adhere to these guidelines:
- Use `this` when you need to resolve naming conflicts.
- Capture `this` in lambdas when referring to member variables or functions.
- Incorporate `this` in operator overloading to improve method readability.
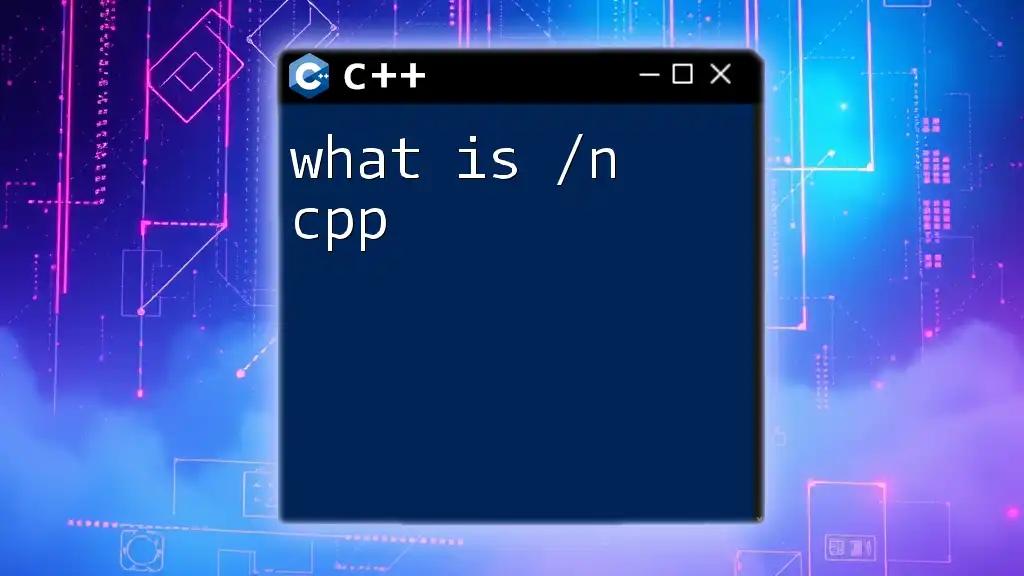
Conclusion
In conclusion, the `this` keyword in C++ is a powerful tool that enhances object-oriented programming by allowing developers to reference the current object instance, avoid naming conflicts, and streamline method chaining. Understanding how and when to use `this` equips you to write cleaner, more efficient, and more maintainable code.
As you practice implementing these concepts in your projects, you'll discover the flexibility and clarity that `this` brings to your C++ programming. Don’t hesitate to reach out with your experiences or questions as you navigate your coding journey!