The Standard Template Library (STL) in C++ provides a collection of template classes and functions for common data structures and algorithms, enabling developers to write efficient and reusable code.
Here's a simple example of using a vector from the STL:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is the Standard Template Library in C++?
Definition of STL
The Standard Template Library (STL) is an essential part of modern C++ programming. It provides a rich set of ready-made classes and functions that significantly ease the development process by offering standard solutions for common programming tasks. STL emphasizes the use of templates, which allows developers to create generic and reusable code components that can work with any data type.
Benefits of Using STL
Using STL offers several key advantages that can help any developer:
-
Code Reusability: One of the most significant advantages of STL is its ability to promote efficient coding practices. Developers can utilize pre-built components instead of reinventing the wheel, thereby saving time and effort.
-
Performance Improvements: STL containers and algorithms are optimized to perform better than many manually implemented data structures. They are designed to handle large data sets efficiently.
-
Consistency: STL encourages consistency across the codebase since developers can rely on standard data structures and algorithms. This uniformity simplifies maintenance and teamwork.
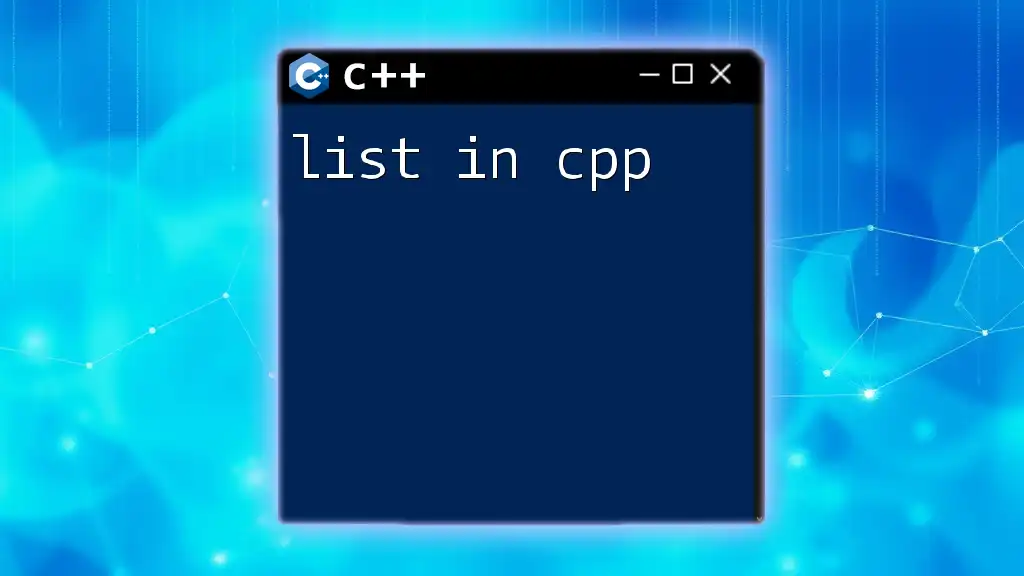
Components of C++ Standard Template Library
Containers
Containers are essential components of STL, acting as data structures that store collections of data. They provide a unified interface for handling data, making it easy to create and manipulate groups of related items.
Definition and Purpose
Containers encapsulate data so that programmers can store, retrieve, and manipulate large sets of elements easily.
Types of Containers
-
Sequence Containers:
- Examples include `std::vector`, `std::list`, and `std::deque`. These allow for storing collections of items in a specific order.
- For instance, a vector is a dynamic array that can resize itself automatically and provides random access capabilities.
-
Associative Containers:
- These containers, such as `std::map` and `std::set`, maintain sorted order and allow fast retrieval based on keys.
- They provide associations between keys and values, making them ideal for look-up operations.
-
Unordered Containers:
- Introduced in C++11, containers like `std::unordered_map` and `std::unordered_set` offer fast access to elements based on a hash table structure.
- They are useful when you need efficient search capabilities without the necessity for sorting.
Examples and Snippets
Here’s a simple example demonstrating how to use a vector to store integers:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5
}
return 0;
}
Algorithms
STL algorithms provide ready-made methods for manipulating and accessing data stored in containers. They streamline operations like sorting, searching, and transforming data.
Understanding C++ STL Algorithms
These algorithms operate on ranges defined by iterators, making them highly flexible. You can apply algorithms to any container type, as long as the container's elements are compatible with the operation.
Commonly Used Algorithms
- Sorting: `std::sort` allows you to arrange elements in order.
- Searching: Use `std::find` to search for an element in a container.
- Transforming: `std::transform` enables you to apply a function to each element in a range.
Example Usage
Here's an example of sorting a vector of integers:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 2, 4, 1};
std::sort(numbers.begin(), numbers.end());
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5
}
return 0;
}
Iterators
Iterators are pivotal to STL, functioning as generalized pointers that enable navigation through container elements.
What is an Iterator?
They abstract the details of container traversal. Instead of dealing with specific container types, iterators provide a common interface for manipulation.
Types of Iterators
- Input Iterators: Read-only access to elements.
- Output Iterators: Write-only access.
- Forward Iterators: Allow reading/writing in a single pass.
- Bidirectional Iterators: Support movement in both directions.
- Random Access Iterators: Provide access similar to array indexing.
Example: Using Iterators with Containers
The following code demonstrates how to use iterators with a `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " "; // Output: 10 20 30 40 50
}
return 0;
}
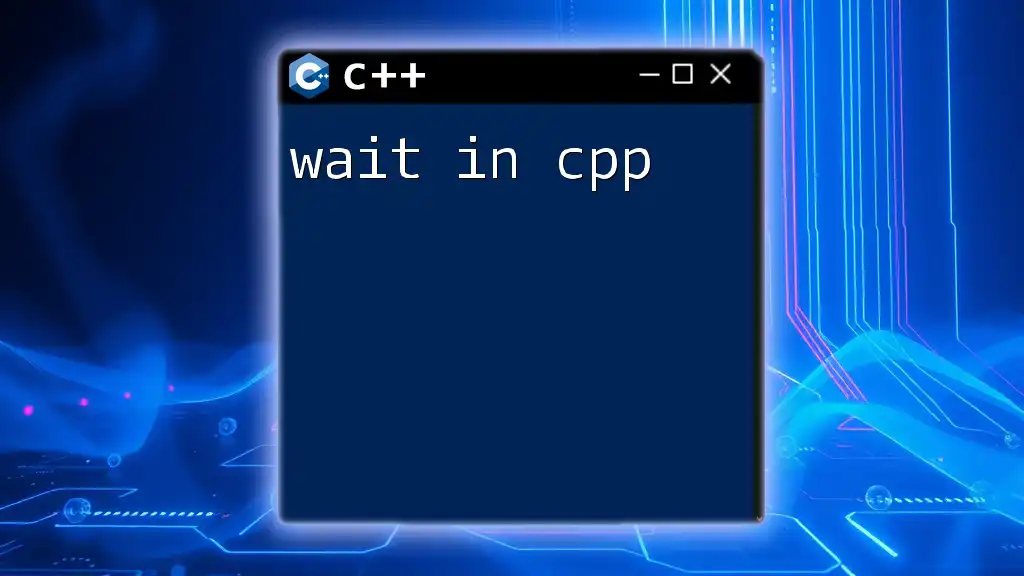
Working with the STL
How to Include STL in Your Code
To utilize STL, simply include the necessary headers for the containers or algorithms you plan to use. For example, you can include `<vector>`, `<algorithm>`, and `<map>` as needed.
Best Practices When Using STL
-
Choosing the Right Container: Understand the usage scenarios for each container type to optimize performance and memory. For example, opt for `std::vector` for dynamic arrays, and `std::map` for sorted key-value pairs.
-
Efficient Use of Algorithms: Familiarize yourself with the available algorithms and choose the right one for the task at hand. Apply STL algorithms instead of manually implementing features whenever feasible.
-
Memory Management: STL containers are generally efficient; however, it’s crucial to understand their memory usage implications. Containers like `std::vector` dynamically adjust their size but can incur overhead.
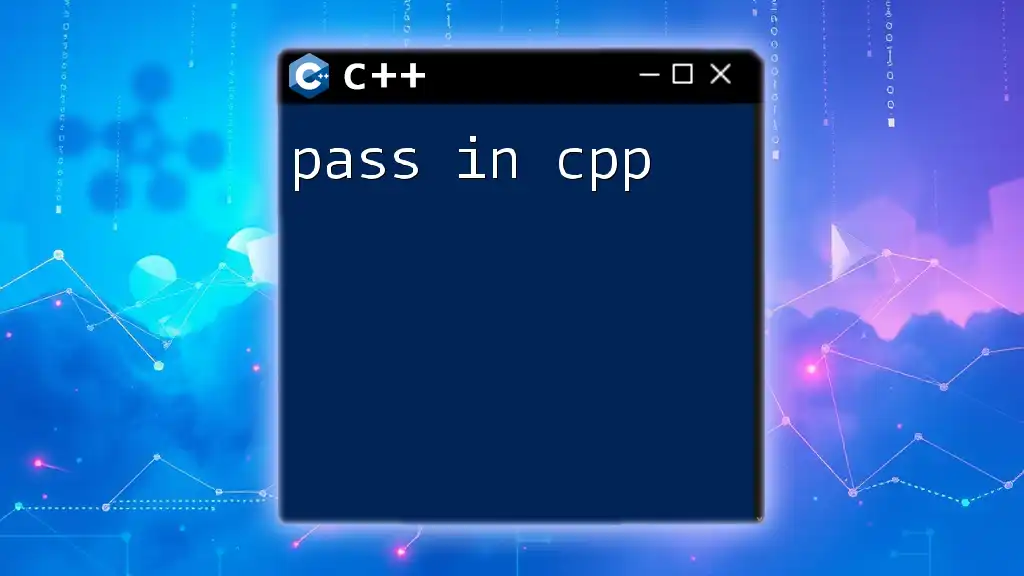
Common Misconceptions about STL
Performance Myths
Some developers falsely believe using STL slows down their programs. In reality, STL components are often more efficient than custom implementations because they utilize well-tested algorithms optimized for performance.
Learning Curve
While STL may appear complex initially, its learning curve is manageable. Once familiar with its components, developers often find that STL enhances productivity and code quality. Numerous resources are available for further learning, making mastering STL attainable.
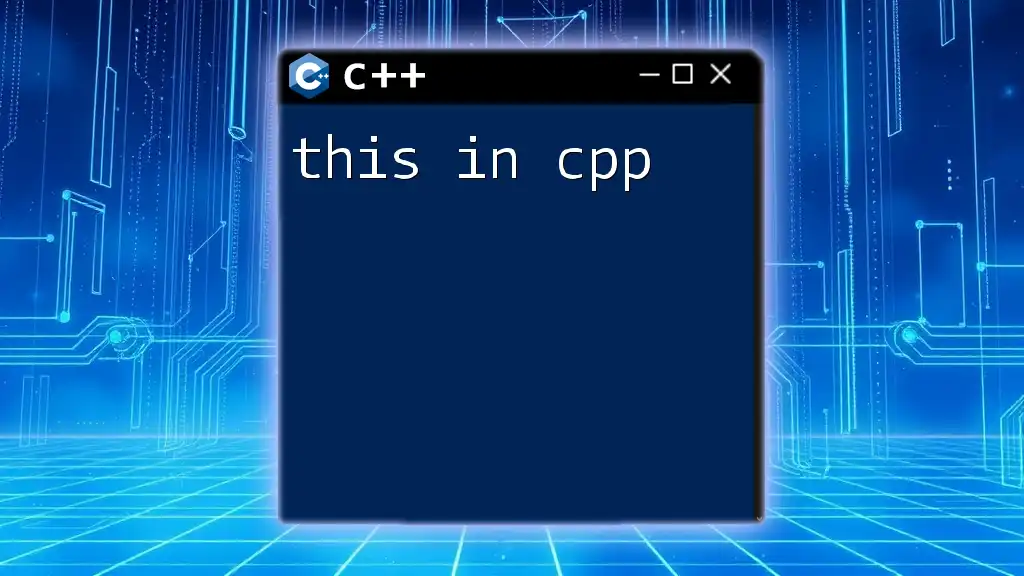
Conclusion
Understanding the STL in C++ is a crucial skill for any programmer. It simplifies many programming tasks and enhances development speed through the use of efficient, generic components. Begin integrating STL into your programming practices to leverage its full potential. Join our courses to further explore the wonders of STL and elevate your C++ skills!
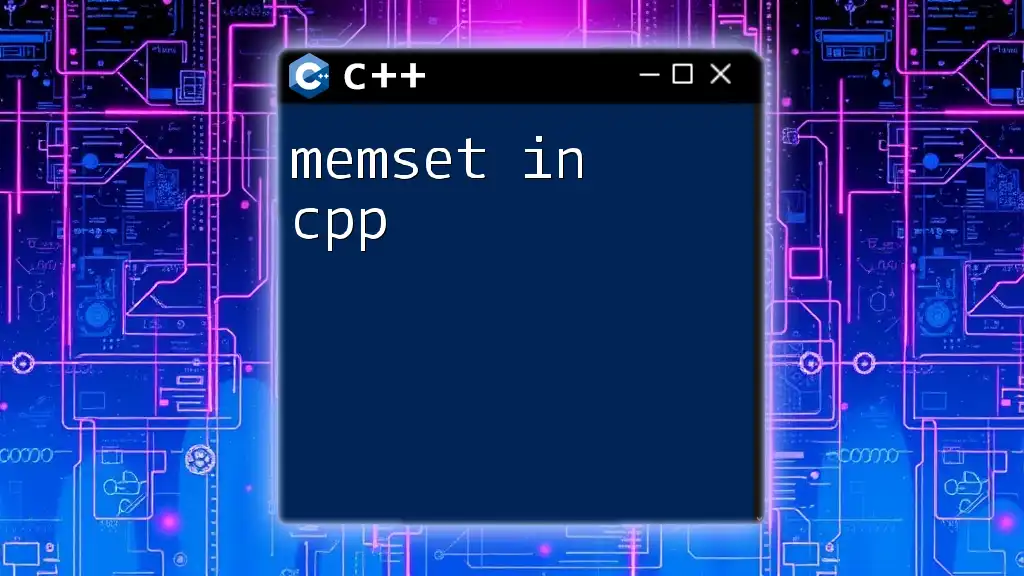
Additional Resources
Explore reputed books and websites dedicated to STL for comprehensive learning. Engaging with online courses and tutorials can also provide valuable insights. Don't forget to connect with community forums for ongoing support and learning opportunities in the vast world of C++.