In C++, a `map` is an associative container that stores elements as key-value pairs, allowing for fast retrieval based on the key.
Here’s a simple example of how to use a `map` in C++:
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
for (const auto& pair : ageMap) {
std::cout << pair.first << " is " << pair.second << " years old." << std::endl;
}
return 0;
}
What is a `map`?
A map in C++ is a container that stores elements as key-value pairs. These pairs allow you to associate unique keys with specific values. With a map, you can access values efficiently using their keys, making it a vital tool for a variety of programming scenarios. Unlike other containers like vectors or arrays, maps automatically arrange the elements in a sorted manner, based on the key.
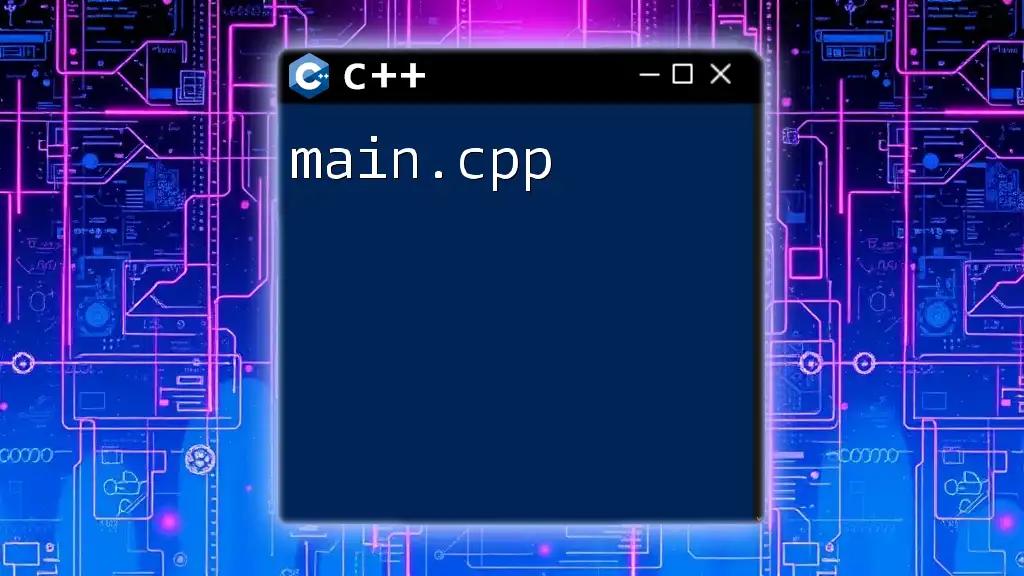
Key Characteristics of `map`
When utilizing a map in C++, it's essential to understand its unique characteristics:
-
Ordered vs Unordered: In C++, `std::map` stores items in sorted order based on keys, while `std::unordered_map` does not maintain any specific order.
-
Key-Value Pairs: Each entry in a map consists of a key and its corresponding value. The key is unique, meaning you cannot have two identical keys in the same map.
-
Unique Keys: Maps internally manage their data by ensuring that each key can only appear once. If you attempt to insert a duplicate key, the map will not add it or will overwrite the existing value associated with that key.

Map Syntax in C++
To start using a map in C++, you need to include the necessary header file.
#include <map>
You can then declare a map. For example, here’s how you can create a map that holds integer keys and string values:
std::map<int, std::string> myMap;

Creating and Initializing a `map`
Default Constructor
To create an empty map, you can simply use the default constructor:
std::map<int, std::string> myMap;
Initializing with Values
You can initialize a map with values using an initializer list:
std::map<int, std::string> myMap = {{1, "Apple"}, {2, "Banana"}};
Using `std::make_pair`
Another method to add entries to a map is by using `std::make_pair`:
myMap.insert(std::make_pair(3, "Cherry"));
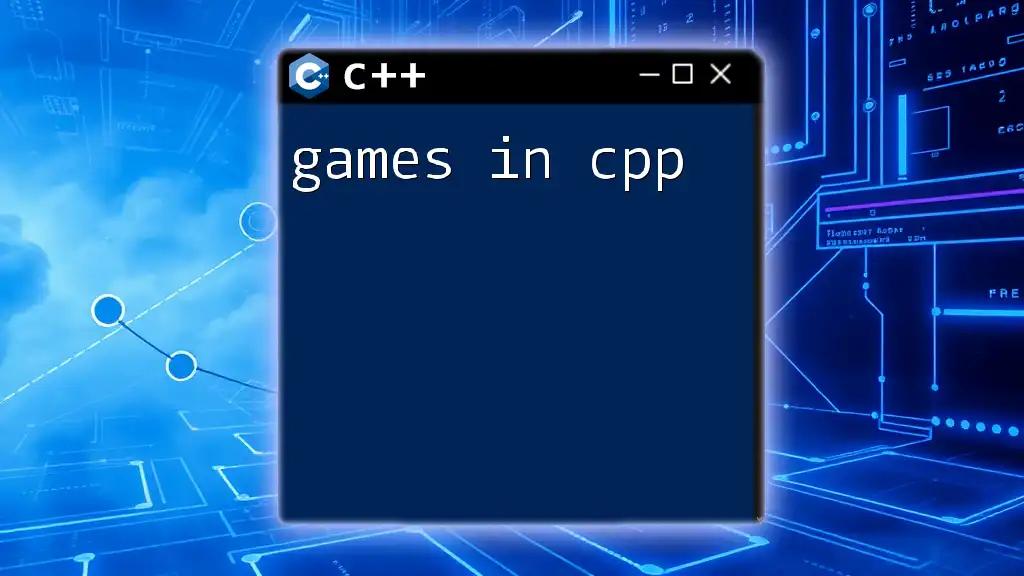
Operations on `map`
Inserting Elements
The map in C++ provides several methods to insert elements.
- Using the `insert` method:
myMap.insert({4, "Date"});
- Using the `[]` operator: This operator enables direct access to the map elements.
myMap[5] = "Elderberry";
Accessing Elements
Accessing elements in a map is straightforward and can be done in multiple ways.
- Using Iterators:
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
// Accessing key and value
std::cout << it->first << " : " << it->second << std::endl;
}
- Using `at` function: This method safely retrieves values as it checks whether the key exists.
std::cout << myMap.at(1); // Outputs: Apple
Erasing Elements
To remove elements from a map, you can use the `erase` method:
myMap.erase(2); // Removes the key-value pair with key 2
Finding Elements
You can search for elements using the `find` method:
auto it = myMap.find(3);
if (it != myMap.end()) {
std::cout << "Found: " << it->second; // Outputs: Found: Cherry
}

Advanced Features of `map`
Iterating with Range-Based For Loop
C++ allows for a more concise iteration method using a range-based for loop:
for (const auto& pair : myMap) {
std::cout << pair.first << " : " << pair.second << std::endl;
}
Custom Comparators
C++ maps also allow the use of custom comparators for managing the order of keys.
struct CustomCompare {
bool operator() (const int& a, const int& b) const {
return a > b; // Reverse order
}
};
std::map<int, std::string, CustomCompare> customMap;
Multi-Map
A `std::multimap` is another variant that allows multiple values for the same key. This is especially useful when there are inherent duplicates in the data.
std::multimap<int, std::string> multiMap;
multiMap.insert({1, "Apple"});
multiMap.insert({1, "Avocado"}); // Allows duplicate keys
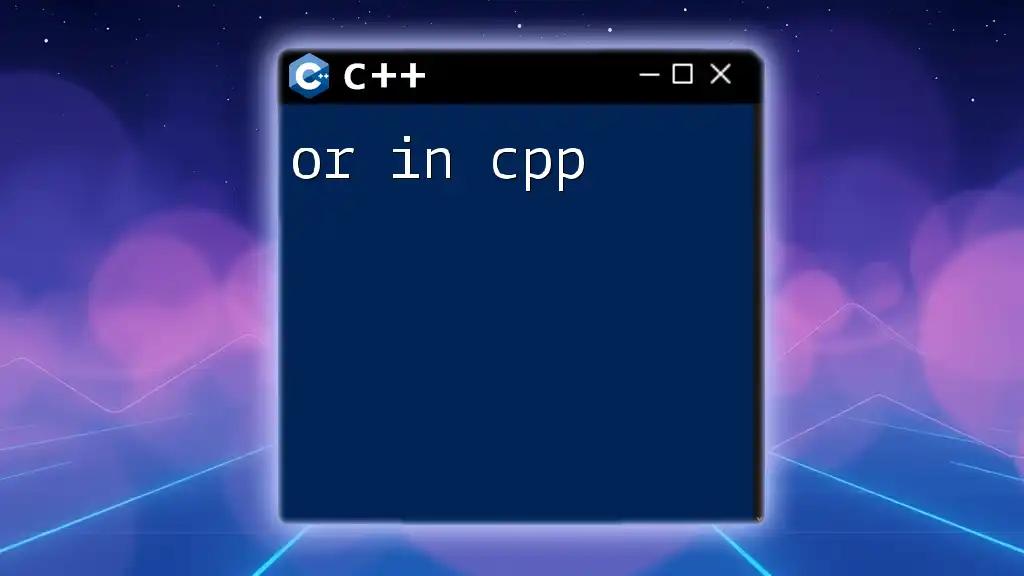
Best Practices for Using `map`
When working with a map in C++, it’s crucial to consider when to choose a map over other data containers. Factors include expected performance, the need for key uniqueness, and specific usage patterns, such as frequent insertions or lookups.
Avoiding Common Pitfalls
Be cautious about key collisions; if you inadvertently insert a value with a duplicate key, your previous value will be overwritten. Also, be aware that iterators can become invalidated under certain operations, such as when keys are erased.
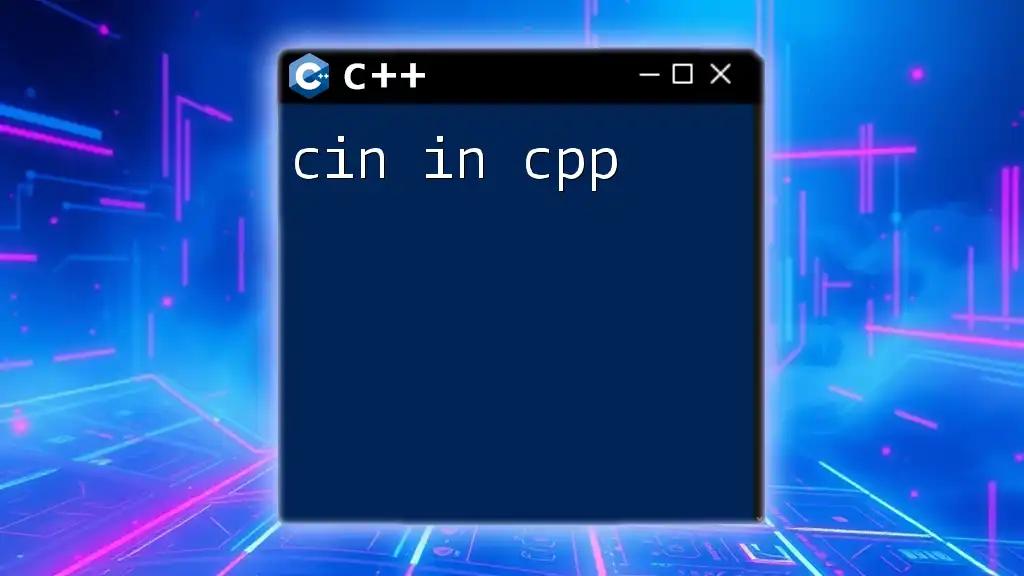
Conclusion
In summary, a map in C++ provides a powerful and efficient way to store and access data through key-value pairs. By understanding its features, usage patterns, and best practices, you can leverage maps to enhance your data management abilities in C++ programming. For further exploration, consider diving into official C++ documentation or recommended books that cover these topics in-depth.
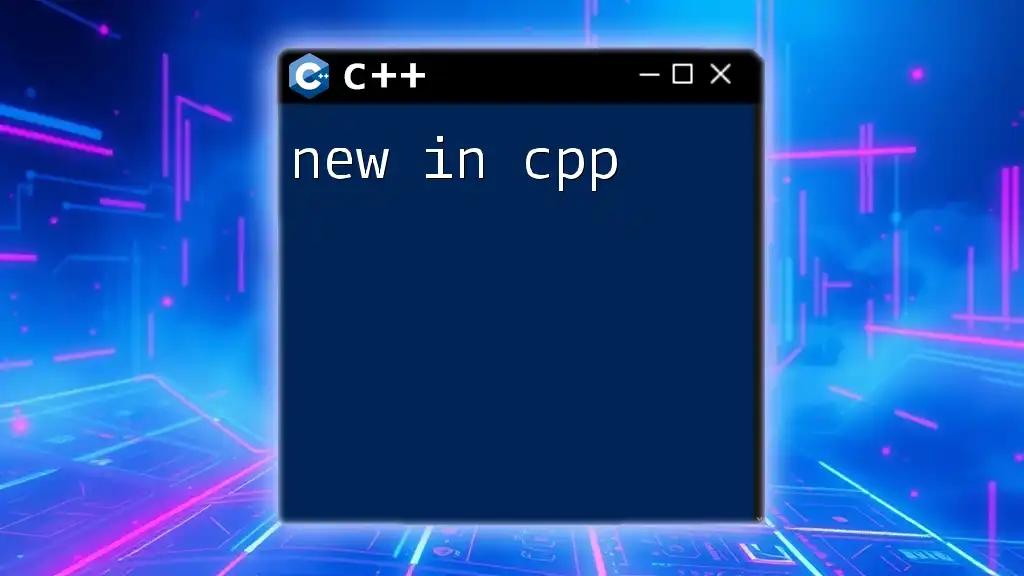
Code Snippets Repository
For practical implementations and examples of using `map` in C++, please refer to the accompanying code snippets repository or GitHub link.