In C++, exponents can be calculated using the `pow` function from the `<cmath>` library, which raises a number to a specified power. Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << "Result: " << result << std::endl; // Output: Result: 8
return 0;
}
Understanding Exponents in C++
What are Exponents?
Exponents are a mathematical construct that represents the number of times a base is multiplied by itself. For example, in the expression \( 2^3 \) (read as "two raised to the power of three"), the base is 2, and the exponent is 3, resulting in \( 2 \times 2 \times 2 = 8 \).
Exponents play a crucial role in various computations in programming, particularly in areas such as scientific calculations, simulations, and algorithms involving mathematical models.
Why Use Exponents in C++?
Using exponents in C++ allows programmers to perform mathematical operations efficiently without the need to manually multiply numbers repeatedly. For example, exponents are essential in calculations involving physics simulations, financial models, and even machine learning algorithms. Knowing how to effectively use exponents can make your code more elegant and maintainable.
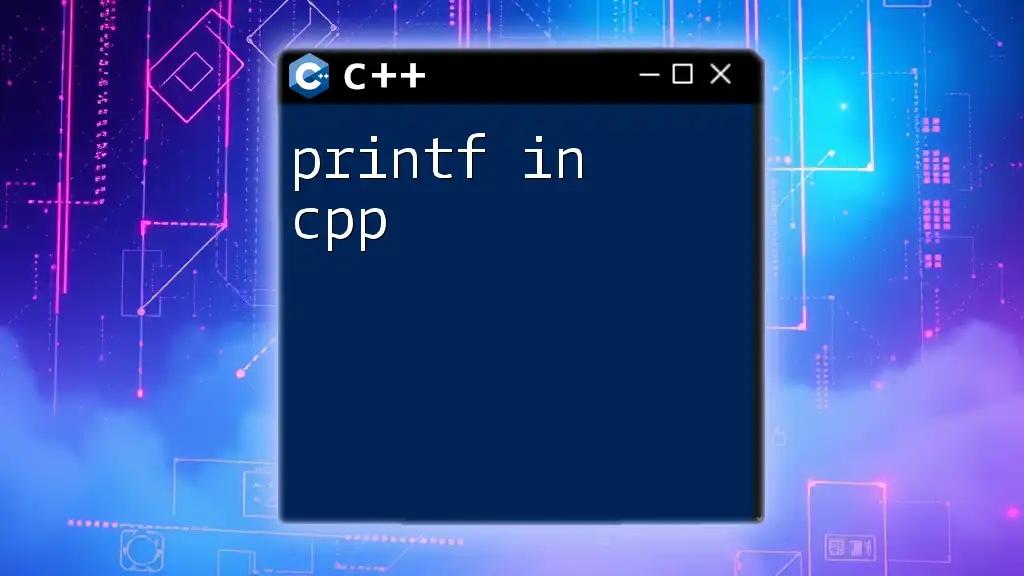
The C++ Power Operator
Introduction to the C++ pow Operator
In C++, the `pow` function is the primary utility for performing exponentiation. Found in the `<cmath>` header, it takes in two arguments: a base and an exponent, neatly encapsulating the logic for calculating powers in a single function call.
Syntax of the pow Function
The `pow` function is declared as follows:
double pow(double base, double exponent);
Here, both the base and exponent can be floating-point numbers, allowing for a versatile range of calculations. The function returns the result as a double.
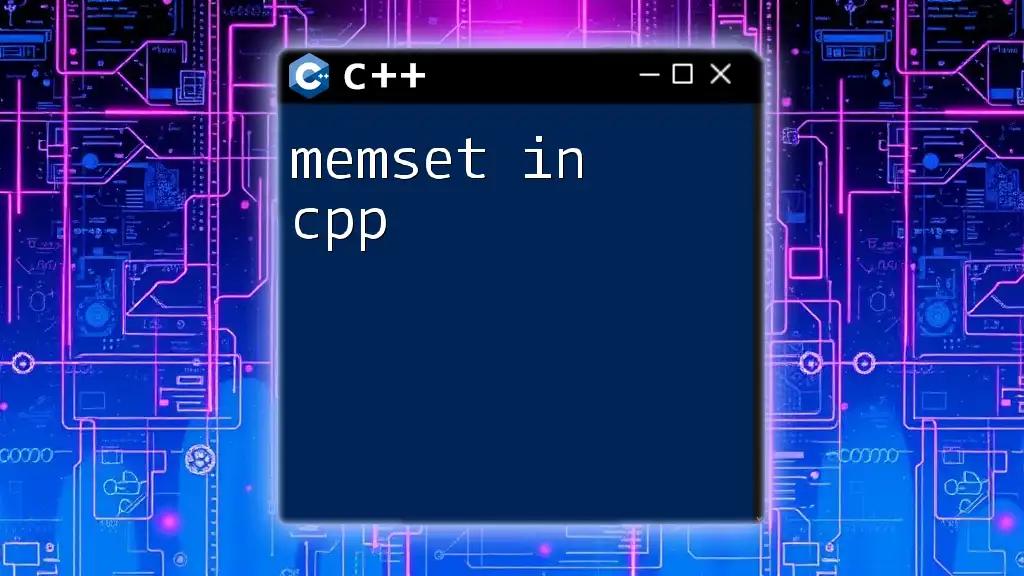
How to Use pow in C++
Importing the Required Header
Before you can use the `pow` function, you must include the `<cmath>` header at the top of your C++ file. Including this header gives you access to the various mathematical functions provided by C++.
Here’s a typical way to include the header:
#include <iostream>
#include <cmath>
Basic Examples of Using pow
Let’s look at a basic example to illustrate how the `pow` function works:
#include <iostream>
#include <cmath>
int main() {
double result = pow(2, 3); // 2 raised to the power of 3
std::cout << "2^3 = " << result << std::endl; // Output: 8
return 0;
}
In the code above, we calculate \( 2^3 \) and display the result. The expected output is 8, demonstrating the function's functionality in a straightforward manner.

C++ Power Operator: More Advanced Usage
Working with Integer Exponents
Using integer values with the `pow` function is straightforward. For instance:
int base = 5;
int exponent = 2;
std::cout << "5^2 = " << pow(base, exponent) << std::endl; // Output: 25
This snippet reveals the result of raising 5 to the power of 2, yielding 25.
Handling Negative Exponents
Understanding how to work with negative exponents is equally important. Negative exponents signify a reciprocal operation. For example, \( 2^{-3} \) can be computed as follows:
std::cout << "2^-3 = " << pow(2, -3) << std::endl; // Output: 0.125
This example displays the result of \( 2^{-3} \), which equals 0.125, showcasing the `pow` function’s capability to handle negative values.
Using Floating Point Values
The `pow` function excels with floating-point numbers too. You could raise 3.5 to the power of 2.2 using:
std::cout << "3.5^2.2 = " << pow(3.5, 2.2) << std::endl; // Outputs a decimal
C++ will accurately compute this expression and return the result as a decimal, leveraging the floating-point capabilities of the function.

Working with Exponents in C++
Alternative Power Functions in C++
While the `pow` function is convenient, it may not always be the most efficient option, especially for integer exponentiation. A manual loop-based method can be implemented as follows:
double manual_pow(double base, int exp) {
double result = 1;
for (int i = 0; i < exp; ++i)
result *= base;
return result;
}
This function iteratively multiplies the base by itself for the specified exponent, giving you more control over performance optimizations for integer calculations.
Performance Considerations
When working with exponentiation, consider when to use `pow` versus manual exponentiation. While `pow` is a general-purpose function and works with all numerical types, it may carry additional overhead in certain cases. If your code focuses primarily on integer math, opting for a loop-based approach may yield better performance.
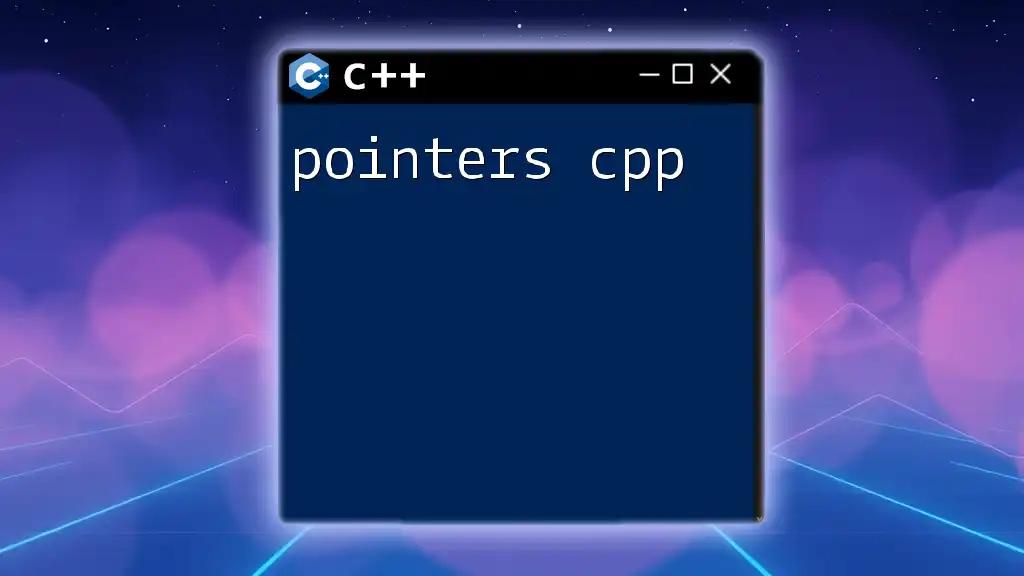
Common Errors and Troubleshooting
Understanding Potential Pitfalls
Common errors when using the `pow` function often relate to mismatched types; for instance, trying to supply a non-numeric type can lead to compilation errors. Always ensure that both parameters are of the correct data type to avoid these issues.
Rounding Errors in Floating-Point Calculations
When dealing with floating-point numbers, rounding errors may arise due to the imprecise representation of decimal numbers in binary. Be mindful of potential inaccuracies, especially when working with large exponents or when high precision is required.

Conclusion
Recap of Exponential Functions in C++
In summary, understanding exponents in C++ through the `pow` function empowers developers to handle a wide array of mathematical operations efficiently. Whether working with integers, negative values, or floating-point numbers, the `pow` function provides versatility and ease of use.
Further Learning
For those aiming to deepen their knowledge, consider exploring additional resources on C++ programming techniques, and practice through exercises that involve calculating powers and applying them in various contexts. Experimenting with exponents will enhance your coding proficiency and expand your understanding of numerical computations in C++.