The `assert` macro in C++ is used to perform runtime assertion checks, providing a way to verify assumptions made by the program and terminate the program if the assumption is false.
#include <cassert>
int main() {
int x = 5;
assert(x == 5); // This assertion will pass
assert(x == 10); // This assertion will fail and terminate the program
return 0;
}
What is Assert?
In C++, assert is a powerful mechanism used for debugging that runs a test during program execution, allowing developers to verify assumptions made in the code. The primary purpose of assertions is to catch programming errors early, thereby improving code quality and reliability.
The Role of Assertions in C++ Development
Assertions are not just another layer of error handling; they are a way of stating assumptions made by the programmer. They signal to both the developer and anyone else reading the code about conditions that should always be true during execution. If an assertion fails, it indicates a significant issue in the code that needs immediate attention. Unlike regular error handling mechanisms, assertions can clarify intended behavior, which helps create self-documenting code.
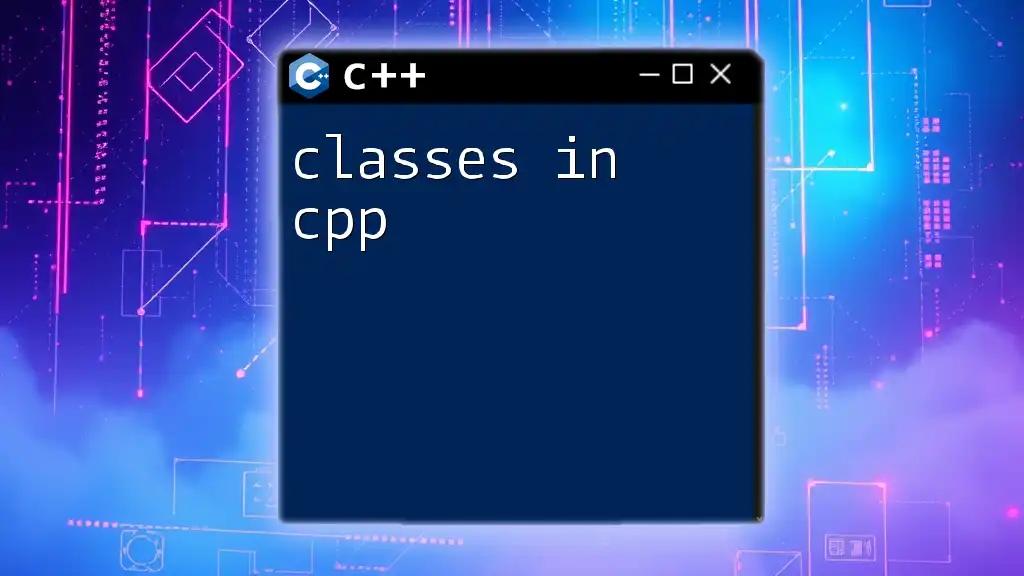
Understanding the Assert Function in C++
The assert Function Signature
The assert function is defined in the `<cassert>` header and has the following signature:
void assert(bool expression);
Here, the expression is a condition that the developer expects to be true. If the expression evaluates to false, the assert function will trigger, halting the program and reporting an error.
Using Assert in C++
Basic Usage of the Assert Statement C++
Using assert is straightforward. Here’s a simple example demonstrating its basic usage:
#include <cassert>
void checkPositive(int number) {
assert(number > 0);
}
In this example, if `checkPositive` is called with a negative number, the program will terminate, indicating a failed assertion. This helps ensure that the function always receives valid inputs.
Common Use Cases for Assert in C++
Assertions can be used in various scenarios:
- Input validation: Ensuring function parameters meet required conditions before proceeding with execution.
- Debugging complex algorithms: Validating intermediate results to ensure algorithms function correctly.
- Ensuring code preconditions: Establishing that certain conditions hold true before executing critical code blocks.
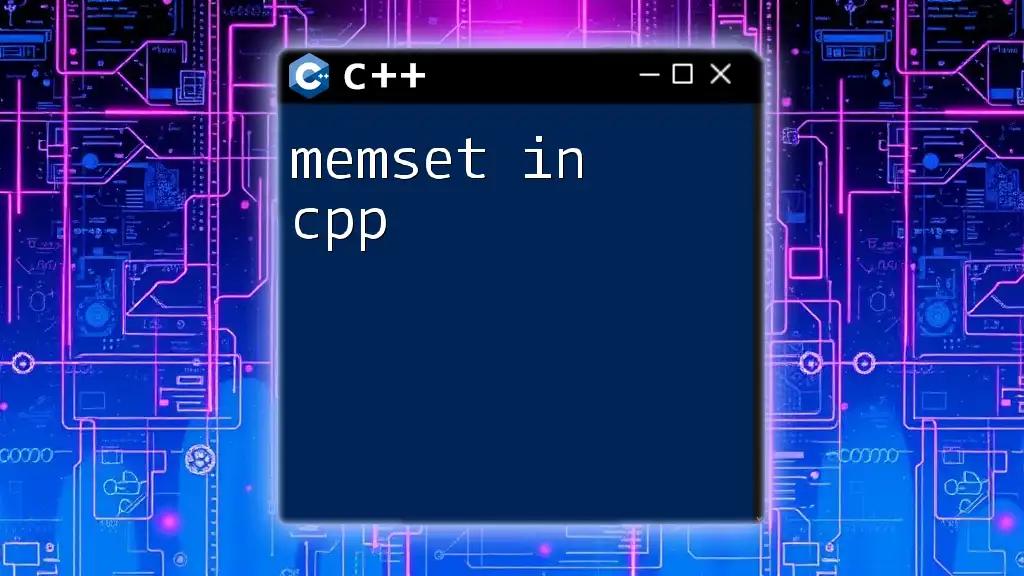
How to Implement Assert in Your C++ Projects
Enabling and Disabling Assertions
The assert mechanism can be influenced by the NDEBUG macro. By defining NDEBUG before including `<cassert>`, all assert statements are ignored in the program. This feature is particularly useful when transitioning from development to production:
#define NDEBUG // Disable assertions
#include <cassert>
void test() {
assert(2 + 2 == 5); // This won't trigger if NDEBUG is defined
}
Best Practices for Using C++ Assertions
For optimal use of assertions in your code, consider the following best practices:
- Timing of assertions: Use asserts primarily during development. Avoid relying on them in production code where error handling should be a priority.
- Meaningful assert statements: Write assert statements that clearly communicate the expected conditions. This improves readability and assists in debugging.

Advantages of Using Assert in C++
Improved Code Quality
One of the main advantages of using assert in C++ is that it aids in catching bugs early in the development process. By actively validating program logic, developers can identify and fix issues before they escalate into larger problems.
Declarative Debugging
Assertions promote a declarative approach to debugging. They differentiate errors in logical assumptions from run-time errors, making it easier to narrow down the source of bugs. Additionally, assert statements can make code more self-documenting by providing contextual information about the expected behavior of the code.
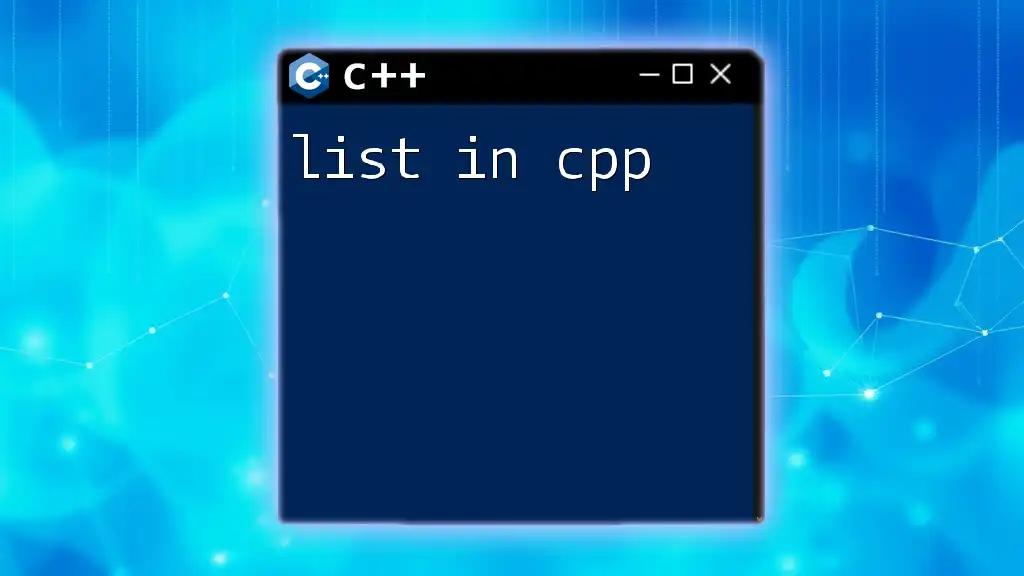
Limitations and Misuse of Assert in C++
When Not to Use Assert in C++
Despite their advantages, assertions should not replace traditional error handling techniques. They should never be used for validating user input or handling exceptional conditions in a production environment, as assertions are intended strictly for debugging purposes. Additionally, relying on assertions in production code can lead to performance issues if they remain active due to frequent checks.
Common Misconceptions about C++ Assertions
A common misconception is that assertions can be used in place of exceptions for error handling. While both mechanisms serve to indicate errors, assertions are meant for situations that should logically never occur. They are not a substitute for robust error handling in situations where runtime failures can happen.
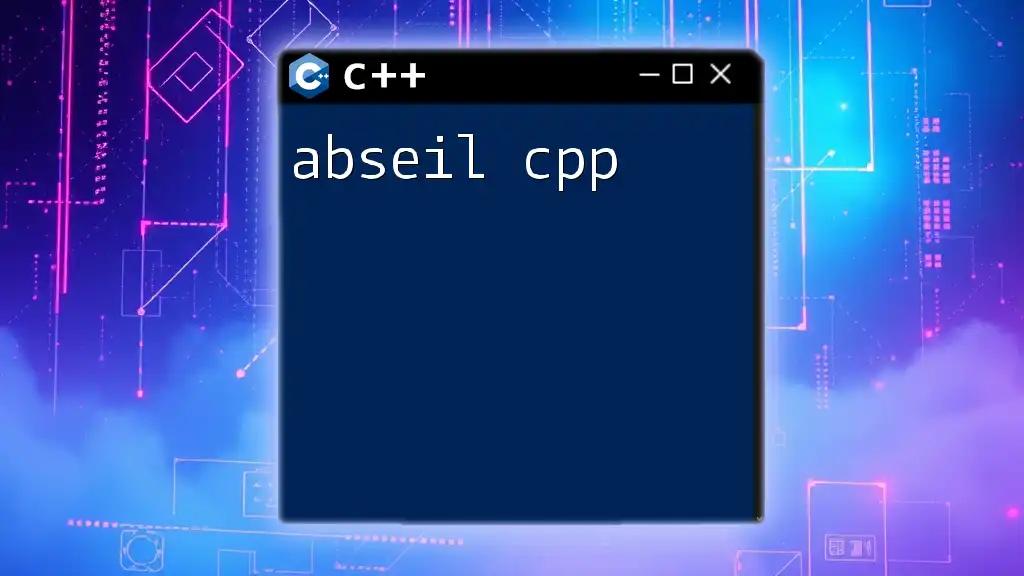
Advanced Topics Related to Assert in C++
Custom Assertion Macros
For greater flexibility, developers can create custom assertion macros to include additional logic or messages. Here’s an example of a custom assert function:
#define CUSTOM_ASSERT(condition, message) \
do { \
if (!(condition)) { \
fprintf(stderr, "Assertion failed: %s\n", message); \
abort(); \
} \
} while (0)
This macro facilitates a customized error message output when an assertion fails, enhancing the debug process by providing more context.
Using Assertions with Multithreading
When using assertions in multithreaded applications, challenges arise in assessing the thread safety of assert statements. It is essential to ensure that the conditions checked by assertions accurately reflect the state of the shared resources across multiple threads, preventing false negatives or positives.
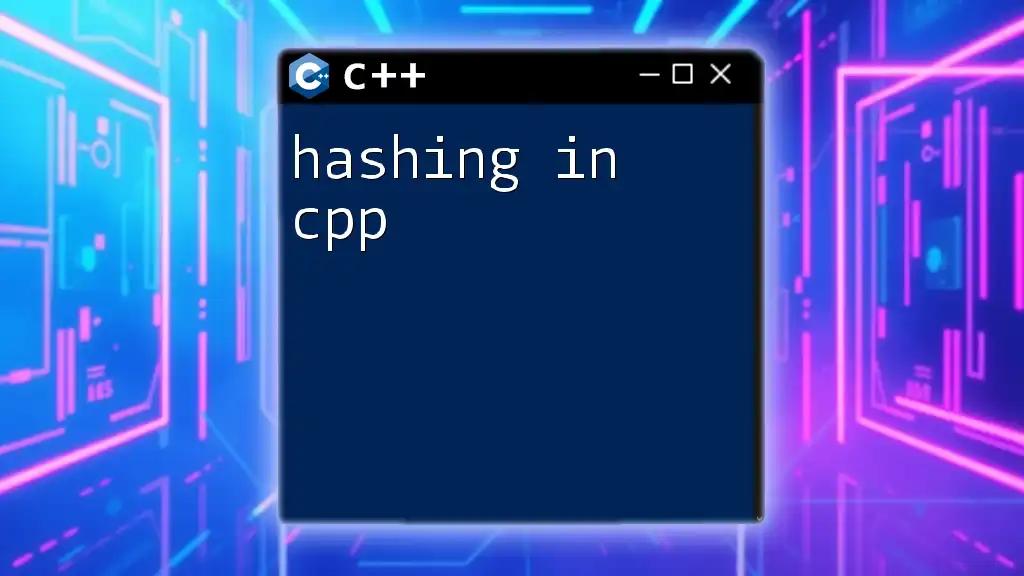
Conclusion
Assert in C++ is a valuable tool in a developer's arsenal for improving code reliability and debugging efficiency. Understanding its proper application and limitations is crucial for effective software development. By incorporating assertions thoughtfully, programmers can create more maintainable, robust, and error-resistant C++ applications.

Further Resources and Learning
For readers interested in a deeper understanding of C++ assertions and debugging, consider exploring advanced C++ programming books, online courses, or official documentation that provides comprehensive insights into effective debugging practices.
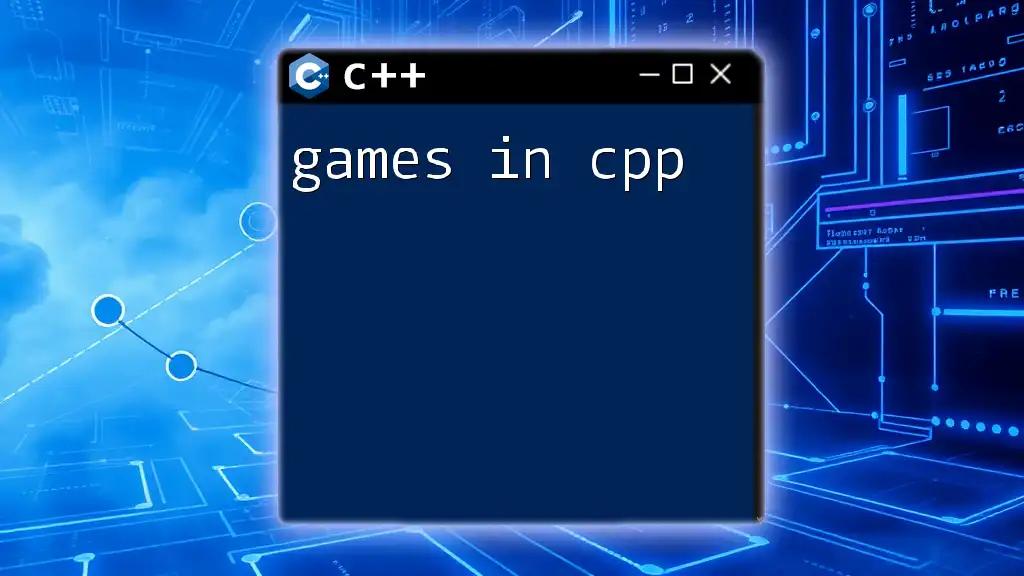
Call to Action
Join our community of enthusiastic programmers and gain access to more resources on C++ programming, attend workshops, and participate in discussions to enhance your knowledge and skills in using assert effectively.