The `app_httpd.cpp` file typically serves as a simple HTTP server implementation in C++, allowing users to handle HTTP requests and responses efficiently.
Here’s a code snippet demonstrating a basic HTTP server in C++:
#include <iostream>
#include <boost/asio.hpp>
using namespace boost::asio;
int main() {
io_service ios;
ip::tcp::acceptor acceptor(ios, ip::tcp::endpoint(ip::tcp::v4(), 8080));
while (true) {
ip::tcp::socket socket(ios);
acceptor.accept(socket);
std::string message = "HTTP/1.1 200 OK\r\nContent-Length: 13\r\n\r\nHello, World!";
boost::asio::write(socket, buffer(message));
}
return 0;
}
Setting Up Your Environment
Prerequisites
Before diving into `app_httpd.cpp`, ensure you have the necessary software components installed on your development machine. These typically include:
- C++ Compiler: Ensure you have a modern C++ compiler like GCC or Clang. They support C++11 and beyond, which is often required for working with contemporary C++ code.
- Library Dependencies: Familiarize yourself with any libraries that are essential for your server, such as Boost.Asio for handling asynchronous operations.
- Development Tools: Consider using an Integrated Development Environment (IDE) such as Visual Studio, Code::Blocks, or even text editors like VSCode or Sublime Text for a streamlined coding experience.
Installation Steps
-
Installing the compiler and libraries: Follow the instructions for your operating system to install the compiler and required libraries. For example, on Ubuntu, you would typically run:
sudo apt-get install g++ libboost-all-dev
-
Setting up your project folder: Create a dedicated directory for your project. In this folder, you can create `app_httpd.cpp` and any other associated files.
-
Verifying the installation: Compile a simple C++ program to ensure everything is set up correctly:
#include <iostream> int main() { std::cout << "Setup successful!" << std::endl; return 0; }
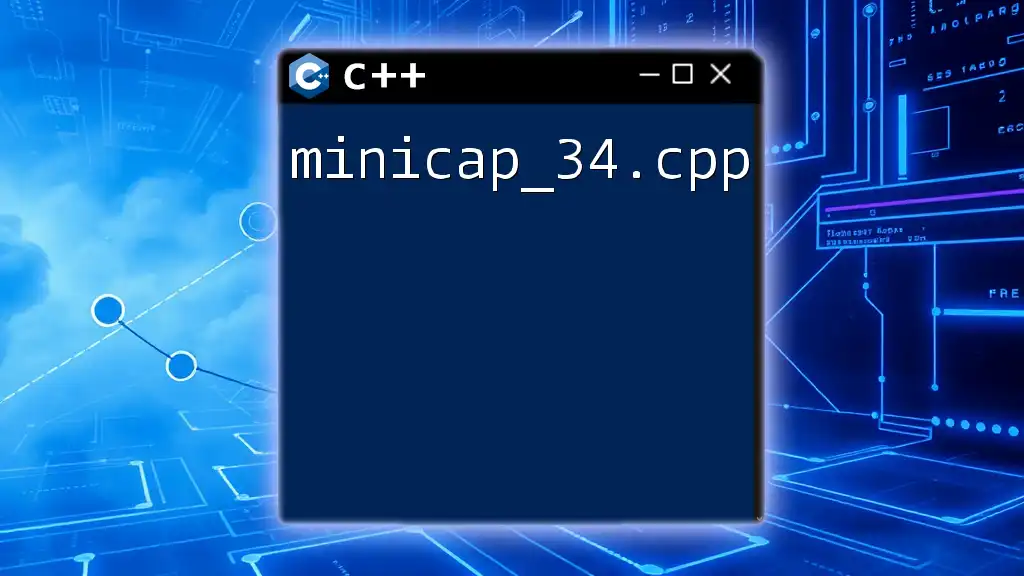
Understanding the Structure of `app_httpd.cpp`
File Structure Overview
Understanding the layout of `app_httpd.cpp` will aid in navigating and modifying the code efficiently. The file typically comprises:
- Headers: Necessary library inclusions.
- Namespace: Likely containing core functionalities related to your HTTP server.
- Main Class: The backbone of your server's functionality.
Key Classes and Functions
Main Class
The main class is where all the action occurs. It typically contains critical methods for starting the server and processing requests. Here’s an example structure for the main class:
class HttpServer {
public:
void start();
void handleRequest(const Request& req, Response& res);
// Additional methods as required
};
This structure illustrates the essential functionality, where `start` initiates the server, and `handleRequest` processes incoming requests.
Routing Mechanism
Routing is crucial in directing client requests to the appropriate handlers based on the requested URL. A simple routing implementation might look like this:
void HttpServer::handleRequest(const Request& req, Response& res) {
if (req.getPath() == "/") {
res.send("<h1>Welcome to my server!</h1>");
} else if (req.getPath() == "/about") {
res.send("<h1>About Us</h1>");
} else {
res.send("<h1>404 Not Found</h1>");
}
}
This code snippet illustrates basic routing logic based on URL paths, serving different content based on the request.
Request Handling
Handling HTTP requests systematically is key to a functioning server. The following code shows how you can leverage request handling:
void HttpServer::handleRequest(const Request& req, Response& res) {
// Parse and handle the request
// Generate a corresponding response
}
By efficiently parsing requests, you can accommodate different HTTP methods and handle them as needed.
Response Generation
Generating responses is as significant as handling requests. Below is a code snippet that demonstrates how to form an HTTP response:
void Response::send(const std::string& body) {
this->body = body;
this->headers["Content-Type"] = "text/html";
// Send the assembled response back to the client
}
This method sets the response body and header appropriately, ensuring the client receives the right content type.
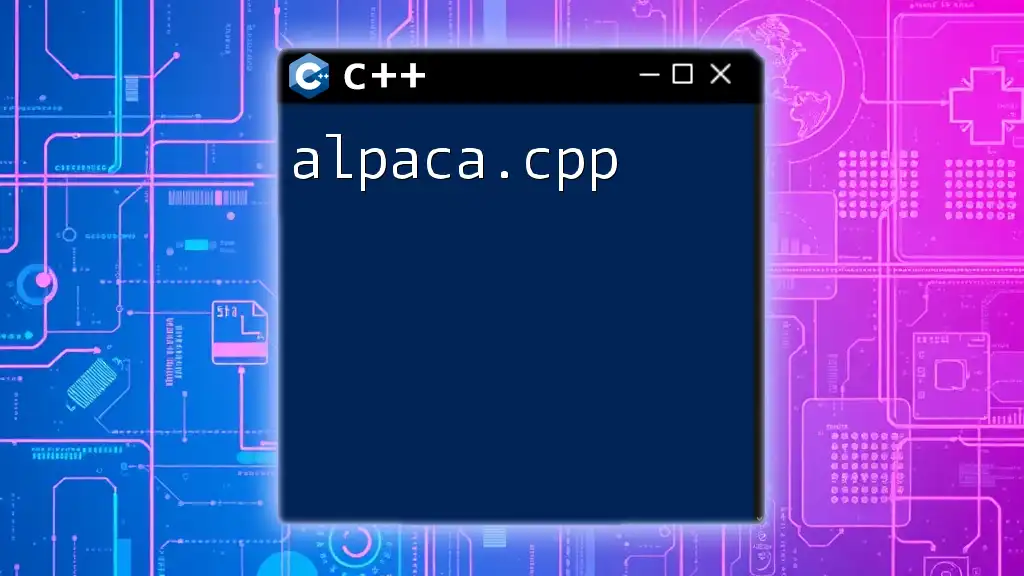
Common Commands and Operations
Starting the HTTP Server
To run your server, you typically need to call the start function from your main method, as shown below:
int main() {
HttpServer server;
server.start();
return 0;
}
This code initializes your server and begins listening for requests.
Handling GET Requests
GET requests are usually directed to obtain data from your server. Here’s how you might implement them:
if (req.getMethod() == "GET") {
handleGet(req, res);
}
This conditional checks if the request is a GET method and delegates the handling to a dedicated function for GET requests.
Handling POST Requests
POST requests often submit data to your server. The handling of such requests can be implemented as follows:
if (req.getMethod() == "POST") {
handlePost(req, res);
}
This pattern allows for organized handling where `handlePost` processes the incoming data payload.
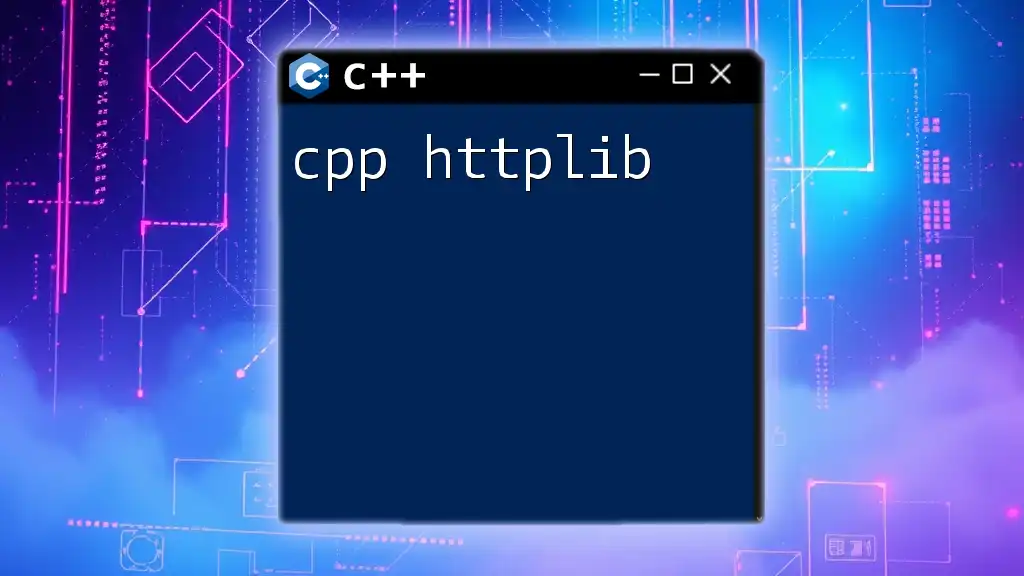
Integrating with HTML and Client-Side Applications
Serving HTML Pages
You can serve HTML pages seamlessly through `app_httpd.cpp`. A simple example of serving an HTML page is provided below:
void HttpServer::handleRequest(const Request& req, Response& res) {
if (req.getPath() == "/index.html") {
std::ifstream file("index.html");
std::string content((std::istreambuf_iterator<char>(file)), std::istreambuf_iterator<char>());
res.send(content);
}
}
This ensures that your server can serve static HTML content by reading from a file.
Client-Side Interaction
To create an interactive experience, you can use AJAX. Consider the following example, where the client might request data:
fetch('/data')
.then(response => response.json())
.then(data => console.log(data));
This snippet represents how a client-side application can asynchronously interact with `app_httpd.cpp`, fetching JSON data.
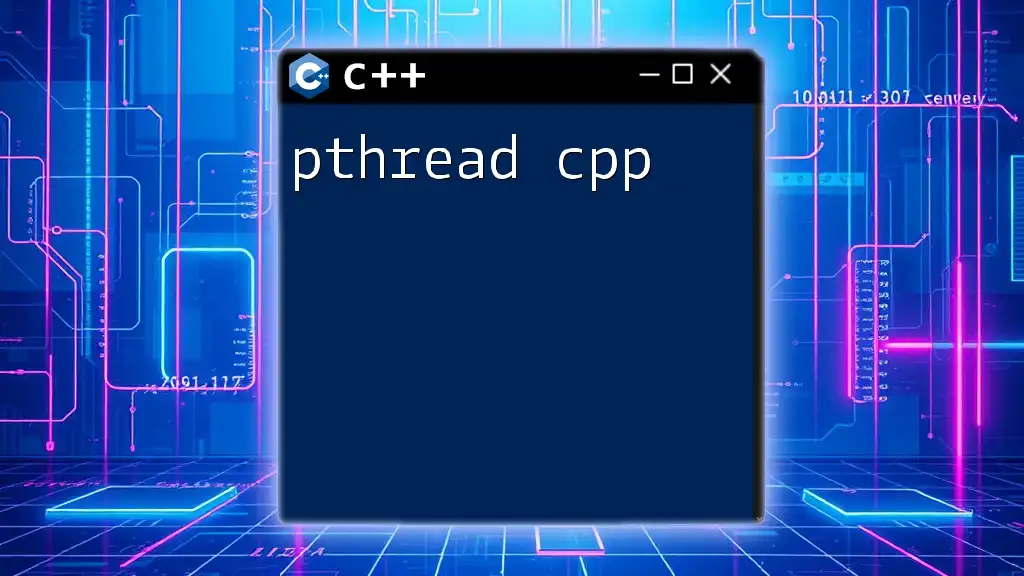
Debugging and Error Handling
Common Errors
When developing with `app_httpd.cpp`, you may encounter common pitfalls, such as:
- Port already in use: You might attempt to start your server on a port that another process is using. Ensure no conflicting applications are running.
- File not found exceptions: This can occur if your requested HTML files or resources are missing. Double-check your file paths.
Debugging Techniques
Effective debugging can significantly streamline your development process. Implement logging mechanisms like:
std::cout << "Received request for: " << req.getPath() << std::endl;
This simple line of code logs incoming request paths, helping identify issues as they arise.
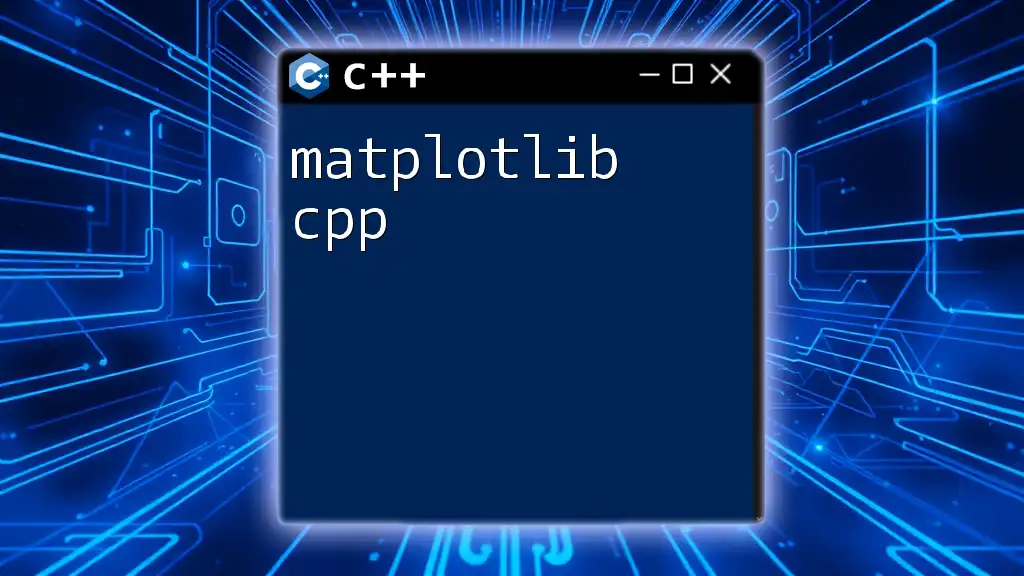
Optimization Best Practices
Performance Tuning
Improving server performance may require several steps:
- Connection Handling: Use asynchronous I/O to enable your server to handle multiple connections simultaneously.
- Caching: Implement response caching to reduce load times for frequently requested resources.
Scalability Considerations
As your application grows, keeping scalability in mind is crucial. Consider:
- Load Balancing: Distribute incoming requests across multiple servers to prevent overload.
- Configuration changes: Ensure your server can accommodate increased traffic by adjusting timeout settings and connection limits.
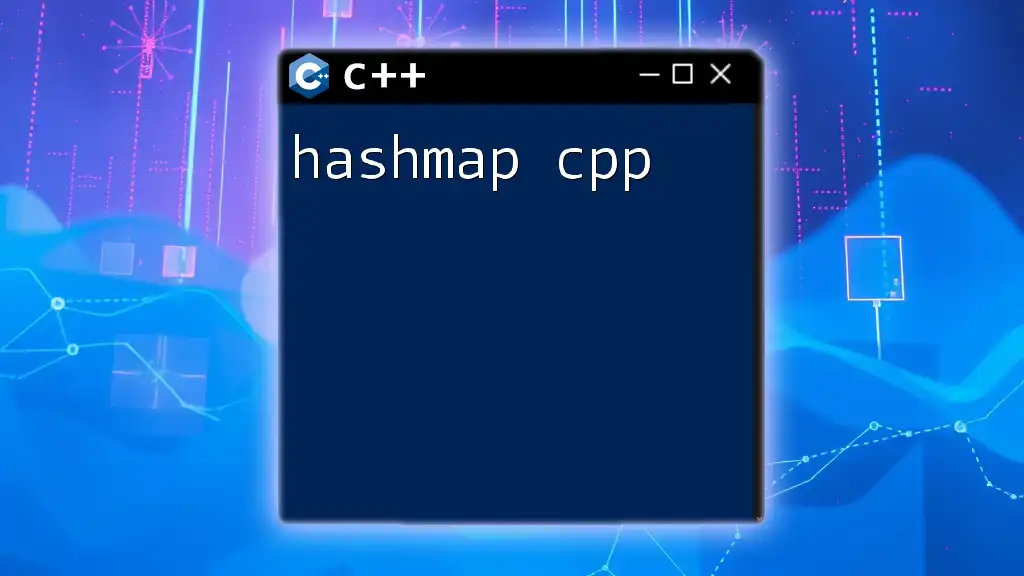
Conclusion
In this guide, we’ve explored the intricacies of `app_httpd.cpp`, from setting up your environment to handling requests and generating responses. Having a deep understanding of HTTP server functionality will empower you to build efficient web applications.
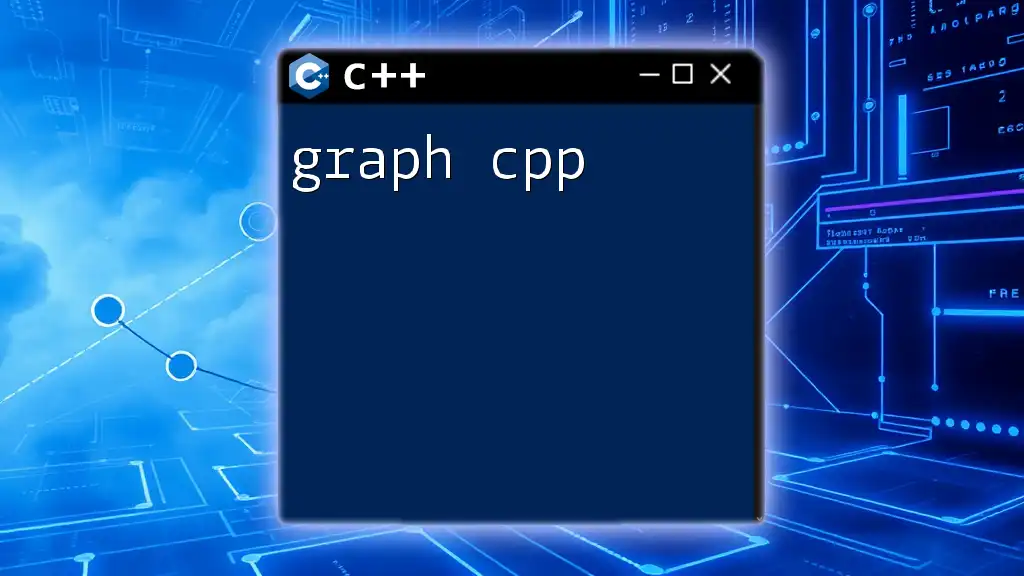
Call to Action
Now is the time to put your newfound knowledge into practice. Try constructing your own HTTP server using `app_httpd.cpp` and experiment with the various features discussed in this guide. Engaging with online communities or taking courses can provide additional support and resources. Happy coding!