The "cpp httplib" is a lightweight C++ HTTP/HTTPS server and client library that makes it easy to develop web applications by handling HTTP requests and responses in a simple and straightforward manner.
Here's a quick example of how to set up a basic HTTP server using the cpp-httplib library:
#include "httplib.h"
int main() {
httplib::Server svr;
svr.Get("/", [](const httplib::Request&, httplib::Response& res) {
res.set_content("Hello, World!", "text/plain");
});
svr.listen("localhost", 8080);
return 0;
}
What is C++ Httplib?
CPP Httplib is a lightweight and easy-to-use header-only C++ library designed for making HTTP requests. It simplifies the process of communicating over HTTP by encapsulating common tasks such as sending GET and POST requests, handling responses, managing cookies, and enabling secure connections via SSL/TLS. Its straightforward API allows developers to quickly implement HTTP functionality in their applications.
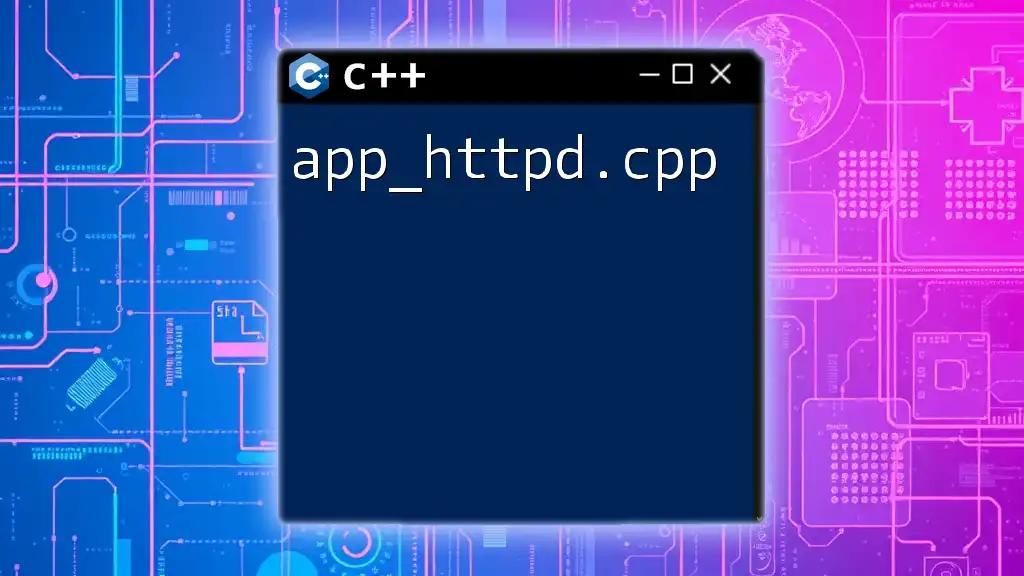
Why Choose C++ Httplib?
CPP Httplib comes with several key features that make it a preferred choice among C++ developers:
- Header-Only Library: There’s no need for separate installation since everything is contained in a single header file.
- Minimalistic API: The design of C++ Httplib is intuitive, making it easy to use even for developers who are new to HTTP networking.
- Supports SSL: It allows for secure communication without requiring complicated setup processes.
- Synchronous Interface: For straightforward use cases, C++ Httplib provides synchronous API calls that are easy to integrate into applications.
Compared to other HTTP libraries, C++ Httplib has less bloat and a simpler learning curve, which makes it particularly useful for quick prototyping or when you need to integrate HTTP functionality efficiently.
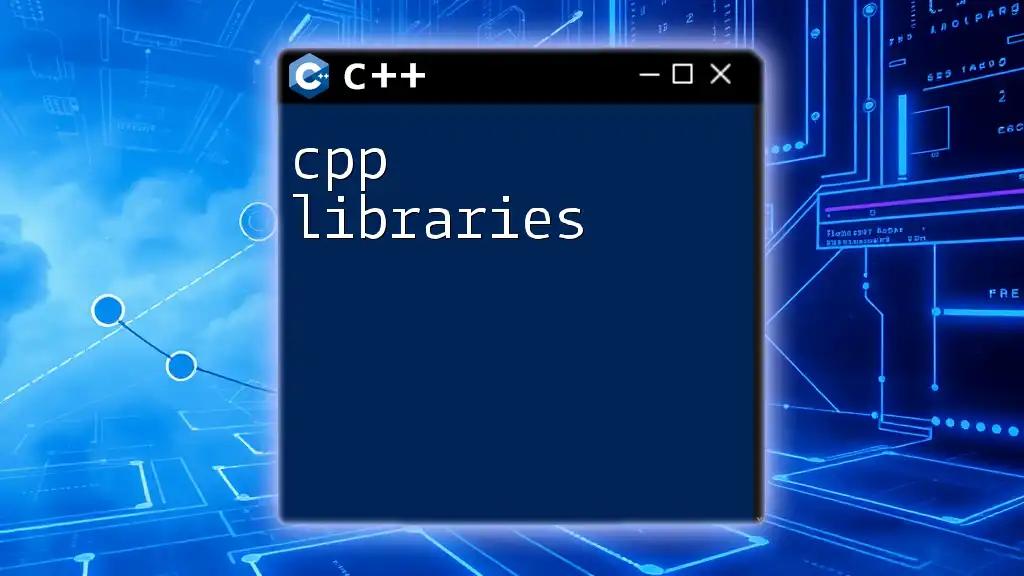
Setting Up C++ Httplib
Prerequisites
Before you get started with C++ Httplib, ensure that you are using a compatible version of C++ (C++11 and onward). Additionally, you may need a C++ compiler and a build tool like CMake for project setup.
Installation Guide
To integrate C++ Httplib into your C++ project, follow these steps:
- Download `httplib.h` directly from its GitHub repository.
- Place it in your project directory.
- If you are using CMake, you may add it to your project with the following command in your `CMakeLists.txt`:
include_directories(${CMAKE_CURRENT_SOURCE_DIR})
You are now ready to start using C++ Httplib in your project!
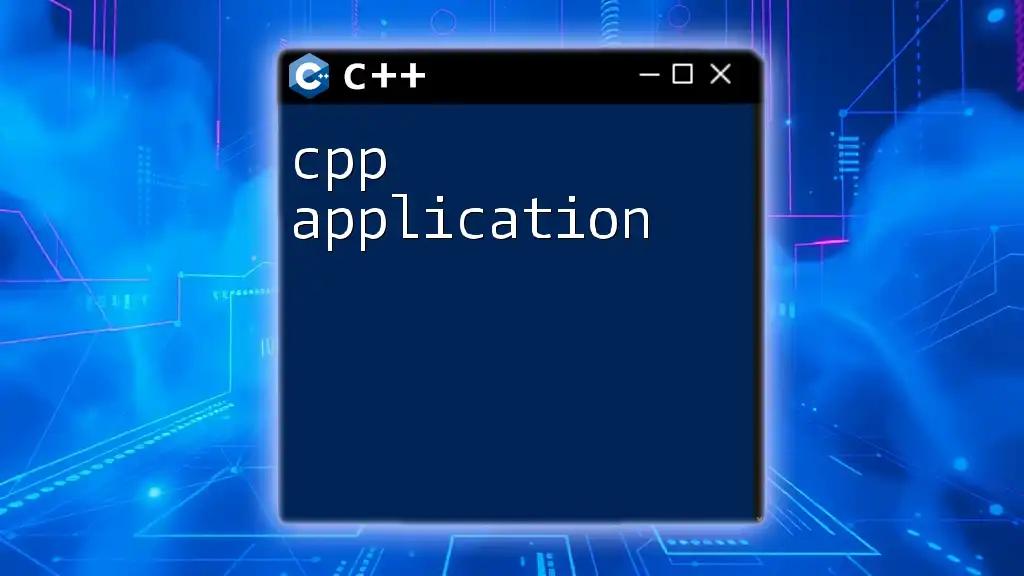
Basics of C++ Httplib
Understanding HTTP and C++ Httplib
HTTP (HyperText Transfer Protocol) is a protocol used for transferring hypertext requests and information on the internet. C++ Httplib allows developers to interact with web servers using standard HTTP methods like GET and POST without getting bogged down by the complexities of TCP/IP communications.
Making Your First HTTP Request
Sending a GET Request
To send a simple GET request with C++ Httplib, you can start with the following code:
#include "httplib.h"
httplib::Client cli("http://example.com");
auto res = cli.Get("/api/v1/resource");
if (res) {
std::cout << "Status: " << res->status << std::endl;
std::cout << "Response Body: " << res->body << std::endl;
}
In this example, we create a client instance pointing to `http://example.com` and request the resource located at `/api/v1/resource`. The response is checked for validity, and the status code along with the body of the response is printed.
Handling Response
When you receive a response from your GET request, it's essential to handle errors gracefully. The `res` object contains the HTTP status code and the response body. You can inspect the response like so:
if (res) {
std::cout << "Status Code: " << res->status << std::endl;
std::cout << "Response Content: " << res->body << std::endl;
} else {
std::cerr << "Error: " << res.error() << std::endl;
}
POST Requests with C++ Httplib
Sending a POST Request
Sending a POST request is just as easy. Below is an example where you send JSON data to an API:
#include "httplib.h"
httplib::Client cli("http://example.com");
httplib::Headers headers = { {"Content-Type", "application/json"} };
auto res = cli.Post("/api/v1/resource", headers, "{\"name\":\"value\"}", "application/json");
if (res) {
std::cout << "Response Status: " << res->status << std::endl;
}
This code demonstrates how to send JSON data in a POST request while specifying the content type. The API's response is evaluated similarly to that of the GET request.
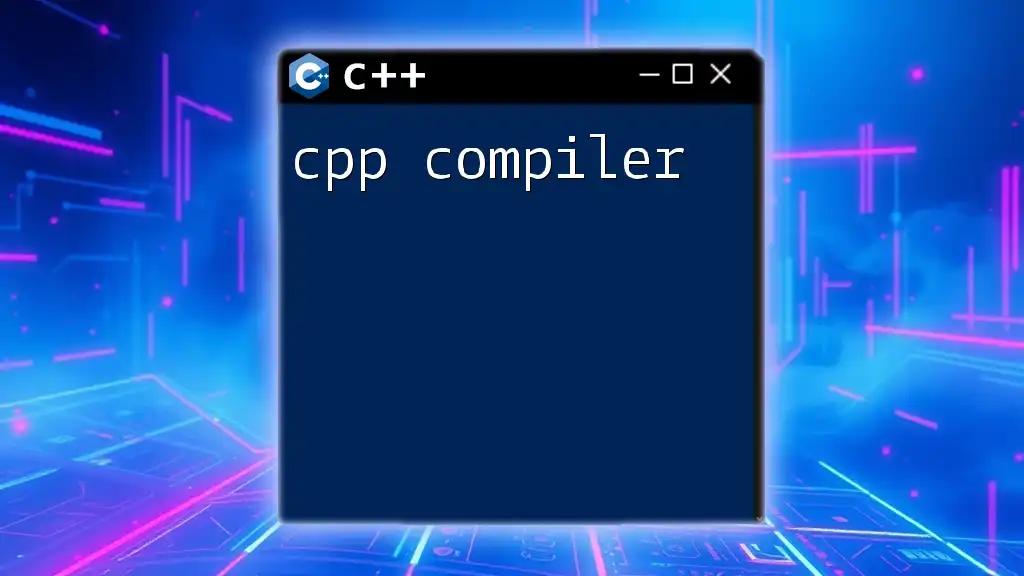
Advanced Features of C++ Httplib
Handling Cookies and Sessions
Cookies are a vital part of the HTTP protocol, offering ways to maintain sessions. C++ Httplib makes it easy to manage cookies. Here's how you can set a cookie:
cli.set_cookie("session_id", "your-session-id");
This ability enables seamless session handling between your client and the server.
SSL Support with C++ Httplib
Enabling HTTPS
Negotiating a secure connection has never been easier. To make HTTPS requests, you simply need to create an `httplib::Client` instance with `https://` as follows:
httplib::Client cli("https://secure.example.com");
auto res = cli.Get("/secure/api");
if (res) {
std::cout << "Secure Response: " << res->body << std::endl;
}
This code will handle SSL/TLS handshake internally, allowing you to focus on your application logic while maintaining secure communication.
Custom Headers and Timeouts
Setting Custom Headers
Often, you will need to include custom headers in your requests, such as authentication tokens. Custom headers in C++ Httplib can be added as shown below:
httplib::Headers headers = { {"Authorization", "Bearer your_token"} };
auto res = cli.Get("/auth/protected/resource", headers);
This allows you to leverage bearer tokens and other headers essential for API security.
Configuring Timeouts
Setting timeouts is crucial for avoiding indefinite waits on network requests. C++ Httplib allows you to configure connection and read timeouts:
cli.set_connection_timeout(5); // 5 seconds
cli.set_read_timeout(10); // 10 seconds
With these configurations, if a request exceeds the specified time, you can handle that gracefully, perhaps by retrying or notifying the user.
File Uploads and Downloads
Uploading Files
CPP Httplib also supports file uploads. To upload a file, you can use the following:
auto res = cli.Post("/upload", "file", "filename.txt");
if (res) {
std::cout << "Upload Response: " << res->status << std::endl;
}
This straightforward approach simplifies file transfer tasks across your HTTP requests.
Downloading Files
Downloading files is just as straightforward. Here’s how to download a file:
cli.download("/download/file.zip", "downloaded_file.zip");
This method streamlines the process, saving the file directly to your local directory.
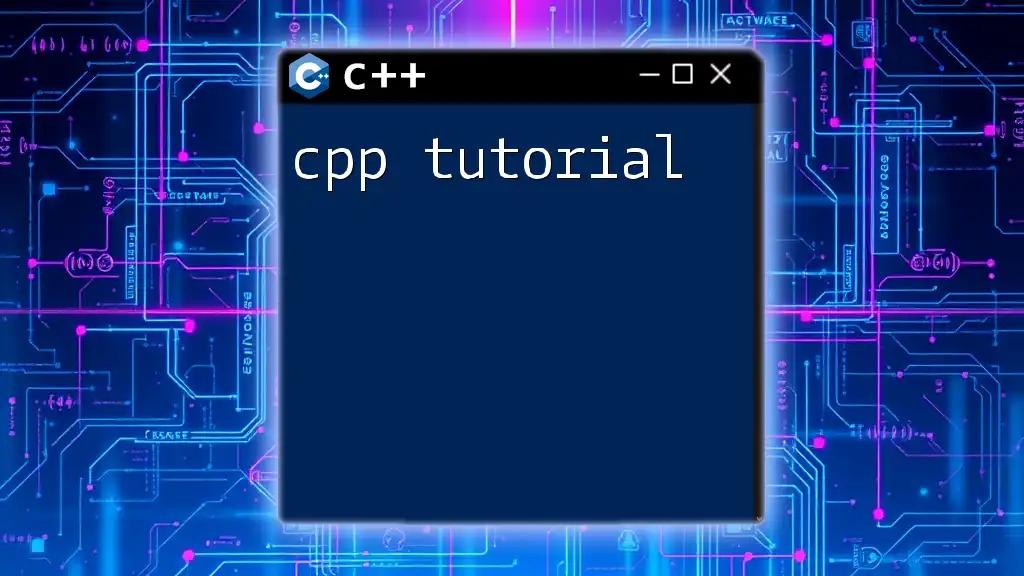
Error Handling in C++ Httplib
Common HTTP Error Codes
Understanding standard HTTP error codes such as 404 (Not Found), 500 (Internal Server Error), and others is essential for handling response data effectively.
Handling Errors in C++ Httplib
When making requests, error handling should be part of your workflow. C++ Httplib provides a robust error-checking mechanism:
if (!res) {
std::cerr << "Error: " << res.error() << std::endl;
}
This allows you to capture and respond to issues like unreachable servers or unrecognized endpoints.
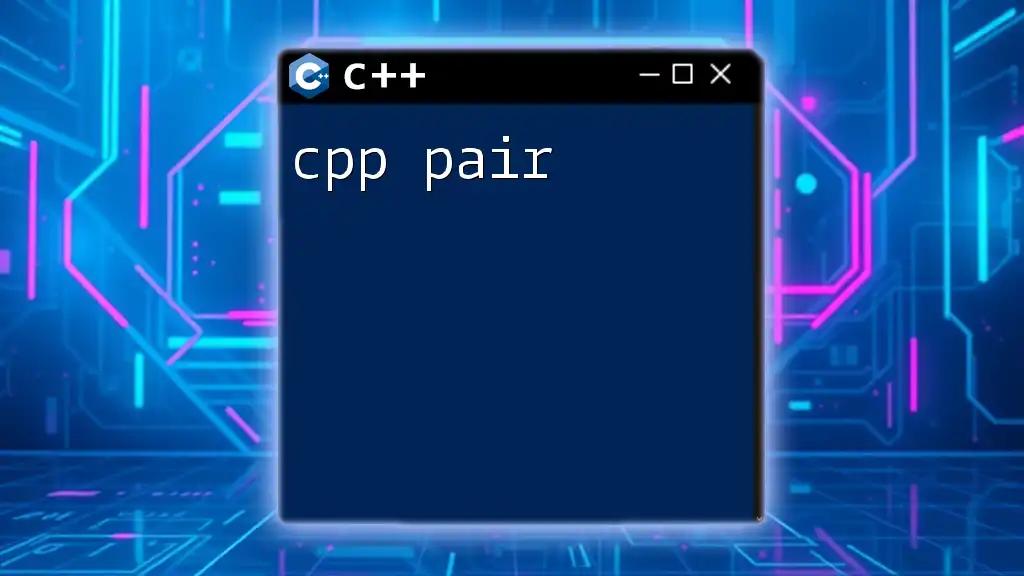
Best Practices for Using C++ Httplib
Writing Clean and Maintainable Code
Structuring your HTTP requests clearly and logically is paramount. Consider creating functions to encapsulate your GET and POST requests, promoting code reuse and readability.
Security Considerations
Always prioritize security when dealing with HTTP communications. Employ HTTPS for secure connections, manage sensitive data carefully, and utilize necessary protective measures like encryption for stored tokens.

Real-world Use Cases
Building a REST API Client
CPP Httplib is ideal for constructing RESTful API clients that interface with web services. It helps developers perform CRUD operations effortlessly while adhering to REST principles.
Web Scraping Using C++ Httplib
Web scraping can be efficiently accomplished with C++ Httplib, allowing you to fetch web pages, parse the content, and extract useful information programmatically.
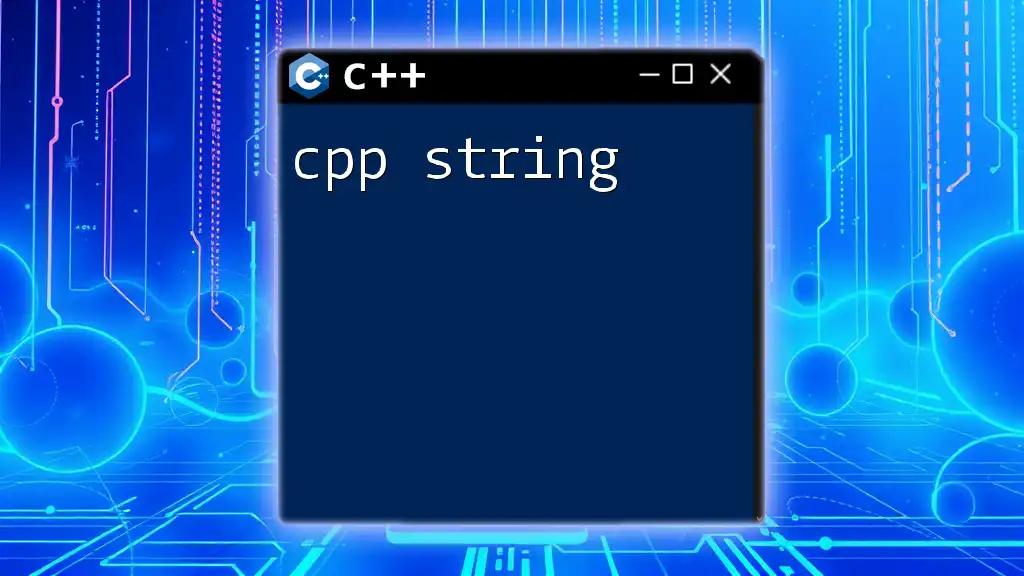
Conclusion
In summary, C++ Httplib is a powerful library that simplifies the complexities of HTTP communication in C++. Whether you are making basic GET and POST requests or handling secure communications, C++ Httplib offers a plethora of functionalities that facilitate your web development journey. As you explore and experiment with C++ Httplib, you will discover its potential to enhance your project with efficient networking capabilities.
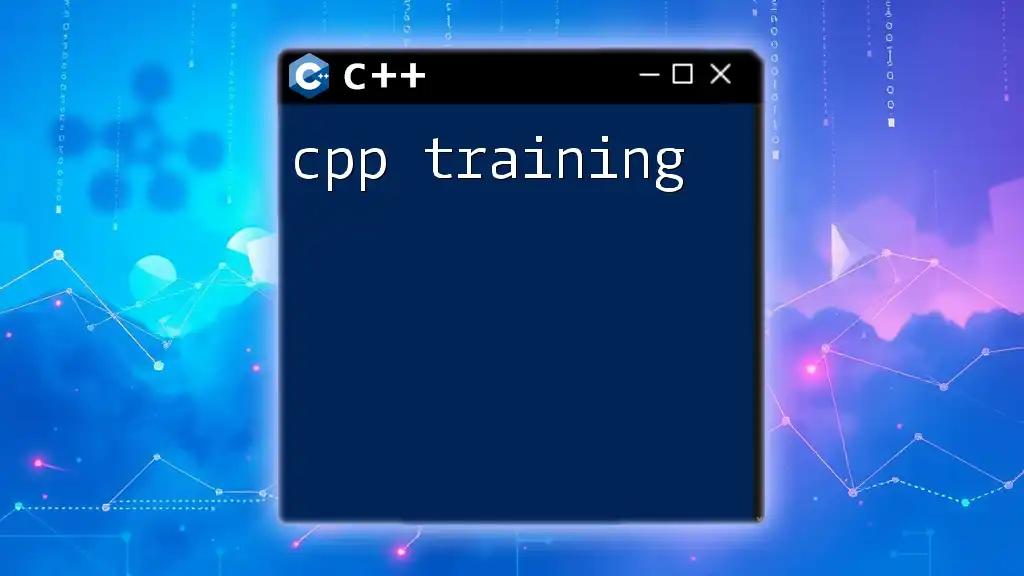
Additional Resources
For further learning, the official C++ Httplib documentation, community forums, and tutorial videos provide excellent supplementary material. Engaging with these resources will help solidify your understanding and expand your skills in leveraging this versatile library.