C++ graphics often involve using libraries such as SDL or SFML to create visual applications and render images, shapes, or animations on the screen. Here's a simple example using SFML to create a window and draw a circle:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "C++ Graphics Example");
sf::CircleShape circle(50); // Radius of 50
circle.setFillColor(sf::Color::Green); // Set circle color to green
circle.setPosition(375, 275); // Position circle in the center
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(circle); // Draw the circle
window.display();
}
return 0;
}
Setting Up Your Development Environment
To start your journey with C++ graphics, you’ll need to set up an appropriate development environment. Here are some recommended Integrated Development Environments (IDEs) that are well-suited for graphics programming:
- Visual Studio: Powerful IDE with extensive debugging tools and support for multiple C++ libraries.
- Code::Blocks: A lightweight, open-source IDE that can be easily configured with different graphics libraries.
- Eclipse CDT: A feature-rich open-source IDE that supports C++ development.
Installing Essential Graphics Libraries
You’ll need to install graphics libraries to begin creating graphics applications in C++. Some of the most popular libraries include SFML, SDL, and OpenGL. Below is a step-by-step guide on installing SFML, but the process is similar for other libraries.
Example: Step-by-Step Guide to Installing SFML
- Download SFML: Go to the official SFML website and download the latest version compatible with your operating system.
- Extract the Files: Unzip the downloaded files to a location on your computer.
- Configure Your IDE: Set up your IDE to include the SFML headers and link against the SFML libraries.
- Test the Installation: Compile a simple SFML program (see the Basic SFML Example below) to ensure everything is set up correctly.
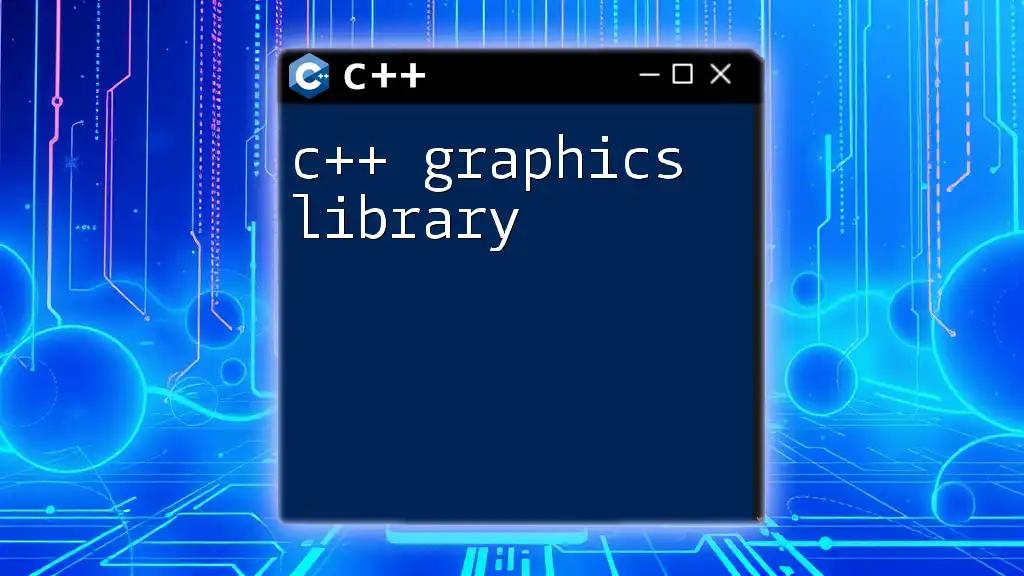
Basics of Graphics in C++
Understanding Graphics Fundamentals
Before diving into coding, it is essential to understand some fundamental concepts in graphics programming.
Coordinate Systems
In C++ graphics, you will commonly work with two types of coordinate systems: 2D and 3D. The coordinate system is integral to understanding how to position and manipulate objects on the screen.
- 2D Coordinates: In 2D graphics, you use an (x, y) coordinate system, where the origin (0, 0) is usually at the top-left corner of the window.
- 3D Coordinates: In 3D graphics, the system extends to (x, y, z), where z represents depth.
Rendering Basics
What is Rendering?
Rendering is the process of generating an image from a model by means of computer programs. Rendering plays a crucial role in transforming geometric data into visuals that can be displayed on the screen.
Rendering Pipeline Overview
The rendering pipeline can be divided into several stages:
- Input Stage: Gathering all necessary information about objects, lights, and cameras.
- Processing Stage: Transforming geometry based on various factors (e.g., transformations, lighting).
- Output Stage: Drawing the processed images onto the screen.
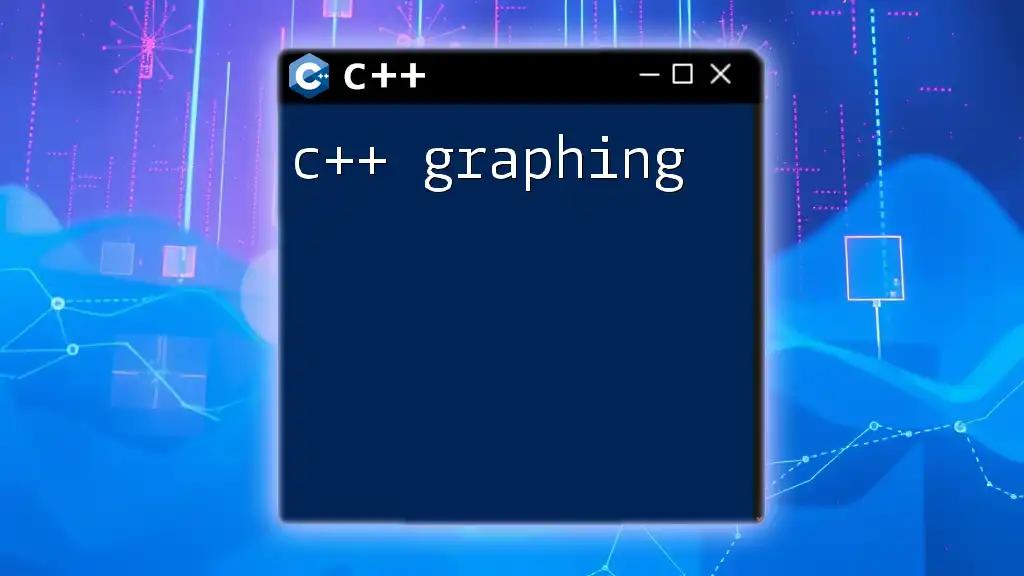
Key Graphics Libraries in C++
SFML (Simple and Fast Multimedia Library)
Overview of SFML
SFML is a modern multimedia library designed to handle graphics, audio, and input in a simple and fast way. It provides various features, making it particularly popular among game developers and multimedia applications.
Setting Up SFML
To set up SFML, ensure you have followed the installation steps outlined earlier.
Basic SFML Example
Here is a minimal example of an SFML application:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Graphics");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
Explanation: This code creates a window of 800x600 pixels. In the main loop, it continuously polls for events (like closing the window) and clears the window before displaying it again.
SDL (Simple DirectMedia Layer)
Overview of SDL
SDL is widely used for developing games and multimedia applications across various platforms. It provides a low-level interface to graphics, sound, and input, making it an excellent alternative to SFML.
Setting Up SDL
Similar to SFML, you will need to follow the appropriate installation and configuration steps for SDL in your IDE.
Basic SDL Example
Here’s a simple SDL application:
#include <SDL2/SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("SDL Graphics", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, 800, 600, SDL_WINDOW_SHOWN);
SDL_Event e;
bool running = true;
while (running) {
while (SDL_PollEvent(&e)) {
if (e.type == SDL_QUIT) {
running = false;
}
}
}
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
Explanation: This example creates a window with the SDL library. The main loop processes events and checks if the user closes the window.
OpenGL
Overview of OpenGL
OpenGL is a powerful graphics API used to render 2D and 3D vector graphics. It is widely adopted in the video game and graphics programming industry due to its cross-platform capabilities.
Setting Up OpenGL
Make sure to install necessary libraries and tools required for OpenGL development according to your operating system.
Basic OpenGL Example
This example demonstrates how to set up a simple OpenGL application:
#include <GL/glut.h>
void display() {
glClear(GL_COLOR_BUFFER_BIT);
// Drawing code goes here
glFlush();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutCreateWindow("OpenGL Graphics");
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
Explanation: This snippet sets up a basic OpenGL environment. The display function is used to clear the color buffer and is essential for rendering.

Drawing Shapes and Colors
Basic Shapes in C++
Creating shapes is fundamental in graphics programming. Depending on the library you are using, the syntax will differ.
Drawing Primitives Using SFML
In SFML, you can easily draw shapes such as circles, rectangles, or lines:
sf::CircleShape circle(50); // Circle with a radius of 50
circle.setFillColor(sf::Color::Red);
Drawing Primitives Using SDL
In SDL, drawing involves manipulating pixels directly or using SDL surfaces.
Color Manipulation
Understanding Color Models
Colors in graphics can be represented through various color models, with RGB and RGBA being the most common. Each of these models allows you to define the color and transparency of shapes accurately.
Applying Colors to Shapes in C++ Graphics
Using SFML, applying a color is straightforward. For instance:
shape.setFillColor(sf::Color::Green);
This line of code sets the fill color of a shape to green.
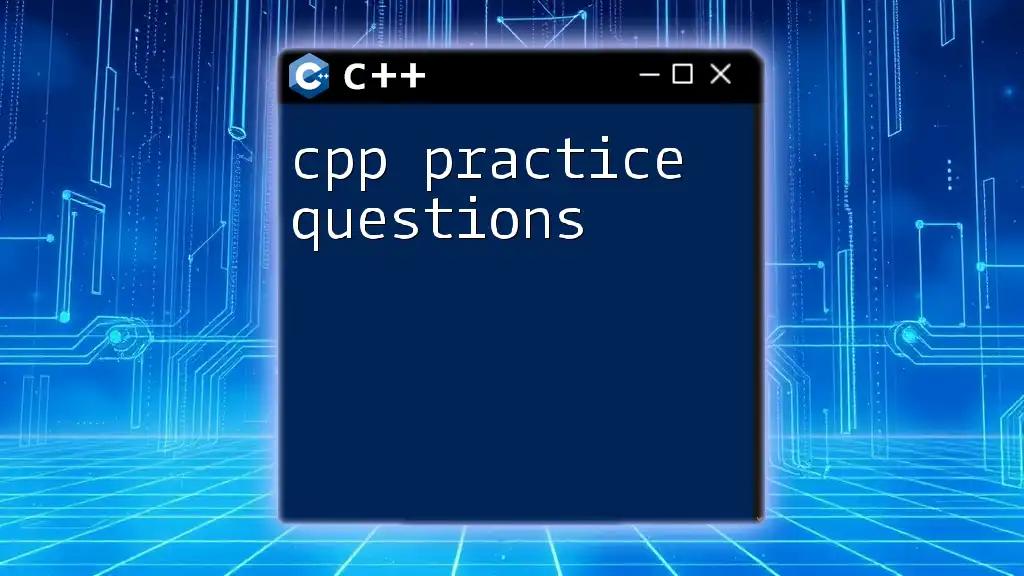
Animation Fundamentals
Introduction to Animation in Graphics
Animation is the process of creating a series of images or frames that, when displayed sequentially, give the illusion of motion. A solid understanding of frame updates is crucial in making smooth animations.
Creating Simple Animations
With SFML, you can implement animations using a game loop where you continuously update the position of a shape. Here’s a simple example of moving a circle:
sf::CircleShape circle(50);
circle.setFillColor(sf::Color::Green);
circle.setPosition(100, 100);
float speed = 0.1f; // Speed of movement
while (window.isOpen()) {
// Update the position
circle.move(speed, 0); // Move right
window.clear();
window.draw(circle);
window.display();
}
Explanation: This code snippet updates the position of the circle in each iteration of the loop, creating a simple horizontal animation.
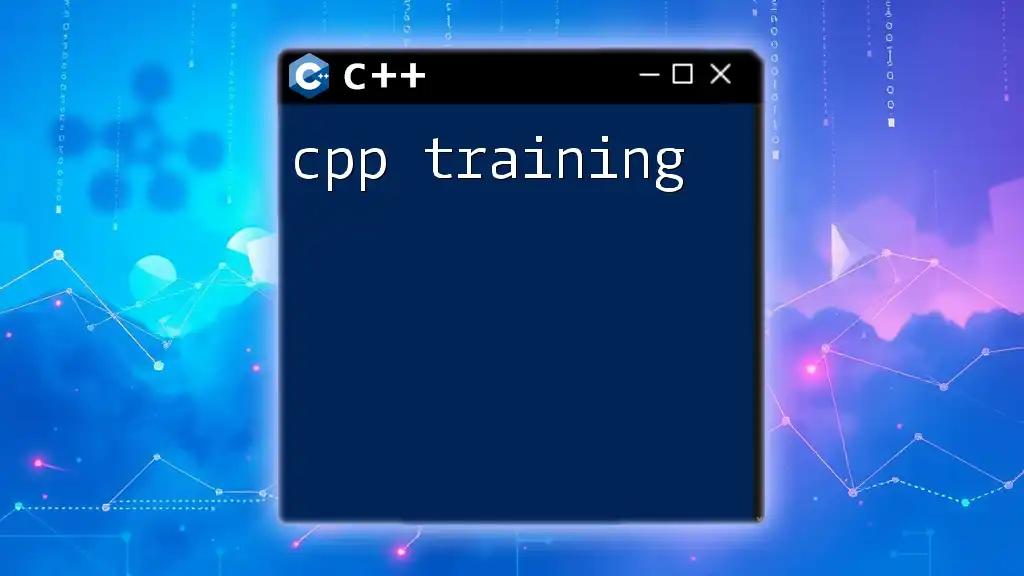
Advanced Graphics Techniques
Textures and Images
Textures are images used to cover the surfaces of shapes. They add a layer of realism to graphic applications.
Loading and Applying Textures
In SFML, you can load a texture and apply it to a shape like this:
sf::Texture texture;
texture.loadFromFile("image.png");
sf::Sprite sprite(texture);
Handling Mouse and Keyboard Input
User input is crucial for interactive applications. Both SFML and SDL provide ways to handle keyboard and mouse events effectively.
Here’s an example of how to handle mouse clicks in SFML:
if (event.type == sf::Event::MouseButtonPressed) {
if (event.mouseButton.button == sf::Mouse::Left) {
// Handle left mouse click
}
}
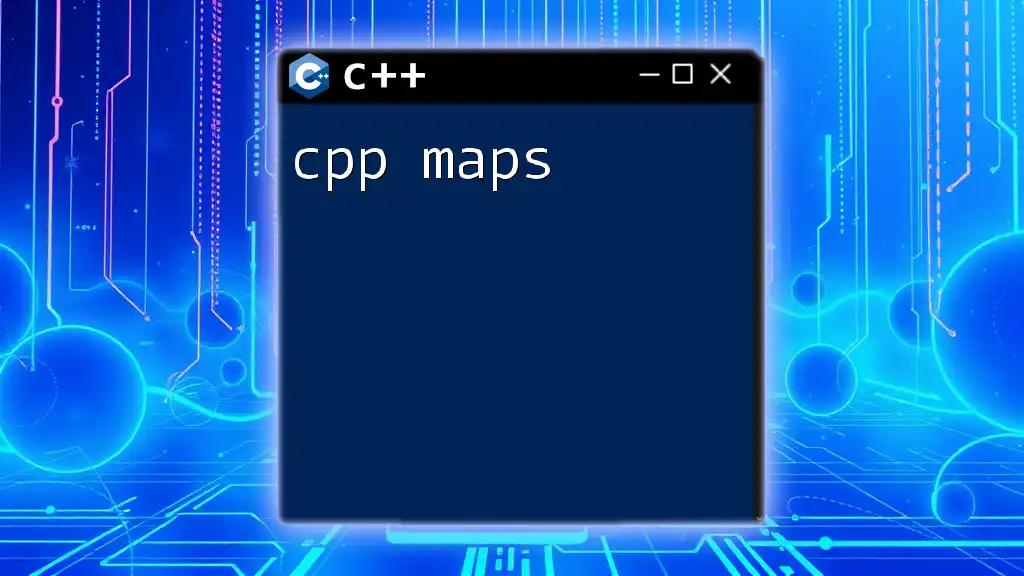
Troubleshooting Common Graphics Issues
Common Error Messages
As you work through your C++ graphics projects, you may encounter various error messages. Understanding common issues—such as linking errors or missing dependencies—can save you significant debugging time.
Performance Tuning
Performance is key in graphics programming. One way to optimize your applications is to manage resources effectively—load resources such as images and sounds at the beginning and reuse them throughout the application rather than loading them repeatedly.
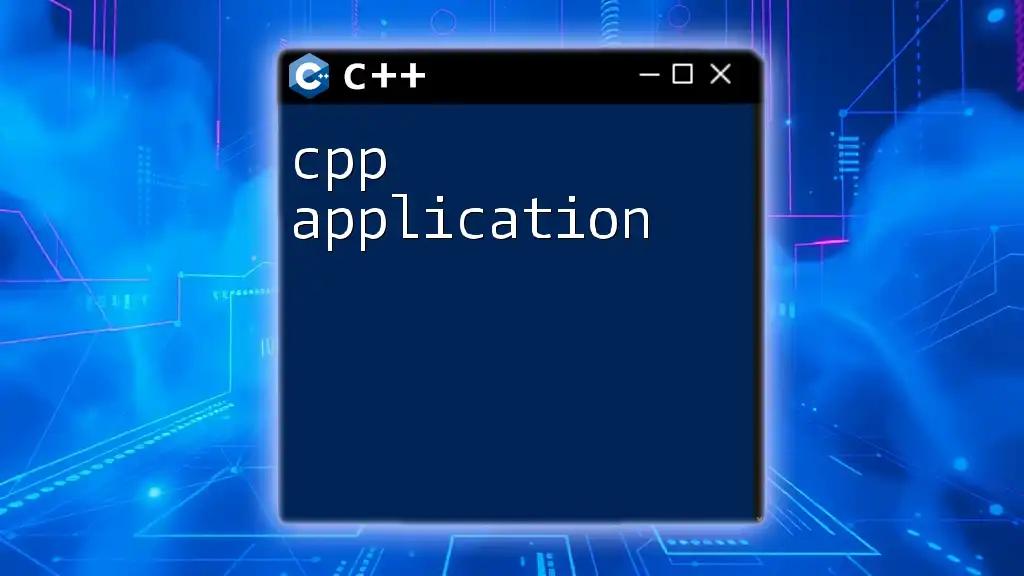
Conclusion
Celebrating the power of C++ graphics programming opens new doors to creativity and intricate applications. Whether you are building games or multimedia applications, understanding graphics fundamentals and leveraging libraries like SFML, SDL, and OpenGL will empower you to create stunning visuals.
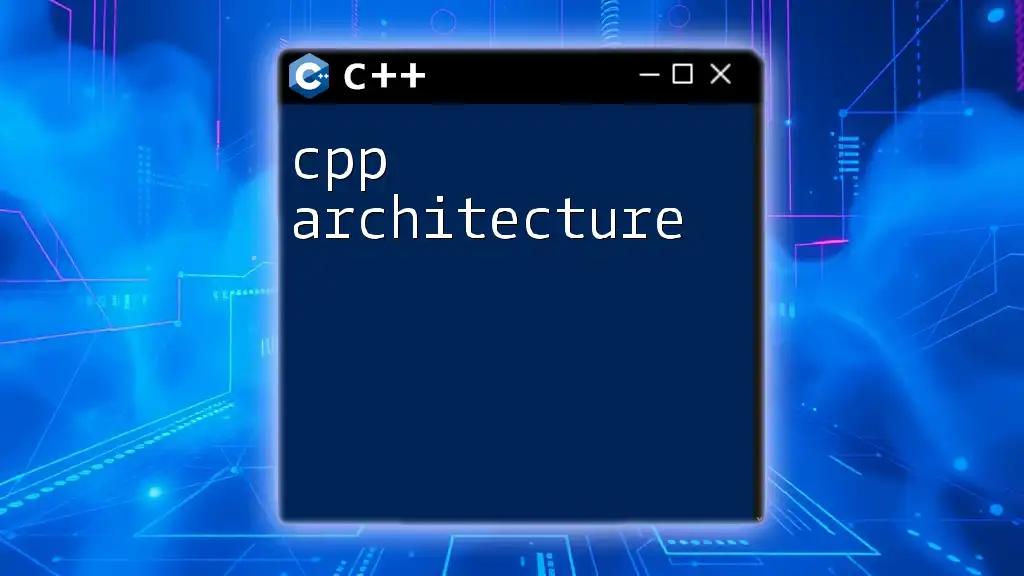
Resources and Further Reading
For deeper insights into C++ graphics, consider exploring recommended books, online courses, and the official documentation provided by the respective graphics libraries. These resources will enhance your knowledge and skills, making you a proficient graphics programmer.