The `static_cast` in C++ is used to perform safe and explicit type conversions between compatible types, ensuring that the conversion is checked at compile time.
Here’s a simple code snippet demonstrating the use of `static_cast`:
#include <iostream>
int main() {
double num = 5.25;
int convertedNum = static_cast<int>(num); // Convert double to int
std::cout << "Converted Number: " << convertedNum << std::endl; // Output: 5
return 0;
}
Understanding static_cast in C++
What is static_cast in C++?
`static_cast` is a type of casting operator in C++ that allows for compile-time type conversion. It is used to explicitly convert one type to another, primarily for built-in types, pointers, or references. The purpose of `static_cast` is to provide a safer type conversion compared to traditional C-style casts by performing compile-time checks to ensure that the conversion is valid.
When comparing `static_cast` with other C++ casting methods such as `dynamic_cast`, `const_cast`, and `reinterpret_cast`, it stands out as being the preferred method for conversions that maintain type safety without the overhead of run-time checks. In general, `static_cast` is best used for well-defined conversions where the types share a known relationship, such as derived and base classes in inheritance hierarchies.
How static_cast Works
`static_cast` utilizes compile-time type checking, meaning that if the types involved in the cast are incompatible, the code will fail to compile. This feature enhances the safety of type conversions and helps prevent undefined behavior that could manifest from incorrect casts. Under certain conditions, `static_cast` can be used to convert pointers from a base class to a derived class and vice versa, ensuring that the types being converted are indeed related.
However, it’s essential to note that while `static_cast` can safely convert pointers to classes up and down the inheritance hierarchy, it does not perform run-time checks to ensure the actual object being pointed to is of the expected type. Because of this, using `static_cast` inappropriately can lead to problematic outcomes, especially if the original object is not of the intended type.
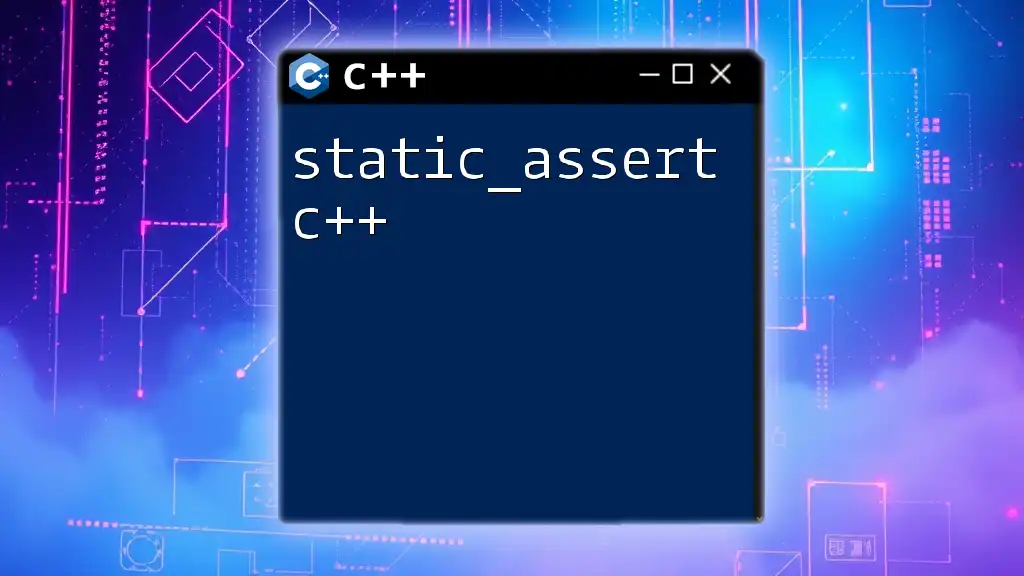
Syntax of static_cast
Basic Syntax
The general structure of `static_cast` is as follows:
static_cast<target_type>(expression);
In this syntax:
- `target_type` specifies the type you want to convert to.
- `expression` is the value you want to convert.
Examples of static_cast Syntax
Simple Casting Example
Here's an example where we convert an integer to a double using `static_cast`:
int a = 10;
double b = static_cast<double>(a);
In this case, the integer `a` is converted to a double `b` without any loss of information since the conversion up from `int` to `double` is safe and straightforward.
Casting Between Pointer Types
Another common scenario involves casting between pointer types. For example:
class Base {};
class Derived : public Base {};
Base* basePtr = new Derived();
Derived* derivedPtr = static_cast<Derived*>(basePtr);
In this situation, we have a `Base` type pointer that actually points to a `Derived` object. Using `static_cast`, we safely convert `basePtr` to a `Derived*`. This cast is valid because the underlying object is indeed of type `Derived`.
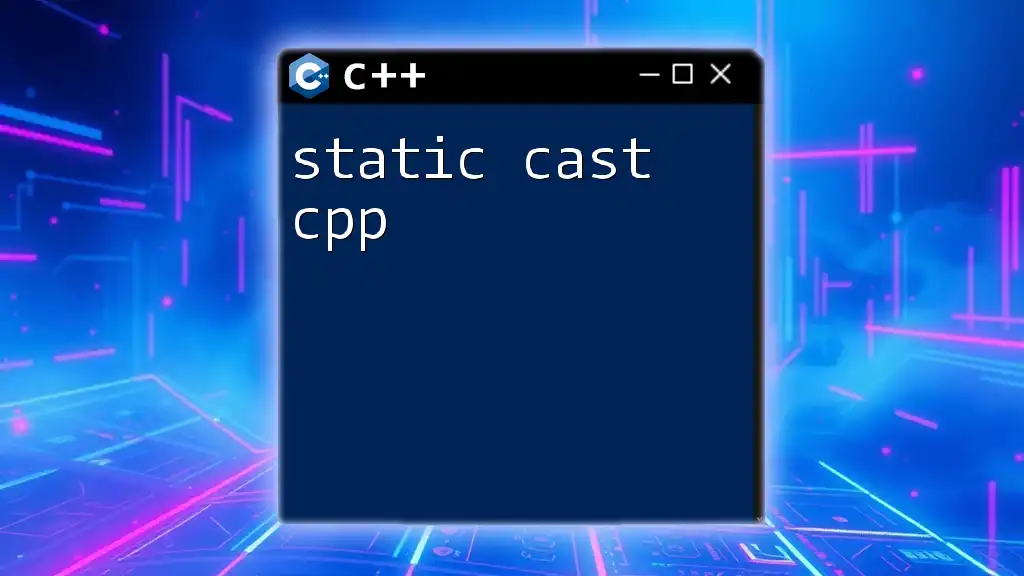
Situational Usage of static_cast
Converting Numeric Types
`static_cast` is often used for converting between different numeric types. Consider the following example:
float f = 3.14f;
int i = static_cast<int>(f); // truncates the float
In this example, the float `f` is converted to an integer `i`. Be aware that this operation truncates the decimal part, leading to a potential loss of information. Such nuances are vital to keep in mind when performing type conversions, as they can impact the program's logic.
Converting Pointers and References
`static_cast` is also frequently used to convert between pointers and references, especially in the context of inheritance. Here's an example:
class Dog {};
class Animal {};
Animal* animal = new Dog();
Dog* dog = static_cast<Dog*>(animal);
In this example, the base class pointer `animal`, which points to an object of type `Dog`, is safely cast to a `Dog*`. However, caution must be exercised. If `animal` were pointing to an object of a different derived class, this conversion would be invalid, potentially leading to undefined behavior.
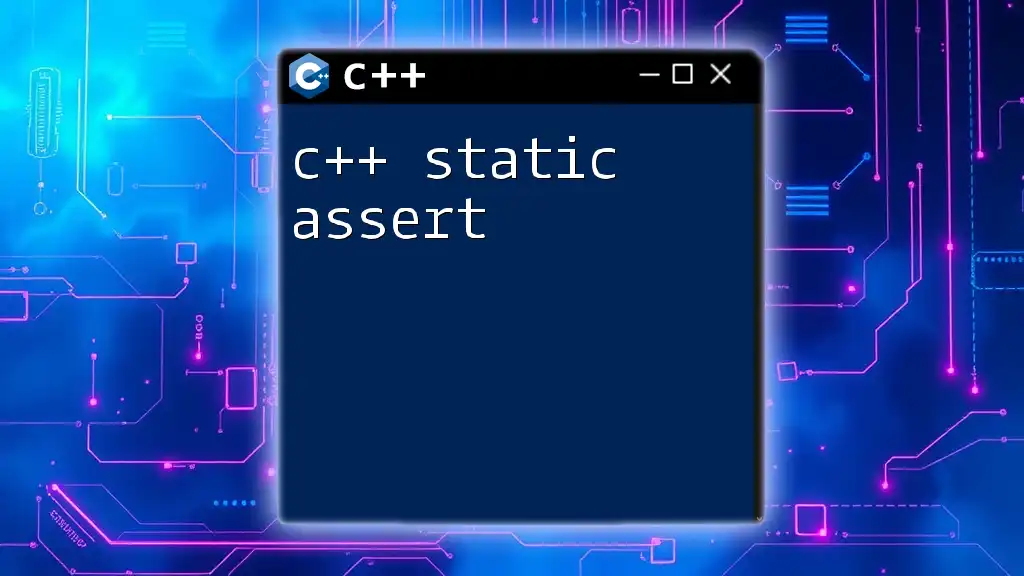
Common Scenarios for static_cast Usage
Working with Function Overloading
`static_cast` can be particularly useful in scenarios involving function overloading:
void func(int x) {
// some implementation
}
func(static_cast<int>(3.14)); // Explicitly calls the int version
Here, we explicitly convert the floating-point number `3.14` to an integer before passing it to the function `func`. This explicit cast makes it clear which function is being invoked and alleviates ambiguity that could arise with overloaded functions.
Using static_cast with Containers
STL containers often require type-safe casting, especially when dealing with polymorphic objects. Consider the following:
std::vector<void*> vec;
vec.push_back(static_cast<void*>(new Dog()));
In this snippet, we store a pointer to a `Dog` object in a vector of `void*`. Using `static_cast<void*>`, we can ensure that our pointer type matches the container's type while maintaining the original allocation type. It's crucial to ensure that when retrieving these pointers later, we cast them back to their original type to avoid runtime errors.
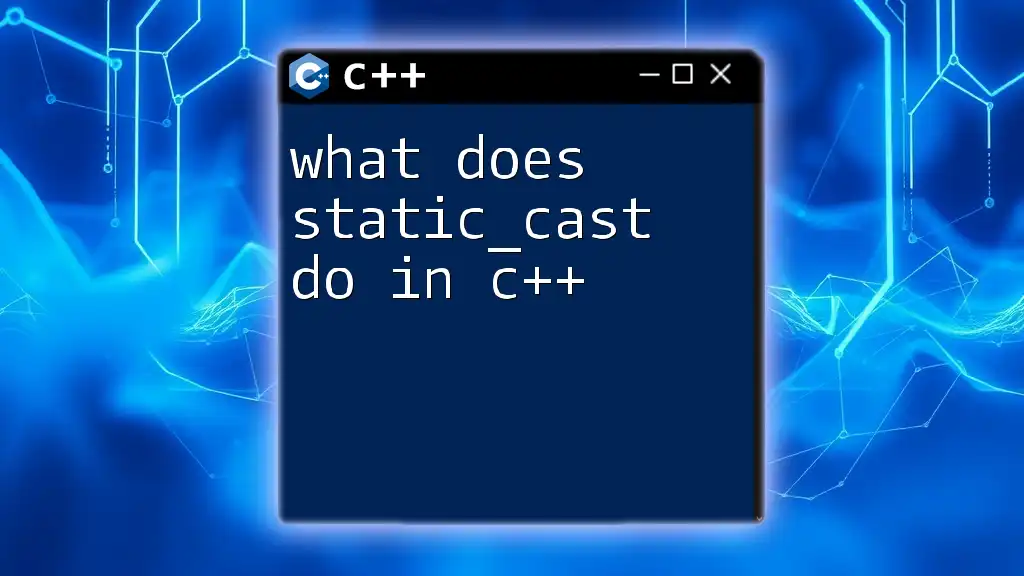
Important Considerations
Performance Implications
One of the advantages of `static_cast` is that it performs its checks at compile-time, offering better performance compared to casting techniques that require run-time evaluation, such as `dynamic_cast`. This characteristic makes `static_cast` an ideal choice when performance is a consideration, especially in scenarios involving complex type hierarchies and polymorphism.
Safety Issues with static_cast
Despite its advantages, it's essential to be aware of the potential pitfalls when using `static_cast`. If you use it to cast pointers or references without proper type safety checks, you can end up with undefined behavior. For instance:
Derived* derived = static_cast<Derived*>(basePtr); // Unsafe if basePtr is not actually of type Derived
In this case, if `basePtr` does not point to a `Derived` object, dereferencing `derived` will lead to a runtime error.
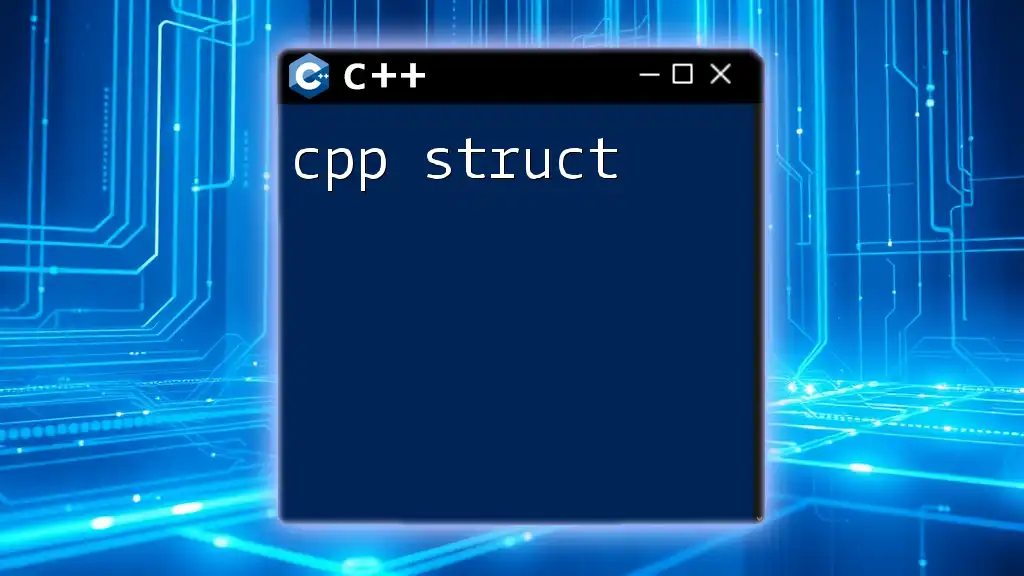
Conclusion
Recap of static_cast in C++
In summary, `static_cast` is a powerful, type-safe casting operator in C++ that provides the ability to convert between types while ensuring that the conversions are valid at compile time. It is best employed for conversions that are well-defined and help maintain the integrity of the codebase.
Further Reading and Resources
For those looking to dive deeper into C++ casting, further resources include the C++ standard and various programming texts that discuss type safety and best practices in C++. Taking the time to understand the nuances of `static_cast` will equip you with the knowledge needed to utilize this powerful feature effectively.
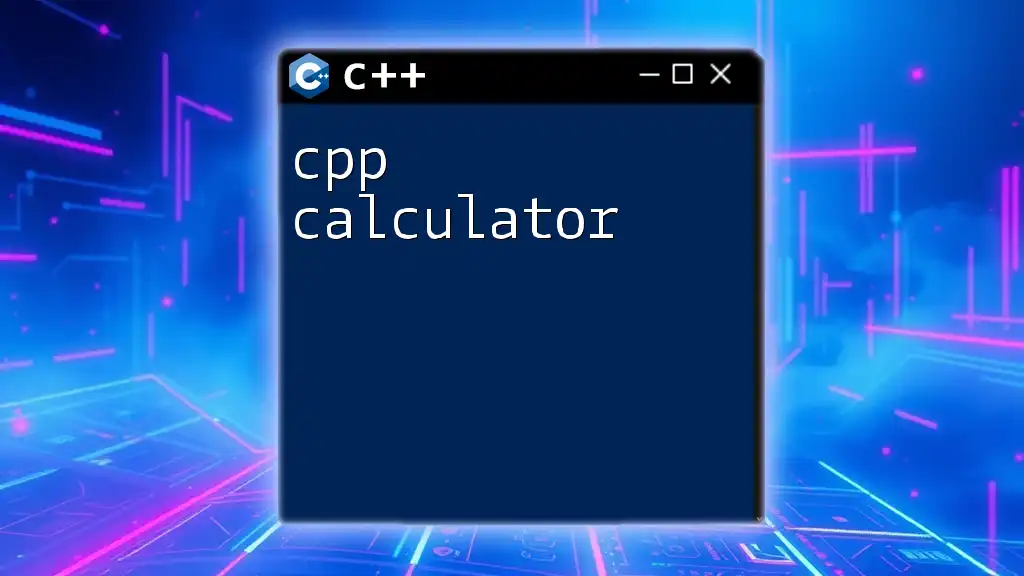
FAQs about static_cast in C++
What is the difference between static_cast and dynamic_cast?
The primary difference lies in their functionality. `dynamic_cast` performs run-time checks to ensure safe downcasting, while `static_cast` does not check the actual object type during casting. Use `dynamic_cast` when you need to verify object types at runtime, especially in polymorphic class hierarchies.
When should I use static_cast instead of reinterpret_cast?
You should prefer `static_cast` when you need a safe conversion between related types, as it is checked during compilation. In contrast, `reinterpret_cast` offers no safety checks and can result in undefined behavior if used incorrectly. Use `reinterpret_cast` sparingly and only when you understand the potential risks.
Can static_cast convert between unrelated types?
No, `static_cast` cannot convert between unrelated types. If you attempt to do so, it will result in a compilation error. Always ensure that the types involved in the cast have a well-defined relationship to maintain type safety.