A `std::variant` in C++ is a type-safe union that can hold one of several types, allowing for more flexible and type-safe programming.
Here's a simple code snippet demonstrating the usage of `std::variant`:
#include <iostream>
#include <variant>
#include <string>
int main() {
std::variant<int, float, std::string> var;
var = "Hello, Variant!";
if (std::holds_alternative<std::string>(var)) {
std::cout << std::get<std::string>(var) << std::endl;
}
return 0;
}
What is a C++ Variant?
A CPP variant is a type that can hold one of several specified types at any given time. It is a modern, type-safe way to store a value that can be of multiple types, often used in situations where a single data structure needs to accommodate a variety of data types. This is especially important as it helps simplify code and reduces the risk of errors that can occur with traditional unions, which do not provide type safety.
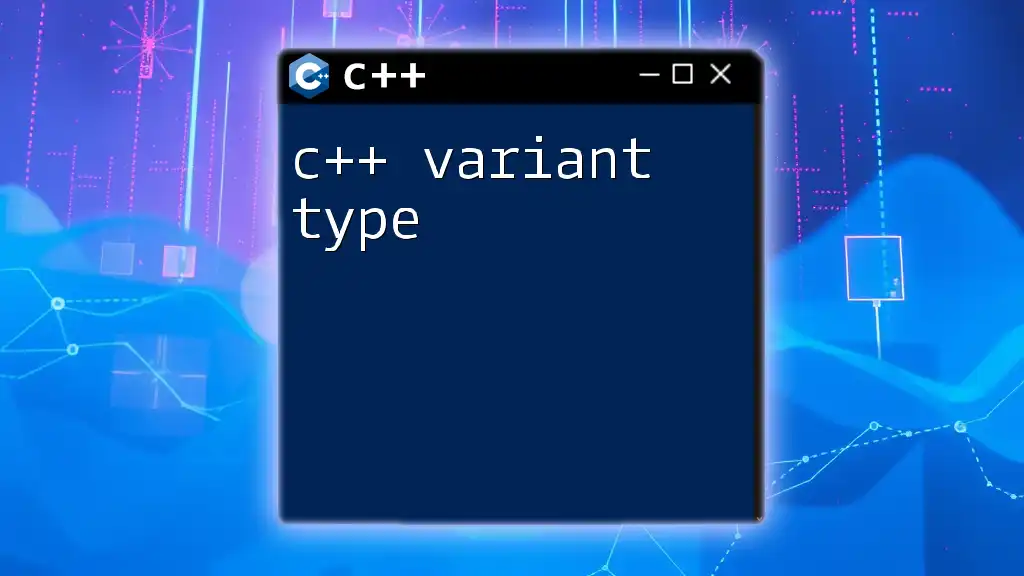
The STD Variant in C++
The C++ Standard Template Library (STL) provides a powerful feature known as `std::variant`. This is part of C++17 and allows developers to create a type-safe union. By using `std::variant`, you gain considerable advantages over older mechanisms, such as unions, which do not inherently include type information.
Important Benefits of Using std::variant
- Type Safety: Ensures you can only access the current type stored in the variant.
- Single Storage: It can store one out of multiple types, thus conserving memory.
- Flexibility: Easily switch between types without complicated logic.
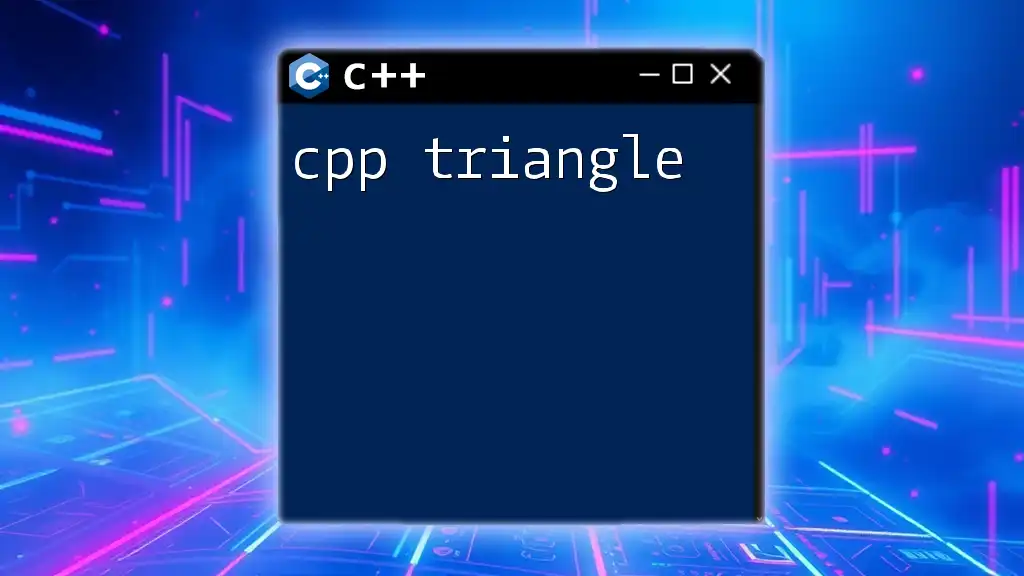
Understanding the Basics of std::variant
Syntax and Type Declaration
To declare a variable of type `std::variant`, you simply list the possible types it can take within angle brackets. Here is a basic example:
#include <variant>
std::variant<int, float, std::string> myVariant;
In this declaration, `myVariant` can hold an `int`, a `float`, or a `std::string`.
Key Characteristics of std::variant
With `std::variant`, you have type safety and the rule that at most one active member exists at any time. This means if you have a variant that can be an `int` or a `float`, it can only store one of these types at any given moment – not both.
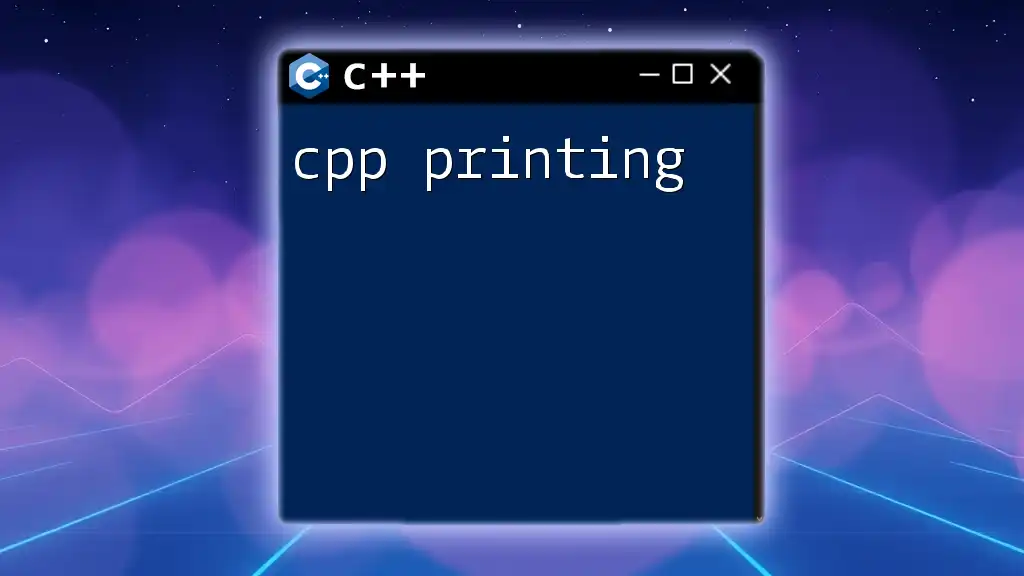
Working with std::variant
Creating a std::variant
Initialization is straightforward. You can assign a value that matches one of the types specified in the variant. Here's an example of how to create and initialize a variant:
std::variant<int, std::string> var = std::string("Hello");
In this case, `var` is initialized to hold a `std::string`.
Accessing Values in a std::variant
Retrieving values from a `std::variant` can be done safely using `std::get` or `std::get_if`. It’s crucial to first check if the variant holds the desired type, to avoid runtime errors. The following demonstrates how to safely access the value:
if (std::holds_alternative<int>(var)) {
std::cout << std::get<int>(var) << std::endl;
}
This uses `std::holds_alternative` to check if `var` contains an `int` before trying to retrieve it.
Setting a Value
You can change the type held by a `std::variant` using an assignment. Upon assignment, it automatically converts the active member. Here’s an example:
var = 42; // Switch to int
After this operation, `var` now holds an `int` value.
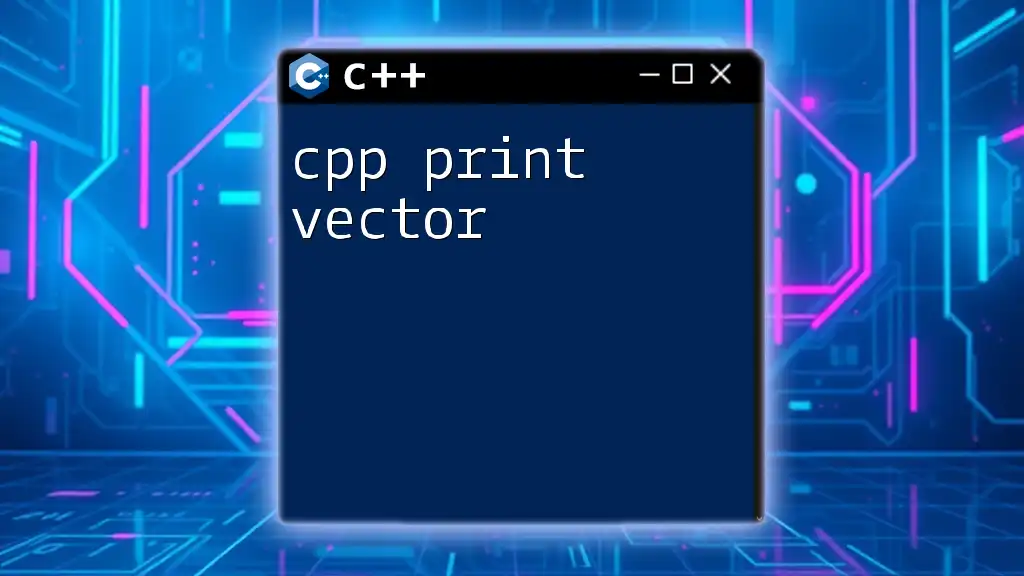
Advanced Features and Functions
Visitor Pattern with std::variant
The visitor pattern allows applying a function based on the current type of a variant. Using `std::visit`, you can implement different behaviors depending on which type is active. Here’s how to utilize it:
struct Visitor {
void operator()(int x) const { std::cout << "int: " << x << '\n'; }
void operator()(const std::string& x) const { std::cout << "string: " << x << '\n'; }
};
std::visit(Visitor{}, var);
In this example, `std::visit` calls the appropriate operator based on what type `var` currently holds.
Comparison and Relational Operators
Comparing two `std::variant` instances can be done using operators like `==`, `!=`, and `<`, among others. This allows you to compare the active types and their values directly. Consider the following example:
std::variant<int, std::string> var1 = 10;
std::variant<int, std::string> var2 = "Hello";
You can check equality as follows:
if (var1 == var2) {
std::cout << "Variants are equal." << std::endl;
} else {
std::cout << "Variants are not equal." << std::endl;
}
Error Handling with std::variant
When accessing a variant, it’s essential to handle potential errors that arise from incorrect type access. Utilizing `std::bad_variant_access`, enables you to catch these errors gracefully. Here’s a short example:
try {
std::cout << std::get<int>(var2) << std::endl;
} catch (const std::bad_variant_access&) {
std::cout << "Error: Incorrect type access!" << std::endl;
}
This mechanism ensures your program doesn’t crash due to incorrect type access but rather handles the situation smoothly.
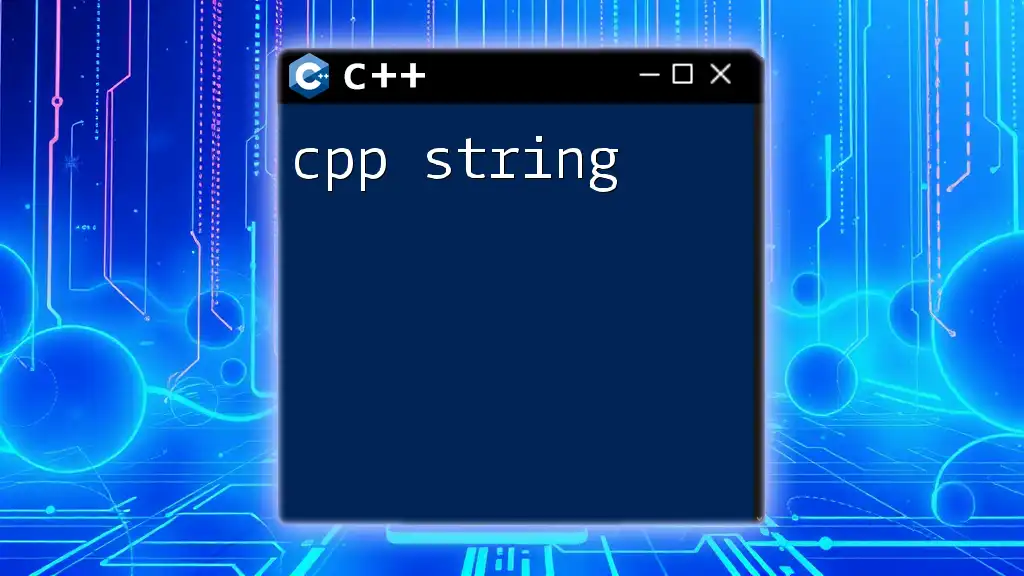
Use Cases for std::variant
Practical Applications in Real Projects
`std::variant` is especially useful in scenarios where you need to deal with multiple data types. For example, in a JSON parser, you can use `std::variant` to store various types of data values (strings, integers, etc.) without relying on cumbersome conditional checks.
Comparing std::variant with Other Alternatives
When dealing with different scenarios, `std::variant`, `std::any`, and `std::optional` each have their own strengths. While `std::any` allows for storing any type without type safety, it can lead to runtime errors. On the other hand, `std::optional` is great for representing optional values but only allows one type. Thus, choose `std::variant` when you need a type-safe storage solution for multiple distinct types.
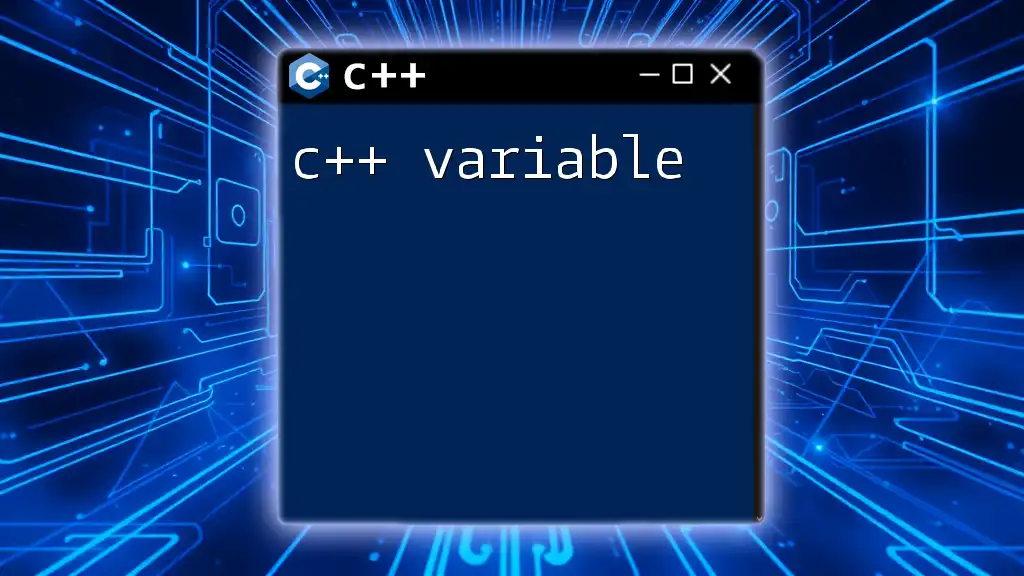
Best Practices and Tips
Choosing Types for std::variant
When defining types for a `std::variant`, consider the frequency of use and the likelihood of being active. It is best practice to keep variant types related in functionality to avoid confusion and maintain code clarity.
Performance Considerations
While `std::variant` offers great flexibility and type safety, be mindful of performance. The memory footprint of a variant is determined by the size of its largest member. When using large types as the base types of variants, this could lead to increased memory usage.
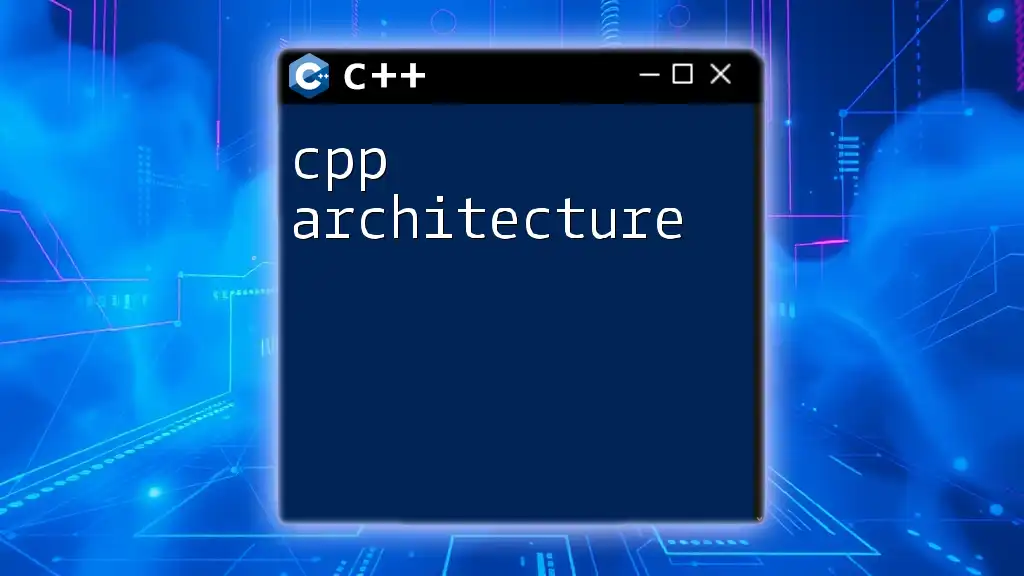
Conclusion
In summary, C++ variants enhance your programming toolbox by allowing you to create robust and type-safe data structures. By mastering `std::variant`, you can handle complex scenarios more effectively and reduce errors in your code. Take the time to explore this feature further as it not only strengthens your C++ skills but also prepares you for more advanced programming challenges. Engage with the community by sharing your experiences and implementations using `std::variant`, and continue to practice to deepen your understanding of its powerful capabilities.