In C++, you can define a triangle using a simple class that stores the lengths of its sides and includes a method to calculate the area using Heron's formula.
#include <iostream>
#include <cmath>
class Triangle {
public:
double a, b, c;
Triangle(double side1, double side2, double side3) : a(side1), b(side2), c(side3) {}
double area() {
double s = (a + b + c) / 2;
return sqrt(s * (s - a) * (s - b) * (s - c));
}
};
int main() {
Triangle tri(3, 4, 5);
std::cout << "Area: " << tri.area() << std::endl;
return 0;
}
Understanding the CPP Triangle
What is the CPP Triangle?
The CPP Triangle represents a vital framework for evaluating and optimizing C++ applications. This triangle consists of three key components: Performance, Functionality, and Code Maintainability. Each vertex of the triangle signifies an essential quality that every programmer should strive to balance in their projects.
Components of the CPP Triangle
By keeping a focus on these elements, developers can ensure that their applications run efficiently, remain functional, and are maintainable over time.
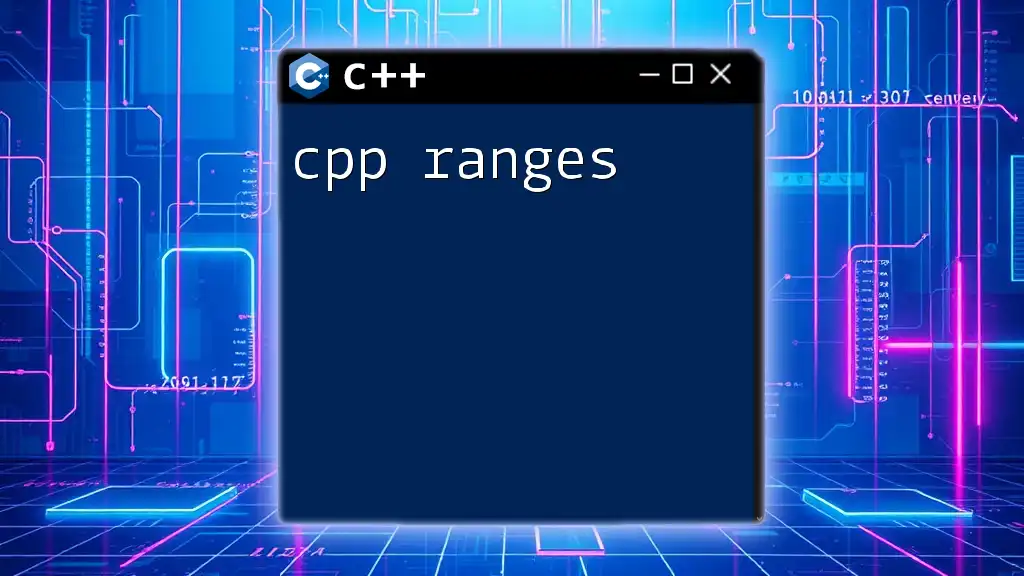
Performance in CPP
Why Performance Matters
Performance is often the first consideration in software development. Slow applications can lead to poor user experiences and can impact overall satisfaction. For instance, a delay in a real-time gaming application can significantly diminish user enjoyment, while a sluggish database query could lead to frustrating experiences during data retrieval.
Optimizing Performance in CPP
Using Efficient Data Structures
Selecting the right data structure is fundamental to achieving high performance. For example, when deciding between `std::vector` and `std::list`, you might choose `std::vector` for quick random access and iteration efficiency:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
In this example, using `std::vector` allows fast access and iteration compared to `std::list`, which is better suited for frequent insertions and deletions.
Memory Management
Effective memory management is crucial for optimizing performance. Dynamic memory allocation can lead to enhanced efficiency, especially in large applications:
#include <iostream>
int main() {
int* ptr = new int(5); // dynamic allocation
std::cout << *ptr << std::endl;
delete ptr; // free the allocated memory
return 0;
}
Improper use of dynamic memory can lead to memory leaks and fragmentation; thus, always remember to delete pointers after use.
Performance Measurement Tools
Understanding application performance is greatly aided by tools that analyze program efficiency. For instance, Valgrind helps identify memory leaks and profiling performance, while gprof facilitates performance analysis through function call graphs. Here is an elementary example of how to use `gprof` with a simple C++ program:
#include <iostream>
void heavyComputation() {
for (int i = 0; i < 1000000; ++i) {
// Simulating heavy computation
}
}
int main() {
heavyComputation();
return 0;
}
Compile the program with `-pg` to enable profiling:
g++ -pg example.cpp -o example
./example
gprof example gmon.out > analysis.txt
This generates a text file containing performance metrics, allowing developers to understand where optimizations are needed.
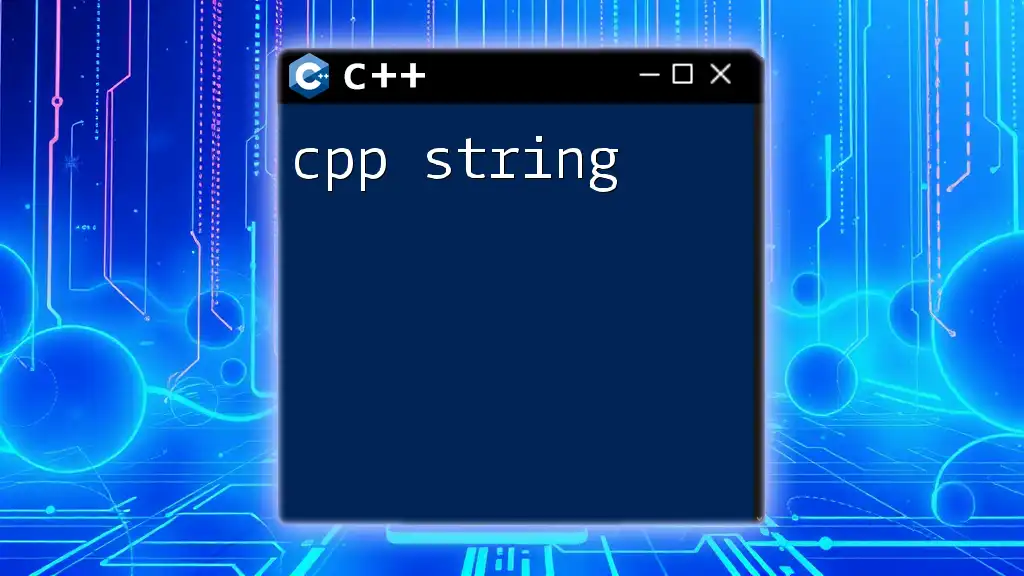
Functionality in CPP
Defining Functionality
Functionality refers to the suite of features that an application provides. Ensuring rich functionality means an application serves a broader and more complex set of user needs. This often translates to more complex code structures, but also a more powerful application.
Building Functionality into CPP Applications
Function Creation
Creating well-defined functions enhances modularity and reusability. For example, consider the following simple function for calculating the factorial of a number:
#include <iostream>
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
int main() {
std::cout << "Factorial of 5 is: " << factorial(5) << std::endl;
return 0;
}
This recursive function showcases modular design, making it easier to understand and maintain.
Utilizing Libraries and Frameworks
Leveraging external libraries can significantly enhance application functionality without reinventing the wheel. For example, using the Boost library can simplify many tasks:
#include <boost/algorithm/string.hpp>
#include <iostream>
int main() {
std::string str = "Boost Libraries";
boost::to_upper(str);
std::cout << str << std::endl; // Outputs: BOOST LIBRARIES
return 0;
}
By integrating such libraries, developers can expand their app's capabilities significantly.
Functionality Testing
To ensure the functionality is maintained, rigorous testing is necessary. Unit testing allows you to verify individual components of your application. Here's a basic unit test example using Google Test:
#include <gtest/gtest.h>
int add(int a, int b) {
return a + b;
}
TEST(AddTest, HandlesPositiveNumbers) {
EXPECT_EQ(add(1, 2), 3);
}
int main(int argc, char **argv) {
::testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
Regular testing during the development cycle ensures that features remain intact and any bugs are caught early.
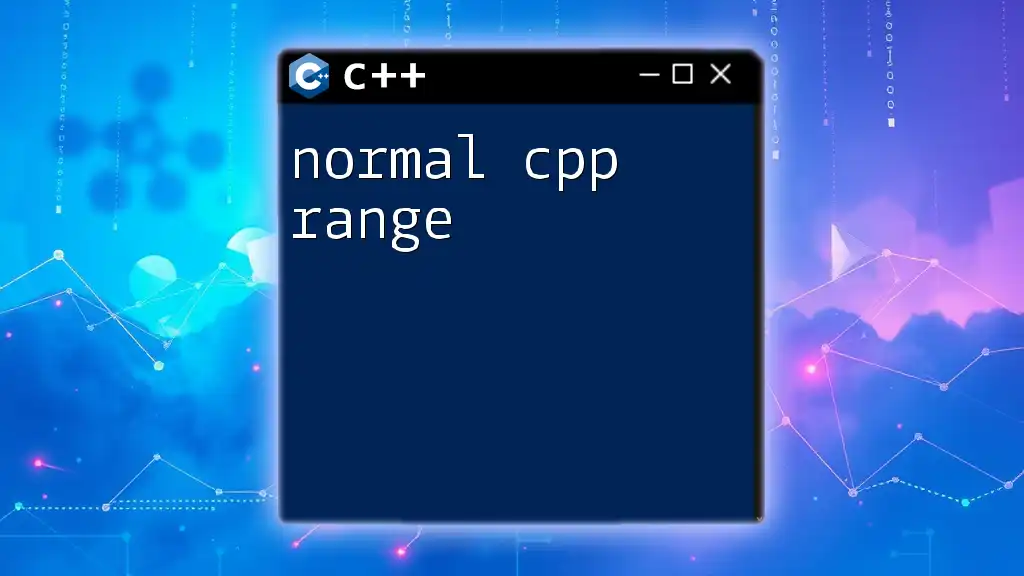
Code Maintainability
Understanding Code Maintainability
Maintainability refers to how easily a developer can modify and enhance the codebase over time. As projects grow, the ability to manage code efficiently becomes paramount, especially when transitioning code ownership or adapting to changing requirements.
Best Practices for Maintainable Code
Code Comments and Documentation
Proper commenting and documentation are essential in making code understandable. Consider the following snippet:
#include <iostream>
// Function to find the maximum of two numbers
int max(int a, int b) {
return (a > b) ? a : b; // Using a ternary operator for conciseness
}
int main() {
std::cout << max(3, 4) << std::endl; // Outputs: 4
return 0;
}
Here, comments provide clarity around what the function does, making maintenance easier.
Consistent Naming Conventions
Using meaningful and consistent naming conventions enhances readability. For example, using `studentCount` in place of `sc` or `x` can make code easier to understand at a glance.
Refactoring and Code Review
Refactoring allows developers to improve the structure of existing code without changing its functionality. A classic example is simplifying nested loops into functions or optimizing algorithms. Here is a before and after example of a nested loop for finding duplicates in a list:
Before Refactoring:
#include <vector>
#include <iostream>
void findDuplicates(const std::vector<int>& vec) {
for (size_t i = 0; i < vec.size(); ++i) {
for (size_t j = i + 1; j < vec.size(); ++j) {
if (vec[i] == vec[j]) {
std::cout << "Duplicate found: " << vec[i] << std::endl;
}
}
}
}
After Refactoring:
#include <vector>
#include <unordered_set>
#include <iostream>
void findDuplicates(const std::vector<int>& vec) {
std::unordered_set<int> seen;
for (auto num : vec) {
if (seen.find(num) != seen.end()) {
std::cout << "Duplicate found: " << num << std::endl;
} else {
seen.insert(num);
}
}
}
This refactoring simplifies the search process with improved efficiency.
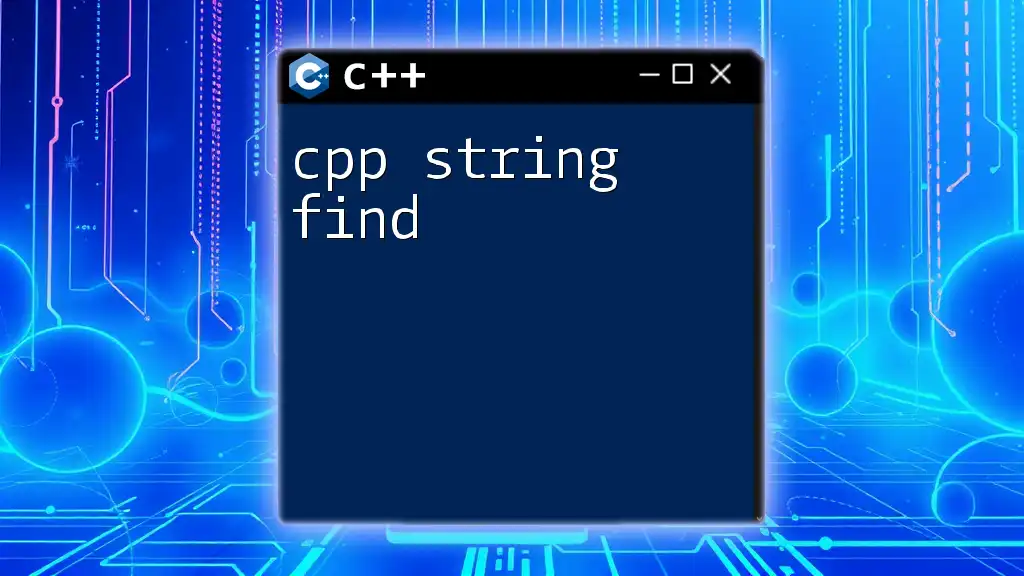
Putting It All Together: Balancing the Triangle
Achieving Harmony in Performance, Functionality, and Maintainability
Balancing the three components of the CPP Triangle is crucial to producing high-quality applications. Developers often face challenges in this area, particularly when a new feature may compromise performance. The key to overcoming these challenges lies in iterative development, allowing developers to assess and re-evaluate code at every stage.
In summary, achieving a balance means continuously measuring your application against the triangle's vertices and making informed decisions to enhance your CPP code.
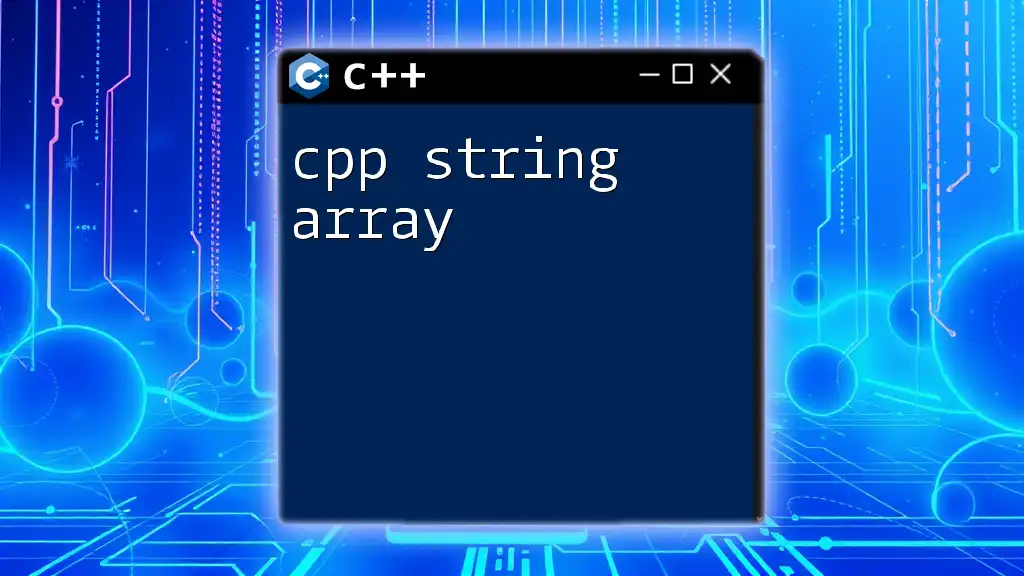
Conclusion
Mastering the CPP Triangle involves understanding the intricate relationship between performance, functionality, and maintainability. As you deepen your knowledge and practice in these areas, you will become more adept at writing efficient, functional, and maintainable C++ code.
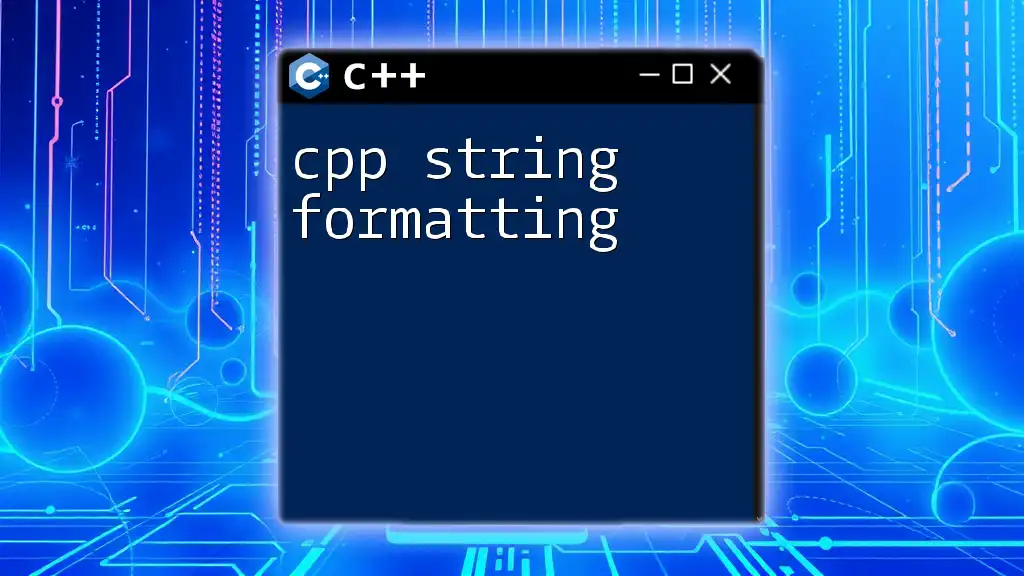
Additional Resources
For further exploration, consider checking out these recommended titles and online resources focused on CPP development and best practices.
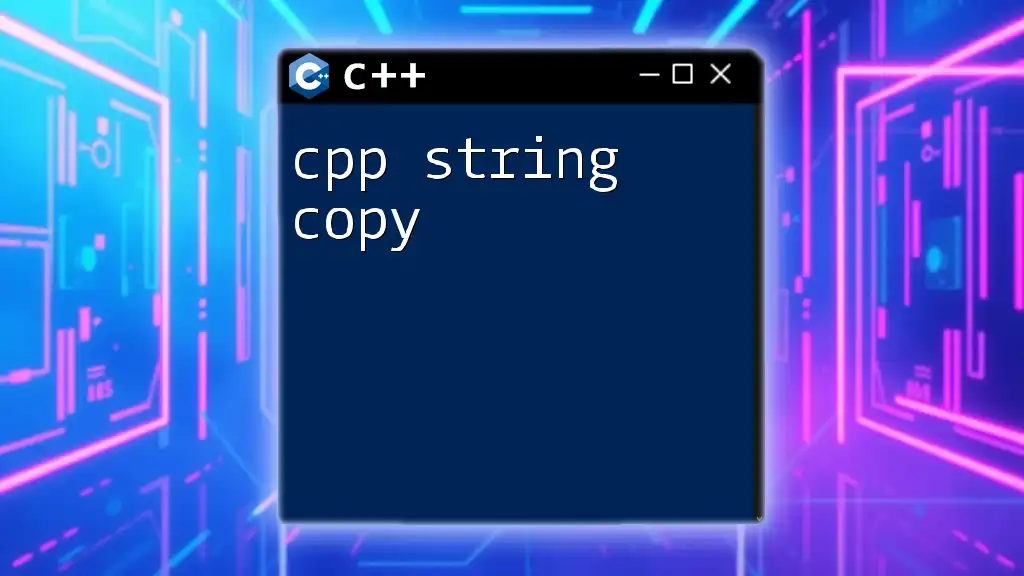
FAQ Section
Should you have common questions about CPP and the CPP Triangle, ensure you revisit your challenges regularly and seek community support for additional insights.