In C++, the normal range is typically defined as the set of possible values that a variable can hold based on its data type, which can be illustrated using the `std::numeric_limits` class to find the minimum and maximum values.
Here's a code snippet that demonstrates how to get the normal range for an integer:
#include <iostream>
#include <limits>
int main() {
std::cout << "Normal Range for int: "
<< "Min = " << std::numeric_limits<int>::min()
<< ", Max = " << std::numeric_limits<int>::max()
<< std::endl;
return 0;
}
What are Ranges in C++?
Ranges are a powerful feature in C++ that allow developers to handle collections of data more efficiently and expressively. They provide a way to simplify operations on sequences, thereby enhancing the readability and maintainability of the code. Ranges are a part of the C++ Standard Library, integrating with algorithms, iterators, and containers to create a more cohesive framework for manipulation.
Types of Ranges
Understanding the different types of ranges helps programmers choose the right tool for the job:
- Input Ranges: These allow data to be read from a sequence, but not modified. They provide a way to iterate through elements in a readable manner.
- Output Ranges: These enable writing or modifying data in a sequence without having to manage the underlying storage directly.
- Forward Ranges: Designed to support the ability to read from a range multiple times. They provide efficient access and support non-copyable types.
- Bidirectional Ranges: These are similar to forward ranges but also allow reverse iteration, making it easier to navigate through data in both directions.
- Random Access Ranges: These provide the highest level of flexibility, allowing access to elements in constant time regardless of their position within the range.
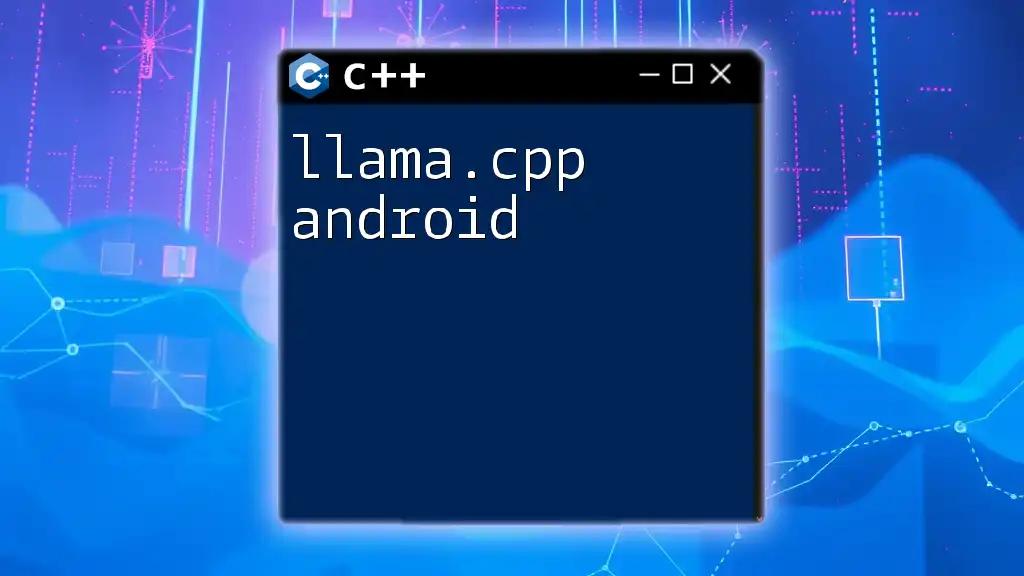
Understanding Normal C++ Range
A normal cpp range refers to a standard, continuous sequence of elements that can be traversed. This concept is essential for implementing many algorithms and data structures, as it provides a clear and efficient way to manage collections.
Characteristics of a normal range include:
- Begin and End: A normal range has a defined starting point and endpoint, facilitating traversal using iterators.
- Contiguity: The elements within a normal range are typically stored contiguously in memory, allowing for efficient access and modification.
By understanding normal ranges, developers can take advantage of the optimizations provided by C++ for data handling.
Syntax for Creating Normal Ranges
Creating a normal range is straightforward. Here’s the basic syntax to initialize a normal range using the C++ Standard Library:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto normalRange = std::ranges::subrange(numbers.begin(), numbers.end());
for (auto num : normalRange) {
std::cout << num << " ";
}
return 0;
}
In this example, `std::ranges::subrange` creates a normal range from the beginning to the end of the `numbers` vector. Each element in `normalRange` can then be accessed simply using a range-based for loop.
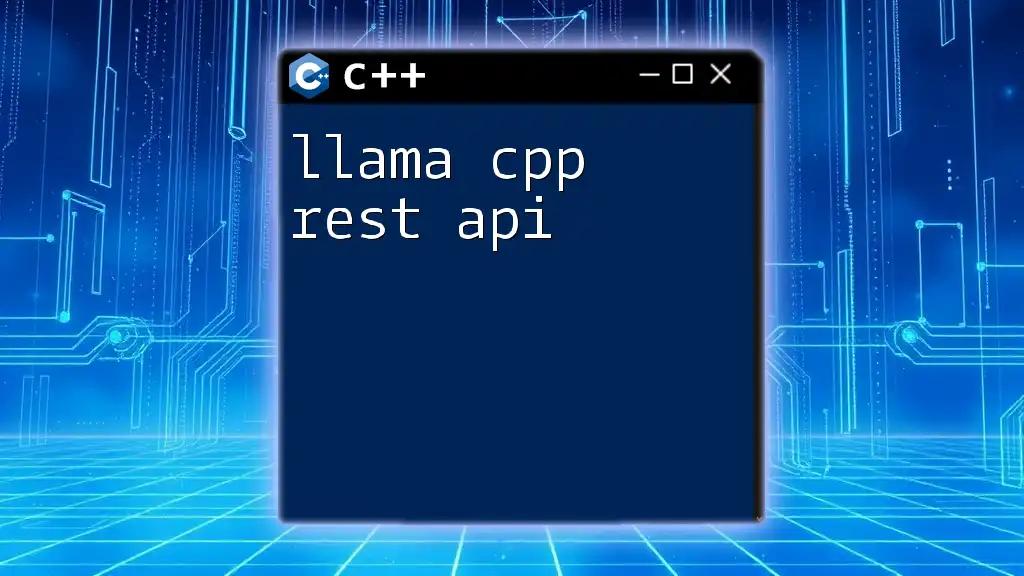
How to Use Ranges in C++
Using normal ranges in C++ allows for cleaner and more concise code. Here are a few common operations:
Iterating through a Range
Iteration is made simple with ranges. Instead of using traditional loops, the range-based for loop provides clarity and ease:
#include <vector>
#include <iostream>
int main() {
std::vector<int> items = {10, 20, 30, 40, 50};
for (auto it = items.begin(); it != items.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
This snippet effectively prints each item in the `items` vector. However, with ranges, this can be simplified even further.
Modifying Elements in a Range
Modifying elements can also be done seamlessly. Here’s how to double each value in a range:
#include <vector>
#include <ranges>
#include <iostream>
int main() {
std::vector<int> values = {1, 2, 3, 4, 5};
for (auto& val : values) {
val *= 2; // Doubling the value
}
for (const auto& val : values) {
std::cout << val << " ";
}
return 0;
}
In this example, each value in the `values` vector is doubled, showcasing how easily ranges allow for direct modification.
Examples of Common Range Operations
Finding Elements
Using ranges with algorithms like `std::find` can be done effortlessly:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> items = {10, 20, 30, 40, 50};
auto it = std::ranges::find(items, 30);
if (it != items.end()) {
std::cout << "Found: " << *it << std::endl;
}
return 0;
}
In this case, `std::ranges::find` is used to locate the first occurrence of the number 30 in the sequence, illustrating the power of ranges combined with algorithms.
Transforming a Range
Applying transformations to ranges can be accomplished with views:
#include <vector>
#include <ranges>
#include <iostream>
int main() {
std::vector<int> values = {1, 2, 3};
auto transformed = values | std::views::transform([](int n) { return n * 2; });
for (auto val : transformed) {
std::cout << val << " ";
}
return 0;
}
The example demonstrates how to create a new range that contains the results of doubling each value in the `values` vector.
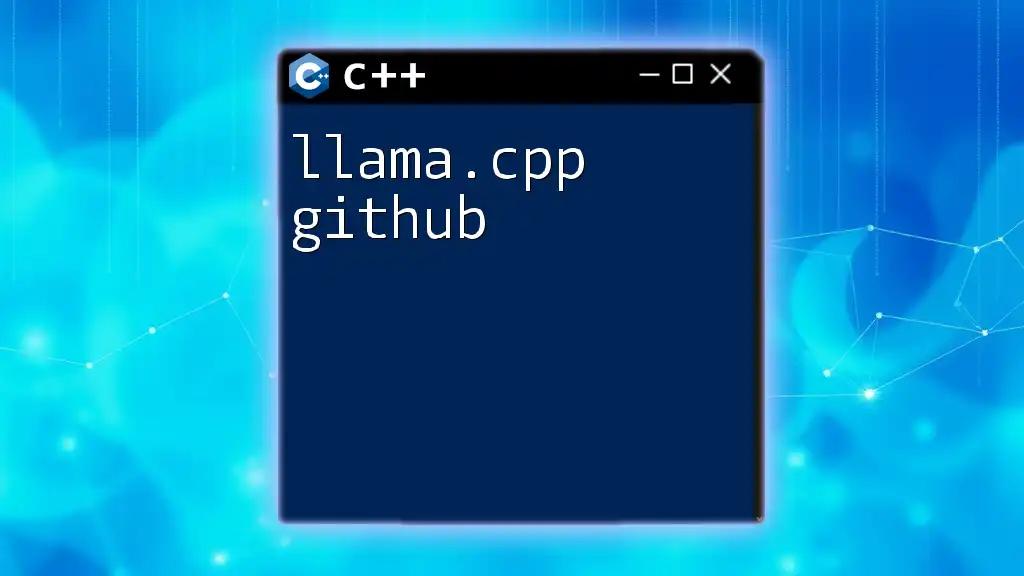
Best Practices for Using Normal Ranges
When working with normal cpp ranges, consider the following best practices:
- Select the Right Range Type: Understand when to use normal ranges versus input, output, or random access ranges, based on the task's requirements.
- Keep Performance in Mind: Analyze performance impacts, particularly with larger datasets. For instance, prefer algorithms that operate directly on ranges to reduce overhead.
- Avoid Nested Loops: Whenever possible, use ranges to eliminate unnecessary nested loops for greater readability and maintainability.
Troubleshooting Common Issues
While using normal ranges, you might encounter several common issues:
- Iterator Invalidations: Modifying a range during iteration can lead to undefined behavior. Ensure the range remains unchanged until the iteration completes.
- Incorrect Type Errors: If you pass incompatible types to range algorithms or views, it can lead to compile-time errors. Ensure type consistency throughout.
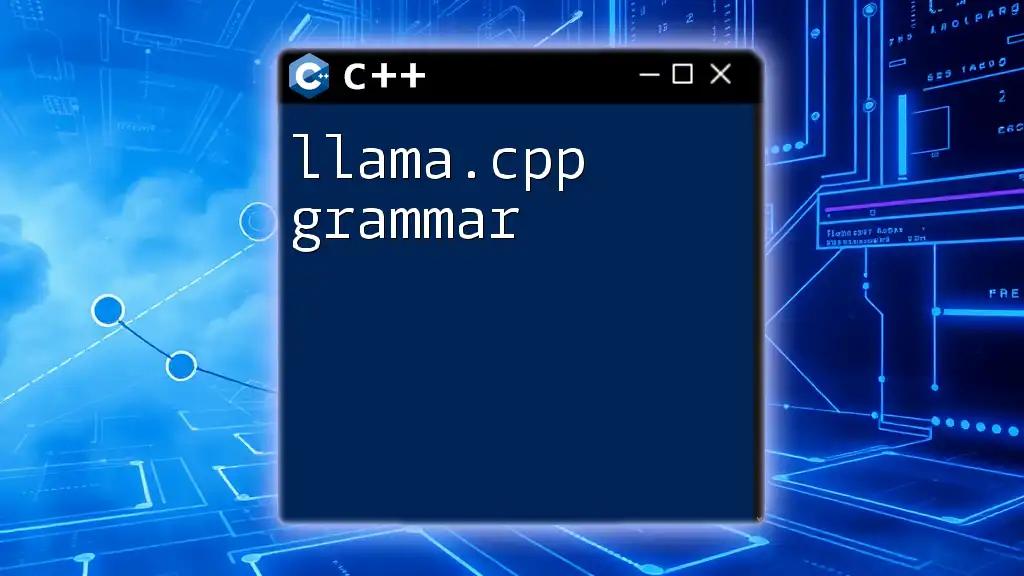
Advanced Range Manipulation
For experienced developers, creating custom iterable objects can enhance flexibility:
Custom Iterable Objects
To create a custom range, you define required operations and ensure that the type adheres to the range requirements:
#include <iostream>
#include <iterator>
class MyRange {
public:
class Iterator {
int current;
public:
explicit Iterator(int start) : current(start) {}
int operator*() const { return current; }
const Iterator& operator++() { ++current; return *this; }
bool operator!=(const Iterator& other) const { return current != other.current; }
};
MyRange(int start, int end) : start(start), end(end) {}
Iterator begin() const { return Iterator(start); }
Iterator end() const { return Iterator(end); }
private:
int start, end;
};
int main() {
MyRange range(1, 5);
for (auto num : range) {
std::cout << num << " ";
}
return 0;
}
This snippet defines a simple custom range that can be iterated over, demonstrating how to control the data flow.
Combining Ranges
Sometimes, ranges can be combined to perform multiple operations at once:
#include <vector>
#include <ranges>
#include <iostream>
int main() {
std::vector<int> values = {1, 2, 3, 4, 5};
auto combinedRange = values | std::views::transform([](int n) { return n * 2; })
| std::views::filter([](int n) { return n > 5; });
for (auto val : combinedRange) {
std::cout << val << " ";
}
return 0;
}
In this example, the combined range first transforms values and then filters them based on the specified criteria—showing how powerful and versatile ranges can be.
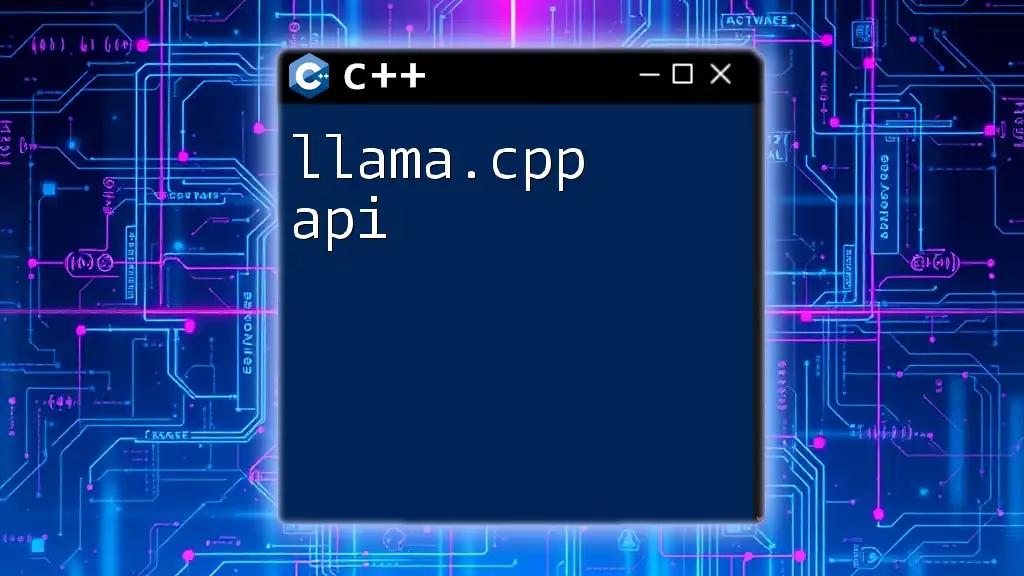
Conclusion
Understanding and utilizing normal cpp ranges significantly enhances programming efficiency in C++. By mastering ranges, developers can write cleaner, more maintainable code while leveraging the power of the C++ Standard Library. Practicing the examples and techniques discussed in this guide will solidify your skills, paving the way for more advanced concepts and applications in C++. Remember, as you continue to explore C++, ranges are a vital tool that can streamline your coding practices.