In 2024, C++ continues to evolve with new features that enhance performance and code clarity, such as the introduction of the `std::format` library for formatted output.
#include <format>
#include <iostream>
int main() {
int value = 42;
std::string formatted = std::format("The answer is: {}", value);
std::cout << formatted << std::endl;
return 0;
}
Understanding C++ Evolution
The Importance of C++ Standards
C++ is a dynamic and evolving language, which ensures it stays relevant in meeting modern programming needs. Each new standard, set by the International Organization for Standardization (ISO), introduces crucial updates. These changes are important as they improve performance, efficiency, and foster modern programming practices. For example, improvements in the library often provide more robust solutions, while syntactical enhancements can lead to cleaner and more readable code.
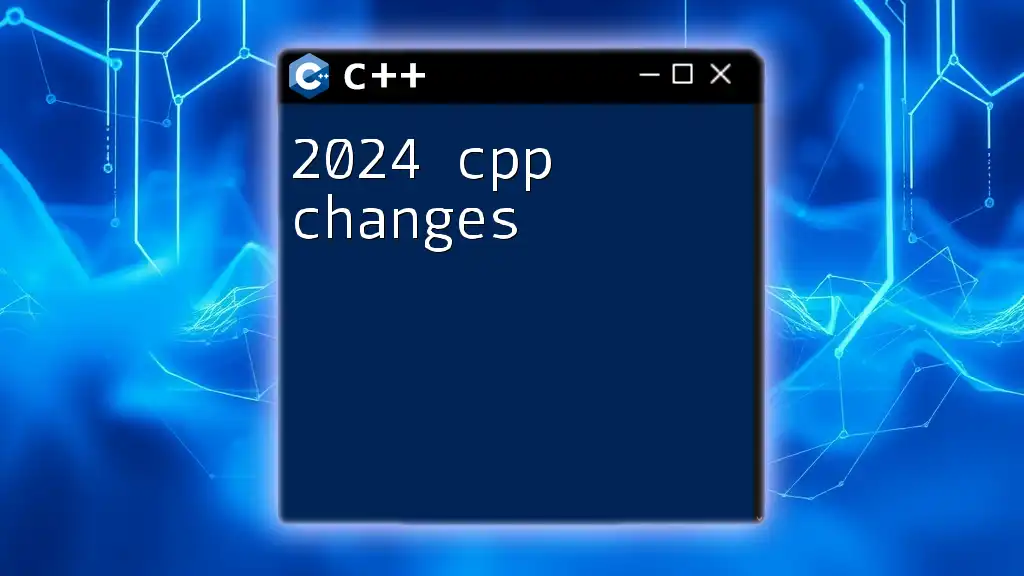
Key Features Introduced in C++ 2024
Swift Compilation Improvements
One of the most significant enhancements in C++ 2024 involves improvements in compilation speed. These advancements reduce compilation time, allowing developers to spend more time writing code and less time waiting for builds.
For example, with the revised compilation process, code structures that were traditionally cumbersome to compile can now be handled more efficiently:
#include <iostream>
void sampleFunction() {
std::cout << "Fast compilation!" << std::endl;
}
Developers can enjoy a faster turnaround between writing code and running it, significantly boosting productivity.
Enhanced Module Support
Modules constitute a substantial addition to C++, introduced to streamline code organization and accelerate compilation processes. Unlike traditional header files, modules allow for better encapsulation and isolation of components, which improves build times and reduces compilation overhead.
Here’s how you might define and use a module in C++ 2024:
export module my_module;
export void hello() {
std::cout << "Hello from my module!" << std::endl;
}
With modules, you can clearly define the interface of your code, which enhances maintainability and reduces the risk of name collisions between various components in larger projects.
New Ranges Library Features
The ranges library has seen exciting updates. New capabilities allow developers to work with data more intuitively and effectively. These enhancements focus on providing sophisticated views and algorithms that can handle collections of data with minimal boilerplate.
For instance, you can use the new ranges functionalities to easily transform collections:
#include <ranges>
#include <vector>
#include <iostream>
void printSquares(const std::vector<int>& nums) {
auto squares = nums | std::views::transform([](int n) { return n * n; });
for (int x : squares) {
std::cout << x << " ";
}
}
This new syntax simplifies functional programming constructs by enabling point-free style and making your code more expressive.
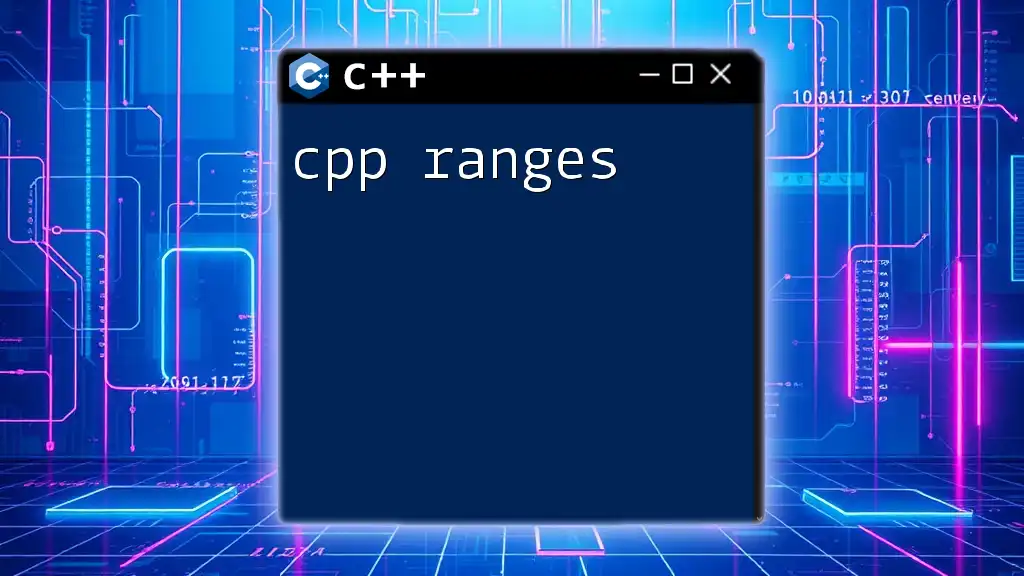
Language Enhancements
Improved Support for Coroutines
C++ 2024 offers improved support for coroutines, which helps in managing asynchronous operations with less complexity and more clarity. Coroutines allow functions to be paused and resumed, which is particularly useful in scenarios where non-blocking calls are necessary, such as in GUI applications or high-performance server code.
Here’s an example that showcases the declaration of a coroutine:
#include <coroutine>
struct Generator {
struct promise_type {
auto get_return_object() { return Generator{}; }
auto initial_suspend() { return std::suspend_always{}; }
auto final_suspend() noexcept { return std::suspend_always{}; }
void unhandled_exception() {}
};
};
With improvements in syntax, handling asynchronous flows becomes more manageable, making it easier for developers to implement complex logic without getting mired in callback hell.
Enhanced constexpr Capabilities
C++ 2024 expands the capabilities of `constexpr` functions significantly. More constructs can now be evaluated at compile-time, which results in faster execution and improved program efficiency. This is especially useful when performance is critical, as computations can be offloaded to the compilation phase rather than runtime.
For example, you can now create complex templated `constexpr` functions:
constexpr int factorial(int n) {
return n <= 1 ? 1 : n * factorial(n - 1);
}
The ability to compute values at compile time facilitates optimization and leads to cleaner, more predictable code.
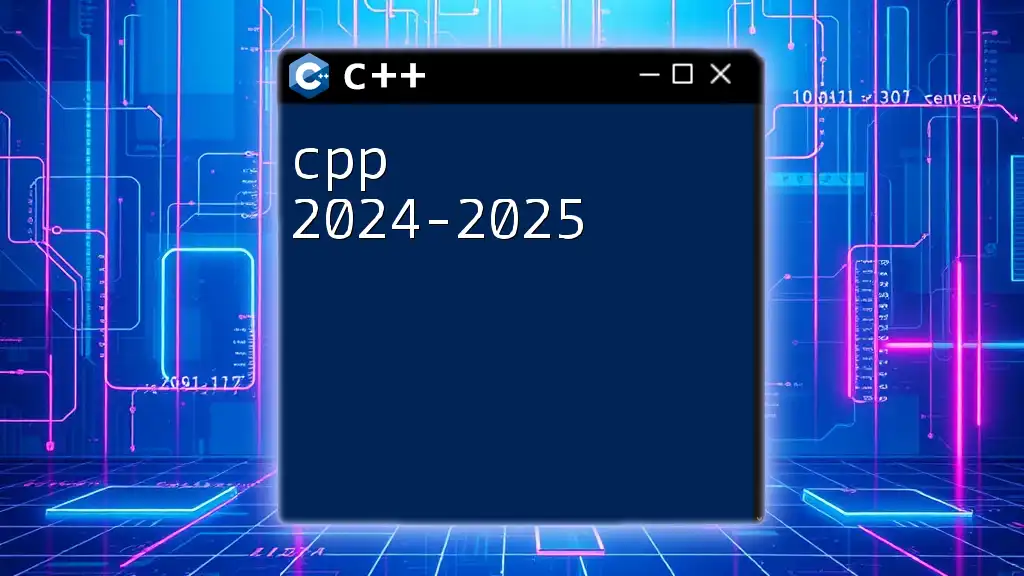
Performance Improvements
Optimizations for Standard Library
C++ 2024 has introduced several optimizations across the Standard Library, enhancing not only performance but also usability. These optimizations allow existing data structures and algorithms to run faster and more efficiently.
For instance, improvements in the implementation of `std::vector` have been made, meaning that operations which often cause performance bottlenecks, such as insertion operations, can now be handled with improved efficiency:
// Example highlighting improved std::vector performance
std::vector<int> myVector;
myVector.reserve(1000); // Optimized for insertion performance
By leveraging these improvements, programmers can create more responsive applications that handle large datasets without compromising on performance.
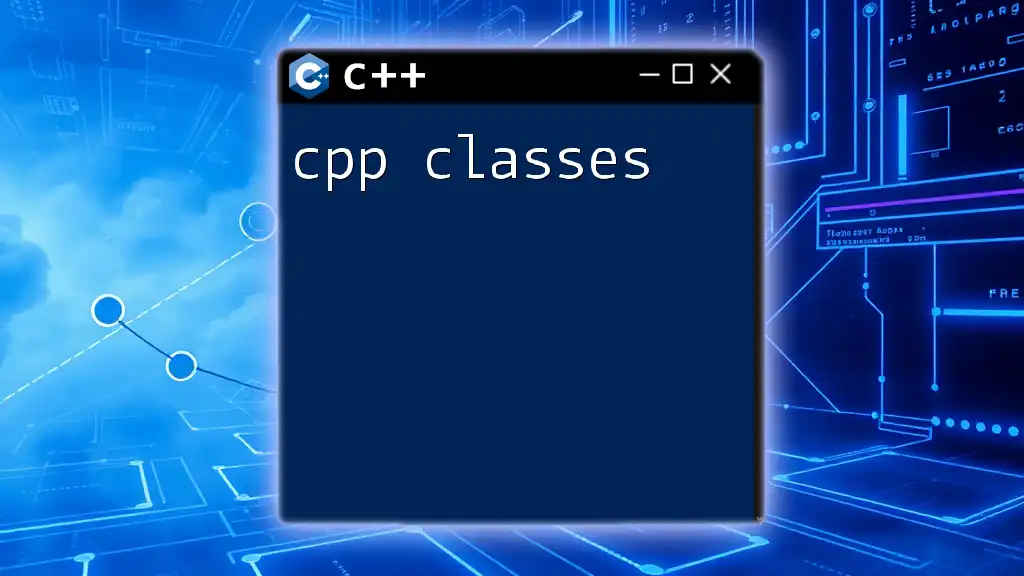
Compatibility and Transition Tips
Migrating from Older C++ Versions
Transitioning to C++ 2024 can be a significant step, especially for developers accustomed to older standards. To ensure a smooth migration, understanding potential pitfalls and embracing new practices is key.
One common issue may arise from deprecated features, such as traditional raw pointers, which can lead to memory mismanagement.
Best practices during the transition include:
- Adopting smart pointers instead of raw pointers for better memory management.
- Utilizing modules instead of headers to reduce compilation dependencies and improve code organization.
Here’s an example highlighting deprecated features:
// Highlighting deprecated features in older versions
// Using raw pointers instead of smart pointers
void oldExample() {
int* pInt = new int(10); // Warning! Use unique_ptr<int> instead
delete pInt;
}
By understanding these shifts, you can write cleaner, safer, and more efficient code with C++ 2024.
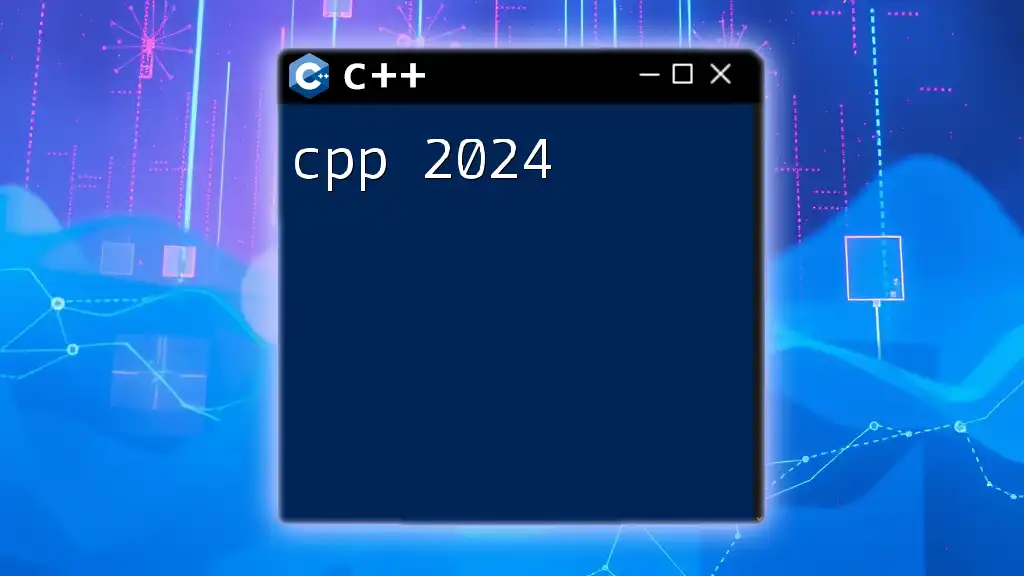
Conclusion
The C++ 2024 standard brings significant updates that enhance performance, improve productivity, and make the language more intuitive. Emphasizing newer paradigms like modules and coroutines, these changes equip developers to tackle modern programming challenges with greater ease and efficiency.
Continuous learning remains essential to keep up with these advancements, and embracing the newest C++ standards will undoubtedly position you favorably in today’s fast-evolving tech landscape.
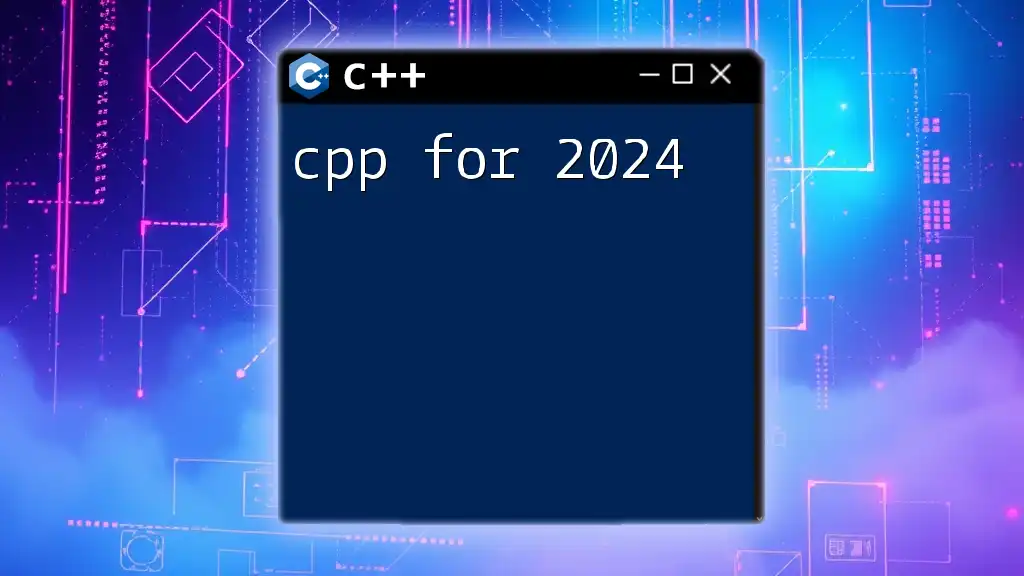
Call to Action
To stay ahead, join our community or sign up for courses that delve deep into the latest C++ features. Equip yourself with the necessary skills and knowledge to fully harness the power of C++ changes in 2024 and beyond.