In 2024, C++ enhancements focus on improving usability, performance, and safety, with features like modules and improved constexpr that streamline code development and maintenance.
// Example of using a module in C++
export module math_util; // Defining a module
export int add(int a, int b) {
return a + b; // Function to add two integers
}
C++ Standards and Key Enhancements in 2024
The C++23 Retrospective
C++23 brought a host of features that significantly changed how developers interact with the language. One of the most notable enhancements was `std::format`, which simplified string formatting and improved readability. Here's a quick example of how `std::format` is utilized:
#include <format>
#include <iostream>
int main() {
std::string name = "World";
std::cout << std::format("Hello, {}!", name) << std::endl;
return 0;
}
Moreover, enhancements to `constexpr` allowed for more complex computations at compile-time, greatly enhancing performance. Instead of limiting what you could do in `constexpr` functions, C++23 expanded the scope, allowing for:
constexpr int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
These features provided developers with tools to write cleaner and more efficient code.
C++24 Expectations
Looking ahead, C++24 promises to introduce several exciting features that will further elevate C++ programming. One anticipated enhancement is the expansion of ranges, enabling more expressive and user-friendly operations on collections.
By involving community feedback, the C++ Standards Committee is shaping these features to address real-world programming needs. For instance, the introduction of enhanced type traits could simplify template metaprogramming, allowing developers to write safer and more maintainable code.
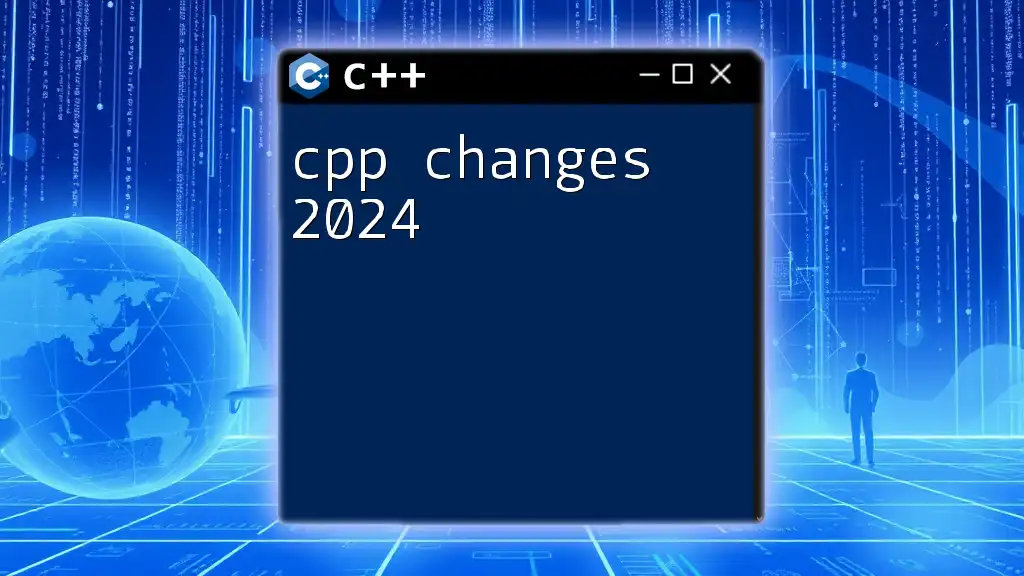
The Role of New Libraries in C++ Enhancement
Standard Library Updates
The Standard Library is pivotal to C++ enhancement, and 2024 will see several critical updates. Notably, the introduction of `std::expected` provides a robust way to handle errors without using exceptions. This can be particularly useful for APIs that might fail without the need for verbose exception handling:
#include <expected>
#include <iostream>
std::expected<int, std::string> safe_divide(int numerator, int denominator) {
if (denominator == 0) return std::unexpected("Division by zero");
return numerator / denominator;
}
int main() {
auto result = safe_divide(10, 0);
if (!result) {
std::cout << "Error: " << result.error() << std::endl;
} else {
std::cout << "Result: " << *result << std::endl;
}
return 0;
}
Third-Party Libraries
In addition to the Standard Library, several third-party libraries are enhancing C++ capabilities. Libraries such as Boost continue to provide invaluable utilities and extensions. For example, Boost’s `Boost.Spirit` enables parsing expression grammars directly in C++, while libraries like OpenCV are essential for computer vision tasks.
By integrating such libraries into your projects, you expand your toolkit significantly, making your applications more powerful.
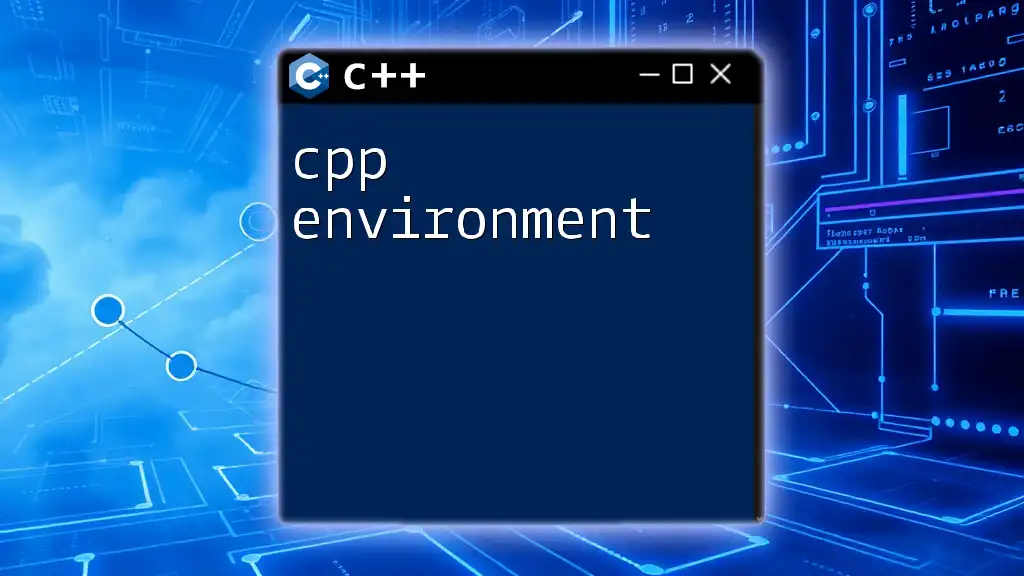
Performance Improvements in C++ 2024
Compiler Improvements
C++ compilers are expected to introduce advanced optimizations in 2024, resulting in impressive performance metrics. These optimizations facilitate faster execution times and reduced memory usage. Developers can expect tools like LLVM and GCC to improve their support for new C++ features, enabling excellent performance out of the box.
Concurrency and Parallelism
Concurrency remains one of C++’s strong suits, and improvements in this area for 2024 are noteworthy. The use of `std::jthread` offers more straightforward syntax for creating threads that join automatically:
#include <iostream>
#include <thread>
void task() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::jthread t(task); // Automatically joins on destruction
return 0;
}
This feature simplifies thread management and helps avoid common pitfalls in concurrent programming.
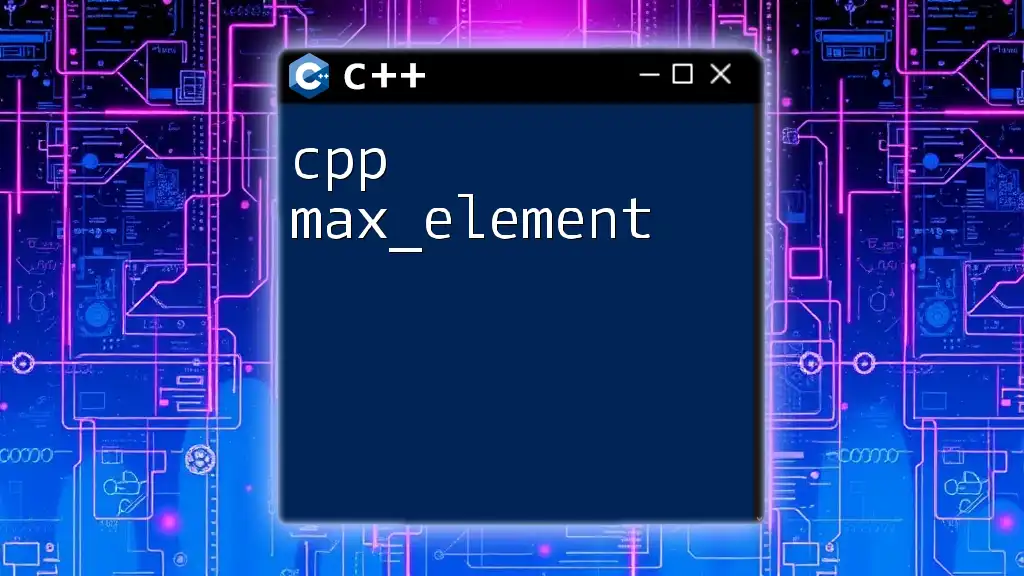
Integration of Modern Programming Concepts
Functional Programming in C++
Functional programming continues to gain traction in C++, and 2024 promises further support for this paradigm. With features such as lambda expressions, developers can write cleaner and more concise code. For example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::for_each(vec.begin(), vec.end(), [](int n) {
std::cout << n * n << " ";
});
return 0;
}
This succinctly demonstrates how to iterate and perform operations on elements in a collection without boilerplate code.
Concepts and Type Safety
The introduction of Concepts in C++20 has established a foundation for stricter type-checking, and further enhancements in 2024 are expected. Concepts allow developers to specify template requirements, promoting cleaner and easier-to-read code.
Here’s how you might enforce a concept on a template function:
#include <concepts>
#include <iostream>
template<typename T>
concept Integral = std::is_integral_v<T>;
template<Integral T>
void print_integral(T value) {
std::cout << "Integral value: " << value << std::endl;
}
int main() {
print_integral(10); // Valid
// print_integral(10.5); // Compilation error
return 0;
}
This concept ensures that the template only accepts integral types, enhancing type safety in your applications.
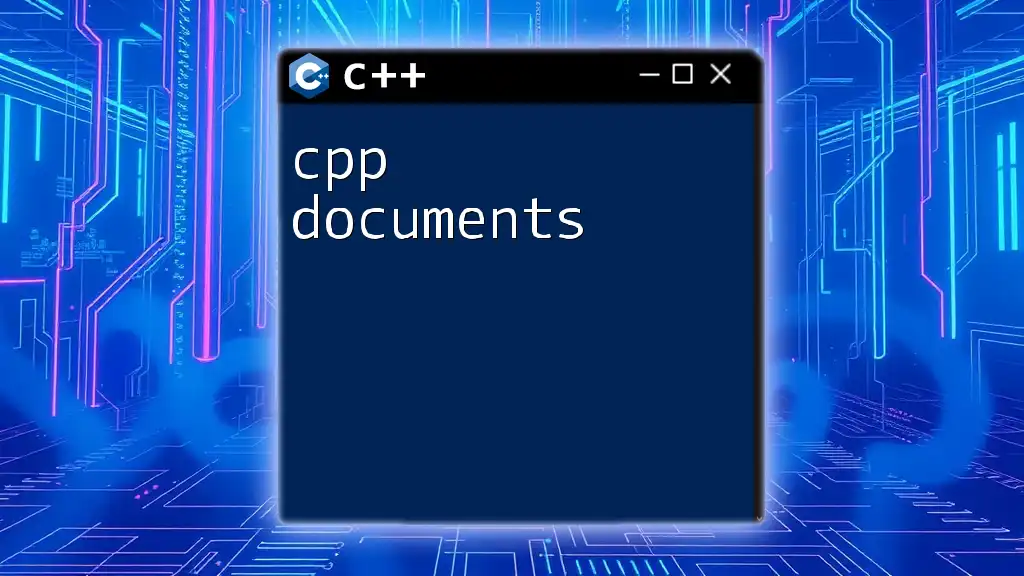
Enhancements in Error Handling and Debugging
Improved Exception Handling
C++24 introduces enhancements in exception handling that aim to reduce boilerplate and improve readability. For instance, stack unwinding changes may be introduced to streamline how resources are cleaned up during exceptions.
Debugging Tools and Techniques
With each new version of C++, debugging tools also improve. In 2024, expect advanced utilities that leverage new language features to provide deeper insights during debugging sessions. Familiarizing yourself with updated tools can significantly enhance your development experience and streamline your workflow.
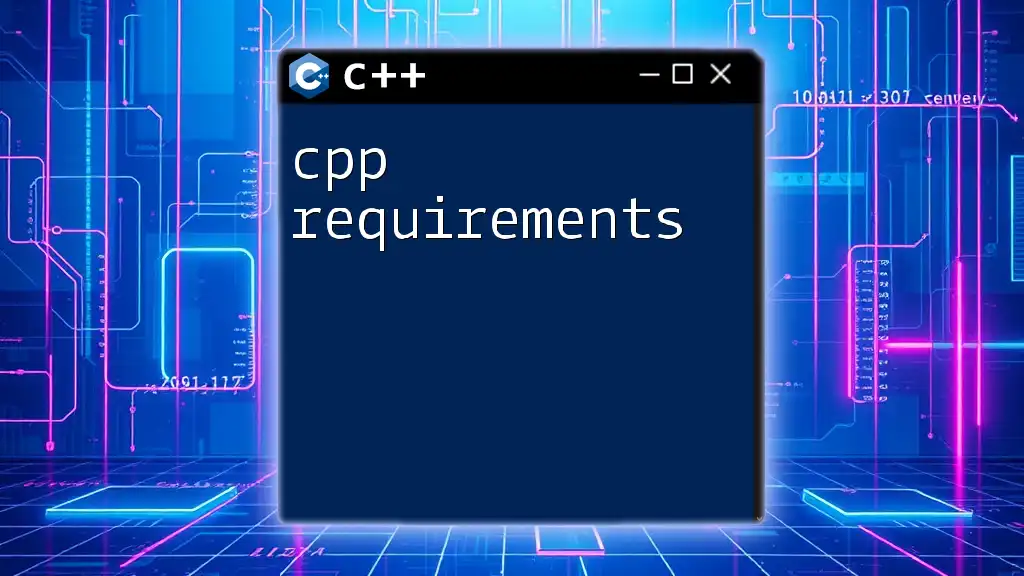
Community Contributions and Open Source
The Importance of Open Source
Open-source contributions are integral to the evolution of C++. Many of the enhancements being discussed stem from community-driven projects. It's crucial for developers to engage not only as users but as contributors to these projects, as this input helps shape the future of the language.
Participating in the C++ Community
Joining the C++ community can significantly aid your development journey. Online forums, code repositories on GitHub, and conferences are great places to learn from peers, share insights, and get feedback on your projects. Engaging with the community fosters collaboration and innovation among developers.
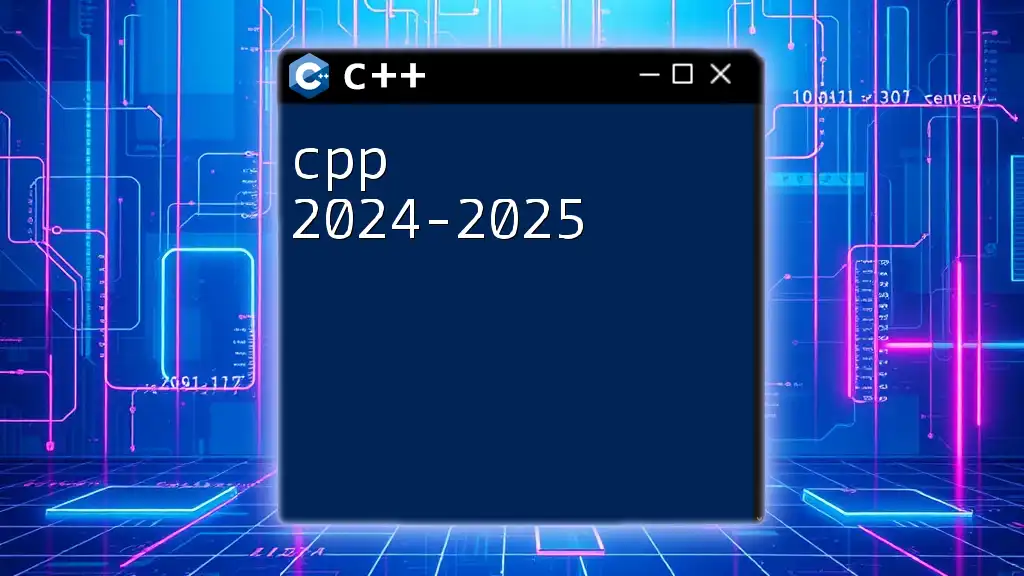
Conclusion
C++ enhancement in 2024 is poised to make a significant impact on the way developers approach programming. By leveraging the improvements and features introduced in C++23 and expected in C++24, developers can create more powerful, efficient, and maintainable code. Stay curious, experiment with the new features, and engage with the vibrant community of C++ developers to navigate this exciting evolution seamlessly.
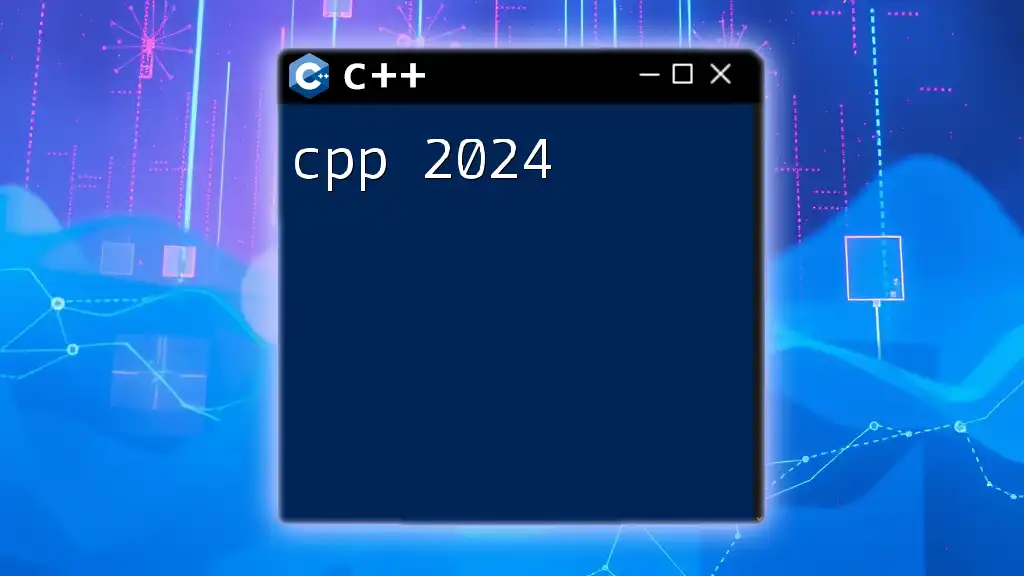
References
For further learning, consider exploring official documentation, C++ community discussions, and other educational resources that dive deeper into these topics. Engage actively to remain at the forefront of C++ development.
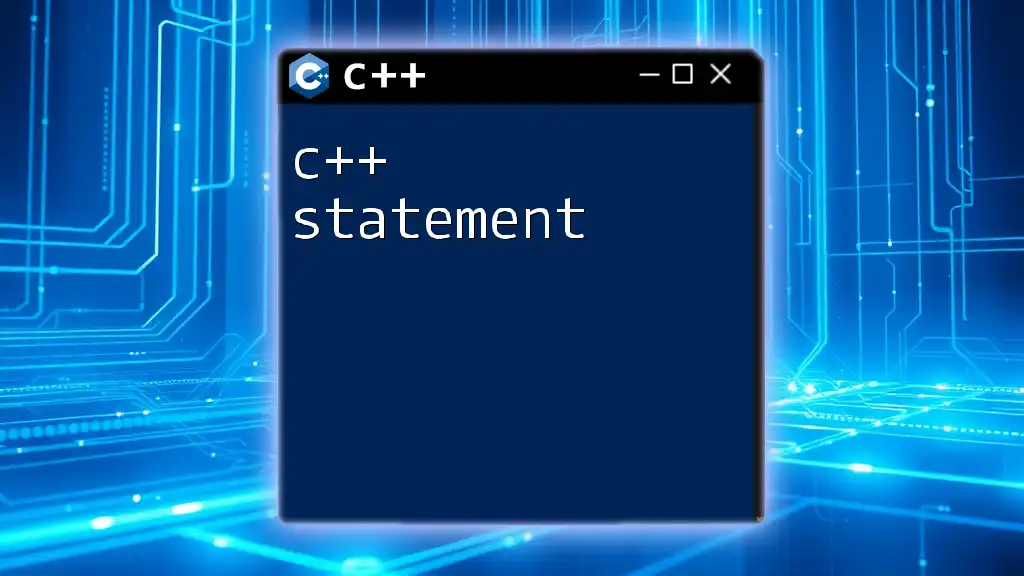
Additional Resources
Enhance your skill set through recommended courses or tutorials specializing in modern C++. Utilize online platforms and code repositories to engage with real-world C++ projects and deepen your understanding of this powerful language.