C++ name mangling is a process by which the compiler encodes additional information (such as function parameters) into the names of functions to enable function overloading and to ensure linkage of the correct function during compilation.
Here's a simple example demonstrating name mangling:
#include <iostream>
void func(int a) {
std::cout << "Function with int parameter: " << a << std::endl;
}
void func(double b) {
std::cout << "Function with double parameter: " << b << std::endl;
}
int main() {
func(5); // Calls func(int)
func(5.0); // Calls func(double)
return 0;
}
Understanding Name Mangling in C++
What is Name Mangling?
Name mangling is a process used by C++ compilers to encode additional information into the names of functions and variables. This encoding allows the compiler to generate unique names for functions that might otherwise conflict, especially when function overloading is involved. Essentially, name mangling helps to differentiate between functions based on their parameters and namespaces.
The importance of name mangling becomes evident when we consider how C++ supports features like function overloading, multiple namespaces, and templates. Without name mangling, the linker would struggle to resolve which function to call when multiple functions with the same name exist.
Historical Context
The evolution of C++ brought about features that are not present in languages like C, which leads to the necessity for name mangling. In C, a function's name alone is sufficient to identify it, as there is no support for overloading. However, as C++ designs emphasized polymorphism and the ability to differentiate similar function names, name mangling became an essential solution to ensure clear function resolution during compilation and linking.
When comparing C++ with languages that do not use name mangling, such as Java, which uses more runtime-based resolution techniques, C++ relies heavily on this compile-time strategy to manage function identities.
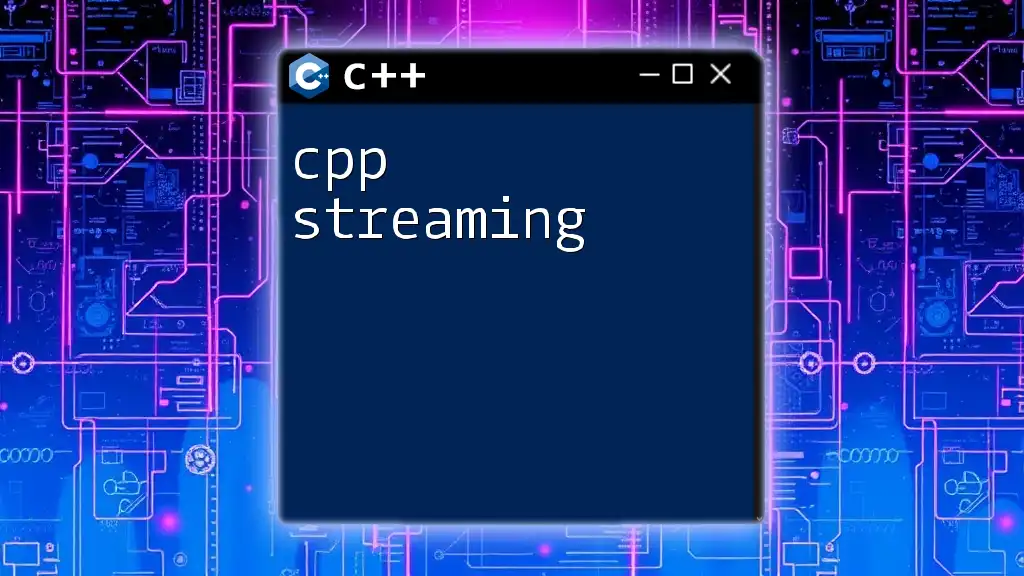
How Name Mangling Works
Basic Concepts of Name Mangling
At its core, name mangling serves to empower function overloading. In C++, it is entirely possible to declare multiple functions with share the same name as long as their parameter lists differ. For example, you can have two functions named `myFunction`, one that accepts an integer and another that accepts a double.
To address this, C++ compilers use a mechanism of decorated names. A decorated name is the mangled representation that includes information about the function name, its parameters, and possibly its namespace. This ensures that even when two functions have identical names, they can be resolved unambiguously by the linker.
Name Mangling Structure
The mangling scheme can vary between different compilers, but most follow a similar structure. A typical mangled name might include:
- The original function name.
- The types of the function parameters, presented in encoded form.
- Any additional information such as namespace or class context.
For example, consider the following class with overloaded functions:
#include <iostream>
using namespace std;
class MyClass {
public:
void myFunction(int a);
void myFunction(double b);
};
void MyClass::myFunction(int a) {
cout << "Integer: " << a << endl;
}
void MyClass::myFunction(double b) {
cout << "Double: " << b << endl;
}
In this case, the compiler might generate mangled names like:
- `MyClass::myFunction(int)` might be mangled to `_ZN8MyClass10myFunctionEi`
- `MyClass::myFunction(double)` might be mangled to `_ZN8MyClass10myFunctionEd`
This transformed output includes encoded information that tells the linker much more than just the function name.
Example of Name Mangling
#include <iostream>
using namespace std;
void myFunction(int a) {
cout << "Integer: " << a << endl;
}
void myFunction(double b) {
cout << "Double: " << b << endl;
}
For the functions above, the mangled names could look something like this in a particular compiler:
- `myFunction(int)` -> `_Z10myFunctioni`
- `myFunction(double)` -> `_Z10myFunctiond`
Practical Implications of Name Mangling
Cross-Compilation and Linking
When working with static and dynamic libraries, name mangling can play a critical role. Each time you compile a C++ program, the compilers produce different mangled names for functions, making it essential that these names precisely match when linking possible external libraries. If they don’t, you might run into "undefined reference" errors or issue during linkage.
Understanding how name mangling works can prevent conflicts when you attempt to use pre-compiled libraries. If the library you are trying to link against has a different mangling scheme than your application, you will not achieve successful linkage.
Debugging Challenges
Debugging can become particularly challenging due to name mangling. When analyzing a stack trace, you may see mangled names that are not immediately meaningful:
0x003f5f56 _Z10myFunctioni
To address this issue, you can use demangling tools. One popular tool for demangling C++ names is `c++filt`, which can convert mangled names back to their original format, allowing you to understand the function calls better.
Portability Considerations
Portability is another important consideration regarding name mangling. Different compilers may use different mangling schemes, which can lead to compatibility issues. For instance, a function compiled with GCC may have a different mangled name than the same function compiled with MSVC. This discrepancy can cause difficulties when developing cross-platform applications.
Efforts have been made to standardize name mangling to some degree in the C++ standards, but inconsistencies still exist. Thus, being aware of how your target compilers handle name mangling is crucial for successfully compiling and linking your applications.
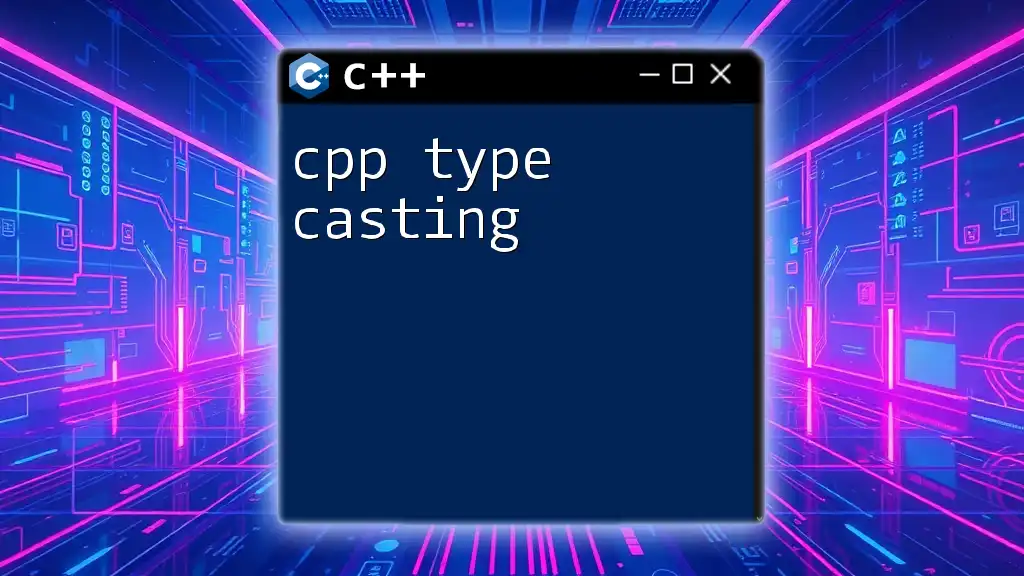
Advanced Topics in Name Mangling
Name Mangling in Templates
Template functions and classes also introduce complexity into the name mangling process. When a template is instantiated, the compiler generates unique mangled names for each specialization based on their type parameters.
For example:
template <typename T>
class MyTemplate {
public:
void myMethod(T param);
};
If you instantiate `MyTemplate<int>` and `MyTemplate<double>`, the compiler will create separate mangled names for `myMethod`, like `_ZN10MyTemplateIi11myMethodE` for `int` parameters and another one for `double`. This ensures that each type has a unique representation, allowing the linker to resolve references accurately.
Namespaces and Name Mangling
Namespaces further complicate the name mangling process. Namespaces help avoid naming conflicts, but they also influence the way functions are mangled. For example:
namespace MyNamespace {
void myFunction();
}
The mangled name in this case might look like `_ZN12MyNamespace10myFunctionEv`. Here, the namespace `MyNamespace` is included in the mangled name, ensuring that even if other libraries declare a `myFunction`, there will be no conflicts.
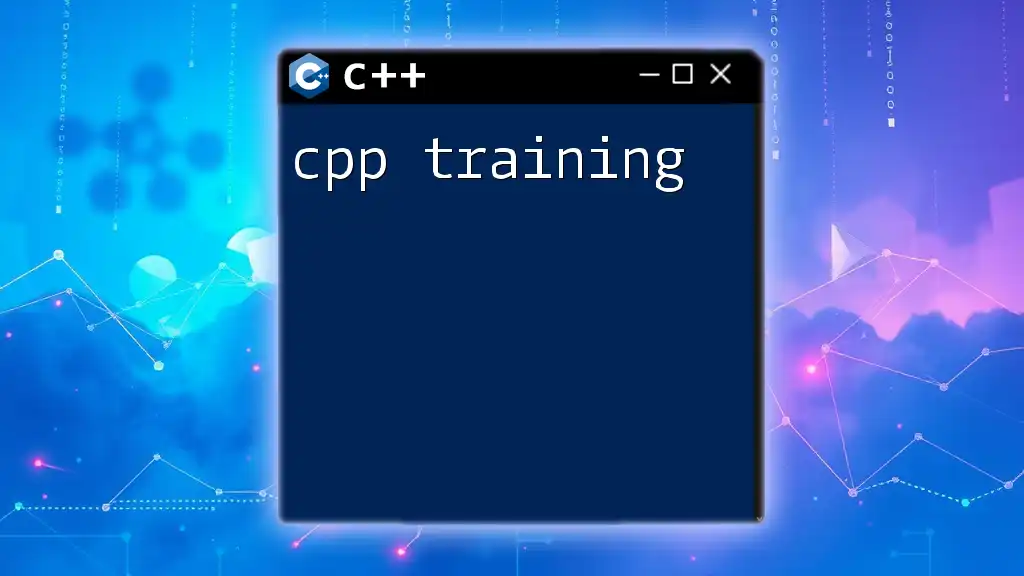
How to View Mangled Names
Using Compiler Options
Many modern compilers provide options to help you see the mangled names of objects and functions. For instance, in GCC and Clang, you can use the following command to generate the assembly output which contains mangled names:
g++ -S myfile.cpp
This command compiles `myfile.cpp` into an assembly file (`myfile.s`) where you can observe the mangled names in context.
Demangling in Practice
Using tools like `c++filt`, you can demangle names right in your terminal. For example, if you have a mangled name:
echo "_Z10myFunctioni" | c++filt
The output will be the original function name, making your debugging process much clearer.
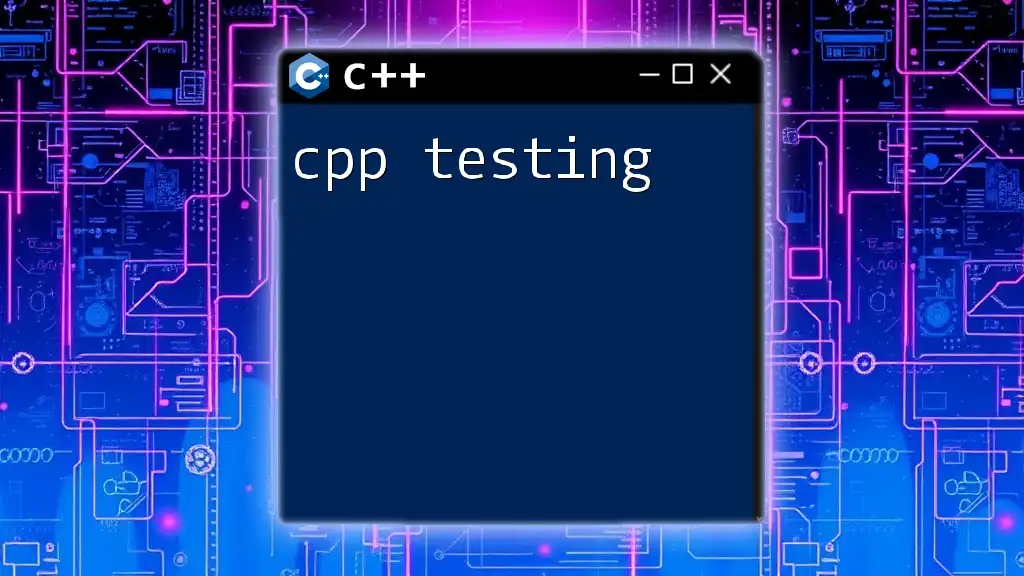
Conclusion
Understanding C++ name mangling is crucial for both new and experienced developers. From handling function overloading to navigating complex templates and namespaces, name mangling plays an integral role in ensuring smooth compilation and linking processes.
By digging deep into its mechanics and implications, you can enhance your ability to write robust C++ code. Explore further, and join our C++ command tutorials to unlock even more insights and enhance your programming skills in this powerful language.