A C++ palindrome is a string that reads the same forwards and backwards, and the following code snippet demonstrates how to check if a given string is a palindrome:
#include <iostream>
#include <string>
#include <algorithm>
bool isPalindrome(const std::string &str) {
std::string s = str;
std::transform(s.begin(), s.end(), s.begin(), ::tolower);
s.erase(std::remove_if(s.begin(), s.end(), ::isspace), s.end());
return std::equal(s.begin(), s.begin() + s.size()/2, s.rbegin());
}
int main() {
std::string input = "A man a plan a canal Panama";
std::cout << (isPalindrome(input) ? "Palindrome" : "Not a palindrome") << std::endl;
return 0;
}
Understanding Palindromes
What is a Palindrome?
A palindrome is a word, phrase, number, or other sequences of characters that reads the same forward and backward, ignoring spaces, punctuation, and capitalization. Classic examples include the words "level" and "radar," as well as phrases like "A man, a plan, a canal, Panama!"
Why Learn About Palindromes in C++?
Understanding palindromes is not just an academic exercise; it has numerous applications in real-world programming. Learning how to check for palindromes can enhance your string manipulation skills, deepen your understanding of algorithms, and even contribute to tasks like data validation and searching algorithms.
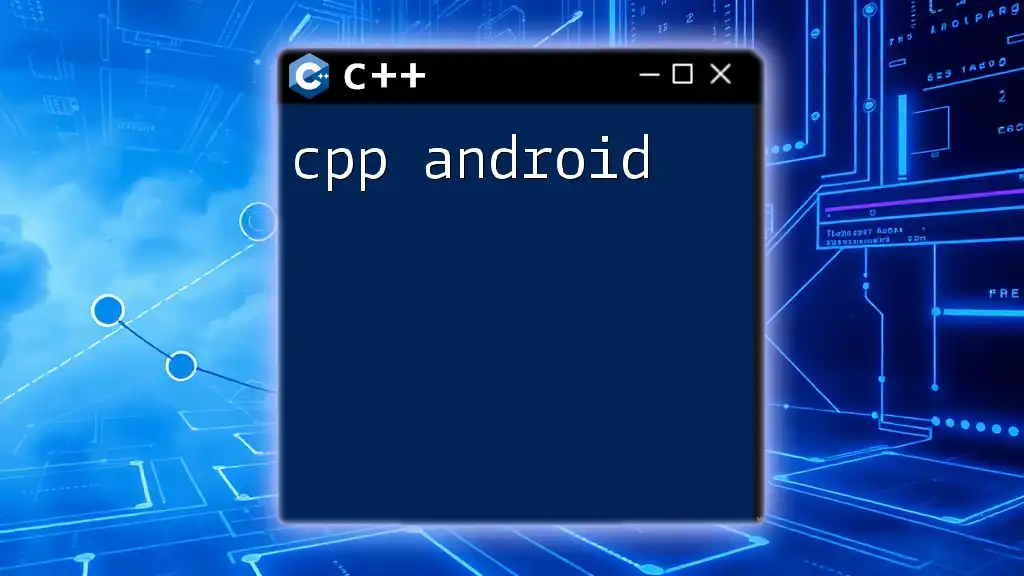
Is Palindrome in C++?
Basic Concept of Checking for Palindromes
The fundamental algorithm to check if a string is a palindrome involves comparing characters from opposite ends of the string, moving inward. The time complexity for this approach is O(n) because each character must be examined at least once, while the space complexity is O(1) as no additional data structures are required.
C++ Code Snippet: Simple Palindrome Check
Here’s a straightforward implementation of a palindrome checker in C++:
#include <iostream>
#include <string>
bool isPalindrome(const std::string& str) {
int left = 0;
int right = str.length() - 1;
while (left < right) {
if (str[left] != str[right]) {
return false;
}
left++;
right--;
}
return true;
}
int main() {
std::string input = "racecar";
std::cout << std::boolalpha << isPalindrome(input) << std::endl;
return 0;
}
In this code snippet, the `isPalindrome` function efficiently checks if the provided string is palindromic. It uses two indices, `left` and `right`, to compare characters from both ends of the string until they meet in the middle. If any mismatch is found, it immediately returns `false`, ensuring efficiency in execution.
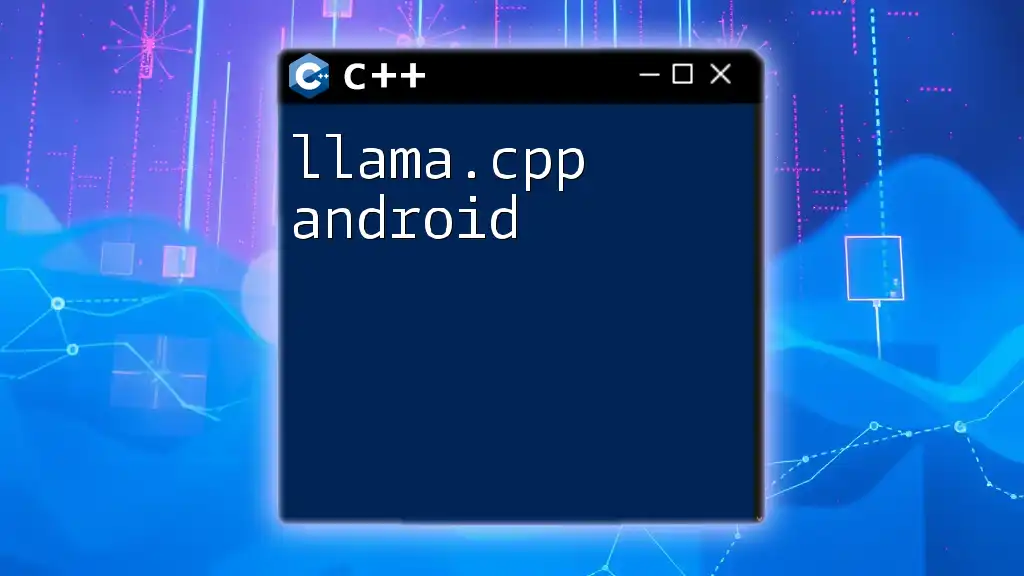
Palindrome in C++ with Edge Cases
Handling Different Cases
One challenge with palindrome checks is ensuring that character case does not affect the outcome. By converting all characters to a common case (either lower or upper), we can ensure a more accurate comparison.
Here’s an improved version of our palindrome check that is case-insensitive:
#include <algorithm>
bool isPalindromeCaseInsensitive(const std::string& str) {
std::string modifiedStr = str;
std::transform(modifiedStr.begin(), modifiedStr.end(), modifiedStr.begin(), ::tolower);
return isPalindrome(modifiedStr); // Use previously defined function
}
In this implementation, the `std::transform` function is employed to convert all characters to lowercase before checking for a palindrome, thus enabling a more rigorous assessment.
Ignoring Spaces and Special Characters
In addition to case sensitivity, we must consider non-alphanumeric characters, particularly when dealing with phrases. Spaces and punctuations can interfere with our palindrome checks. Here’s how to enhance our function:
#include <cctype>
bool isAlphanumeric(char c) {
return std::isalnum(c);
}
bool isPalindromeIgnoringSpaces(const std::string& str) {
std::string filteredStr;
for (char c : str) {
if (isAlphanumeric(c)) {
filteredStr += std::tolower(c);
}
}
return isPalindrome(filteredStr);
}
In this code, we build a new string containing only the alphanumeric characters of the original string. The `isAlphanumeric` function is used for filtering, and then `isPalindrome` checks the filtered string. This makes the palindrome check robust against irrelevant characters.
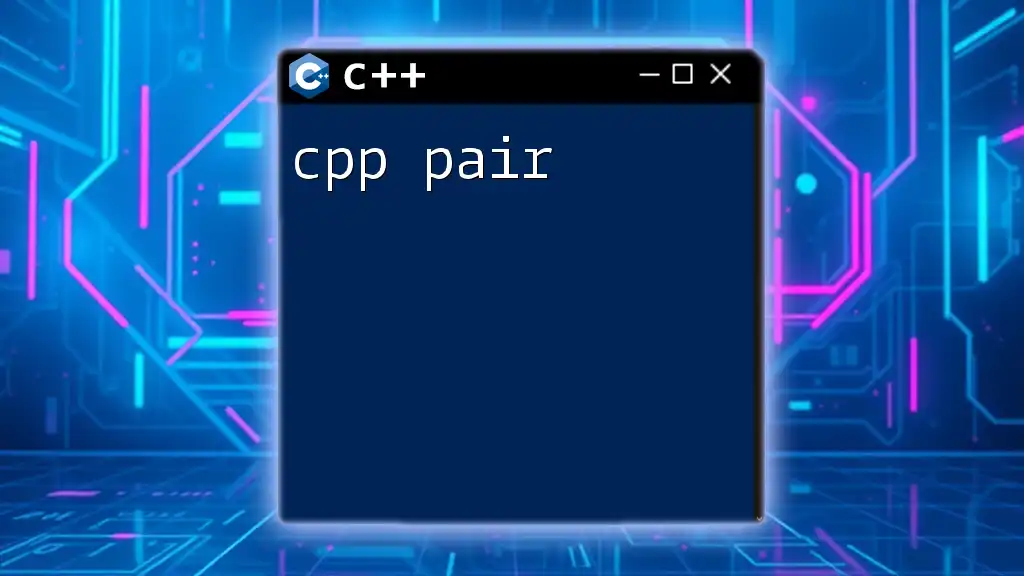
Palindrome in CPP: Advanced Techniques
Using Recursion to Check for Palindromes
The recursive approach presents an elegant alternative for implementing palindrome checks. It works by comparing the outer characters while invoking itself on the inner substring:
bool isPalindromeRecursive(const std::string& str, int left, int right) {
if (left >= right) return true;
return (str[left] == str[right]) && isPalindromeRecursive(str, left + 1, right - 1);
}
// Usage
std::cout << std::boolalpha << isPalindromeRecursive("racecar", 0, 6) << std::endl;
This function checks if the characters at the given `left` and `right` indices are equal, subsequently calling itself with the next inner indices. This technique offers a different perspective on the palindrome problem and showcases the power of recursion in C++.
Using STL Functions for Palindrome Checks
C++ provides rich libraries through the Standard Template Library (STL), allowing for more concise code. Here’s how you can implement a palindrome check using STL functions:
#include <algorithm>
bool isPalindromeSTL(const std::string& str) {
std::string modifiedStr = str;
std::transform(modifiedStr.begin(), modifiedStr.end(), modifiedStr.begin(), ::tolower);
modifiedStr.erase(std::remove_if(modifiedStr.begin(), modifiedStr.end(), [](char c) {
return !std::isalnum(c);
}), modifiedStr.end());
return std::equal(modifiedStr.begin(), modifiedStr.begin() + modifiedStr.size()/2,
modifiedStr.rbegin());
}
In this example, we transform the string to lowercase, eliminate non-alphanumeric characters with `std::remove_if`, and finally, utilize `std::equal` to compare the first half of the string with the reversed second half, achieving an efficient palindrome check in fewer lines of code.
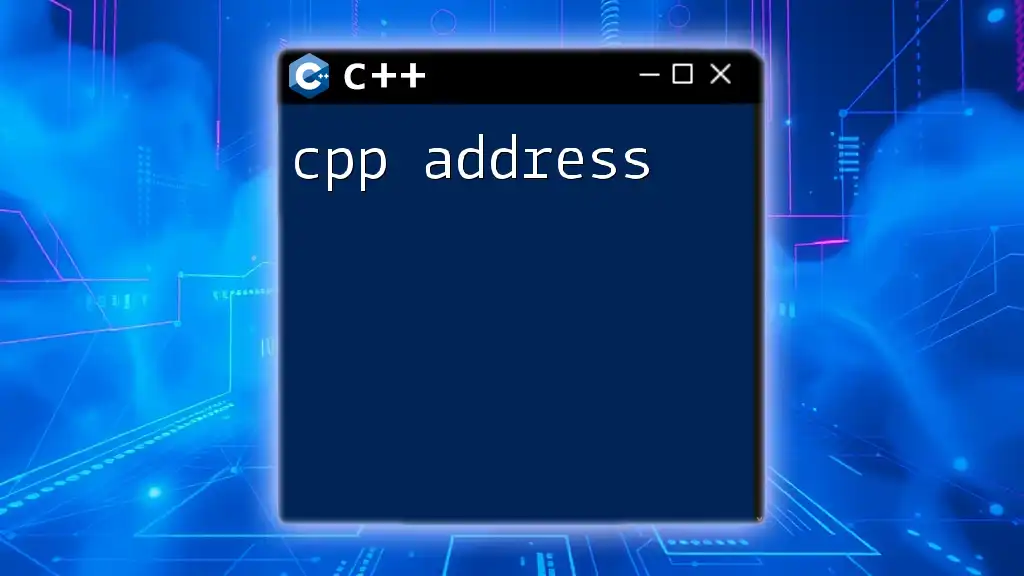
Real-World Applications of Palindrome Checks
Why Knowing Palindromes Can Be Useful
The ability to check for palindromes can be incredibly useful in various programming applications. For instance, it can serve as a fundamental component in data validation, ensuring that certain input criteria are met. Furthermore, palindrome checks can enhance search algorithms, particularly in text analysis or natural language processing where identifying palindromic structures may have semantic significance.
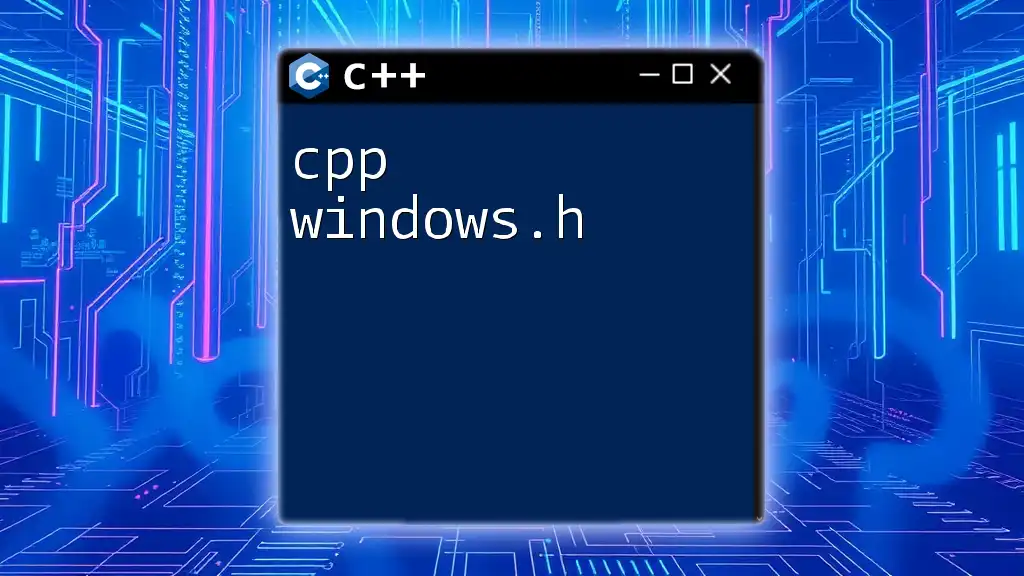
Conclusion
Understanding cpp palindrome checks is essential for any aspiring C++ programmer. By mastering the different techniques for identifying palindromes—from basic string comparison to leveraging STL functions—you lay a solid foundation for more complex programming tasks.
Encouraging you to put these principles into practice, consider implementing your own variations of palindrome checks. Whether you are handling strings, phrases, or even sets of numbers, the possibilities are endless! If you have experiences or questions about implementing palindrome checks in C++, feel free to share them and join the discussion!
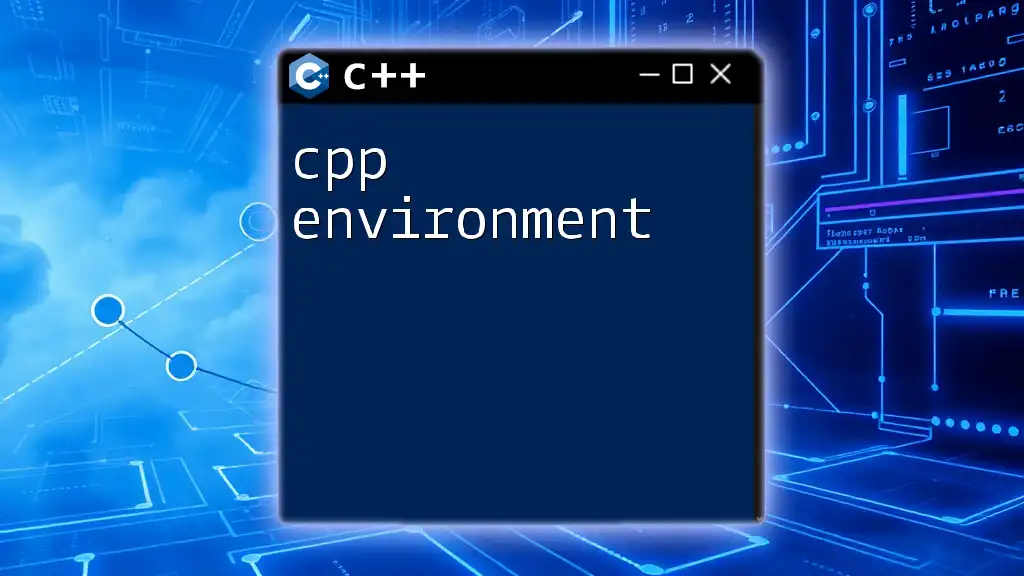
Additional Resources
To further enhance your C++ skills, consider exploring books, online courses, or websites dedicated to programming and algorithms. The C++ Standard Library documentation can also provide invaluable insights into string manipulation and algorithm utilization.